


Detailed explanation of JavaScript inheritance (3)_js object-oriented
Note: The implementation of jClass in this chapter refers to the practice of Simple JavaScript Inheritance.
First let us review the examples introduced in Chapter 1:
function Person(name) {<BR> this.name = name;<BR> }<BR> Person.prototype = {<BR> getName: function() {<BR> return this.name;<BR> }<BR> }<br><br> function Employee(name, employeeID) {<BR> this.name = name;<BR> this.employeeID = employeeID;<BR> }<BR> Employee.prototype = new Person();<BR> Employee.prototype.getEmployeeID = function() {<BR> return this.employeeID;<BR> };<BR> var zhang = new Employee("ZhangSan", "1234");<BR> console.log(zhang.getName()); // "ZhangSan" <BR>
Fix constructor pointing error
From the description of the constructor in the previous article, we know that the constructor of the Employee instance will have a pointing error, as shown below:
var zhang = new Employee("ZhangSan", "1234");<BR> console.log(zhang.constructor === Employee); // false<BR> console.log(zhang.constructor === Object); // true <BR>
function Employee(name, employeeID) {<BR> this.name = name;<BR> this.employeeID = employeeID;<BR> }<BR> Employee.prototype = new Person();<BR> Employee.prototype.constructor = Employee;<BR> Employee.prototype.getEmployeeID = function() {<BR> return this.employeeID;<BR> };<BR> var zhang = new Employee("ZhangSan", "1234");<BR> console.log(zhang.constructor === Employee); // true<BR> console.log(zhang.constructor === Object); // false<BR>
It is inappropriate to instantiate Person when creating the Employee class
But on the other hand, we must rely on this mechanism to implement inheritance. The solution is not to initialize the data in the constructor, but to provide a prototype method (such as init) to initialize the data.
// 空的构造函数<BR> function Person() {<BR> }<BR> Person.prototype = {<BR> init: function(name) {<BR> this.name = name;<BR> },<BR> getName: function() {<BR> return this.name;<BR> }<BR> }<BR> // 空的构造函数<BR> function Employee() {<BR> }<BR> // 创建类的阶段不会初始化父类的数据,因为Person是一个空的构造函数<BR> Employee.prototype = new Person();<BR> Employee.prototype.constructor = Employee;<BR> Employee.prototype.init = function(name, employeeID) {<BR> this.name = name;<BR> this.employeeID = employeeID;<BR> };<BR> Employee.prototype.getEmployeeID = function() {<BR> return this.employeeID;<BR> };<BR>
var zhang = new Employee();<BR> zhang.init("ZhangSan", "1234");<BR> console.log(zhang.getName()); // "ZhangSan"<BR>
How to automatically call the init function?
Two effects must be achieved: do not call the init function when constructing a class and automatically call the init function when instantiating an object. It seems that we need to have a status indicator when calling the empty constructor.
// 创建一个全局的状态标示 - 当前是否处于类的构造阶段<BR> var initializing = false;<BR> function Person() {<BR> if (!initializing) {<BR> this.init.apply(this, arguments);<BR> }<BR> }<BR> Person.prototype = {<BR> init: function(name) {<BR> this.name = name;<BR> },<BR> getName: function() {<BR> return this.name;<BR> }<BR> }<BR> function Employee() {<BR> if (!initializing) {<BR> this.init.apply(this, arguments);<BR> }<BR> }<BR> // 标示当前进入类的创建阶段,不会调用init函数<BR> initializing = true;<BR> Employee.prototype = new Person();<BR> Employee.prototype.constructor = Employee;<BR> initializing = false;<BR> Employee.prototype.init = function(name, employeeID) {<BR> this.name = name;<BR> this.employeeID = employeeID;<BR> };<BR> Employee.prototype.getEmployeeID = function() {<BR> return this.employeeID;<BR> };<br><br> // 初始化类实例时,自动调用类的原型函数init,并向init中传递参数<BR> var zhang = new Employee("ZhangSan", "1234");<BR> console.log(zhang.getName()); // "ZhangSan"<BR>
How to avoid introducing global variable initializing?
We need to introduce a global function to simplify the class creation process, while encapsulating internal details to avoid introducing global variables.
// 当前是否处于创建类的阶段<BR> var initializing = false;<BR> function jClass(baseClass, prop) {<BR> // 只接受一个参数的情况 - jClass(prop)<BR> if (typeof (baseClass) === "object") {<BR> prop = baseClass;<BR> baseClass = null;<BR> }<BR> // 本次调用所创建的类(构造函数)<BR> function F() {<BR> // 如果当前处于实例化类的阶段,则调用init原型函数<BR> if (!initializing) {<BR> this.init.apply(this, arguments);<BR> }<BR> }<BR> // 如果此类需要从其它类扩展<BR> if (baseClass) {<BR> initializing = true;<BR> F.prototype = new baseClass();<BR> F.prototype.constructor = F;<BR> initializing = false;<BR> }<BR> // 覆盖父类的同名函数<BR> for (var name in prop) {<BR> if (prop.hasOwnProperty(name)) {<BR> F.prototype[name] = prop[name];<BR> }<BR> }<BR> return F;<BR> };<BR>
var Person = jClass({<BR> init: function(name) {<BR> this.name = name;<BR> },<BR> getName: function() {<BR> return this.name;<BR> }<BR> });<BR> var Employee = jClass(Person, {<BR> init: function(name, employeeID) {<BR> this.name = name;<BR> this.employeeID = employeeID;<BR> },<BR> getEmployeeID: function() {<BR> return this.employeeID;<BR> }<BR> });<br><br> var zhang = new Employee("ZhangSan", "1234");<BR> console.log(zhang.getName()); // "ZhangSan"<BR>
How to call the method of the same name in the parent class?
We can point to the prototype of the parent class (constructor) by providing a base attribute for the instantiated object, as follows:
// 当前是否处于创建类的阶段<BR> var initializing = false;<BR> function jClass(baseClass, prop) {<BR> // 只接受一个参数的情况 - jClass(prop)<BR> if (typeof (baseClass) === "object") {<BR> prop = baseClass;<BR> baseClass = null;<BR> }<BR> // 本次调用所创建的类(构造函数)<BR> function F() {<BR> // 如果当前处于实例化类的阶段,则调用init原型函数<BR> if (!initializing) {<BR> // 如果父类存在,则实例对象的base指向父类的原型<BR> // 这就提供了在实例对象中调用父类方法的途径<BR> if (baseClass) {<BR> this.base = baseClass.prototype;<BR> }<BR> this.init.apply(this, arguments);<BR> }<BR> }<BR> // 如果此类需要从其它类扩展<BR> if (baseClass) {<BR> initializing = true;<BR> F.prototype = new baseClass();<BR> F.prototype.constructor = F;<BR> initializing = false;<BR> }<BR> // 覆盖父类的同名函数<BR> for (var name in prop) {<BR> if (prop.hasOwnProperty(name)) {<BR> F.prototype[name] = prop[name];<BR> }<BR> }<BR> return F;<BR> };<BR>
var Person = jClass({<BR> init: function(name) {<BR> this.name = name;<BR> },<BR> getName: function() {<BR> return this.name;<BR> }<BR> });<BR> var Employee = jClass(Person, {<BR> init: function(name, employeeID) {<BR> // 调用父类的原型函数init,注意使用apply函数修改init的this指向<BR> this.base.init.apply(this, [name]);<BR> this.employeeID = employeeID;<BR> },<BR> getEmployeeID: function() {<BR> return this.employeeID;<BR> },<BR> getName: function() {<BR> // 调用父类的原型函数getName<BR> return "Employee name: " + this.base.getName.apply(this);<BR> }<BR> });<br><br> var zhang = new Employee("ZhangSan", "1234");<BR> console.log(zhang.getName()); // "Employee name: ZhangSan"<BR>
So far, we have corrected the shortcomings of manual implementation of inheritance in Chapter 1. Create classes and subclasses through our custom jClass function, initialize data through the prototype method init, and call the prototype function of the parent class through the instance attribute base.
