


Collection of options operation methods in javascript Select tag_form effects
JavaScript operates the options collection in the Select tag
Let’s first look at these methods of the options collection:
options.add(option) method adds an option object to the collection;
options.remove(index) Method removes the specified item in the options collection;
options(index) or options.item(index) can obtain the specified item in the options collection through the index;
The javascript code is as follows:
var selectTag = null; //select tag
var OPTONLENGTH = 10; //Number of options filled each time
var colls = []; //Reference to select tag options
window.onload = function(){
selectTag = document.getElementById("SelectBox"); //Get the select tag
colls = selectTag.options; //Get the reference
//initSelectBox(); //Self-initialization select.options
};
//Use random numbers to fill select.options
function initSelectBox(){
var random = 0;
var optionItem = null;
var item = null;
if(colls.length > 0 && isClearOption()){
clearOptions(colls);
}
for(var i=0;i
random = Math.floor(Math.random()*9000) 1000;
item = new Option(random,random); //Created through the Option() constructor option object
selectTag.options.add(item); //Add to the options collection
}
watchState();
}
//Whether before adding a new option item Clear the current options
function isClearOption(){
return document.getElementById("chkClear").checked;
}
//Clear the options collection
function clearOptions(colls){
var length = colls.length;
for(var i=length-1;i>=0;i--){
colls.remove(i);
}
}
//Add a new option
function addOption(){
colls.add(createOption());
lastOptionOnFocus(colls.length-1);
watchState();
}
//Create an option object
function createOption(){
var random = Math.floor(Math.random()*9000) 1000;
return new Option(random,random);
}
//Delete an option specified in the options collection
function removeOption(index){
if(index >= 0){
colls.remove(index);
lastOptionOnFocus(colls.length-1);
}
watchState();
}
//Get the currently selected option index
function getSelectedIndex(){
return selectTag. selectedIndex;
}
//Get the total number of options collection
function getOptionLength(){
return colls.length;
}
//Get the currently selected option text
function getCurrentOptionValue(index){
if(index >= 0)
return colls(index).value;
}
//Get the currently selected option value
function getCurrentOptionText( index){
if(index >= 0)
return colls(index).text;
}
//Use the last item in the options collection to get focus
function lastOptionOnFocus(index) {
selectTag.selectedIndex = index;
}
//Show the select tag status
function watchState(){
var divWatch = document.getElementById("divWatch");
var innerHtml="";
innerHtml = "Total number of options:" getOptionLength();
innerHtml = "
Current item index:" getSelectedIndex();
innerHtml = "
Current item text :" getCurrentOptionText(getSelectedIndex());
innerHtml = "
Current item value:" getCurrentOptionValue(getSelectedIndex());
divWatch.innerHTML = innerHtml;
divWatch.align = "justify";
}
Notice that when creating the option item above, the Option() constructor is used. This constructor has two versions of overloads.
1. var option = new Option(text,value); // Capitalize Option() here
2. var option = new Option();
option.text = text;
option .value=value;
I personally prefer the first method to create option objects.
In addition, the select tag also has a more useful attribute called selectedIndex, through which it is possible to obtain the currently selected option index, or to specify which item in the options collection is selected through the index setting.
select.selectedIndex = select.options.length-1; //Select the last item in the options collection
var selectedItem = select.options(select.selectedIndex);//Get the currently selected item
selectedItem .text; //The text of the selected item
selectedItem.value; //The value of the selected item
Initialize SelectBox with random numbers
clear
Add option item
Delete option item
Check whether it is selected
if(objSelect.selectedIndex > -1) {
//Description is selected
} else {
//Description is not selected
}
Delete the selected item
objSelect.options[objSelect.selectedIndex ] = null;
Add item
objSelect.options[objSelect.length] = new Option("Hello","hello");
Modify the selected item
objSelect.options[objSelect.selectedIndex] = new Option("Hello","hello");
Get the text of the selected item
objSelect.options[objSelect.selectedIndex].text;
Get the value of the selected item
objSelect.options[objSelect.selectedIndex].value;

