More detailed javascript DOM study notes_javascript skills
After reading a lot of js dom learning materials, I found this one is quite detailed, so I reprint it for everyone to learn about
1. DOM basics
1. Node level
Document--the most The top-level node to which all other nodes are attached.
DocumentType - the object representation of a DTD reference (using the syntax), which cannot contain child nodes.
DocumentFragment - can save other nodes like Document.
Element - represents the content between the start tag and the end tag, such as
Attr - represents a pair of attribute names and attribute values. This node type cannot contain child nodes.
Text - represents the ordinary text between the start tag and the end tag in the XML document, or contained in the CDataSection. This node type cannot contain child nodes.
CDataSection - the object representation of . This node type can only contain text nodes Text as child nodes.
Entity - represents an entity definition in the DTD, such as . This node type cannot contain child nodes.
EntityReference - represents an entity reference, such as ". This node type cannot contain child nodes.
ProcessingInstruction - represents a PI. This node type cannot contain child nodes.
Comment - represents XML comments. This node cannot contain child nodes.
Notation - represents the notation defined in the DTD. The
Node interface defines properties and methods that are included in all node types.
In addition to nodes, DOM also defines some helper objects, which can be used with nodes, but are not a necessary part of the DOM document.
NodeList - Node array, indexed according to value; used to represent the child nodes of an element.
NamedNodeMap - a node table indexed by both values and names; used to represent element characteristics.
2. Access related nodes
Consider the following HTML page in the following sections
<html> <head> <title>DOM Example</title> </head> <body> <p>Hello World!</p> <p>Isn't this exciting?</p> <p>You're learning to use the DOM!</p> </body> </html>
To access the
element (you should understand that this is the document element of the document), you can use the documentElement attribute of document:var oHtml = document.documentElement;
Now The variable oHtml contains an HTMLElement object representing
var oHead = oHtml.firstChild;
var oBody = oHtml.lastChild;
can also be used childNodes attribute to accomplish the same job. Just treat it as a normal javascript array, marked with square brackets:
var oHead = oHtml.childNodes[0];
var oBody = oHtml.childNodes[1];
Note that the square brackets are actually Simple implementation of NodeList in javascript. In fact, the official way to get child nodes from the childNodes list is to use the item() method:
var oHead = oHtml.childNodes.item(0);
var oBody = oHtml.childNodes.item(1);
The HTML DOM page defines document.body as a pointer to the
var oBody = document.body;
With the three variables oHtml, oHead and oBody, you can try to determine the relationship between them:
alert(oHead.parentNode==oHtml);
alert(oBody.parentNode==oHtml);
alert(oBody.previousSibling==oHead);
alert(bHead.nextSibling==oBody);
alert(oHead.ownerDocument==document);
The above all outputs "true".
3. Processing attributes
As mentioned earlier, even though the Node interface already has the attributes method and has been inherited by all types of nodes, however, only the
Element node can have attributes. The attributes attribute of the Element node is actually NameNodeMap, which provides some methods for accessing and processing its content:
getNamedItem(name)--returns the node whose nodename attribute value is equal to name;
removeNamedItem(name)--delete A node whose nodename attribute value is equal to name;
setNamedItem(node)--add node to the list and index according to its nodeName attribute;
item(pos)--like NodeList, return the node at position pos ;
Note: Please remember that these methods return an Attr node, not an attribute value.
The NamedNodeMap object also has a length attribute to indicate the number of nodes it contains.
When NamedNodeMap is used to represent attributes, where each node is an Attr node, the nodeName attribute is set to the attribute name, and the nodeValue attribute is set to the attribute value. For example, suppose there is such an element:
Hello world!
At the same time, suppose the variable oP contains a pointer to this element Quote. So you can access the value of the id attribute like this:
var sId = oP.attributes.getNamedItem("id").nodeValue;
Of course, you can also access the id attribute numerically, but this is a little unintuitive:
var sId = oP.attributes.item(1).nodeValue;
You can also change the id attribute by assigning a new value to the nodeValue attribute:
oP.attributes.getNamedItem("id").nodeValue=" newId";
Attr node also has a value attribute that is completely equivalent to (and completely synchronized with) the nodeValue attribute, and has a name attribute that is synchronized with the nodeName attribute. We are free to use these properties to modify or change properties.
