javascript code to detect browser type and version_javascript skills
getBrowser : function(){
var browser = {
msie: false, firefox: false, opera: false, safari: false,
chrome: false, netscape: false, appname: 'unknown' , version: 0
},
userAgent = window.navigator.userAgent.toLowerCase();
if ( /(msie|firefox|opera|chrome|netscape)D (d[d.]*) /.test( userAgent ) ){
browser[RegExp.$1] = true;
browser.appname = RegExp.$1;
browser.version = RegExp.$2;
} else if ( / versionD (d[d.]*).*safari/.test( userAgent ) ){ // safari
browser.safari = true;
browser.appname = 'safari';
browser.version = RegExp.$2;
}
return browser.appname browser.version;
}
Object/feature detection method
This method is a general way to determine the capabilities of a browser (rather than the exact model of the browser). Most JS experts consider this approach the most appropriate because they believe scripts written this way are future-proof.
<span style="COLOR: green">//获取IE浏览器的版本号</span>
<span style="COLOR: green">//返回数值,显示IE的主版本号</span>
<span style="COLOR: blue">function </span>getIEVer() {
<span style="COLOR: blue">var </span>ua = navigator.userAgent; <span style="COLOR: green">//获取用户端信息</span>
<span style="COLOR: green"> </span><span style="COLOR: blue">var </span>b = ua.indexOf(<span style="COLOR: #a31515">"MSIE "</span>); <span style="COLOR: green">//检测特殊字符串"MSIE "的位置</span>
<span style="COLOR: green"> </span><span style="COLOR: blue">if </span>(b < 0) {
<span style="COLOR: blue">return </span>0;
}
<span style="COLOR: blue">return </span>parseFloat(ua.substring(b + 5, ua.indexOf(<span style="COLOR: #a31515">";"</span>, b))); <span style="COLOR: green">//截取版本号字符串,并转换为数值</span>
}
alert(getIEVer()); <span style="COLOR: green">//返回数值8(我的IE8)</span>
Use this method if you are more concerned about the capabilities of the browser than its actual identity.
user-agent string detection method
The user-agent string provides a wealth of information about the web browser, including the browser's name and version.
<span style="COLOR: blue">var </span>ua = navigator.userAgent.toLowerCase(); <span style="COLOR: green">//获取用户端信息</span>
<span style="COLOR: blue">var </span>info = {
ie: /msie/.test(ua) && !/opera/.test(ua), <span style="COLOR: green">//匹配IE浏览器</span>
<span style="COLOR: green"> </span>op: /opera/.test(ua), <span style="COLOR: green">//匹配Opera浏览器</span>
<span style="COLOR: green"> </span>sa: /version.*safari/.test(ua), <span style="COLOR: green">//匹配Safari浏览器</span>
<span style="COLOR: green"> </span>ch: /chrome/.test(ua), <span style="COLOR: green">//匹配Chrome浏览器</span>
<span style="COLOR: green"> </span>ff: /gecko/.test(ua) && !/webkit/.test(ua) <span style="COLOR: green">//匹配Firefox浏览器</span>
};
(info.ie) && alert(<span style="COLOR: #a31515">"IE浏览器"</span>);
(info.op) && alert(<span style="COLOR: #a31515">"Opera浏览器"</span>);
(info.sa) && alert(<span style="COLOR: #a31515">"Safari浏览器"</span>);
(info.ff) && alert(<span style="COLOR: #a31515">"Firefox浏览器"</span>);
(info.ch) && alert(<span style="COLOR: #a31515">"Chrome浏览器"</span>);
Usually what we do the most is to determine whether it is IE. Other browsers generally meet the standards. Some customers only need to comply with IE and FF. Then we can do this:
