Summary of using && and || in Javascript_javascript tips
&& and || in ordinary cases are relatively simple and will not be discussed here.
Prepare two objects for the following discussion.
var alice = {
name: "alice" ,
toString: function () {
return this.name;
}
}
var smith = {
name: "smith",
toString: function () {
return this.name;
}
}
In javascript, && can not only be used for boolean type, nor can it only return Boolean type results.
l If the first operand is of type Boolean and the value is false, then return false directly.
l If the first operand is of type Boolean and the value is true, and the other operand is of type object, then this object will be returned.
l If both operands are of object type, return the second object.
l If any operand is null, then null is returned.
l If either operand is NaN, return NaN.
l If any operand is undefined, return undefined.
alert(false && alice); // false
alert(true && alice); // alice
alert(alice && smith); // smith
alert(smith && alice); // alice
alert(null && alice); // null
alert(NaN && alice); // NaN
alert(undefined && alice); // undefined
alert(alice && undefined); // undefined
For ||, it is not only used for Boolean type, nor only returns Boolean type results.
In fact, null, undefined, and NaN will be treated as false. And the object is treated as true.
l If the first operand is of type boolean and the value is true, then return true directly.
l If the first operand is of type Boolean and the value is false and the second operand is object, then the object object is returned.
l If both operands are of type object, return the first object.
l If both operands are null, then null is returned.
l If both operands are NaN, return NaN.
l If both operands are undefined, then undefined is returned.
alert(false || alice); // alice
alert(true || alice); // true
alert(alice || smith); // alice
alert(smith || alice); // smith
alert(null || alice); // alice
alert(alice || null); // alice
alert(null || null); // null
alert(NaN || alice); // alice
alert(alice || NaN); // alice
alert(NaN || NaN); // NaN
alert(undefined || alice); // alice
alert(alice || undefined); // alice
alert(undefined || undefined); // undefined
You don’t need to make it so complicated. I recommend you read this part of the explanation.
a && b: Convert a and b to Boolean types, and then perform logical AND. True returns b, false returns a
a || b: Convert a, b to Boolean type, and then perform logical OR, true returns a, false returns b
Conversion rules:
Object is true
Non-zero number is true
Non-empty strings are true
Others are false
Related articles can refer to the following articles to summarize
js AND or operator || && Magical Uses
JS uses AND or operator priority to implement if else conditional judgment expression
Alternative usage skills of javascript && and || algorithms

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


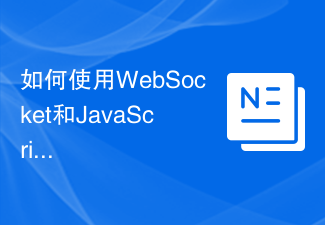
How to use WebSocket and JavaScript to implement an online speech recognition system Introduction: With the continuous development of technology, speech recognition technology has become an important part of the field of artificial intelligence. The online speech recognition system based on WebSocket and JavaScript has the characteristics of low latency, real-time and cross-platform, and has become a widely used solution. This article will introduce how to use WebSocket and JavaScript to implement an online speech recognition system.
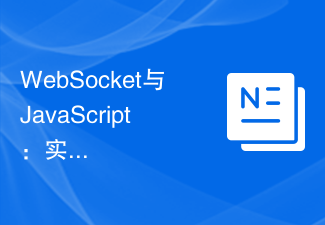
WebSocket and JavaScript: Key technologies for realizing real-time monitoring systems Introduction: With the rapid development of Internet technology, real-time monitoring systems have been widely used in various fields. One of the key technologies to achieve real-time monitoring is the combination of WebSocket and JavaScript. This article will introduce the application of WebSocket and JavaScript in real-time monitoring systems, give code examples, and explain their implementation principles in detail. 1. WebSocket technology
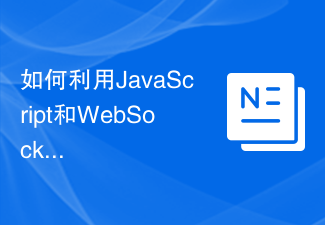
Introduction to how to use JavaScript and WebSocket to implement a real-time online ordering system: With the popularity of the Internet and the advancement of technology, more and more restaurants have begun to provide online ordering services. In order to implement a real-time online ordering system, we can use JavaScript and WebSocket technology. WebSocket is a full-duplex communication protocol based on the TCP protocol, which can realize real-time two-way communication between the client and the server. In the real-time online ordering system, when the user selects dishes and places an order
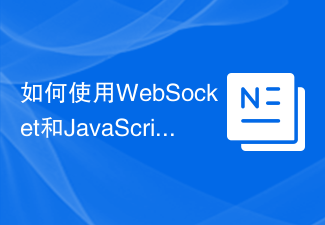
How to use WebSocket and JavaScript to implement an online reservation system. In today's digital era, more and more businesses and services need to provide online reservation functions. It is crucial to implement an efficient and real-time online reservation system. This article will introduce how to use WebSocket and JavaScript to implement an online reservation system, and provide specific code examples. 1. What is WebSocket? WebSocket is a full-duplex method on a single TCP connection.
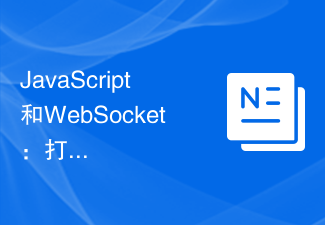
JavaScript and WebSocket: Building an efficient real-time weather forecast system Introduction: Today, the accuracy of weather forecasts is of great significance to daily life and decision-making. As technology develops, we can provide more accurate and reliable weather forecasts by obtaining weather data in real time. In this article, we will learn how to use JavaScript and WebSocket technology to build an efficient real-time weather forecast system. This article will demonstrate the implementation process through specific code examples. We
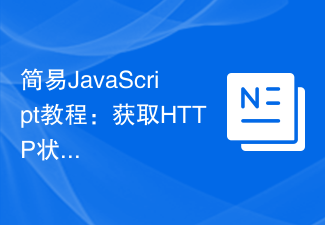
JavaScript tutorial: How to get HTTP status code, specific code examples are required. Preface: In web development, data interaction with the server is often involved. When communicating with the server, we often need to obtain the returned HTTP status code to determine whether the operation is successful, and perform corresponding processing based on different status codes. This article will teach you how to use JavaScript to obtain HTTP status codes and provide some practical code examples. Using XMLHttpRequest

Usage: In JavaScript, the insertBefore() method is used to insert a new node in the DOM tree. This method requires two parameters: the new node to be inserted and the reference node (that is, the node where the new node will be inserted).
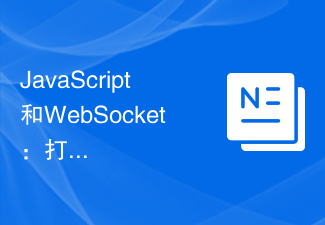
JavaScript is a programming language widely used in web development, while WebSocket is a network protocol used for real-time communication. Combining the powerful functions of the two, we can create an efficient real-time image processing system. This article will introduce how to implement this system using JavaScript and WebSocket, and provide specific code examples. First, we need to clarify the requirements and goals of the real-time image processing system. Suppose we have a camera device that can collect real-time image data
