Experience in debugging Javascript scripts_javascript skills
As you learn more about programming in JavaScript, you will begin to understand the opaque error messages that JavaScript gives. Once you understand the common mistakes you make, you'll quickly know how to avoid them, so you'll write code with fewer and fewer errors.
Programming is actually a technology that continues to improve rapidly over time. But no matter how proficient you become, you still have to spend some time debugging your code. If you've done homework, or have experience programming in JavaScript, you'll know that a considerable amount of time is spent debugging. This is normal - it's just one of the things programmers have to do. In fact, according to numerous studies, programmers spend an average of fifty percent of their time solving bugs in their code.
The key is to learn how to debug your program effectively. I have some tips that can help you figure out why your program isn't running as it should, or help you avoid writing buggy code in the first place:
1. Print out variables in different ways
2. Watch out for common mistakes
3. Think before coding
---------------------------------------- ------------------------------------------
If JavaScript fails to capture Your mistake, you also didn't find the error by looking at the code, sometimes printing out the variables will help you. The simplest way is to use an alert() like this:
// theGreeting gets a name using getName, then presents
// one or two alert boxes depending on what the name is
// function getName()
{
var first_name = prompt("what's your first name?","");
var last_name = prompt("what's your last name?","");
var the_name = first_name " " last_name;
alert("in getName, the_name is: " the_name);
}
-------------------------------- ----------Error found----------------------------------------- ------------
1. General program errors
Most of the errors are just boring syntax errors. Remembering to close those quotes, braces, and parentheses can take a long time, but luckily JavaScript's automatic error detector catches most of these errors. Although JavaScript error detectors continue to improve as browsers become more sophisticated, some errors will still slip through. Here are some common mistakes to look out for:
2. Confusing variable or function names
Errors caused by capitalizing and pluralizing variable and function names appear annoyingly often, and sometimes JavaScript error detectors don't catch them. By establishing and sticking to a naming convention for variables and functions, the number of these headaches can be greatly reduced. For example, I define variables in all lowercase letters, replace spaces with underscores (my_variable, the_data, an_example_variable), and use built-in symbols for functions (addThreeNumbers(), writeError(), etc.). I avoid using any plurals because I always forget whether those variables are plural or not.
3. Accidental use of reserved words
Some words cannot be used as variable names because they are already used by JavaScript. For example, you can't define a variable called "if" because that's actually part of JavaScript - if you use "if", you'll run into all kinds of trouble. When you're going crazy with variables named "if", it's tempting to have a variable named "document". Unfortunately, "document" is a JavaScript object. Another frequently encountered problem is naming variables "name" (form elements have a "names" attribute). Naming a variable "name" doesn't always cause problems, it just sometimes - and it's more confusing - is why you should avoid using "name" variables.
Unfortunately, different browsers have different reserved words, so there is no way to know which words to avoid. The safest bet is to avoid using words that are already part of JavaScript and used by HTML. If you have problems with variables and can't figure out what's wrong, try changing the variable's name. If successful, you may have avoided reserved words.
4. Remember to use two equal signs when making logical judgments
Some browsers can catch this error, but some cannot. This is a very common mistake, but it's hard to spot if your browser can't point it out for you. Here is an example of this error:
var the_name = prompt("what's your name?", "");
if (the_name = "the monkey")
{
alert(" hello monkey!");
} else {
alert("hello stranger.");
}
This code will generate the "hello monkey!" alert dialog - regardless of where you are in the prompt What's knocking - this is not what we want. The reason is that there is only one equal sign in an if-then statement, which tells JavaScript that you want one thing to be equal to another. Let’s say you typed “robbie the robot” in the prompt. Initially, the value of the variable the_name is "robbie the robot", but then the if statement tells JavaScript that you want to set the_name to "the monkey." So JavaScript happily executes your command and sends a "true" message to the if-then statement, resulting in the warning dialog box showing "hello monkey!" every time. This insidious mistake can drive you crazy, so be careful to use two equal signs.
5. Accidentally quoted the variable, or forgot to quote the string
I run into this problem from time to time. The only way JavaScript distinguishes variables from strings is that strings have quotes, variables don't. There is an obvious error below:
var the_name = 'koko the gorilla';
alert("the_name is very happy");
Although the_name is a variable, the program will also generate a A warning dialog box prompting "the_name is very happy," appears. This is because once JavaScript sees something surrounded by quotes it no longer considers it, so when you put the_name in quotes, you prevent JavaScript from looking up it from memory. Here's a less obvious extension of this type of error:
Function wakeMeIn3()
{
var the_message = "Wake up! Hey! Hey! WAKE UP!!!!";
setTimeout ("alert(the_message);", 3000);
}
The problem here is that you tell JavaScript to execute alert(the_message) after three seconds. However, after three seconds the_message will no longer exist because you have exited the function. This problem can be solved like this:
function wakeMeIn3()
{
var the_message = "Wake up!";
setTimeout("alert('" the_message "');", 3000);
}
Put the_message outside the quotation marks, and the command "alert('Wakeup!');" is scheduled by setTimeout, and you can get what you want. These are just some of the hard-to-debug bugs that might be causing trouble in your code. Once they are discovered, there are different better and worse ways to correct them. You're lucky because you get to benefit from my experience and mistakes.
