The picture below is my design idea
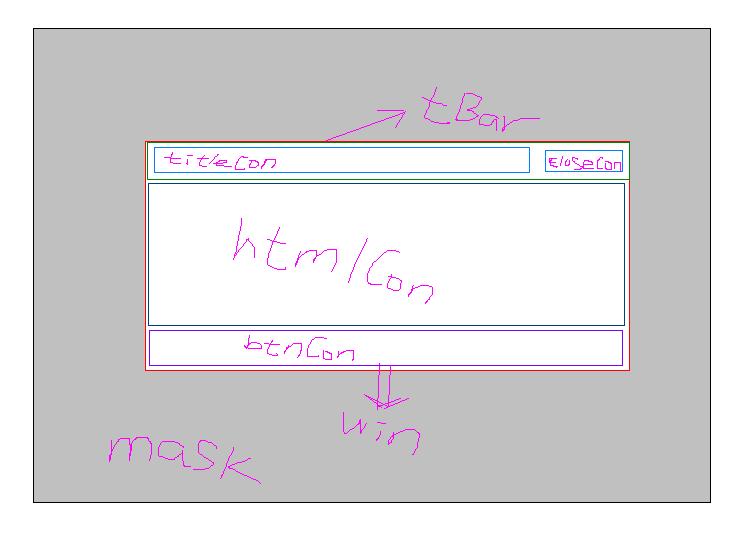
The following is the specific js code
1. First define several custom functions
Code
//Determine whether it is an array
function isArray(v)
{
return v && typeof v.length == 'number' && typeof v.splice == 'function';
}
//Create element
function createEle(tagName)
{
return document.createElement (tagName);
}
//Add child elements in body
function appChild(eleName)
{
return document.body.appendChild(eleName);
}
//Remove child elements from body
function remChild(eleName)
{
return document.body.removeChild(eleName);
}
2, Specific form implementation code
Code
//Pop-up window, title (html form), html, width, height, whether it is a modal dialog box (true, false), button (the close button is the default, the format is ['button 1', fun1 , 'Button 2', fun2] array form, the front is the button value, and the back is the button onclick event)
function showWindow(title,html,width,height,modal,buttons)
{
//Avoid Window is too small
if (width < 300)
{
width = 300;
}
if (height < 200)
{
height = 200;
}
//Declare the width and height of the mask (that is, the width and height of the entire screen)
var w,h;
//Visible area width and height
var cw = document .body.clientWidth;
var ch = document.body.clientHeight;
//The width and height of the body
var sw = document.body.scrollWidth;
var sh = document.body.scrollHeight ;
//The distance between the top of the visible area and the top and left side of the body
var st = document.body.scrollTop;
var sl = document.body.scrollLeft;
w = cw > sw ? cw :sw;
h = ch > sh ? ch:sh;
//Avoid the window being too large
if (width > w)
{
width = w;
}
if (height > h)
{
height = h;
}
//If modal is true, that is, a modal dialog box, a transparent mask must be created membrane
if (modal)
{
var mask = createEle('div');
mask.style.cssText = "position:absolute;left:0;top:0px;background:# fff;filter:Alpha(Opacity=30);opacity:0.5;z-index:10000;width:" w "px;height:" h "px;";
appChild(mask);
}
//This is the main form
var win = createEle('div');
win.style.cssText = "position:absolute;left:" (sl cw/2 - width/2) " px;top:" (st ch/2 - height/2) "px;background:#f0f0f0;z-index:10001;width:" width "px;height:" height "px;border:solid 2px #afccfe; ";
//Title bar
var tBar = createEle('div');
//afccfe,dce8ff,2b2f79
tBar.style.cssText = "margin:0;width:" width "px;height:25px;background:url(top-bottom.png);cursor:move;";
//The text part in the title bar
var titleCon = createEle('div');
titleCon.style.cssText = "float:left;margin:3px;";
titleCon.innerHTML = title;//firefox does not support innerText, so innerHTML is used here
tBar.appendChild(titleCon);
//"Close button" in the title bar
var closeCon = createEle('div');
closeCon.style.cssText = "float:right;width:20px;margin:3px;cursor:pointer; ";//cursor:hand is not available in firefox
closeCon.innerHTML = "×";
tBar.appendChild(closeCon);
win.appendChild(tBar);
//Form In the content part, overflow in CSS causes a scroll bar to appear when the content size exceeds this range.
var htmlCon = createEle('div');
htmlCon.style.cssText = "text-align:center; width:" width "px;height:" (height - 50) "px;overflow:auto;";
htmlCon.innerHTML = html;
win.appendChild(htmlCon);
//Form Bottom button part
var btnCon = createEle('div');
btnCon.style.cssText = "width:" width "px;height:25px;text-height:20px;background:url(top- bottom.png);text-align:center;padding-top:3px;";
//If the parameter buttons is an array, a custom button will be created
if (isArray(buttons))
{
var length = buttons.length;
if (length > 0)
{
if (length % 2 == 0)
{
for (var i = 0 ; i < length; i = i 2)
{
//Button array
var btn = createEle('button');
btn.innerHTML = buttons[i];//firefox The value attribute is not supported, so innerHTML is used here
// btn.value = buttons[i];
btn.onclick = buttons[i 1];
btnCon.appendChild(btn);
/ /User fills the space between buttons
var nbsp = createEle('label');
nbsp.innerHTML = "  ";
btnCon.appendChild(nbsp);
}
}
}
}
//This is the default close button
var btn = createEle('button');
// btn.setAttribute("value","close");
btn.innerHTML = 'Close';
// btn.value = 'Close';
btnCon.appendChild(btn);
win.appendChild(btnCon);
appChild(win );
/*************************************The following is the drag form event******** *************/
//X coordinate of the mouse stay
var mouseX = 0;
//Y coordinate of the mouse stay
var mouseY = 0;
//The distance from the form to the top of the body
var toTop = 0;
//The distance from the form to the left side of the body
var toLeft = 0;
//Determine whether the form can be moved
var moveable = false;
//Mouse down event of the title bar
tBar.onmousedown = function()
{
var eve = getEvent();
moveable = true ;
mouseX = eve.clientX;
mouseY = eve.clientY;
toTop = parseInt(win.style.top);
toLeft = parseInt(win.style.left);
//Move mouse event
tBar.onmousemove = function()
{
if(moveable)
{
var eve = getEvent();
var x = toLeft eve.clientX - mouseX;
var y = toTop eve.clientY - mouseY;
if (x > 0 && (x width < w) && y > 0 && (y height < h))
{
win.style.left = x "px";
win.style.top = y "px";
}
}
}
//Put down the mouse event, note that this is document instead of tBar
document.onmouseup = function()
{
moveable = false;
}
}
// Method to get browser events, compatible with IE and Firefox
function getEvent()
{
return window.event || arguments.callee.caller.arguments[0];
}
/ /Close event of the "Close Button" in the top title bar and bottom button bar
btn.onclick = closeCon.onclick = function()
{
remChild(win);
remChild( mask);
}
}
Instance call
str = "Look at the effect of my form";
showWindow('My prompt box',str,850,250,true,['region',fun1,'mineral type ',fun2]);
More complete and practical code, including definition and calling
code
此脚本在ie,firefox浏览器下运行通过。。。。