Because this method is somewhat cumbersome, DOM defines three element methods to help access attributes:
getAttribute(name) - equal to attributes.getNamedItem(name).value;
setAttribute(name,newvalue) --Equal to attribute.getNamedItem(name).value=newvalue;
removeAttribute(name) --Equal to attribute.removeNamedItem(name).
4. Access the specified node
(1)getElementsByTagName()
Core (XML) DOM defines the getElementsByTagName() method to return a tagName() A NodeList of elements whose tag name) attribute is equal to a specified value. In an Element object, the tagName attribute is always equal to the name immediately following the less than sign -- for example, the tagName for
var oImgs = document.getElementsByTagName("img");
After storing all graphics in oImgs, just use square brackets or Item() method (getElementsByTagName() returns a NodeList the same as childNodes), you can access these nodes one by one like accessing child nodes:
alert(oImgs[0].tagName); //outputs "IMG"
If you only want to get all the images in the first paragraph of a page, you can do it by calling getElementsByTagName() on the first paragraph element, like this:
var oPs = document.getElementByTagName("p") ;
var oImgsInp = oPs[0].getElementByTagName("img");
You can use an asterisk method to get all elements in the document:
var oAllElements = document.getElementsByTagName("*" );
When the parameter is an asterisk, IE6.0 does not return all elements. Document.all must be used instead.
(2)getElementsByName()
HTML DOM defines getElementsByName(), which is used to obtain all elements whose name attribute is equal to the specified value.
(3)getElementById()
这是HTML DOM定义的第二种方法,它将返回id特性等于指定值的元素。在HTML中,id特性是唯一的--这意味着没有两个元素可以共享同一个id。毫无疑问这是从文档树中获取单个指定元素最快的方法。
注:如果给定的ID匹配某个元素的name特性,IE6.0还会返回这个元素。这是一个bug,也是必须非常小心的一个问题。
5.创建新节点
最常用到的几个方法是
createDocumentFragment()--创建文档碎片节点
createElement(tagname)--创建标签名为tagname的元素
createTextNode(text)--创建包含文本text的文本节点
createElement()、createTextNode()、appendChild()
<html> <head> <title>createElement() Example</title> <script type="text/javascript"> function createMessage() { var oP = document.createElement("p"); var oText = document.createTextNode("Hello World!"); oP.appendChild(oText); document.body.appendChild(oP); } </script> </head> <body onload="createMessage()"> </body> </html>
removeChild()、replaceChild()、insertBefore()
删除节点
<html> <head> <title>removeChild() Example</title> <script type="text/javascript"> function removeMessage() { var oP = document.body.getElementsByTagName("p")[0]; oP.parentNode.removeChild(oP); } </script> </head> <body onload="removeMessage()"> <p>Hello World!</p> </body> </html>
替换
<html> <head> <title>replaceChild() Example</title> <script type="text/javascript"> function replaceMessage() { var oNewP = document.createElement("p"); var oText = document.createTextNode("Hello Universe!"); oNewP.appendChild(oText); var oOldP = document.body.getElementsByTagName("p")[0]; oOldP.parentNode.replaceChild(oNewP, oOldP); } </script> </head> <body onload="replaceMessage()"> <p>Hello World!</p> </body> </html>
新消息添加到旧消息之前