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


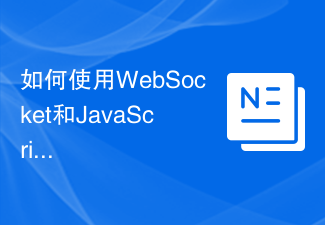
How to use WebSocket and JavaScript to implement an online speech recognition system Introduction: With the continuous development of technology, speech recognition technology has become an important part of the field of artificial intelligence. The online speech recognition system based on WebSocket and JavaScript has the characteristics of low latency, real-time and cross-platform, and has become a widely used solution. This article will introduce how to use WebSocket and JavaScript to implement an online speech recognition system.
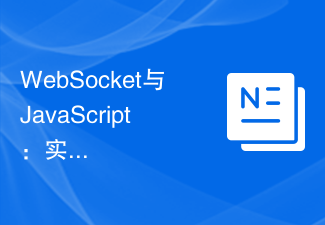
WebSocket and JavaScript: Key technologies for realizing real-time monitoring systems Introduction: With the rapid development of Internet technology, real-time monitoring systems have been widely used in various fields. One of the key technologies to achieve real-time monitoring is the combination of WebSocket and JavaScript. This article will introduce the application of WebSocket and JavaScript in real-time monitoring systems, give code examples, and explain their implementation principles in detail. 1. WebSocket technology
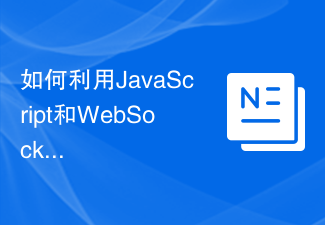
Introduction to how to use JavaScript and WebSocket to implement a real-time online ordering system: With the popularity of the Internet and the advancement of technology, more and more restaurants have begun to provide online ordering services. In order to implement a real-time online ordering system, we can use JavaScript and WebSocket technology. WebSocket is a full-duplex communication protocol based on the TCP protocol, which can realize real-time two-way communication between the client and the server. In the real-time online ordering system, when the user selects dishes and places an order
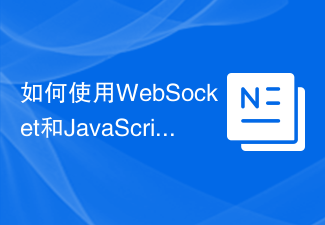
How to use WebSocket and JavaScript to implement an online reservation system. In today's digital era, more and more businesses and services need to provide online reservation functions. It is crucial to implement an efficient and real-time online reservation system. This article will introduce how to use WebSocket and JavaScript to implement an online reservation system, and provide specific code examples. 1. What is WebSocket? WebSocket is a full-duplex method on a single TCP connection.
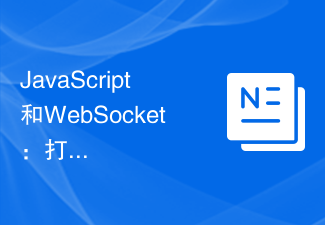
JavaScript and WebSocket: Building an efficient real-time weather forecast system Introduction: Today, the accuracy of weather forecasts is of great significance to daily life and decision-making. As technology develops, we can provide more accurate and reliable weather forecasts by obtaining weather data in real time. In this article, we will learn how to use JavaScript and WebSocket technology to build an efficient real-time weather forecast system. This article will demonstrate the implementation process through specific code examples. We
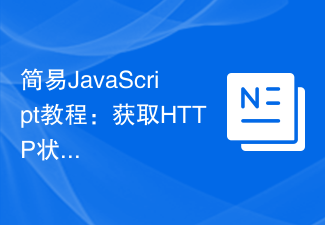
JavaScript tutorial: How to get HTTP status code, specific code examples are required. Preface: In web development, data interaction with the server is often involved. When communicating with the server, we often need to obtain the returned HTTP status code to determine whether the operation is successful, and perform corresponding processing based on different status codes. This article will teach you how to use JavaScript to obtain HTTP status codes and provide some practical code examples. Using XMLHttpRequest

Usage: In JavaScript, the insertBefore() method is used to insert a new node in the DOM tree. This method requires two parameters: the new node to be inserted and the reference node (that is, the node where the new node will be inserted).

jQuery is a popular JavaScript library that can be used to simplify DOM manipulation, event handling, animation effects, etc. In web development, we often encounter situations where we need to change event binding on select elements. This article will introduce how to use jQuery to bind select element change events, and provide specific code examples. First, we need to create a dropdown menu with options using labels:
