


Javascript combines cookies to realize browsing history_javascript skills
I recently encountered a problem at work. There is a page that needs to add a browsing history function. Specifically, it needs to record the user’s click history on this website and sort them in descending order (only the first 6 browsing histories are displayed. and cannot be repeated).
Because I didn’t know JavaScript well enough before, I was at a loss for a while.
Later, after receiving the guidance of two master colleagues (the admiration for these two colleagues is like an endless river...), I suddenly realized and became enlightened.
Successfully completed adding this feature.
First, let’s introduce some objects and methods about this function in JavaScript:
1. window.event object:
Event represents the status of the event, such as the element that triggered the event object, the position and status of the mouse, the key pressed, etc.
The event object is only valid during the event.
2. event.srcElement:
indicates the source of the event. In layman’s terms, it is the place where the event is triggered.
3. srcElement.parentNode:
represents the parent node of the source of the event
4. srcElement.tagName:
Indicates the tag name of the event source
5. toUpperCase():
How to capitalize the corresponding string
Basically these are these properties and methods. These objects may be a bit unfamiliar to friends who have just come into contact with JavaScript or those who have rarely used such functions before, but it doesn’t matter. After understanding it, it is not difficult to find out. , not much different from JavaScript validation forms and the like.
The following will give you a step-by-step explanation based on the procedure (the procedure will inevitably have unreasonable points, I hope everyone will correct me and make progress together):
Part 1: JavaScript records browsing actions.
Copy code The code is as follows:
function glog(evt) //Define the function that records mouse click actions
{
evt=evt?evt:window.event;var srcElem=(evt.target)?evt.target:evt.srcElement;
try
{
while(srcElem.parentNode&&srcElem!=srcElem.parentNode)
//The above statement determines whether the mouse action occurs in a valid area to prevent the user's invalid clicks from being recorded.
{
if(srcElem.tagName&&srcElem.tagName.toUpperCase()=="A")//Determine whether the object clicked by the user is a link
{
linkname=srcElem.innerHTML; //Get the name of the event source, which is the text between and , which is the link name.
address=srcElem.href "_www.achome.cn_"; //Get the href value of the event source, which is the address of the link
wlink=linkname " " address; //Integrate the link name and link address into one variable
old_info=getCookie("history_info"); //Get the previously recorded browsing history from Cookies, there is a statement after this function
//The following program starts to determine whether the new browsing action is repeated with the previous 6 historical actions. If it is not repeated, it will be written to cookies.
var insert=true;
if(old_info==null) //Determine whether the cookie is empty
{
insert=true;
}
else
{
var old_link=old_info.split("_www.achome.cn_");
for(var j=0;j<=5;j )
{
if(old_link[j].indexOf(linkname)!=-1)
insert=false;
if(old_link[j]=="null")
break;
}
}
if(insert)
{
wlink =getCookie("history_info");
setCookie("history_info",wlink); //Write cookie, there is a statement after this function
history_show().reload();
break;
}
}
srcElem = srcElem.parentNode;
}
}
catch(e){}
return true;
}
document.onclick=glog; // Make every click on the page execute the glog function
Part 2: Cookies related functions.
Copy code The code is as follows:
//Cookie related functions
//Read the content specified in the cookie
function getCookieVal (offset) {
var endstr = document.cookie.indexOf (";", offset);
if (endstr == -1) endstr = document.cookie.length;
return unescape(document.cookie.substring(offset, endstr));
}
function getCookie (name) {
var arg = name "=";
var alen = arg.length;
var clen = document.cookie.length;
var i = 0;
while (i < clen) {
var j = i alen;
if (document.cookie.substring(i, j) == arg) return getCookieVal (j);
i = document.cookie.indexOf(" ", i) 1;
if (i == 0) break;
}
return null;
}
//Write browsing actions into cookies
function setCookie (name, value) {
var exp = new Date();
exp.setTime (exp.getTime() 3600000000);
document.cookie = name "=" value "; expires=" exp.toGMTString();
}
Part 3: Page display function.