<html> <head> <title>insertBefore() Example</title> <script type="text/javascript"> function insertMessage() { var oNewP = document.createElement("p"); var oText = document.createTextNode("Hello Universe!"); oNewP.appendChild(oText); var oOldP = document.getElementsByTagName("p")[0]; document.body.insertBefore(oNewP, oOldP); } </script> </head> <body onload="insertMessage()"> <p>Hello World!</p> </body> </html>
createDocumentFragment()
一旦把节点添加到document.body(或者它的后代节点)中,页面就会更新并反映出这个变化。对于少量的更新,这是很好的,然而,当要向document添加大量数据时,如果逐个添加这些变动,这个过程有可能会十分缓慢。为解决这个问题,可以创建一个文档碎片,把所有的新节点附加其上,然后把文档碎片的内容一次性添加到document中,假如想创建十个新段落。
<html> <head> <title>insertBefore() Example</title> <script type="text/javascript"> function addMessages() { var arrText = ["first", "second", "third", "fourth", "fifth", "sixth", "seventh", "eighth", "ninth", "tenth"]; var oFragment = document.createDocumentFragment(); for (var i=0; i < arrText.length; i++) { var oP = document.createElement("p"); var oText = document.createTextNode(arrText[i]); oP.appendChild(oText); oFragment.appendChild(oP); } document.body.appendChild(oFragment); } </script> </head> <body onload="addMessages()"> </body> </html>
6.让特性像属性一样
大部分情况下,HTML DOM元素中包含的所有特性都是可作为属性。
假设有如下图像元素:
如果要使用核心的DOM来获取和设置src和border特性,那么要用getAttribute()和setAttribute()方法:
alert(oImg.getAttribute("src"));
alert(oImg.getAttribute("border"));
oImg.setAttribute("src","mypicture2.jpg");
oImg.setAttribute("border",1);
然而,使用HTML DOM,可以使用同样名称的属性来获取和设置这些值:
alert(oImg.src);
alert(oImg.border);
oImg.src="mypicture2.jpg";
oImg.border ="1";
唯一的特性名和属性名不一样的特例是class属性,它是用来指定应用于某个元素的一个CSS类,因为class在ECMAScript中是一个保留字,在javascript中,它不能被作为变量名、属性名或都函数名。于是,相应的属性名就变成了className;
注:IE在setAttribute()上有很大的问题,最好尽可能使用属性。
7.table方法
为了协助建立表格,HTML DOM给
给
特性/方法 | 说明 |
caption | 指向 |
tBodies | |
tFoot | 指向 |
tHead | 指向元素(如果存在) |
rows | 表格中所有行的集合 |
createTHead() | 创建元素并将其放入表格 |
createTFood() | 创建 |
createCpation() | 创建 |
deleteTHead() | 删除元素 |
deleteTFood() | 删除 |
deleteCaption() | 删除 |
deleteRow(position) | 删除指定位置上的行 |
insertRow(position) | 在rows集合中的指定位置上插入一个新行 |
特性/方法 | 说明 |
rows | |
deleteRow(position) | 删除指定位置上的行 |
insertRow(position) | 在rows集合中的指定位置上插入一个新行 |
特性/方法 | 说明 |
cells | |
deleteCell(postion) | 删除给定位置上的单元格 |
insertCell(postion) | 在cells集合的给点位置上插入一个新的单元格 |
8. Traverse the DOM
NodeIterator, TreeWalker
DOM Level2 functions, these functions are only available in Mozilla and Konqueror/Safari, and will not be introduced here.

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
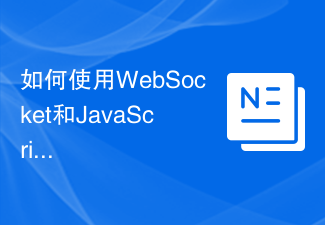
How to use WebSocket and JavaScript to implement an online speech recognition system Introduction: With the continuous development of technology, speech recognition technology has become an important part of the field of artificial intelligence. The online speech recognition system based on WebSocket and JavaScript has the characteristics of low latency, real-time and cross-platform, and has become a widely used solution. This article will introduce how to use WebSocket and JavaScript to implement an online speech recognition system.