<span style="COLOR: blue">var </span>isIE = (navigator.appName == <span style="COLOR: #a31515">"Microsoft Internet Explorer"</span>);
Judging IE is far more than the above method. You can use more unique things of IE, such as: window.ActiveXObject, document.all, etc. These are all object/feature detection methods! Usually they need to be used in different browsers. If you write different styles (because IE style parsing is also different), then you have to judge the version. You can do this
<span style="COLOR: green">//获取IE浏览器的版本号</span>
<span style="COLOR: green">//返回数值,显示IE的主版本号</span>
<span style="COLOR: blue">function </span>getIEVer() {
<span style="COLOR: blue">var </span>ua = navigator.userAgent; <span style="COLOR: green">//获取用户端信息</span>
<span style="COLOR: green"> </span><span style="COLOR: blue">var </span>b = ua.indexOf(<span style="COLOR: #a31515">"MSIE "</span>); <span style="COLOR: green">//检测特殊字符串"MSIE "的位置</span>
<span style="COLOR: green"> </span><span style="COLOR: blue">if </span>(b < 0) {
<span style="COLOR: blue">return </span>0;
}
<span style="COLOR: blue">return </span>parseFloat(ua.substring(b + 5, ua.indexOf(<span style="COLOR: #a31515">";"</span>, b))); <span style="COLOR: green">//截取版本号字符串,并转换为数值</span>
}
alert(getIEVer()); <span style="COLOR: green">//返回数值7</span>
Detect operating system:
<span style="COLOR: blue">var </span>isWin = (navigator.userAgent.indexOf(<span style="COLOR: #a31515">"Win"</span>) != -1); <span style="COLOR: green">//如果是Windows系统,则返回true</span>
<span style="COLOR: blue">var </span>isMac = (navigator.userAgent.indexOf(<span style="COLOR: #a31515">"Mac"</span>) != -1); <span style="COLOR: green">//如果是Macintosh系统,则返回true</span>
<span style="COLOR: blue">var </span>isUnix = (navigator.userAgent.indexOf(<span style="COLOR: #a31515">"X11"</span>) != -1); <span style="COLOR: green">//如果是Unix系统,则返回true</span>
<span style="COLOR: blue">var </span>isLinux = (navigator.userAgent.indexOf(<span style="COLOR: #a31515">"Linux"</span>) != -1); <span style="COLOR: green">//如果是Linux系统,则返回true</span>
Most of the content of the article comes from "Javascript Journey"

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


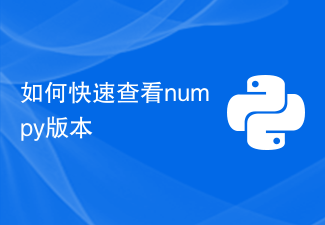
Numpy is an important mathematics library in Python. It provides efficient array operations and scientific calculation functions and is widely used in data analysis, machine learning, deep learning and other fields. When using numpy, we often need to check the version number of numpy to determine the functions supported by the current environment. This article will introduce how to quickly check the numpy version and provide specific code examples. Method 1: Use the __version__ attribute that comes with numpy. The numpy module comes with a __
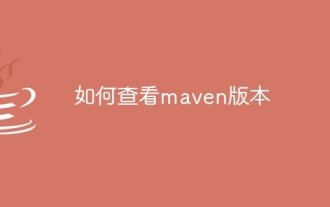
Methods to check the maven version: 1. Use the command line; 2. Check the environment variables; 3. Use the IDE; 4. Check the pom.xml file. Detailed introduction: 1. Use the command line, enter "mvn -v" or "mvn --version" in the command line, and then press Enter. This will display the Maven version information and Java version information; 2. View the environment variables , on some systems, you can check the environment variables to find the Maven version information, enter the command on the command line, and then press Enter, etc.
