JQuery code to implement custom dialog box_jquery
In order to get richer custom dialog box functions, I wrote a dialog box plug-in using JQuery; you can get rich custom dialog box functions by simply quoting the relevant JavaScript.
Plug-in features:
Allows appearance control through CSS.
You can display any element of the page as a dialog box.
When the dialog box is activated, any elements outside the dialog box cannot accept mouse operations.
The dialog box can be moved arbitrarily within the work area, and the centering function is adaptive according to the size of the work area.
There is no need to write complex JavaScript, just describe it through simple HTML attributes.
After testing, the plug-in can work normally under Firefox and IE.
Simple usage description:
Quote JQuery and dialog plug-in files:
< ;script src=messageBox.js>
Define related buttons as rows:
Define the content displayed in the relevant dialog box:
/* JQuery 模式对话框插件
* writer: FanJianHan (henryfan@msn.com)
* License: GPL (GPL-LICENSE.txt) licenses.
*/
//是否已初始化过对话框
var MessageOninit = false;
//记录body滚动条的x,y偏移量;显示内容的元素对象,显示内容元素对象的父对象
var MessageBox_scrolltop,MessageBox_scrollleft,Messagebox_AC,MessageBox_PC;
//对话框对象,对话框宽度,对话框高度
var MessageBox_win,MessageBox_width,MessageBox_height;
//对话框是否处于移动状态
var MessageBox_Moving = false;
//显示模式提示框
function ShowMessageBox(option)
{
var container,iframe,enabled,enabledframe;
var height=400;
var width =400;
MessageBox_scrolltop =0;
MessageBox_scrollleft =0;
if(!MessageOninit)
{
CreateContainer(option);
MessageOninit = true;
$('#messagebox_close').click(function(){
CloseMessageBox();
});
$(window).resize(function(){
SetStyle(option);
SetEnabledStyle();
});
$(window).scroll(function(e){
MessageBox_scrolltop =document.documentElement.scrollTop;
MessageBox_scrollleft = document.documentElement.scrollLeft;
SetEnabledStyle();
});
MessageBox_win = $(”#messagebox_win”);
$('#messagebox_title').mousedown(handleMouseDown);
$('#messagebox_title').mouseup(handleMouseUp);
$('#messagebox_title').mousemove(handleMouseMove);
document.onmouseup = handleMouseUp;
}
if(option.height)
height = parseInt(option.height);
if(option.width)
width = parseInt(option.width);
MessageBox_height = height;
MessageBox_width = width;
Messagebox_AC = $('#'+option.control);
MessageBox_PC = Messagebox_AC.parent();
Messagebox_AC.css('display',”);
enabled=''
enabledframe='';
$('#messagebox_enabledframe').remove()_
$('#messagebox_enabled').remove();
$('#messagebox_title').html(option.title);
$('#messagebox_from').append(Messagebox_AC);
SetStyle(option);
$(document.body).append(enabledframe);
$(document.body).append(enabled);
SetEnabledStyle();
$('#messagebox_win').fadeIn(”slow”);
//创建对话框容器
function CreateContainer(option)
{
var html;
html='
if(option.parent)
{
$('#' + option.parent).append(html);
}
else
{
$(document.body).append(html);
}
}
//设置显示时背景式样
function SetEnabledStyle()
{
var de,w,h,css,region;
region = GetDocumentRegion();
css ={width:region.width+”px”,height:region.height+”px”,
left: MessageBox_scrollleft+'px',top: MessageBox_scrolltop +'px'}
GetOpacity(css);
$(”#messagebox_enabled”).css(css);
$(”#messagebox_enabledframe”).css(css);
}
//设置透明式样
function GetOpacity(css)
{
if(window.navigator.userAgent.indexOf('MSIE')>=1)
{
css.filter= ‘progid:DXImageTransform.Microsoft.Alpha(opacity=30)';
}
else
{
css.opacity= ‘0.3′;
}
}
//设置对话框试样
function SetStyle(option)
{
var region,css;
region = GetDocumentRegion();
css ={width:MessageBox_width+'px',height:MessageBox_height+'px',
left: ((region.width - MessageBox_width)/2)+'px',top: ((region.height - MessageBox_height)/2)+'px'}
if(region.height < MessageBox_height )//如果body显示的高度小于对话框高度
{
css.top=10+'px';
}
else
{
css.top=((region.height - MessageBox_height)/2)+'px'
}
$('#messagebox_win').css(css);
