高质量缩略图的生成函数(多种剪切模式,按高度宽度最佳缩放等)
函数|缩略图
/**
* 可扩展的缩略图生成函数
* 在http://yodoo.com的论坛里可以获得最新版本(注册用户)
* 演示效果也请登录http://yodoo.com看看,该站所有的缩略图(jpg,png)都是使用该函数生成的
*
* 转载请保留完整信息
*
* @author Austin Chin
* @version $Revision: 1.7 $
*
*
* version
*
* + 表示增加的功能
* - 表示丢弃的功能
* C 表示修正的功能
* E 表示扩展了功能
*
* v1.5
* makeThumb($srcFile, $dstFile, $dstW, $dstH, $option=1)
*
* v1.6
* + 增加了剪切模式
* + $option 8: 宽度最佳缩放
* + $option 16: 高度最佳缩放
* makeThumb($srcFile, $dstFile, $dstW, $dstH, $option=1, $cutmode=0, $startX=0,
* $startY=0)
*
* v1.7
* E 返回值改为数组,第一个元素是代码 0 表示正常, 其它位错误代码;第二个元素是错误描述。
* 错误代码:
* -1 源文件不存在。
* -2 不支持的图片输出函数
* -3 不支持的图片创建函数
* -4 HTTP头信息已经输出,无法向浏览器输出图片。
* -5 无法检测输出的图片类型
* + 增加函数 message2Image 可以把字符串输出成图片格式
*/
/**
* 可扩展的缩略图生成函数
*
* @param string $srcFile 源文件
* @param string $srcFile 目标文件
* @param int $dstW 目标图片的宽度(单位:像素)
* @param int $dstH 目标图片的高度(单位:像素)
* @param int $option 附加参数,可以相加使用,如1+2(或者 1|2)表示同时执行1和2的功能。
* 1: 默认,输出到指定文件 2: 图片内容输出到浏览器 4: 不保持图片比例
* 8:宽度最佳缩放 16:高度最佳缩放
* @param int $cutmode 剪切模式 0: 默认模式,剪切模式 1: 左或上 2: 中 3: 右或下
* @param int $startX 剪切的起始横坐标(像素)
* @param int $startY 剪切的起始纵坐标(像素)
* @return array return[0]=0: 正常; return[0] 为错误代码 return[1] string: 错误描述
*/
define(OP_TO_FILE, 1); //输出到指定文件
define(OP_OUTPUT, 2); //图片内容输出到浏览器
define(OP_NOT_KEEP_SCALE, 4); //不保持图片比例, 即使用拉伸
define(OP_BEST_RESIZE_WIDTH, 8); //宽度最佳缩放
define(OP_BEST_RESIZE_HEIGHT, 16); //高度最佳缩放
define(CM_DEFAULT, 0); // 默认模式
define(CM_LEFT_OR_TOP, 1); // 左或上
define(CM_MIDDLE, 2); // 中
define(CM_RIGHT_OR_BOTTOM, 3); // 右或下
function makeThumb($srcFile, $dstFile, $dstW, $dstH, $option=OP_TO_FILE,
$cutmode=CM_DEFAULT, $startX=0, $startY=0) {
$img_type = array(1=>"gif", 2=>"jpeg", 3=>"png");
$type_idx = array("gif"=>1, "jpg"=>2, "jpeg"=>2, "jpe"=>2, "png"=>3);
if (!file_exists($srcFile)) {
return array(-1, "Source file not exists: $srcFile.");
}
$path_parts = @pathinfo($dstFile);
$ext = strtolower ($path_parts["extension"]);
if ($ext == "") {
return array(-5, "Can't detect output image's type.");
}
$func_output = "image" . $img_type[$type_idx[$ext]];
if (!function_exists ($func_output)) {
return array(-2, "Function not exists for output:$func_output.");
}
$data = @GetImageSize($srcFile);
$func_create = "imagecreatefrom" . $img_type[$data[2]];
if (!function_exists ($func_create)) {
return array(-3, "Function not exists for create:$func_create.");
}
$im = @$func_create($srcFile);
$srcW = @ImageSX($im);
$srcH = @ImageSY($im);
$srcX = 0;
$srcY = 0;
$dstX = 0;
$dstY = 0;
if ($option & OP_BEST_RESIZE_WIDTH) {
$dstH = round($dstW * $srcH / $srcW);
}
if ($option & OP_BEST_RESIZE_HEIGHT) {
$dstW = round($dstH * $srcW / $srcH);
}
$fdstW = $dstW;
$fdstH = $dstH;
if ($cutmode != CM_DEFAULT) { // 剪切模式 1: 左或上 2: 中 3: 右或下
$srcW -= $startX;
$srcH -= $startY;
if ($srcW*$dstH > $srcH*$dstW) {
$testW = round($dstW * $srcH / $dstH);
$testH = $srcH;
} else {
$testH = round($dstH * $srcW / $dstW);
$testW = $srcW;
}
switch ($cutmode) {
case CM_LEFT_OR_TOP: $srcX = 0; $srcY = 0; break;
case CM_MIDDLE: $srcX = round(($srcW - $testW) / 2);
$srcY = round(($srcH - $testH) / 2); break;
case CM_RIGHT_OR_BOTTOM: $srcX = $srcW - $testW;
$srcY = $srcH - $testH;
}
$srcW = $testW;
$srcH = $testH;
$srcX += $startX;
$srcY += $startY;
} else { // 原始缩放
if (!($option & OP_NOT_KEEP_SCALE)) {
// 以下代码计算新大小,并保持图片比例
if ($srcW*$dstH>$srcH*$dstW) {
$fdstH=round($srcH*$dstW/$srcW);
$dstY=floor(($dstH-$fdstH)/2);
$fdstW=$dstW;
} else {
$fdstW=round($srcW*$dstH/$srcH);
$dstX=floor(($dstW-$fdstW)/2);
$fdstH=$dstH;
}
$dstX=($dstX
$dstY=($dstX
$dstX=($dstX>($dstW/2))?floor($dstW/2):$dstX;
$dstY=($dstY>($dstH/2))?floor($dstH/s):$dstY;
}
} /// end if ($cutmode != CM_DEFAULT) { // 剪切模式
