JavaScript's public, private and privileged modes_javascript tips
Private variables are declared inside the object using the 'var' keyword, and it can only be accessed by private functions and privileged methods.
Private functions are declared in the object's constructor (or defined through var functionName=function(){...}). They can be called by privileged functions (including the object's constructor) and private functions.
Privileged methods are declared via this.methodName=function(){...} and may be called by code outside the object. It can use: this.privileged function() method to call privileged functions, and use: private function() method to call private functions.
Public properties are defined through this.variableName and are readable and writable outside the object. Cannot be called by private functions.
Public methods are defined by ClassName.prototype.methodName=function(){...} and can be called from outside the object.
Prototype properties are defined by ClassName.prototype.propertyName=someValue.
Static properties are defined by ClassName.propertyName=someValue.
Also pay attention to this writing method: var function name = function function name () {...} This function has the characteristics of a privileged function and a private function when it is called.
Example:
< ;div onclick="start()" style="color:blue">click me
JavaScript It is the most misunderstood programming language in the world. Some argue that it lacks information hiding features because JavaScript objects cannot have methods on private variables.
But this is a misunderstanding. JavaScript objects can have private members.
Objects
JavaScript is fundamentally about objects. Arrays are objects, methods are objects, and Objects are also objects. What is an object? An object is a collection of key-value pairs. Keys are strings and
values can be strings, numbers, booleans and objects (including arrays and methods). Objects are usually implemented as Hashtables so that values can be retrieved quickly.
If the value is a function, I can call it a method. When an object's method is called, the "this" variable is assigned to the object. Methods can access instance
variables through the "this" variable.
Objects can be generated by the method of initializing objects - the constructor. Constructors provide features that classes provide in other programming languages, including static variables and methods.
Public
The members of the object are all public members. Any object can access, modify, delete these members or add new members. There are two main ways to place members in a new object:
In the constructor
This technique is usually used to initialize public instance variables. The "this" variable of the constructor is used to add members to the object.
Java code
functin Container(param) {
this.member = param;
}
functin Container(param) {
this.member = param;
}
In this way, if we construct a new Object var myContainer = new Container('abc'), then myContainer.member is 'abc'.
In prototype
This technique is usually used to add public methods. When looking for a member and it is not in the object itself, it is found in the prototype member of the object's constructor.
The prototype mechanism is used for inheritance. To add a method to all objects created by the constructor, just add to the constructor's prototype:
Java code
Container.prototype.stamp = function (string) {
return this.member string;
}
Container.prototype.stamp = function (string) {
return this. member string;
}
In this way, we can call the method myContainer.stamp('def'), and the result is 'abcdef'.
Private
Private members are generated by the constructor. Ordinary var variables and constructor parameters are called private members.
Java code
function Container(param) {
this.member = param;
var secret = 3;
var that = this;
}
function Container(param) {
this.member = param;
var secret = 3;
var that = this;
}
The constructor creates 3 private instance variables: param, secret and that. They are added to the object, but cannot be accessed externally, nor by the object's own
public methods. They can only be accessed by private methods. Private methods are internal methods of the constructor.
Java code
function Container(param) {
function dec() {
if (secret > 0) {
secret -= 1;
return true;
} else {
return false;
}
}
this.member = param;
var secret = 3;
var that = this;
}
function Container(param) {
function dec() {
if (secret > 0) {
secret -= 1;
return true;
} else {
return false;
}
}
this.member = param;
var secret = 3;
var that = this;
}
Private method dec checks the secret instance variable. If it is greater than 0, decrement the secret and return true, otherwise return false. It can be used to limit the use of this object to 3 times.
As usual, we define a private that variable. This is used to make this object available to private methods. This is done because there is a bug in the ECMAScript language specification.
This bug prevents the correct setting of this to internal methods.
Private methods cannot be called by public methods. In order to make private methods useful, we need to introduce privileged methods.
Privileged
The privileged method can access private variables and methods, and it can itself be accessed by public methods and the outside world. A privileged method can be removed or replaced, but it cannot
be changed or forced to reveal its secrets.
Privileged methods are allocated using this in the constructor.
Java code
function Container(param) {
function dec() {
if (secret > 0) {
secret -= 1;
return true;
} else {
return false;
}
}
this.member = param;
var secret = 3;
var that = this;
this.service = function() {
if (dec()) {
return that.member;
} else {
return null;
}
};
}
function Container(param) {
function dec() {
if (secret > 0) {
secret -= 1;
return true;
} else {
return false;
}
}
this.member = param;
var secret = 3;
var that = this;
this.service = function() {
if (dec()) {
return that.member;
} else {
return null;
}
};
}
service is a privileged method. The first three calls to myContainer.service() will return 'abc'. After that it will return null. The service calls the private dec method, and the
dec method accesses the private secret variable. Service is visible to other objects and methods, but it does not allow direct access to private variables.
Closures
Since JavaScript has closures, the pattern of public, private and privileged members is possible. This means that an inner method can always access its outer method's
var variables and parameters, even after the outer method returns. This is a very powerful feature of the JavaScript language. There are currently no JavaScript
programming books that show how to exploit this feature, and most don't even mention it.
Private and privileged members can only be generated when the object is constructed. Public members can be added at any time.
