


SlideView picture sliding (expansion/shrinking) display effect_javascript skills
May 16, 2016 pm 06:21 PMThis is actually an improved version of the picture sliding display effect I wrote before. It was my first article that attracted more attention. It’s time to sort it out.
has the following features:
1, there are four direction modes to choose from;
2, combined with the tween algorithm to achieve a variety of sliding effects;
3, can automatically calculate the display size based on sliding elements;
4. You can also customize the display or shrink the size;
5. The automatic switching function can be expanded;
6. The sliding prompt function can be expanded.
Compatible with: ie6/7/8, firefox 3.6.8, opera 10.51, safari 4.0.5, chrome 5.0
Program description
[Basic principles]
By setting the position coordinates of the sliding element (left/right/top/bottom), the target element entered by the mouse can be displayed slidingly, and other elements can slide and shrink.
The difficulty is how to control multiple sliding elements to perform different sliding at the same time. The key here is to decompose the overall sliding into individual sliding elements for their own sliding.
The method is to set the target value for each sliding element, and then slide each one towards its own target value. When all of them reach the target value, it is completed.
[Container Settings]
Configure the container in the _initContainer method. Since the container will be used in the calculation of the sliding parameters later, the container must be set first.
Set the container style first. To achieve sliding, you need to set relative or absolute positioning of the container, and set overflow to "hidden" to fix the container size. The sliding element must also be set to absolute positioning.
The _LEAVE removal function will be triggered when the mouse moves out of the container:
$$E.addEvent( container, "mouseleave", this._LEAVE );
The _LEAVE function It is like this:
Code
var CLOSE = $$F.bind( this.close, this );
this._LEAVE = $$F.bind( function(){
clearTimeout(this._timerDelay);
$$CE.fireEvent( this, "leave" );
if ( this.autoClose ) { this._timerDelay = setTimeout( CLOSE, this.delay ); }
}, this );
The close method will be triggered with a delay when the autoClose attribute is true.
[Sliding Object]
When the program is initialized, a sliding object collection will be created based on the sliding element.
Get the sliding element first:
var nodes = opt.nodes ? $$A.map( opt.nodes, function(n) { return n; } )
: $$ A.filter( container.childNodes, function(n) { return n.nodeType == 1; });
If there is no custom nodes sliding element, get the childNodes from the container as the sliding element.
Also use nodeType to filter, because browsers other than IE will use spaces as part of childNodes.
Then use the obtained sliding elements to generate the _nodes sliding object collection required by the program:
this._nodes = $$A.map( nodes, function(node){ return { "node" : node }; });
The sliding object records the sliding element using the "node" attribute.
Then initialize the sliding object in the _initNodes method.
Each sliding object has 3 attributes used to calculate the sliding target value: defaultTarget default target value, max display size, and min shrink size.
If there is a custom max size or min size, it will be calculated based on the custom size.
The program will give priority to calculation based on max:
max = Math.max( max <= 1 ? max * clientSize : Math.min( max, clientSize ), defaultSize );
min = (clientSize - max) / maxIndex;
Where clientSize is the visible area size of the container, and defaultSize is the average allocation size.
If max is a decimal or 1, calculate it as a percentage, and then limit the size to the range from defaultSize to clientSize.
Then calculate the average size of other shrinking elements after subtracting max, and you can get min.
If there is no custom max, then calculate it according to the custom min:
min = Math.min( min < 1 ? min * clientSize : min, defaultSize );
max = clientSize - maxIndex * min;
Similarly, if min is a decimal, calculate it as a percentage, then limit the range, and then calculate max.
Finally get the custom size calculation function:
getMax = function(){ return max; };
getMin = function(){ return min; };
If there is no customized max or min, it is calculated based on the element size:
getMax = function(o){ return Math.max( Math.min( o.node[ offset ], clientSize ) , defaultSize ); };
getMin = function(o){ return ( clientSize - o.max ) / maxIndex; };
Calculate the element size as the display size, also limit the range, and then calculate the shrinkage size.