Copy code The code is as follows:
function history_show()
{
var history_info=getCookie("history_info"); //Get the history record in the cookie
var content=""; //Define a display variable
if(history_info!=null)
{
history_arg=history_info.split("_www.achome.cn_");
var i;
for(i=0;i<=5;i )
{
if(history_arg[i]!="null")
{
var wlink=history_arg[i].split(" ");
content =("↑" "" wlink[0 ] "
");
}
document.getElementById("history").innerHTML=content;
}
}
else
{document.getElementById("history").innerHTML="Sorry, you don't have any browsing history";}
}
View effect:
<html> <body> <script> //cookie的相关函数 function getCookieVal (offset) { var endstr = document.cookie.indexOf (";", offset); if (endstr == -1) endstr = document.cookie.length; return unescape(document.cookie.substring(offset, endstr)); } function getCookie (name) { var arg = name + "="; var alen = arg.length; var clen = document.cookie.length; var i = 0; while (i < clen) { var j = i + alen; if (document.cookie.substring(i, j) == arg) return getCookieVal (j); i = document.cookie.indexOf(" ", i) + 1; if (i == 0) break; } return null; } function setCookie (name, value) { var exp = new Date(); exp.setTime (exp.getTime()+3600000000); document.cookie = name + "=" + value + "; expires=" + exp.toGMTString(); } //////////////////////////////////// function glog(evt) { evt=evt?evt:window.event;var srcElem=(evt.target)?evt.target:evt.srcElement; try { while(srcElem.parentNode&&srcElem!=srcElem.parentNode) { if(srcElem.tagName&&srcElem.tagName.toUpperCase()=="A") { linkname=srcElem.innerHTML; address=srcElem.href+"_www.achome.cn_"; wlink=linkname+"+"+address; old_info=getCookie("history_info"); var insert=true; //////////////////////// if(old_info==null) //判断cookie是否为空 { insert=true; } else { var old_link=old_info.split("_www.achome.cn_"); for(var j=0;j<=5;j++) { if(old_link[j].indexOf(linkname)!=-1) insert=false; if(old_link[j]=="null") break; } } ///////////////////////////// if(insert) //如果符合条件则重新写入数据 { wlink+=getCookie("history_info"); setCookie("history_info",wlink); history_show().reload(); break; } } srcElem = srcElem.parentNode; } } catch(e){} return true; } document.onclick=glog; //////////////////////////////////////////////////////////////////////////////// function history_show() { var history_info=getCookie("history_info"); var content=""; if(history_info!=null) { history_arg=history_info.split("_www.achome.cn_"); var i; for(i=0;i<=5;i++) { if(history_arg[i]!="null") { var wlink=history_arg[i].split("+"); content+=("<font color='#ff000'>↑</font>"+"<a href='"+wlink[1]+"' target='_blank'>"+wlink[0]+"</a> "); } document.getElementById("history").innerHTML=content; } } else {document.getElementById("history").innerHTML="对不起,您没有任何浏览纪录";} } // JavaScript Document </script> <div>浏览历史排行(只显示6个最近访问站点并且没有重复的站点出现)</div> <div id="history"> <script> history_show(); </script> </div> <div> 点击链接: <a href="#">网站1</a> <a href="#">网站2</a> <a href="#">网站3</a> <a href="#">网站4</a> <a href="#">网站5</a> <a href="#">网站6</a> <a href="#">网站7</a> <a href="#">网站8</a> <a href="#">网站9</a> </div> <div>如果有其他疑问请登陆<a href=http://www.jb51.net>脚本之家</a>与我联系</div> </body> </html>

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
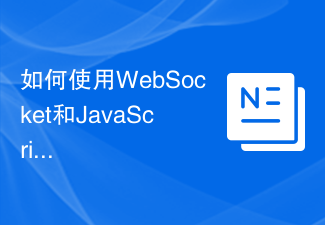
How to use WebSocket and JavaScript to implement an online speech recognition system Introduction: With the continuous development of technology, speech recognition technology has become an important part of the field of artificial intelligence. The online speech recognition system based on WebSocket and JavaScript has the characteristics of low latency, real-time and cross-platform, and has become a widely used solution. This article will introduce how to use WebSocket and JavaScript to implement an online speech recognition system.