if( function_exists("imagecopyresampled") and
function_exists("imagecreatetruecolor") ){
$func_create = "imagecreatetruecolor";
$func_resize = "imagecopyresampled";
} else {
$func_create = "imagecreate";
$func_resize = "imagecopyresized";
}
$newim = @$func_create($dstW,$dstH);
$black = @ImageColorAllocate($newim, 0,0,0);
$back = @imagecolortransparent($newim, $black);
@imagefilledrectangle($newim,0,0,$dstW,$dstH,$black);
@$func_resize($newim,$im,$dstX,$dstY,$srcX,$srcY,$fdstW,$fdstH,$srcW,$srcH);
if ($option & OP_TO_FILE) {
@$func_output($newim,$dstFile);
}
if ($option & OP_OUTPUT) {
if (function_exists("headers_sent")) {
if (headers_sent()) {
return array(-4, "HTTP already sent, can't output image to browser.");
}
}
header("Content-type: image/" . $img_type[$type_idx[$ext]]);
@$func_output($newim);
}
@imagedestroy($im);
@imagedestroy($newim);
return array(0, "OK");
}

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


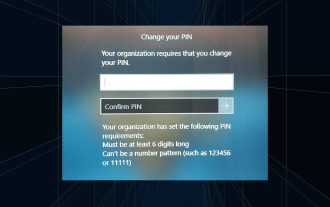
The message "Your organization has asked you to change your PIN" will appear on the login screen. This happens when the PIN expiration limit is reached on a computer using organization-based account settings, where they have control over personal devices. However, if you set up Windows using a personal account, the error message should ideally not appear. Although this is not always the case. Most users who encounter errors report using their personal accounts. Why does my organization ask me to change my PIN on Windows 11? It's possible that your account is associated with an organization, and your primary approach should be to verify this. Contacting your domain administrator can help! Additionally, misconfigured local policy settings or incorrect registry keys can cause errors. Right now
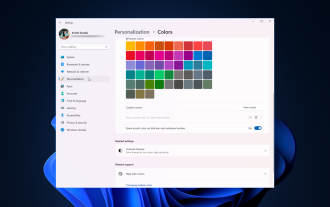
Windows 11 brings fresh and elegant design to the forefront; the modern interface allows you to personalize and change the finest details, such as window borders. In this guide, we'll discuss step-by-step instructions to help you create an environment that reflects your style in the Windows operating system. How to change window border settings? Press + to open the Settings app. WindowsI go to Personalization and click Color Settings. Color Change Window Borders Settings Window 11" Width="643" Height="500" > Find the Show accent color on title bar and window borders option, and toggle the switch next to it. To display accent colors on the Start menu and taskbar To display the theme color on the Start menu and taskbar, turn on Show theme on the Start menu and taskbar
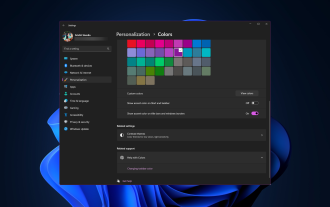
By default, the title bar color on Windows 11 depends on the dark/light theme you choose. However, you can change it to any color you want. In this guide, we'll discuss step-by-step instructions for three ways to change it and personalize your desktop experience to make it visually appealing. Is it possible to change the title bar color of active and inactive windows? Yes, you can change the title bar color of active windows using the Settings app, or you can change the title bar color of inactive windows using Registry Editor. To learn these steps, go to the next section. How to change title bar color in Windows 11? 1. Using the Settings app press + to open the settings window. WindowsI go to "Personalization" and then
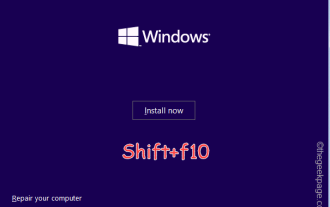