After getting the size calculation function, use the _each method to traverse and set the sliding object:
o.current = o.defaultTarget = getDefaultTarget(i);
o .max = getMax(o); o.min = getMin(o);
where current is the current coordinate value, which is used as the starting value during movement calculation.
The defaultTarget is the default target value, that is, the target value of movement in the default state, which is obtained based on defaultSize and index.
Also set the show display function to be triggered when the mouse enters the sliding element:
Code
var node = o.node, SHOW = $$F.bind( this. show, this, i );
o.SHOW = $$F.bind( function(){
clearTimeout(this._timerDelay);
this._timerDelay = setTimeout( SHOW, this.delay );
$$CE.fireEvent( this, "enter", i );
}, this );
$$E.addEvent( node, "mouseenter", o.SHOW );
To Trigger in the "mouseenter" event of the sliding element, and pass the index of the current sliding object, plus the delay setting.
[Sliding Display]
When the mouse enters one of the sliding elements, the show method will be triggered to start displaying.
First execute the _setMove method to set the sliding parameters and use the index as the parameter.
In _setMove, you mainly set the target value, start value and change value required when calculating the movement value.
Correct the index first, the wrong index value will be set to 0:
this._index = index = index < 0 || index > maxIndex ? 0 : index | 0;
Then obtain the sliding object to be displayed according to the index, and get the getTarget target value function through the min and max of the displayed object:
var nodeShow = nodes[ index ], min = nodeShow.min, max = nodeShow .max;
getTarget = function(o, i){ return i <= index ? min * i : min * ( i - 1 ) max; };
If the sliding object is the display object or is displaying the object Previously, the target value is min * i, because the target value of the i-th sliding object is the size of i min.
Otherwise, the target value is min * ( i - 1 ) max, which actually means changing the position of the display object to max.
Then set the parameter attributes of each sliding object:
this._each( function(o, i){
o.target = getTarget(o, i);
o.begin = o.current;
o.change = o.target - o.begin;
});
where target records the target value, and begin gets the start value and target value through current The difference from the starting value is the change value.
After the setting is completed, execute the _easeMove method to start sliding, reset the _time attribute to 0, and then execute the _move program to officially start moving.
First determine whether _time reaches the duration. If not, continue moving.
The program sets up a _tweenMove movement function to set easing:
this._setPos( function(o) {
return this.tween( this._time, o. begin, o.change, this.duration );
});
Using the tween algorithm, combined with the current time, start value, change value and duration, you can get the current coordinate value to be moved.
ps: Regarding tween easing, you can refer to the tween algorithm and easing effects.
When _time reaches duration, it means that the sliding has been completed. Execute the _targetMove target value movement function again:
this._setPos( function(o) { return o.target; } );
Moving directly to the target value can prevent possible calculation errors from causing inaccurate shifts.
[Close and reset]
The close method can close the display, that is, slide it to the default state, which will be executed when the container is moved out.
The default state refers to the state where all sliding elements are located at the default target value of defaultTarget.
First use _setMove to set the movement parameters. When _setMove has no index parameter, the target value will be set to the default target value:
getTarget = function(o){ return o.defaultTarget; }
After completing parameter settings, execute _easeMove to slide, similar to sliding display.
The reset method can reset the display. Reset means moving directly to the target value without sliding.
If there is no index parameter, the _defaultMove default value movement function will be executed directly:
this._setPos( function(o) { return o.defaultTarget; } );
Directly Sliding elements move to their default state.
If there is an index parameter, first use _setMove to set the target value according to the index, and then execute _targetMove to move directly to the target value.
After the program is initialized, a reset will be performed and a custom defaultIndex will be used as a parameter.
Use defaultIndex to display the sliding object corresponding to the index from the beginning.
[Direction Mode]
The program can customize the direction mode. There are four direction modes: bottom, top, right, left (default).