--------------------------------Clear errors------------- -----------------------
Finding errors, although sometimes difficult, is only the first step. Then you have to clear the error. Here are some things you should do when clearing errors:
Make a copy of your program first
Some errors are difficult to clear. In fact, sometimes when eradicating a bug, you break the entire program - one little bug drives you crazy. Saving your program before starting to debug is the best way to ensure that bugs don't take advantage of you.
Fix one error at a time
If you know there are several errors, you should fix one, check the results, and then start the next one. Fixing many errors at once without verifying your work will only invite more errors.
Beware of confusing errors
Sometimes you know there is an error but don’t really know why. Suppose there is a variable "index". For some reason "index" is always 1 less than you expect. You can do one of two things: sit there for a while and figure out why it got smaller, or just shrug; add 1 before using "index" and move on. The latter approach is called obfuscation programming. You're applying a bandage to the tape when you start thinking, "What the hell - why is index 2 instead of 3? Well... I'll make it work now and fix it later." A potential flaw.
Obfuscated programming may work in the short term, but you can see long-term doom - if you don't fully understand your code to the point where you can actually clean up the bug, that bug will come back to haunt you. It either comes back as another weird bug that you can't fix, or when the next poor damned soul reads your code, he'll find it extremely difficult to understand.
Looking for Small Bugs
Sometimes, the ability to cut and paste code is a bad thing for programmers. Typically, you'll write some JavaScript code in a function and then cut and paste it into another function. If there was a problem with the first function, now there is a problem with both functions. I'm not saying you shouldn't cut and paste code. But bugs have a way of multiplying, and if you find one bug you should look for other bugs that are similar to it. (Or know exactly what to expect before making several versions of it.) Variable name misspellings will crop up many times in a piece of JavaScript code - misspell the_name instead of teh_name in one place, and you'll have a chance of finding it elsewhere. mistake.
If all else fails
If you're sitting there staring at an error and can't figure out what's going on (or you don't see the error at all, but you know there's an error because the program doesn't run correctly ), you'd better walk away from the computer. Go read a book, take a walk around the corner, or grab a nice drink - do something, anything, but don't think about the program or the problem. This technique is called "brewing" in some situations, and it works very well. After you take a break and relax, try to find the mistake again. You'll get a clearer picture. Brewing works because it frees you from mental clutter. If you go too far down a wrong path, you sometimes find you can't turn around. In this case it is best to create a new path. I know this is infuriating, but it works. real!
If the above method is not successful...
Ask others for help. Sometimes your thinking becomes stereotyped, and only by looking at it differently can you gain insight into the problem. In a structured programming environment, programmers review each other's code regularly. This is appropriately called "code review" and will not only help eliminate errors but also result in better code. Don't be afraid to show others your JavaScript code, it will make you a better JavaScript programmer.
But the absolute best way to eliminate errors is...
Create error-free code in the first place.
--------------------------------Create error-free code---------- ----------------------------
The key to programming well is that programs are written for people, not for computers. If you can understand that others may read your JavaScript, you'll write cleaner code. The clearer the code, the less likely you are to make mistakes. Clever code is lovely, but it's this clever code that creates bugs. The best rule of thumb is KISS, which stands for Keep It Simple, Sweetie. Another helpful technique is to comment before writing code. This forces you to think before you act. Once the comment is written, you can write code underneath it.
Here is an example of writing a function in this way:
Step 1: Write comments
Step 2: Fill in the code
This strategy of writing comments first not only forces you to write comments before writing code Think and make the coding process look easier - By breaking the task into small, easy-to-code pieces, your problem will look less like Mount Everest and more like a group of pleasantly rolling hills.
Finally... always end each of your statements with a semicolon.
Although not strictly required, you should get into the habit of ending each statement with a semicolon to avoid having code after that line. Forget the semicolon and the next line of good code will suddenly produce an error. Initialize variables to "var" unless you have a better reason not to. Using "var" to localize variables reduces the chance of confusing one function with another unrelated function.