Mode
public
Java code
function Constructor(...) {
this.membername = value;
}
Constructor.prototype.membername = value;
function Constructor(...) {
this.membername = value;
}
Constructor.prototype.membername = value;
Private
Java code
function Constructor( ...) {
var that = this;
var membername = value;
function membername(...) {...}
}
// Note: function statement
// function membername(...) {...}
// is the abbreviation of the following code
// var membername = function membername(...) {...};
function Constructor(...) {
var that = this;
var membername = value;
function membername(...) {...}
}
// Note: function statement
// function membername(...) {...}
// is the abbreviation of the following code
// var membername = function membername(...) {...};
Privileged
Java code
function Constructor(...) {
this.membername = function (...) {...};
}
function Constructor(...) {
this. membername = function (...) {...};
}
Translator’s Note: I think the privileged method can simply be regarded as a public method in the constructor , because the privileged method can be accessed by the outside world and public methods,
and it itself can access private variables.

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
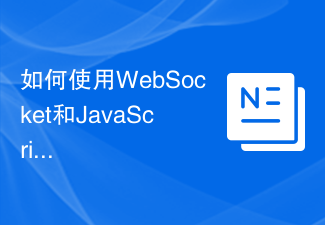
How to use WebSocket and JavaScript to implement an online speech recognition system Introduction: With the continuous development of technology, speech recognition technology has become an important part of the field of artificial intelligence. The online speech recognition system based on WebSocket and JavaScript has the characteristics of low latency, real-time and cross-platform, and has become a widely used solution. This article will introduce how to use WebSocket and JavaScript to implement an online speech recognition system.
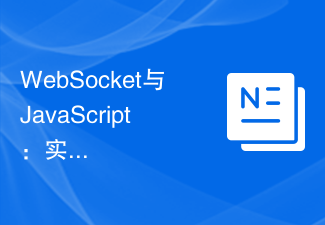
WebSocket and JavaScript: Key technologies for realizing real-time monitoring systems Introduction: With the rapid development of Internet technology, real-time monitoring systems have been widely used in various fields. One of the key technologies to achieve real-time monitoring is the combination of WebSocket and JavaScript. This article will introduce the application of WebSocket and JavaScript in real-time monitoring systems, give code examples, and explain their implementation principles in detail. 1. WebSocket technology
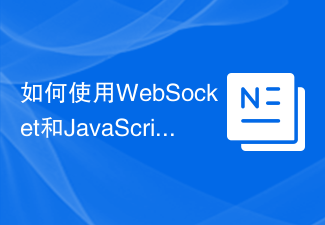
How to use WebSocket and JavaScript to implement an online reservation system. In today's digital era, more and more businesses and services need to provide online reservation functions. It is crucial to implement an efficient and real-time online reservation system. This article will introduce how to use WebSocket and JavaScript to implement an online reservation system, and provide specific code examples. 1. What is WebSocket? WebSocket is a full-duplex method on a single TCP connection.
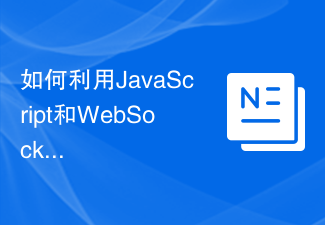
Introduction to how to use JavaScript and WebSocket to implement a real-time online ordering system: With the popularity of the Internet and the advancement of technology, more and more restaurants have begun to provide online ordering services. In order to implement a real-time online ordering system, we can use JavaScript and WebSocket technology. WebSocket is a full-duplex communication protocol based on the TCP protocol, which can realize real-time two-way communication between the client and the server. In the real-time online ordering system, when the user selects dishes and places an order
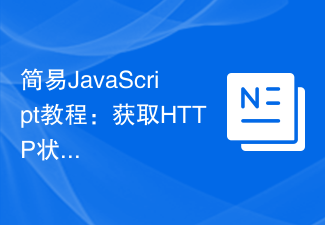
JavaScript tutorial: How to get HTTP status code, specific code examples are required. Preface: In web development, data interaction with the server is often involved. When communicating with the server, we often need to obtain the returned HTTP status code to determine whether the operation is successful, and perform corresponding processing based on different status codes. This article will teach you how to use JavaScript to obtain HTTP status codes and provide some practical code examples. Using XMLHttpRequest
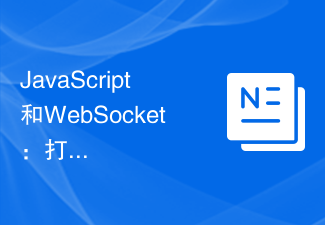
JavaScript and WebSocket: Building an efficient real-time weather forecast system Introduction: Today, the accuracy of weather forecasts is of great significance to daily life and decision-making. As technology develops, we can provide more accurate and reliable weather forecasts by obtaining weather data in real time. In this article, we will learn how to use JavaScript and WebSocket technology to build an efficient real-time weather forecast system. This article will demonstrate the implementation process through specific code examples. We
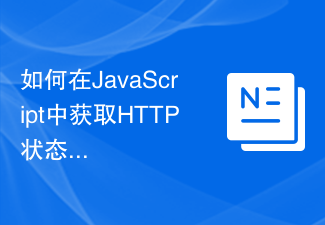
Introduction to the method of obtaining HTTP status code in JavaScript: In front-end development, we often need to deal with the interaction with the back-end interface, and HTTP status code is a very important part of it. Understanding and obtaining HTTP status codes helps us better handle the data returned by the interface. This article will introduce how to use JavaScript to obtain HTTP status codes and provide specific code examples. 1. What is HTTP status code? HTTP status code means that when the browser initiates a request to the server, the service

Usage: In JavaScript, the insertBefore() method is used to insert a new node in the DOM tree. This method requires two parameters: the new node to be inserted and the reference node (that is, the node where the new node will be inserted).