The right and left slide in the horizontal direction, while the bottom and top slide in the vertical direction.
The difference between right and left is that the direction of the fixed point is different. Left uses the left as the fixed point to slide on the right, while right does the opposite.
You should understand it by referring to specific examples. The difference between bottom and top is also similar.
The program is implemented by modifying the coordinate style of the corresponding direction for different directions.
For example, left mode uses the "left" style to create movement effects, and top mode uses the "top" style.
The _pos attribute set in the initialization program is used to record the coordinate style to be used in the current mode:
this._pos = /^(bottom|top|right|left)$/ .test( opt.mode.toLowerCase() ) ? RegExp.$1 : "left";
Then use the coordinate style specified by _pos in the _setPos method to set the coordinate value:
var pos = this._pos;
this._each( function(o, i) {
o.node.style[ pos ] = (o.current = Math.round(method.call( this, o ))) " px";
});
The _horizontal attribute records whether it slides horizontally, that is, whether it is right or left.
Use it to specify whether to use horizontal or vertical dimensions when calculating dimensions.
There is also a _reverse attribute to determine whether it is bottom or right mode.
<div class="container"> ;
<div style="right:0;">2</div>
<div style="right:100px;">1</div>
<div style ="right:200px;">0</div>
</div>
But this requires modifying the dom structure, or setting the stacking order through zIndex:
<div class="container">
<div style ="right:200px;z-index:3;">0</div>
<div style="right:100px;z-index:2;">1</div>
<div style="right:0;z-index:1;">2</div>
</div>
Obviously the way to set zIndex is better, program This method was also used.
The program uses the _reverse attribute to determine whether these corrections need to be made.
First, in _initContainer, reset the zIndex according to _reverse:
var zIndex = 100, gradient = this._reverse ? -1 : 1;
this._each( function(o){
var style = o.node.style;
style.position = "absolute"; style.zIndex = zIndex = gradient;
});
In _initNodes, you must also judge when getting the default target value:
getDefaultTarget = this._reverse
? function(i){ return defaultSize * ( maxIndex - i ); }
: function(i){ return defaultSize * i; },
when_reverse When it is true, since the fixed-point position is in the opposite direction of the index, the element should be set in the opposite direction, so maxIndex should be used to subtract it.
In _setMove, when setting the sliding target value according to the index, you must also judge:
if ( this._reverse ) {
var get = getTarget;
index = maxIndex - index;
getTarget = function(o, i){ return get( o, maxIndex - i ); }
}
Not only the index of the sliding object collection needs to be modified, but also the index of the display object needs to be modified.
[Automatic display expansion]
This expansion uses the combination mode. For the principle, please refer to the expansion mode part of the ImageZoom expansion article.
The difference is that a property extension is added to add extension methods:
$$.extend( this, prototype );
Note that it cannot be added to SlideView.prototype, as this will Affects the structure of SlideView.
"Automatic display" aims to realize the automatic rotation display of sliding objects, and cancel the default state and implement forced display, which can be used for the rotation display of pictures.
As long as you add the automatic display extension behind the SlideView and set the auto parameter to true, it will be enabled.
The principle is also very simple, that is, after each slide/move is completed, just use the timer to perform the next slide.
First, add a _NEXT program in the "init" initialization program to display the next sliding object:
this._NEXT = $$F.bind( function( ){ this.show( this._index 1 ); }, this );
In fact, it is to add 1 to the current index _index and execute it as a parameter of show.
Add another _autoNext method:
if ( !this._autoPause ) {
clearTimeout(this._autoTimer);
this._autoTimer = setTimeout( this._NEXT, this.autoDelay );
}
The function is to delay the execution of the _NEXT program, and there is an _autoPause attribute to lock the execution.
Then set several places that need to be executed.
First, in the "finish" sliding event, execute the _autoNext method, thus realizing basic automatic display.