Do you see "A problem occurred" along with the "OOBELANGUAGE" statement on the Windows Installer page? The installation of Windows sometimes stops due to such errors. OOBE means out-of-the-box experience. As the error message indicates, this is an issue related to OOBE language selection. There is nothing to worry about, you can solve this problem with nifty registry editing from the OOBE screen itself. Quick Fix – 1. Click the “Retry” button at the bottom of the OOBE app. This will continue the process without further hiccups. 2. Use the power button to force shut down the system. After the system restarts, OOBE should continue. 3. Disconnect the system from the Internet. Complete all aspects of OOBE in offline mode
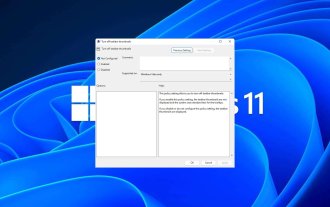
Taskbar thumbnails can be fun, but they can also be distracting or annoying. Considering how often you hover over this area, you may have inadvertently closed important windows a few times. Another disadvantage is that it uses more system resources, so if you've been looking for a way to be more resource efficient, we'll show you how to disable it. However, if your hardware specs can handle it and you like the preview, you can enable it. How to enable taskbar thumbnail preview in Windows 11? 1. Using the Settings app tap the key and click Settings. Windows click System and select About. Click Advanced system settings. Navigate to the Advanced tab and select Settings under Performance. Select "Visual Effects"
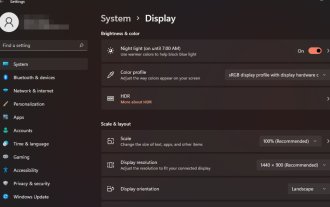
We all have different preferences when it comes to display scaling on Windows 11. Some people like big icons, some like small icons. However, we all agree that having the right scaling is important. Poor font scaling or over-scaling of images can be a real productivity killer when working, so you need to know how to customize it to get the most out of your system's capabilities. Advantages of Custom Zoom: This is a useful feature for people who have difficulty reading text on the screen. It helps you see more on the screen at one time. You can create custom extension profiles that apply only to certain monitors and applications. Can help improve the performance of low-end hardware. It gives you more control over what's on your screen. How to use Windows 11
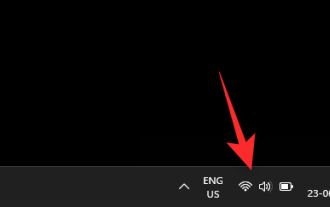
Screen brightness is an integral part of using modern computing devices, especially when you look at the screen for long periods of time. It helps you reduce eye strain, improve legibility, and view content easily and efficiently. However, depending on your settings, it can sometimes be difficult to manage brightness, especially on Windows 11 with the new UI changes. If you're having trouble adjusting brightness, here are all the ways to manage brightness on Windows 11. How to Change Brightness on Windows 11 [10 Ways Explained] Single monitor users can use the following methods to adjust brightness on Windows 11. This includes desktop systems using a single monitor as well as laptops. let's start. Method 1: Use the Action Center The Action Center is accessible
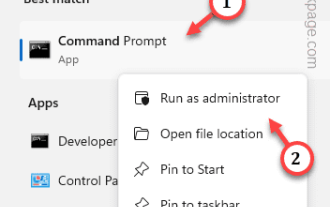
The activation process on Windows sometimes takes a sudden turn to display an error message containing this error code 0xc004f069. Although the activation process is online, some older systems running Windows Server may experience this issue. Go through these initial checks, and if they don't help you activate your system, jump to the main solution to resolve the issue. Workaround – close the error message and activation window. Then restart the computer. Retry the Windows activation process from scratch again. Fix 1 – Activate from Terminal Activate Windows Server Edition system from cmd terminal. Stage – 1 Check Windows Server Version You have to check which type of W you are using