css.top='0px';
css.left='0px';
$('#messagebox_table').css(css);
css.width='100%';
css.height='16px';
$('#messagebox_title_td').css(css);
css.height= height-46 +'px';
$('#messagebox_body_td').css(css);
}
var down_x,down_y,cx,cy;
function handleMouseDown(e)
{
var evt = e || event;
down_x=evt.clientX;
down_y = evt.clientY;
cx =(parseInt(MessageBox_win.css('left'))|0);
cy = (parseInt(MessageBox_win.css('top'))|0)
MessageBox_Moving= true;³
document.documentElement.onselectstart = function(){return false};
document.documentElement.ondrag = function(){return false};
document.onmousemove = handleMouseMove;
$(document.body).append('');
$('#messagebox_move').css('width',MessageBox_win.css('width'));
$('#messagebox_move').css('height',MessageBox_win_u99 ?ss('height'));
$('#messagebox_move').css('left',MessageBox_win.css('left'));
$('#messagebox_move').css('top',MessageBox_win.css('top'));
}
function GetDocumentRegion()
{
var w,h,de;
de = document.documentElement;
w = self.innerWidth || (de&&de.clientWidth) || document.body.clientWidth;
h = self.innerHeight || (de&&de.clientHeight)|| document.body.clientHeight;
return {height:h,width:w};
}
function handleMouseMove(e)
{
var left,top,region;
if (MessageBox_Moving)
{
var evt = e || event;
left =evt.clientX cx-down_x;
top = evt.clientY cy-down_y;
region = GetDocumentRegion();
if(left MessageBox_width > region.width)
{
left = region.width - 10- MessageBox_width;
}
if(top MessageBox_height >region.height)³
{
top = region.height-10 - MessageBox_height;
}
if(left <10)
left =10;
if(top <10)
top =10;
var css ={left:left 'px',top:top 'px'}
$('#messagebox_move').css(css);
}
}
function handleMouseUp()
{
if(MessageBox_Moving)
{
MessageBox_win.css('width',$('#messagebox_move').css(”width”));
MessageBox_win.css('height',$('#messagebox_move').css(”height”));
MessageBox_win.css('left',$('#messagebox_move').css(”left”));
MessageBox_win.css('top',$('#messagebox_move').css(”top”));
}
MessageBox_Moving _u61 ? false;
document.onmousemove = null;
$('#messagebox_move').remove();
}
}
//关闭模式对话框
function CloseMessageBox()
{
if(MessageOninit)
{
$('#messagebox_win').hide();
$('#messagebox_enabled').remove();
$('#messagebox_enabledframe').remove();
Messagebox_AC.css('display','none');
MessageBox_PC.append(Messagebox_AC);
}
document.documentElement.onselectstart = null;
document.documentElement.ondrag = null;
}
$(document).ready(function(){
$(document).find('[@showoption]‘).each(function(){
var namevalue;
//虽然显示的元素id,显示宽度,显示高度,标题,对话框寄居的元素对象id
var option={control:”,width:'400′,height:'400′,title:”,parent:null};
var properties = $(this).attr('showoption').split(';');
for(i=0;i
{
namevalue = properties[i].split(':');
if(namevalue.length >1)
{
execute =”option.” namevalue[0] '=” namevalue[1] '';';
eval(execute);
}
}
$(this).click(function(){
ShowMessageBox(option);
document.body.focus();
});
});
});


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


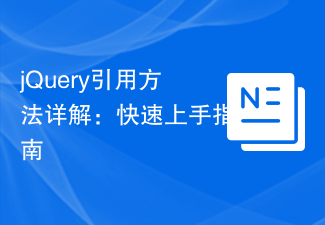
Detailed explanation of jQuery reference method: Quick start guide jQuery is a popular JavaScript library that is widely used in website development. It simplifies JavaScript programming and provides developers with rich functions and features. This article will introduce jQuery's reference method in detail and provide specific code examples to help readers get started quickly. Introducing jQuery First, we need to introduce the jQuery library into the HTML file. It can be introduced through a CDN link or downloaded
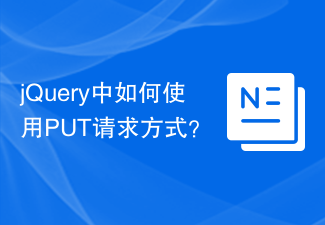