After the mouse enters the sliding element, automatic switching should stop, so in the event of "enter" entering the sliding element, the timer will be cleared and _autoPause set to true to lock.
Correspondingly, in the "leave" event when the mouse leaves the container, set _autoPause back to false to unlock it, and then execute the _autoNext method to restart the automatic program.
And set autoClose to false in "leave" to prevent automatic restoration of the default state.
Finally, reset must be rewritten:
reset.call( this, index == undefined ? this._index : index );
this._autoNext();
The rewritten reset will force the index to be displayed and execute _autoNext for the next slide.
[Prompt information expansion]
The "prompt information" effect means that each sliding object corresponds to a layer (element) of prompt information (content).
This prompt message will be displayed when the sliding object is displayed, and will be closed when it is contracted and closed.
As long as you add the tip extension and set the tip parameter to true, it will be enabled.
The prompt extension supports four position prompts: bottom, top, right, and left.
In "init", get the _tipPos coordinate style according to the custom tipMode:
this._tipPos = /^(bottom|top|right|left)$/.test( this. options.tipPos.toLowerCase() ) ? RegExp.$1 : "bottom";
Then define a function in "initNodes" that can get the tip element based on the sliding element:
var opt = this.options, tipTag = opt.tipTag, tipClass = opt.tipClass,
re = tipClass && new RegExp("(^|\s)" tipClass "(\s|$)"),
getTipNode = function(node){
var nodes = node.getElementsByTagName( tipTag );
if ( tipClass ) {
nodes = $$A.filter( nodes, function(n){ return re.test(n.className); } );
}
return nodes [0];
};
If tipTag is customized, elements will be obtained based on the tag, otherwise all elements will be obtained according to the default value "*".
If tipClass is customized, elements will be filtered based on className. Note that it may contain multiple styles and cannot be directly equal.
After getting the function, create the prompt object:
this._each( function(o) {
var node = o.node, tipNode = getTipNode(node);
node.style.overflow = "hidden";
tipNode.style.position = "absolute"; tipNode.style.left = 0;
o.tip = {
"node": tipNode,
"show": tipShow != undefined ? tipShow : 0,
"close": tipClose != undefined ? tipClose : -tipNode[offset]
};
});
Get the tip element first, and set the relevant style, and then give Add a tip attribute to the sliding object and save the corresponding prompt object.
The "node" attribute saves the prompt element, "show" is the coordinate value when displayed, and "close" is the coordinate value when closed.
If tipShow is not customized, the default display coordinate value is 0, that is, the prompt element is just attached to the edge of the sliding element;
If tipClose is not customized, the default coordinate value when closed is the size of the prompt element, that is, The tooltip element is hidden just outside the sliding element.
Set the prompt movement target value in "setMove":
var maxIndex = this._nodes.length - 1;
this._each( function(o, i) {
var tip = o.tip;
if ( this. _reverse ) { i = maxIndex -i; }
tip.target = index == undefined || index != i ? tip.close : tip.show;
tip.begin = tip.current; tip.change = tip.target - tip.begin;
});
This is much simpler than the setting of the sliding object. When the index parameter is set, and the index is equal to the index of the sliding object It needs to be shown, otherwise it is hidden.
It should be noted that, like the sliding object, the index needs to be corrected when _reverse is true.
The corresponding movement functions must also be set in "tweenMove", "targetMove", and "defaultMove".
In order to facilitate style setting, a _setTipPos method has been extended:
var pos = this._tipPos;
this._each( function(o, i) {
var tip = o.tip;
tip.node.style[ pos ] = (tip.current = method.call( this, tip )) "px";
});
Set coordinate values according to _tipPos coordinate style.
Usage Tips
[Display Size]
To customize the display size, you can set it through max and min, which can be calculated in pixels or percentages.
If not set, it will be displayed according to the size of the element itself.
So the size displayed by the sliding element does not need to be consistent, the program can automatically calculate it.