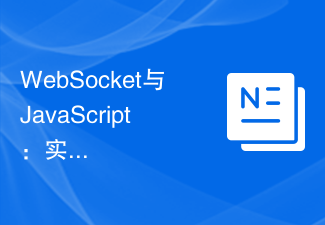
WebSocket and JavaScript: Key technologies for realizing real-time monitoring systems Introduction: With the rapid development of Internet technology, real-time monitoring systems have been widely used in various fields. One of the key technologies to achieve real-time monitoring is the combination of WebSocket and JavaScript. This article will introduce the application of WebSocket and JavaScript in real-time monitoring systems, give code examples, and explain their implementation principles in detail. 1. WebSocket technology
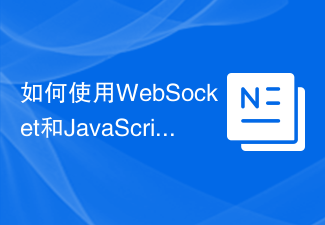
How to use WebSocket and JavaScript to implement an online reservation system. In today's digital era, more and more businesses and services need to provide online reservation functions. It is crucial to implement an efficient and real-time online reservation system. This article will introduce how to use WebSocket and JavaScript to implement an online reservation system, and provide specific code examples. 1. What is WebSocket? WebSocket is a full-duplex method on a single TCP connection.
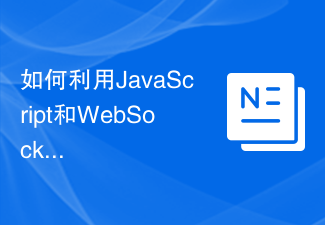
Introduction to how to use JavaScript and WebSocket to implement a real-time online ordering system: With the popularity of the Internet and the advancement of technology, more and more restaurants have begun to provide online ordering services. In order to implement a real-time online ordering system, we can use JavaScript and WebSocket technology. WebSocket is a full-duplex communication protocol based on the TCP protocol, which can realize real-time two-way communication between the client and the server. In the real-time online ordering system, when the user selects dishes and places an order
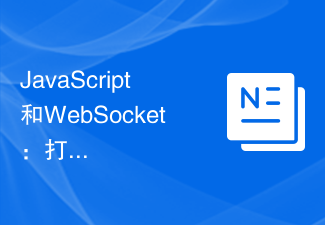
JavaScript and WebSocket: Building an efficient real-time weather forecast system Introduction: Today, the accuracy of weather forecasts is of great significance to daily life and decision-making. As technology develops, we can provide more accurate and reliable weather forecasts by obtaining weather data in real time. In this article, we will learn how to use JavaScript and WebSocket technology to build an efficient real-time weather forecast system. This article will demonstrate the implementation process through specific code examples. We
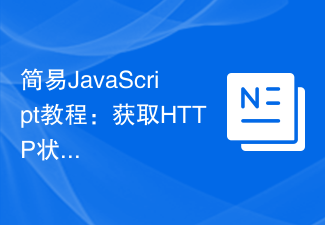
JavaScript tutorial: How to get HTTP status code, specific code examples are required. Preface: In web development, data interaction with the server is often involved. When communicating with the server, we often need to obtain the returned HTTP status code to determine whether the operation is successful, and perform corresponding processing based on different status codes. This article will teach you how to use JavaScript to obtain HTTP status codes and provide some practical code examples. Using XMLHttpRequest

Usage: In JavaScript, the insertBefore() method is used to insert a new node in the DOM tree. This method requires two parameters: the new node to be inserted and the reference node (that is, the node where the new node will be inserted).
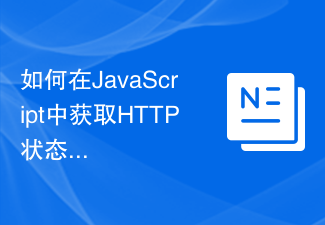
Introduction to the method of obtaining HTTP status code in JavaScript: In front-end development, we often need to deal with the interaction with the back-end interface, and HTTP status code is a very important part of it. Understanding and obtaining HTTP status codes helps us better handle the data returned by the interface. This article will introduce how to use JavaScript to obtain HTTP status codes and provide specific code examples. 1. What is HTTP status code? HTTP status code means that when the browser initiates a request to the server, the service