Okay, now that you know how to code, let’s learn how to make
your JavaScript run fast. >>
------------------------------------------------ ----------------
Optimize JavaScript code according to speed
1. Limit the workload within the loop
2. Customize the if-then-else statement to the most likely The most unlikely sequence
3. Minimize repeated expressions

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


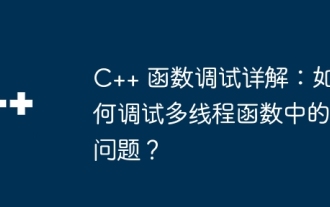
C++ multi-thread debugging can use GDB: 1. Enable debugging information compilation; 2. Set breakpoints; 3. Use infothreads to view threads; 4. Use thread to switch threads; 5. Use next, stepi, and locals to debug. Actual case debugging deadlock: 1. Use threadapplyallbt to print the stack; 2. Check the thread status; 3. Single-step the main thread; 4. Use condition variables to coordinate access to solve the deadlock.
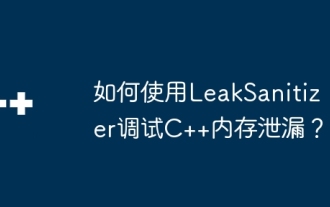
How to use LeakSanitizer to debug C++ memory leaks? Install LeakSanitizer. Enable LeakSanitizer via compile flag. Run the application and analyze the LeakSanitizer report. Identify memory allocation types and allocation locations. Fix memory leaks and ensure all dynamically allocated memory is released.
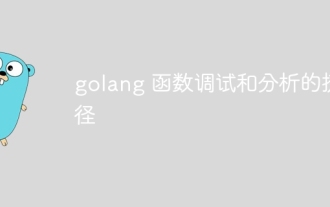
This article introduces shortcuts for Go function debugging and analysis, including: built-in debugger dlv, which is used to pause execution, check variables, and set breakpoints. Logging, use the log package to record messages and view them during debugging. The performance analysis tool pprof generates call graphs and analyzes performance, and uses gotoolpprof to analyze data. Practical case: Analyze memory leaks through pprof and generate a call graph to display the functions that cause leaks.

Efficiently debug Lambda expressions: IntelliJ IDEA Debugger: Set breakpoints on variable declarations or methods, inspect internal variables and state, and see the actual implementation class. Java9+JVMTI: Connect to the runtime JVM to obtain identifiers, inspect bytecode, set breakpoints, and monitor variables and status during execution.
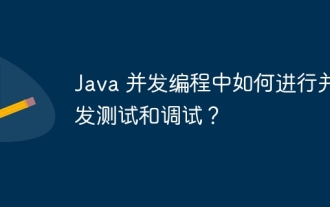
Concurrency testing and debugging Concurrency testing and debugging in Java concurrent programming are crucial and the following techniques are available: Concurrency testing: Unit testing: Isolate and test a single concurrent task. Integration testing: testing the interaction between multiple concurrent tasks. Load testing: Evaluate an application's performance and scalability under heavy load. Concurrency Debugging: Breakpoints: Pause thread execution and inspect variables or execute code. Logging: Record thread events and status. Stack trace: Identify the source of the exception. Visualization tools: Monitor thread activity and resource usage.
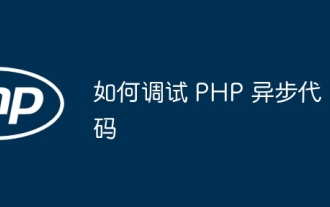
Tools for debugging PHP asynchronous code include: Psalm: a static analysis tool that can find potential errors. ParallelLint: A tool that inspects asynchronous code and provides recommendations. Xdebug: An extension for debugging PHP applications by enabling a session and stepping through the code. Other tips include using logging, assertions, running code locally, and writing unit tests.
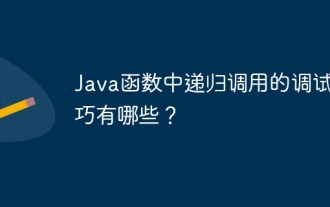
The following techniques are available for debugging recursive functions: Check the stack traceSet debug pointsCheck if the base case is implemented correctlyCount the number of recursive callsVisualize the recursive stack
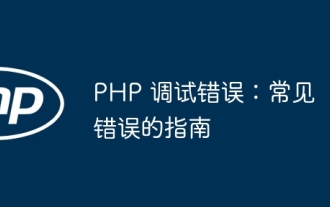
Common PHP debugging errors include: Syntax errors: Check the code syntax to make sure there are no errors. Undefined variable: Before using a variable, make sure it is initialized and assigned a value. Missing semicolons: Add semicolons to all code blocks. Function is undefined: Check that the function name is spelled correctly and make sure the correct file or PHP extension is loaded.