[Accordion effect]
Accordion is a collapsible panel control with an effect similar to an accordion. SlideView can also achieve similar effects through settings.
First set autoClose to false to cancel automatic closing, and then set defaultIndex so that SlideView will not close in the expanded state.
Generally Accordion has a fixed size title, which can be set using min.
In this way, a simple Accordion effect is achieved. Please refer to the third example for details.
Instructions for use
When instantiating, there must be a container object or id as a parameter:
new SlideView( "idSlideView" );
Optional parameters are used to set the default attributes of the system, including:
Attribute: Default value//Description
nodes: null,//Custom display element collection
mode: "left",/ /Direction
max: 0, //Display size (pixels or percentage)
min: 0, //Shrink size (pixels or percentage)
delay: 100, //Trigger delay
interval : 20,//Sliding interval
duration: 20,//Sliding duration
defaultIndex: null,//Default display index
autoClose: true,//Whether to automatically restore
tween: function( t,b,c,d){ return -c * ((t=t/d-1)*t*t*t - 1) b; },//tween operator
onShow: function(index) {}, //Executed when sliding display
onClose: function(){}//Executed when sliding close
The interval, delay, duration, tween, autoClose, onShow, and onClose attributes can be set dynamically after the program is initialized.
also provides the following methods:
show: sliding display according to the index;
close: sliding to the default state;
reset: resetting to the default state or expanding the sliding object corresponding to the index;
dispose: Destroy the program.
To use automatic display, just add the automatic display extension behind SlideView and set the auto parameter to true.
Add the following optional parameters:
autoDelay: 2000//Display time
To use prompt information, just add the prompt information extension and set the tip parameter to true.
Add the following optional parameters:
Attribute: Default value//Description
tipPos: "bottom",//Tip position
tipTag: "*",//Tip element tag
tipClass: "",//Prompt element style
tipShow: null,//Target coordinates when displaying
tipClose: null//Target coordinates when closing
Program source code
var SlideView = function(container, options){
this._initialize( container, options );
this._initContainer();
this._initNodes();
this.reset( this.options.defaultIndex );
};
SlideView.prototype = {
//初始化程序
_initialize: function(container, options) {
var container = this._container = $$(container);//容器对象
this._timerDelay = null;//延迟计时器
this._timerMove = null;//移动计时器
this._time = 0;//时间
this._index = 0;//索引
var opt = this._setOptions(options);
this.interval = opt.interval | 0;
this.delay = opt.delay | 0;
this.duration = opt.duration | 0;
this.tween = opt.tween;
this.autoClose = !!opt.autoClose;
this.onShow = opt.onShow;
this.onClose = opt.onClose;
//设置参数
var pos =this._pos = /^(bottom|top|right|left)$/.test( opt.mode.toLowerCase() ) ? RegExp.$1 : "left";
this._horizontal = /right|left/.test( this._pos );
this._reverse = /bottom|right/.test( this._pos );
//获取滑动元素
var nodes = opt.nodes ? $$A.map( opt.nodes, function(n) { return n; } )
: $$A.filter( container.childNodes, function(n) { return n.nodeType == 1; });
//创建滑动对象集合
this._nodes = $$A.map( nodes, function(node){
var style = node.style;
return { "node": node, "style": style[pos], "position": style.position, "zIndex": style.zIndex };
