this and execution context implementation code_javascript skills
The execution context of a function is determined by the current running environment:
1. Global variables and global functions are attached to the global object (window), so using "var" or "this" to define global variables is equivalent.
2. Execution context and scope are different. The execution context is determined at runtime and may change at any time, while the scope is determined at definition time and never changes.
3. If the method of an object is currently executed, the execution context is the object to which this method is attached.
4. If the current process is to create an object or execute a method of an object, the execution context is the object being created.
5. If a method does not explicitly specify an attached object when executing, the context of this method is the global object.
6. Use call and apply to change the execution context of the object. " global variable"; //The global variable is attached to the object
//this.v1 = "global variable with this"; //When global variables are defined, the two methods var v1 and this.v1 are equivalent.
function func1(){
Look at a slightly more complicated example:
Copy the code
The code is as follows:
function ftest(){
var v = "v1v1v1";
this.this_v = "this_v";
//this_v
When ftest is executed as a function, the context is the global object. So the variables defined using this in ftest become global variables. So we directly use the variable name to access the value of this_v outside ftest. However, since the anonymous function returned in ftest is defined inside ftest, the scope of this anonymous function is inside ftest. Therefore, when there is a global variable v and a local variable v with the same name, this anonymous function accesses the variable v defined internally in ftest.
Next, treat ftest as a class and instantiate it using the new keyword:
Copy the code
The code is as follows:
function ftest(){
var v = "v1v1v1";
this.this_v = "this_v";
//undefined
When instantiating ftest as an object, the context is created during the object creation process the object itself. Note that the object created at this time is an instance of ftest, and a function is returned after the creation is completed. This causes new ftest() to return a function after instantiation, rather than a reference to the object after instantiation of ftest(). Therefore, this instantiated object cannot be referenced. When we define the returned function, because the context of this function is not specified with this, the context of the returned function is a global object and the scope is inside the ftest() function. Therefore, when function a() is executed, the this_v variable is not defined in the context, resulting in an access error.
Note, the above code:
Copy the code
The code is as follows:
function ftest( ){
return function(){
}
Copy code
The code is as follows:
var v = "global variable";
function method(){
writeHtml(v);
writeHtml(this.v);
}
var Class1 = function(){
var v = "private variable";
this.v = "object variable";
var method2 = method;
this.method2 = method;
var method3 = function(){
writeHtml(v);
writeHtml(this.v);
}
this.method3 = function(){
writeHtml(v) ;
writeHtml(this.v);
}
method2(); //global variable
//global variable
this.method2(); //global variable
//object variable
method3(); //private variable
//global variable
this.method3();//private variable
//object variable
}
var obj = new Class1();
Since method is defined globally, the scope of method is determined to be global when it is defined. So when method2 is called inside Class1, its scope is global and the context is the global object. Therefore, variables accessed in functions are global variables.
Similarly, when this.method2 is called, its scope is global, but because the function uses the this keyword when it is defined to indicate the object whose context is Class1, the function accesses variables without context qualification. When accessing a global variable, accessing a context-limited variable accesses the corresponding variable in the current context.
When calling method3 and this.method3, local variables are accessed when accessing variables without context, because local variables hide global variables. When there is context limitation, it is the same as method 2, and the variables in the current context are accessed.
Using call and apply can change the execution context. Since call and apply only have different parameter types, call is used in the following examples to demonstrate.
var v = "global variable";
var method = function(){
writeHtml(this.v);
}
var Class2 = function(){
this.v = "object variable in instance of Class2";
this .method = function(){
writeHtml(this.v);
}
}
var Class3 = function(){
this.v = "object variable in instance of Class3" ;
this.method = function(){
writeHtml(this.v);
}
}
var obj2 = new Class2();
var obj3 = new Class3();
method(); //global variable
obj2.method(); //object variable in instance of Class2
obj3.method(); //object variable in instance of Class3
method.call(obj2); //object variable in instance of Class2
method.call(obj3); //object variable in instance of Class3
obj2.method.call (obj3); //object variable in instance of Class3
obj2.method.call(this); //global variable
obj3.method.call(obj2); //object variable in instance of Class2
obj3.method.call(this); //global variable
As you can see, you can use call or apply to bind the method to the specified context. In the global environment, the context pointed to by this is the global object.

