理解 Laravel Eloquent ORM
什么是 Eloquent
Eloquent 是 Laravel 的 'ORM',即 'Object Relational Mapping',对象关系映射。ORM 的出现是为了帮我们把对数据库的操作变得更加地方便。Eloquent 让一个 'Model类' 对应一张数据库表,并且在底层封装了很多 'function',可以让 Model 类非常方便地调用。所有的 Eloquent 模型都继承于 Illuminate\Database\Eloquent\Model。
通过 make:model 命令自动生成 Eloquent 模型:
php artisan make:model Article -m
-m 参数:自动为这个模型生成对应的 articles表
php artisan make:migration create_articles_table --create=articles
为模型创建一个对应的表(=后面指定表名)
注意:我们并没有告诉 Eloquent 模型会使用哪个数据库表。若没有特别指定,系统会默认自动对应名称为「类名称的小写复数形态」的数据库表。所以,在上面的例子中, Eloquent 会假设 Article 模型将把数据存在 articles 数据库表。您也可以在类中定义 table 属性自定义要对应的数据库表。
class User extends Model { protected $table = 'your_table';}
批量赋值
在建立一个新的模型时,由于盲目地将用户输入写入模型数据,可能会造成 严重的安全隐患。基于这个理由,所有的 Eloquent 模型默认会阻止批量赋值 。
fillable属性指定了哪些字段支持批量赋值 。可以设定在类的属性里或是实例化后设定。
class Article extends Model { protected $fillable = ['title', 'content', 'email'];}
在上面的例子里,只有三个属性(表中的字段)允许批量赋值。
guarded与 fillable相反,是作为「黑名单」而不是「白名单」:
class Article extends Model { protected $guarded = ['id', 'password'];}
上面的例子中, id 和 password 属性不会被批量赋值,而所有其他的属性则允许批量赋值。您也可以使用 guard 属性阻止所有属性被批量赋值
hidden属性指定那些字段被隐藏了,即输出了也不会被看到
class Article extends Model { protected $hidden = ['id', 'password'];}
基本用法
多看看文档
找到id为2的并且打印其标题
$article = Article::find(2);echo $article->title;
有时, 您可能想要在找不到模型数据时抛出异常,通过 firstOrFail 方法。
$article = Article::findOrFail(100);echo $article->title;
查找标题为“我是标题”的文章,并打印 id
$article = Article::where('title', '我是标题')->first();echo $article->id;
查询出所有文章并循环打印出所有标题
$articles = Article::all(); // 此处得到的 $articles 是一个对象集合,可以在后面加上 '->toArray()' 变成多维数组。foreach ($articles as $article) { echo $article->title;}
查找 id 在 10~20 之间的所有文章并打印所有标题
$articles = Article::where('id', '>', 10)->where('id', '<', 20)->get();foreach ($articles as $article) { echo $article->title;}
查询出所有文章并循环打印出所有标题,按照 updated_at 倒序排序
$articles = Article::where('id', '>', 10)->where('id', '<', 20)->orderBy('updated_at', 'desc')->get();foreach ($articles as $article) { echo $article->title;}
使用要点
-
每一个继承了 Eloquent 的类都有两个 '固定用法' Article::find($number) Article::all(),前者会得到一个带有数据库中取出来值的对象,后者会得到一个包含整个数据库的对象合集。
-
所有的中间方法如 where() orderBy()等都能够同时支持 '静态' 和 '非静态链式' 两种方式调用,即 Article::where()...和 Article::....->where()。
-
所有的 '非固定用法' 的调用最后都需要一个操作来 '收尾',本片教程中有两个 '收尾操作': ->get()和 ->first()。

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


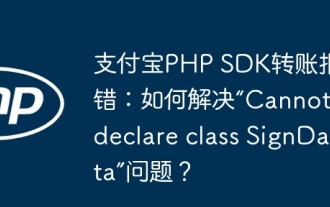
Alipay PHP...
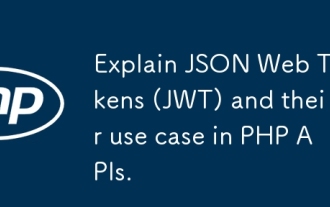
JWT is an open standard based on JSON, used to securely transmit information between parties, mainly for identity authentication and information exchange. 1. JWT consists of three parts: Header, Payload and Signature. 2. The working principle of JWT includes three steps: generating JWT, verifying JWT and parsing Payload. 3. When using JWT for authentication in PHP, JWT can be generated and verified, and user role and permission information can be included in advanced usage. 4. Common errors include signature verification failure, token expiration, and payload oversized. Debugging skills include using debugging tools and logging. 5. Performance optimization and best practices include using appropriate signature algorithms, setting validity periods reasonably,
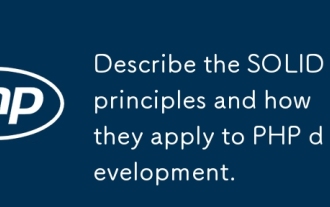
The application of SOLID principle in PHP development includes: 1. Single responsibility principle (SRP): Each class is responsible for only one function. 2. Open and close principle (OCP): Changes are achieved through extension rather than modification. 3. Lisch's Substitution Principle (LSP): Subclasses can replace base classes without affecting program accuracy. 4. Interface isolation principle (ISP): Use fine-grained interfaces to avoid dependencies and unused methods. 5. Dependency inversion principle (DIP): High and low-level modules rely on abstraction and are implemented through dependency injection.
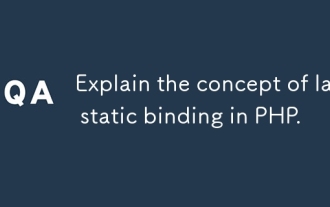
Article discusses late static binding (LSB) in PHP, introduced in PHP 5.3, allowing runtime resolution of static method calls for more flexible inheritance.Main issue: LSB vs. traditional polymorphism; LSB's practical applications and potential perfo
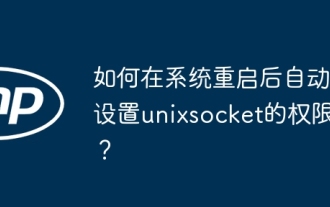
How to automatically set the permissions of unixsocket after the system restarts. Every time the system restarts, we need to execute the following command to modify the permissions of unixsocket: sudo...
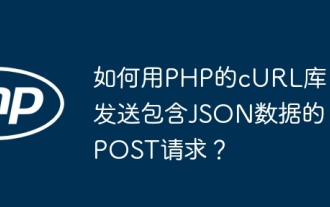
Sending JSON data using PHP's cURL library In PHP development, it is often necessary to interact with external APIs. One of the common ways is to use cURL library to send POST�...
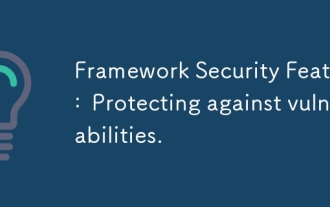
Article discusses essential security features in frameworks to protect against vulnerabilities, including input validation, authentication, and regular updates.
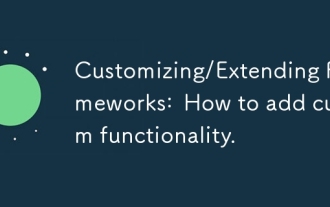
The article discusses adding custom functionality to frameworks, focusing on understanding architecture, identifying extension points, and best practices for integration and debugging.