});
//设置程序
this._MOVE = $$F.bind( this._move, this );
var CLOSE = $$F.bind( this.close, this );
this._LEAVE = $$F.bind( function(){
clearTimeout(this._timerDelay);
$$CE.fireEvent( this, "leave" );
if ( this.autoClose ) { this._timerDelay = setTimeout( CLOSE, this.delay ); }
}, this );
$$CE.fireEvent( this, "init" );
},
//设置默认属性
_setOptions: function(options) {
this.options = {//默认值
nodes: null,//自定义展示元素集合
mode: "left",//方向
max: 0,//展示尺寸(像素或百分比)
min: 0,//收缩尺寸(像素或百分比)
delay: 100,//触发延时
interval: 20,//滑动间隔
duration: 20,//滑动持续时间
defaultIndex: null,//默认展示索引
autoClose: true,//是否自动恢复
tween: function(t,b,c,d){ return -c * ((t=t/d-1)*t*t*t - 1) b; },//tween算子
onShow: function(index){},//滑动展示时执行
onClose: function(){}//滑动关闭执行
};
return $$.extend(this.options, options || {});
},
//设置容器
_initContainer: function() {
//容器样式设置
var container = this._container, style = container.style, position = $$D.getStyle( container, "position" );
this._style = { "position": style.position, "overflow": style.overflow };//备份样式
if ( position != "relative" && position != "absolute" ) { style.position = "relative"; }
style.overflow = "hidden";
//移出容器时
$$E.addEvent( container, "mouseleave", this._LEAVE );
//设置滑动元素
var zIndex = 100, gradient = this._reverse ? -1 : 1;
this._each( function(o){
var style = o.node.style;
style.position = "absolute"; style.zIndex = zIndex = gradient;
});
$$CE.fireEvent( this, "initContainer" );
},
//设置滑动对象
_initNodes: function() {
var len = this._nodes.length, maxIndex = len - 1,
type = this._horizontal ? "Width" : "Height", offset = "offset" type,
clientSize = this._container[ "client" type ],
defaultSize = Math.round( clientSize / len ),
//计算默认目标值的函数
getDefaultTarget = this._reverse
? function(i){ return defaultSize * ( maxIndex - i ); }
: function(i){ return defaultSize * i; },
max = this.options.max, min = this.options.min, getMax, getMin;
//设置参数函数
if ( max > 0 || min > 0 ) {//自定义参数值
//小数按百分比设置
if ( max > 0 ) {
max = Math.max( max <= 1 ? max * clientSize : Math.min( max, clientSize ), defaultSize );
min = ( clientSize - max ) / maxIndex;
} else {
min = Math.min( min < 1 ? min * clientSize : min, defaultSize );
max = clientSize - maxIndex * min;
}
getMax = function(){ return max; };
getMin = function(){ return min; };
} else {//根据元素尺寸设置参数值
getMax = function(o){ return Math.max( Math.min( o.node[ offset ], clientSize ), defaultSize ); };
getMin = function(o){ return ( clientSize - o.max ) / maxIndex; };
}
//设置滑动对象
this._each( function(o, i){
//移入滑动元素时执行程序
var node = o.node, SHOW = $$F.bind( this.show, this, i );
o.SHOW = $$F.bind( function(){
clearTimeout(this._timerDelay);
this._timerDelay = setTimeout( SHOW, this.delay );
$$CE.fireEvent( this, "enter", i );
}, this );
$$E.addEvent( node, "mouseenter", o.SHOW );
//Calculate size
o.current = o.defaultTarget = getDefaultTarget(i);//Default Target value
o.max = getMax(o); o.min = getMin(o);
});
$$CE.fireEvent( this, "initNodes" );
},
//Sliding display based on index
show: function(index) {
this._setMove( index | 0 );
this.onShow( this._index );
this._easeMove();
},
//Slide to the default state
close: function() {
this._setMove();
this.onClose();
this._easeMove();
},
//Reset to the default state or expand the index corresponding sliding object
reset: function(index) {
clearTimeout(this._timerDelay);
if ( index == undefined ) {
this._defaultMove();
} else {
this._setMove(index);