How to use PUT request method in jQuery? In jQuery, the method of sending a PUT request is similar to sending other types of requests, but you need to pay attention to some details and parameter settings. PUT requests are typically used to update resources, such as updating data in a database or updating files on the server. The following is a specific code example using the PUT request method in jQuery. First, make sure you include the jQuery library file, then you can send a PUT request via: $.ajax({u
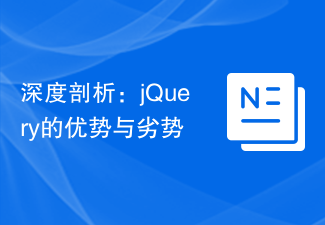
jQuery is a fast, small, feature-rich JavaScript library widely used in front-end development. Since its release in 2006, jQuery has become one of the tools of choice for many developers, but in practical applications, it also has some advantages and disadvantages. This article will deeply analyze the advantages and disadvantages of jQuery and illustrate it with specific code examples. Advantages: 1. Concise syntax jQuery's syntax design is concise and clear, which can greatly improve the readability and writing efficiency of the code. for example,
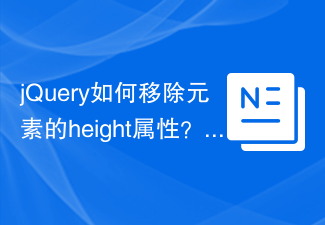
How to remove the height attribute of an element with jQuery? In front-end development, we often encounter the need to manipulate the height attributes of elements. Sometimes, we may need to dynamically change the height of an element, and sometimes we need to remove the height attribute of an element. This article will introduce how to use jQuery to remove the height attribute of an element and provide specific code examples. Before using jQuery to operate the height attribute, we first need to understand the height attribute in CSS. The height attribute is used to set the height of an element
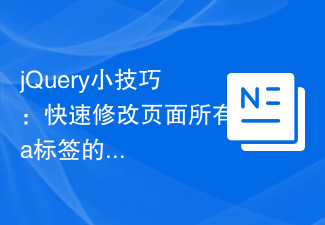
Title: jQuery Tips: Quickly modify the text of all a tags on the page In web development, we often need to modify and operate elements on the page. When using jQuery, sometimes you need to modify the text content of all a tags in the page at once, which can save time and energy. The following will introduce how to use jQuery to quickly modify the text of all a tags on the page, and give specific code examples. First, we need to introduce the jQuery library file and ensure that the following code is introduced into the page: <
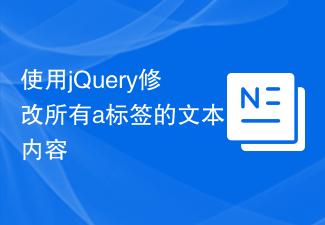
Title: Use jQuery to modify the text content of all a tags. jQuery is a popular JavaScript library that is widely used to handle DOM operations. In web development, we often encounter the need to modify the text content of the link tag (a tag) on the page. This article will explain how to use jQuery to achieve this goal, and provide specific code examples. First, we need to introduce the jQuery library into the page. Add the following code in the HTML file:
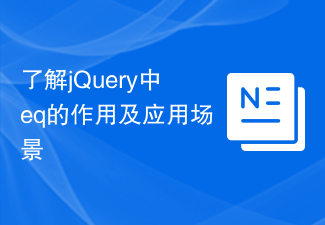
jQuery is a popular JavaScript library that is widely used to handle DOM manipulation and event handling in web pages. In jQuery, the eq() method is used to select elements at a specified index position. The specific usage and application scenarios are as follows. In jQuery, the eq() method selects the element at a specified index position. Index positions start counting from 0, i.e. the index of the first element is 0, the index of the second element is 1, and so on. The syntax of the eq() method is as follows: $("s
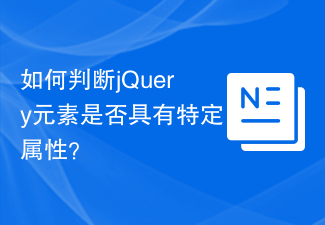
How to tell if a jQuery element has a specific attribute? When using jQuery to operate DOM elements, you often encounter situations where you need to determine whether an element has a specific attribute. In this case, we can easily implement this function with the help of the methods provided by jQuery. The following will introduce two commonly used methods to determine whether a jQuery element has specific attributes, and attach specific code examples. Method 1: Use the attr() method and typeof operator // to determine whether the element has a specific attribute