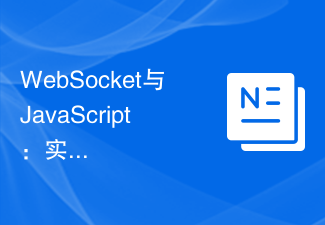
WebSocket and JavaScript: Key technologies for realizing real-time monitoring systems Introduction: With the rapid development of Internet technology, real-time monitoring systems have been widely used in various fields. One of the key technologies to achieve real-time monitoring is the combination of WebSocket and JavaScript. This article will introduce the application of WebSocket and JavaScript in real-time monitoring systems, give code examples, and explain their implementation principles in detail. 1. WebSocket technology
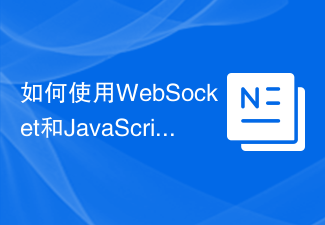
How to use WebSocket and JavaScript to implement an online reservation system. In today's digital era, more and more businesses and services need to provide online reservation functions. It is crucial to implement an efficient and real-time online reservation system. This article will introduce how to use WebSocket and JavaScript to implement an online reservation system, and provide specific code examples. 1. What is WebSocket? WebSocket is a full-duplex method on a single TCP connection.
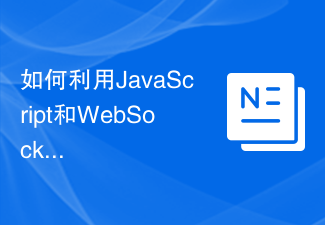
Introduction to how to use JavaScript and WebSocket to implement a real-time online ordering system: With the popularity of the Internet and the advancement of technology, more and more restaurants have begun to provide online ordering services. In order to implement a real-time online ordering system, we can use JavaScript and WebSocket technology. WebSocket is a full-duplex communication protocol based on the TCP protocol, which can realize real-time two-way communication between the client and the server. In the real-time online ordering system, when the user selects dishes and places an order
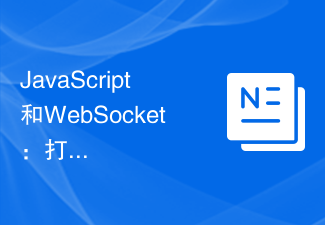
JavaScript and WebSocket: Building an efficient real-time weather forecast system Introduction: Today, the accuracy of weather forecasts is of great significance to daily life and decision-making. As technology develops, we can provide more accurate and reliable weather forecasts by obtaining weather data in real time. In this article, we will learn how to use JavaScript and WebSocket technology to build an efficient real-time weather forecast system. This article will demonstrate the implementation process through specific code examples. We
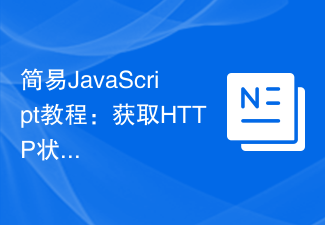
JavaScript tutorial: How to get HTTP status code, specific code examples are required. Preface: In web development, data interaction with the server is often involved. When communicating with the server, we often need to obtain the returned HTTP status code to determine whether the operation is successful, and perform corresponding processing based on different status codes. This article will teach you how to use JavaScript to obtain HTTP status codes and provide some practical code examples. Using XMLHttpRequest
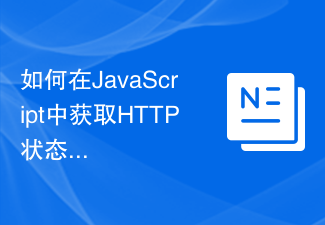
Introduction to the method of obtaining HTTP status code in JavaScript: In front-end development, we often need to deal with the interaction with the back-end interface, and HTTP status code is a very important part of it. Understanding and obtaining HTTP status codes helps us better handle the data returned by the interface. This article will introduce how to use JavaScript to obtain HTTP status codes and provide specific code examples. 1. What is HTTP status code? HTTP status code means that when the browser initiates a request to the server, the service

Usage: In JavaScript, the insertBefore() method is used to insert a new node in the DOM tree. This method requires two parameters: the new node to be inserted and the reference node (that is, the node where the new node will be inserted).