this.onShow( this._index );
this._targetMove();
}
},
//Set sliding parameters
_setMove: function(index) {
var setTarget;//Set target value function
if ( index == undefined ) {//Set the default state target value
getTarget = function(o){ return o.defaultTarget; }
} else {//Set the sliding target value according to the index
var nodes = this._nodes, maxIndex = nodes.length - 1;
//Set index
this._index = index = index < 0 || index > maxIndex ? 0 : index | 0;
//Set display parameters
var nodeShow = nodes[ index ], min = nodeShow.min, max = nodeShow.max;
getTarget = function(o, i){ return i <= index ? min * i : min * ( i - 1 ) max; };
if ( this._reverse ) {
var get = getTarget;
index = maxIndex - index;
getTarget = function(o, i) { return get( o, maxIndex - i ); }
}
}
this._each( function(o, i){
o.target = getTarget(o, i);// Set target value
o.begin = o.current;//Set start value
o.change = o.target - o.begin;//Set change value
});
$$ CE.fireEvent( this, "setMove", index );
},
//Slip program
_easeMove: function() {
this._time = 0; this._move ();
},
//Move program
_move: function() {
if ( this._time < this.duration ){//Not reached
this._tweenMove( );
this._time ;
this._timerMove = setTimeout( this._MOVE, this.interval );
} else {//Complete
this._targetMove();//Prevent calculation errors
$$CE.fireEvent( this, "finish" );
}
},
//tween move function
_tweenMove: function() {
this. _setPos( function(o) {
return this.tween( this._time, o.begin, o.change, this.duration );
});
$$CE.fireEvent( this, " tweenMove" );
},
//Target value movement function
_targetMove: function() {
this._setPos( function(o) { return o.target; } );
$$CE.fireEvent( this, "targetMove" );
},
//Default value move function
_defaultMove: function() {
this._setPos( function(o) { return o .defaultTarget; } );
$$CE.fireEvent( this, "defaultMove" );
},
//Set coordinate value
_setPos: function(method) {
clearTimeout( this._timerMove);
var pos = this._pos;
this._each( function(o, i) {
o.node.style[ pos ] = (o.current = Math.round( method.call( this, o ))) "px";
});
},
//Traverse the sliding object collection
_each: function(callback) {
$$A.forEach( this._nodes, callback, this );
},
//Destroy the program
dispose: function() {
clearTimeout(this._timerDelay);
clearTimeout(this._timerMove);
$$CE.fireEvent( this, "dispose" );
var pos = this._pos;
this._each( function (o) {
var style = o.node.style;
style[pos] = o.style; style.zIndex = o.zIndex; style.position = o.position;//Restore style
$$E.removeEvent( o.node, "mouseenter", o.SHOW ); o.SHOW = o.node = null;
});
$$E.removeEvent( this._container, " mouseleave", this._LEAVE );
$$D.setStyle( this._container, this._style );
this._container = this._nodes = this._MOVE = this._LEAVE = null;
$$CE.clearEvent( this );
}
};
Automatically display extensions
SlideView.prototype._initialize = (function(){
var init = SlideView.prototype._initialize,
reset = SlideView.prototype.reset,
methods = {
"init": function(){
this.autoDelay = this.options.autoDelay | 0;
this._autoTimer = null;//定时器
this._autoPause = false;//暂停自动展示
//展示下一个滑动对象
this._NEXT = $$F.bind( function(){ this.show( this._index 1 ); }, this );
},
"leave": function(){
this.autoClose = this._autoPause = false;
this._autoNext();
},
"enter": function(){
clearTimeout(this._autoTimer);
this._autoPause = true;
},
"finish": function(){
this._autoNext();
},
"dispose": function(){
clearTimeout(this._autoTimer);