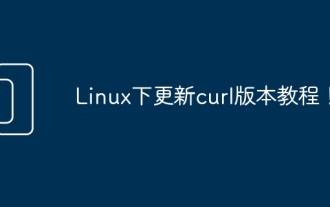
To update the curl version under Linux, you can follow the steps below: Check the current curl version: First, you need to determine the curl version installed in the current system. Open a terminal and execute the following command: curl --version This command will display the current curl version information. Confirm available curl version: Before updating curl, you need to confirm the latest version available. You can visit curl's official website (curl.haxx.se) or related software sources to find the latest version of curl. Download the curl source code: Using curl or a browser, download the source code file for the curl version of your choice (usually .tar.gz or .tar.bz2
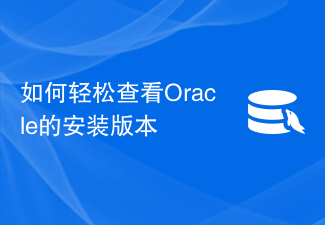
How to easily check the installed version of Oracle requires specific code examples. As a software widely used in enterprise-level database management systems, the Oracle database has many versions and different installation methods. In our daily work, we often need to check the installed version of the Oracle database for corresponding operations and maintenance. This article will introduce how to easily check the installed version of Oracle and give specific code examples. Method 1: Through SQL query in the Oracle database, we can
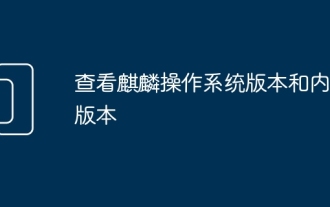
Checking the Kylin operating system version and kernel version In the Kirin operating system, knowing how to check the system version and kernel version is the basis for system management and maintenance. Method 1 to check the Kylin operating system version: Use the /etc/.kyinfo file. To check the Kylin operating system version, you can check the /etc/.kyinfo file. This file contains operating system version information. Execute the following command: cat/etc/.kyinfo This command will display detailed version information of the operating system. Method 2: Use the /etc/issue file Another way to check the operating system version is by looking at the /etc/issue file. This file also provides version information, but may not be as good as the .kyinfo file
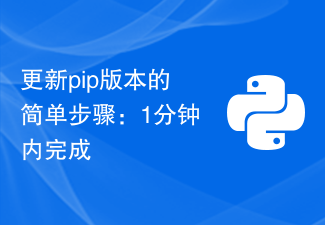
Done in one minute: How to update the pip version, specific code examples are required. With the rapid development of Python, pip has become a standard tool for Python package management. However, as time goes by, pip versions are constantly updated. In order to be able to use the latest features and fix possible security vulnerabilities, it is very important to update the pip version. This article will explain how to quickly update pip in one minute and provide specific code examples. First, we need to open a command line window. In Windows systems, you can use
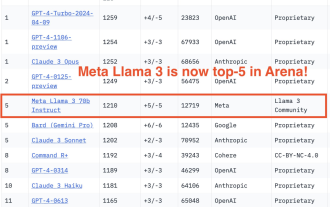
Regarding Llama3, new test results have been released - the large model evaluation community LMSYS released a large model ranking list. Llama3 ranked fifth, and tied for first place with GPT-4 in the English category. The picture is different from other benchmarks. This list is based on one-on-one battles between models, and the evaluators from all over the network make their own propositions and scores. In the end, Llama3 ranked fifth on the list, followed by three different versions of GPT-4 and Claude3 Super Cup Opus. In the English single list, Llama3 overtook Claude and tied with GPT-4. Regarding this result, Meta’s chief scientist LeCun was very happy and forwarded the tweet and
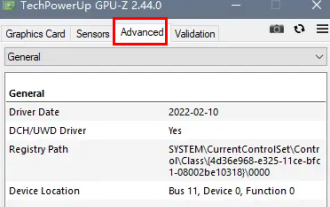
The DP interface is an important interface cable in the computer. When using the computer, many users want to know how to check whether the DP interface is 1.2 or 1.4. In fact, they only need to check it in GPU-Z. How to determine whether the dp interface is 1.2 or 1.4: 1. First, select "Advanced" in GPU-Z. 2. Look at "Monitor1" in "General" under "Advanced", you can see the two items "LinkRate (current)" and "Lanes (current)". 3. Finally, if 8.1Gbps×4 is displayed, it means DP1.3 version or above, usually DP1.4. If it is 5.4Gbps×4, then