The only shortcoming is that the code for calling the parent class is too long and difficult to understand. Wouldn’t it be better if it could be called as follows:
var Employee = jClass(Person, {<BR> init: function(name, employeeID) {<BR> // 如果能够这样调用,就再好不过了<BR> this.base(name);<BR> this.employeeID = employeeID;<BR> }<BR> });<BR>
Optimize jClass function
// 当前是否处于创建类的阶段<BR> var initializing = false;<BR> function jClass(baseClass, prop) {<BR> // 只接受一个参数的情况 - jClass(prop)<BR> if (typeof (baseClass) === "object") {<BR> prop = baseClass;<BR> baseClass = null;<BR> }<BR> // 本次调用所创建的类(构造函数)<BR> function F() {<BR> // 如果当前处于实例化类的阶段,则调用init原型函数<BR> if (!initializing) {<BR> // 如果父类存在,则实例对象的baseprototype指向父类的原型<BR> // 这就提供了在实例对象中调用父类方法的途径<BR> if (baseClass) {<BR> this.baseprototype = baseClass.prototype;<BR> }<BR> this.init.apply(this, arguments);<BR> }<BR> }<BR> // 如果此类需要从其它类扩展<BR> if (baseClass) {<BR> initializing = true;<BR> F.prototype = new baseClass();<BR> F.prototype.constructor = F;<BR> initializing = false;<BR> }<BR> // 覆盖父类的同名函数<BR> for (var name in prop) {<BR> if (prop.hasOwnProperty(name)) {<BR> // 如果此类继承自父类baseClass并且父类原型中存在同名函数name<BR> if (baseClass &&<BR> typeof (prop[name]) === "function" &&<BR> typeof (F.prototype[name]) === "function") {<br><br> // 重定义函数name - <BR> // 首先在函数上下文设置this.base指向父类原型中的同名函数<BR> // 然后调用函数prop[name],返回函数结果<br><br> // 注意:这里的自执行函数创建了一个上下文,这个上下文返回另一个函数,<BR> // 此函数中可以应用此上下文中的变量,这就是闭包(Closure)。<BR> // 这是JavaScript框架开发中常用的技巧。<BR> F.prototype[name] = (function(name, fn) {<BR> return function() {<BR> this.base = baseClass.prototype[name];<BR> return fn.apply(this, arguments);<BR> };<BR> })(name, prop[name]);<br><br> } else {<BR> F.prototype[name] = prop[name];<BR> }<BR> }<BR> }<BR> return F;<BR> };<BR>
var Person = jClass({<BR> init: function(name) {<BR> this.name = name;<BR> },<BR> getName: function() {<BR> return this.name;<BR> }<BR> });<BR> var Employee = jClass(Person, {<BR> init: function(name, employeeID) {<BR> this.base(name);<BR> this.employeeID = employeeID;<BR> },<BR> getEmployeeID: function() {<BR> return this.employeeID;<BR> },<BR> getName: function() {<BR> return "Employee name: " + this.base();<BR> }<BR> });<br><br> var zhang = new Employee("ZhangSan", "1234");<BR> console.log(zhang.getName()); // "Employee name: ZhangSan"<BR>
So far, we have created a complete function jClass to help us implement classes and inheritance in JavaScript in a more elegant way.
In the following chapters, we will successively analyze the implementation of some of the more popular JavaScript classes and inheritance on the Internet. However, everything remains the same, and those implementations are nothing more than "hype" that shakes up the concepts we mentioned in this chapter, just for the sake of a more elegant way of calling.

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
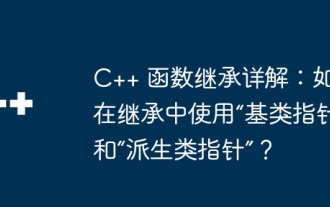
In function inheritance, use "base class pointer" and "derived class pointer" to understand the inheritance mechanism: when the base class pointer points to the derived class object, upward transformation is performed and only the base class members are accessed. When a derived class pointer points to a base class object, a downward cast is performed (unsafe) and must be used with caution.
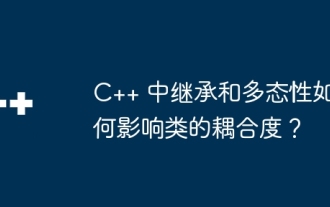
Inheritance and polymorphism affect the coupling of classes: Inheritance increases coupling because the derived class depends on the base class. Polymorphism reduces coupling because objects can respond to messages in a consistent manner through virtual functions and base class pointers. Best practices include using inheritance sparingly, defining public interfaces, avoiding adding data members to base classes, and decoupling classes through dependency injection. A practical example showing how to use polymorphism and dependency injection to reduce coupling in a bank account application.