}
},
prototype = {
_autoNext: function(){
if ( !this._autoPause ) {
clearTimeout(this._autoTimer);
this._autoTimer = setTimeout( this._NEXT, this.autoDelay );
}
},
reset: function(index) {
reset.call( this, index == undefined ? this._index : index );
this._autoNext();
}
};
return function(){
var options = arguments[1];
if ( options && options.auto ) {
//扩展options
$$.extend( options, {
autoDelay: 2000//展示时间
}, false );
//扩展属性
$$.extend( this, prototype );
//扩展钩子
$$A.forEach( methods, function( method, name ){
$$CE.addEvent( this, name, method );
}, this );
}
init.apply( this, arguments );
}
})();
提示信息扩展
SlideView.prototype._initialize = (function(){
var init = SlideView.prototype._initialize,
methods = {
"init": function(){
//坐标样式
this._tipPos = /^(bottom|top|right|left)$/.test( this.options.tipPos.toLowerCase() ) ? RegExp.$1 : "bottom";
},
"initNodes": function(){
var opt = this.options, tipTag = opt.tipTag, tipClass = opt.tipClass,
re = tipClass && new RegExp("(^|\s)" tipClass "(\s|$)"),
getTipNode = function(node){
var nodes = node.getElementsByTagName( tipTag );
if ( tipClass ) {
nodes = $$A.filter( nodes, function(n){ return re.test(n.className); } );
}
return nodes[0];
};
//设置提示对象
var tipShow = opt.tipShow, tipClose = opt.tipClose,
offset = /right|left/.test( this._tipPos ) ? "offsetWidth" : "offsetHeight";
this._each( function(o) {
var node = o.node, tipNode = getTipNode(node);
node.style.overflow = "hidden";
tipNode.style.position = "absolute"; tipNode.style.left = 0;
//创建提示对象
o.tip = {
"node": tipNode,
"show": tipShow != undefined ? tipShow : 0,
"close": tipClose != undefined ? tipClose : -tipNode[offset]
};
});
},
"setMove": function(index){
var maxIndex = this._nodes.length - 1;
this._each( function(o, i) {
var tip = o.tip;
if ( this._reverse ) { i = maxIndex -i; }
tip.target = index == undefined || index != i ? tip.close : tip.show;
tip.begin = tip.current; tip.change = tip.target - tip.begin;
});
},
"tweenMove": function(){
this._setTipPos( function(tip) {
return Math.round( this.tween( this._time, tip.begin, tip.change, this.duration ) );
});
},
"targetMove": function(){
this._setTipPos( function(tip){ return tip.target; });
},
"defaultMove": function(){
this._setTipPos( function(tip){ return tip.close; });
},
"dispose": function(){
this._each( function(o){ o.tip = null; });
}
},
prototype = {
//设置坐标值函数
_setTipPos: function(method) {
var pos = this._tipPos;
this._each( function(o, i) {
var tip = o.tip;
tip.node.style[ pos ] = (tip.current = method.call( this, tip )) "px";
});
}
};
return function(){
var options = arguments[1];
if ( options && options.tip == true ) {
//扩展options
$$.extend( options, {
tipPos: "bottom",//提示位置
tipTag: "*",//提示元素标签
tipClass: "",//提示元素样式
tipShow: null,//展示时目标坐标
tipClose: null//关闭时目标坐标
}, false );
//扩展属性
$$.extend( this, prototype );
//扩展钩子
$$A.forEach( methods, function( method, name ){
$$CE.addEvent( this, name, method );
}, this );
}
init.apply( this, arguments );
}
})();
完整实例下载
原文:http://www.cnblogs.com/cloudgamer/archive/2010/07/29/SlideView.html

Hot Article

Hot tools Tags

Hot Article

Hot Article Tags

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
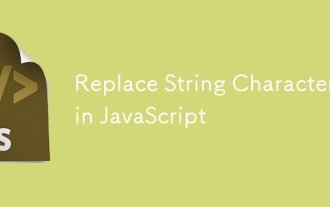
Replace String Characters in JavaScript
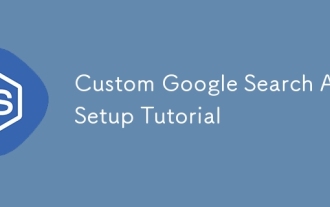
Custom Google Search API Setup Tutorial
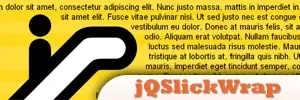
8 Stunning jQuery Page Layout Plugins