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
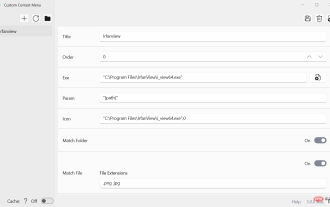
Microsoft has changed the context menu in File Explorer when launching the Windows 11 operating system. File Explorer has two context menus in Windows 11: When the user right-clicks a file or folder in File Manager, the new compact menu opens first. The classic context menu can be opened from this new menu or using a shortcut. There is also an option to restore the classic context menu in Windows 11’s File Explorer so that it opens by default. Programs can add their entries to the new context menu, but they need to have the correct programming to do so. Windows 11 users can use Windows app custom context menus to add their
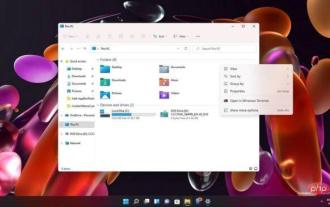
Windows 11 continues to be updated regularly, and reports suggest that SunValley 2 will address most of the major issues with the new operating system. Windows 11 brings several design overhauls, including a new Start menu, Notification Center, taskbar, and modern context menus. Context menus are a core part of the operating system's interface (especially File Explorer) and have been updated to a new design, which seems to be causing slow performance. There is an issue with Windows 11's context menu when you right-click on a file or folder in File Explorer. As part of its efforts to modernize its operating system, Microsoft has reduced the number of options in context menus and started using icons/buttons for options like copy or cute. Although this
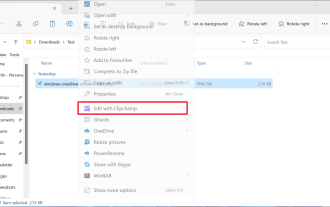
Clipchamp is the new default application for Microsoft's Windows 11 operating system. Microsoft purchased the web-based video editor in 2021 and integrated it into development builds of Windows 11 in early 2022. The free version of Clipchamp wasn't really usable at the time because it had too many limitations. It watermarks all videos and limits exports to 480p. Microsoft has lifted some restrictions and changed available plans. Export now supports 1080p, and the free version no longer adds a watermark to the export. Microsoft makes Clipchamp a default app in Windows 11 2022 update. Windows1
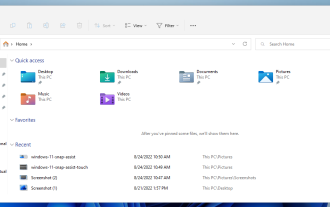
In the fourth part of this series, we’ll take a look at the default file manager tool, File Explorer, and the changes Microsoft plans to introduce in the Windows 11 2022 update. When Microsoft launches the new Windows 11 operating system in 2021, it makes changes to File Explorer in several key areas. One of the most prominent changes is the introduction of a compact context menu, which File Explorer displays by default. Tip: Here are the instructions for restoring the classic File Explorer context menu in Windows 11. This change isn't the only one, as Microsoft simplified File Explorer's main toolbar, which resulted in many items only being accessible after an extra click or two. Hope Microsoft is around the corner

While Microsoft is working day and night to make Windows 11 more modern and polished, the operating system retains remnants of an older era beneath its skin. For example, there are two context menus: one modern and one traditional. If you're unhappy with the look of your old menus in Windows 11 (and Windows 10), here's a small open source app (via OnMsft) that thanks them for using Acrylic effects to make them prettier. TranslucentFlyouts is a small application that replaces boring-looking solid backgrounds with translucent effects. You can personalize styles (Acrylic, Aero, Transpare

A colleague got stuck due to a bug pointed by this. Vue2’s this pointing problem caused an arrow function to be used, resulting in the inability to get the corresponding props. He didn't know it when I introduced it to him, and then I deliberately looked at the front-end communication group. So far, at least 70% of front-end programmers still don't understand it. Today I will share with you this link. If everything is wrong If you haven’t learned it yet, please give me a big mouth.

1. this keyword 1. Type of this: Which object is called is the reference type of that object 2. Usage summary 1. this.data;//Access attribute 2. this.func();//Access method 3.this( );//Call other constructors in this class 3. Explanation of usage 1.this.data is used in member methods. Let us see what will happen if this is not added classMyDate{publicintyear;publicintmonth;publicintday; publicvoidsetDate(intyear,intmonth,intday){ye
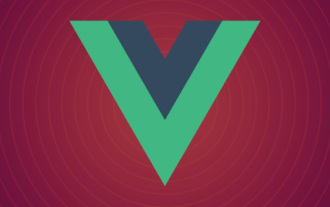
This article will help you interpret the vue source code and introduce why you can use this to access properties in various options in Vue2. I hope it will be helpful to everyone!