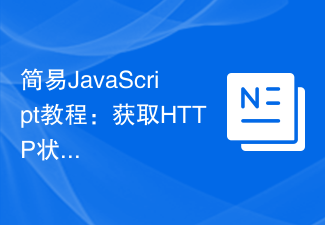
JavaScript tutorial: How to get HTTP status code, specific code examples are required. Preface: In web development, data interaction with the server is often involved. When communicating with the server, we often need to obtain the returned HTTP status code to determine whether the operation is successful, and perform corresponding processing based on different status codes. This article will teach you how to use JavaScript to obtain HTTP status codes and provide some practical code examples. Using XMLHttpRequest
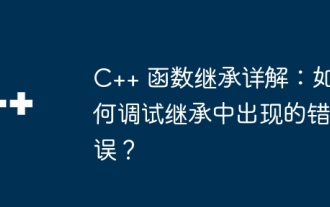
Inheritance error debugging tips: Ensure correct inheritance relationships. Use the debugger to step through the code and examine variable values. Make sure to use the virtual modifier correctly. Examine the inheritance diamond problem caused by hidden inheritance. Check for unimplemented pure virtual functions in abstract classes.
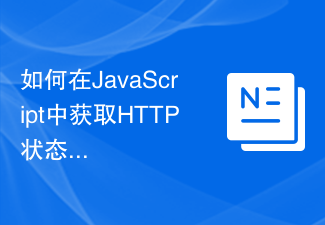
Introduction to the method of obtaining HTTP status code in JavaScript: In front-end development, we often need to deal with the interaction with the back-end interface, and HTTP status code is a very important part of it. Understanding and obtaining HTTP status codes helps us better handle the data returned by the interface. This article will introduce how to use JavaScript to obtain HTTP status codes and provide specific code examples. 1. What is HTTP status code? HTTP status code means that when the browser initiates a request to the server, the service

C++ function inheritance should not be used in the following situations: When a derived class requires a different implementation, a new function with a different implementation should be created. When a derived class does not require a function, it should be declared as an empty class or use private, unimplemented base class member functions to disable function inheritance. When functions do not require inheritance, other mechanisms (such as templates) should be used to achieve code reuse.
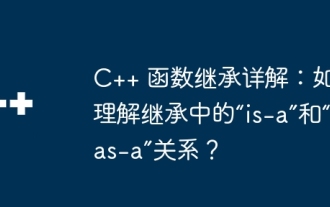
Detailed explanation of C++ function inheritance: Master the relationship between "is-a" and "has-a" What is function inheritance? Function inheritance is a technique in C++ that associates methods defined in a derived class with methods defined in a base class. It allows derived classes to access and override methods of the base class, thereby extending the functionality of the base class. "is-a" and "has-a" relationships In function inheritance, the "is-a" relationship means that the derived class is a subtype of the base class, that is, the derived class "inherits" the characteristics and behavior of the base class. The "has-a" relationship means that the derived class contains a reference or pointer to the base class object, that is, the derived class "owns" the base class object. SyntaxThe following is the syntax for how to implement function inheritance: classDerivedClass:pu
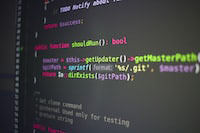
What is object-oriented programming? Object-oriented programming (OOP) is a programming paradigm that abstracts real-world entities into classes and uses objects to represent these entities. Classes define the properties and behavior of objects, and objects instantiate classes. The main advantage of OOP is that it makes code easier to understand, maintain and reuse. Basic Concepts of OOP The main concepts of OOP include classes, objects, properties and methods. A class is the blueprint of an object, which defines its properties and behavior. An object is an instance of a class and has all the properties and behaviors of the class. Properties are characteristics of an object that can store data. Methods are functions of an object that can operate on the object's data. Advantages of OOP The main advantages of OOP include: Reusability: OOP can make the code more
