Track Image Loading effect code analysis_javascript skills
Purpose
During the image loading process, provide a callback function that defines when the image is loaded successfully or fails/times out, and ensures execution.
Motivation
Native JavaScript already provides onload and onerror registration events for the Image object. However, due to the influence of browser cache and other factors, the onload event often fails to trigger stably when users use the back button or refresh the page. In the photo album system I developed, I hope that pictures can be displayed according to a custom size to avoid page deformation. For example, the maximum width must not exceed 500px, and pictures with a width smaller than 500px will be displayed at the original size. CSS2 provides the max-width attribute to help us achieve this goal. But unfortunately, IE6, which has suffered a thousand dollars, does not support it.
One way for IE6 to compensate is to register the img.onload event and automatically resize the image after it is loaded. The following code is taken from the processing of displayed images in version 4.1 of the famous Discuz! forum system. onload="if(this.width>screen.width *0.7) {this.resized=true; this.width=screen.width*0.7;
this.alt='Click here to open new windowCTRL Mouse wheel to zoom in/out';}"
onmouseover= "if(this.width>screen.width*0.7) {this.resized=true; this.width=screen.width*0.7; this.style.cursor='hand'; this.alt='Click here to open new windownCTRL Mouse wheel to zoom in/out';}"
onclick="if(!this.resized) {return true;} else {window.open('http://img8.imagepile.net/img8/47104p155 .jpg');}"
onmousewheel="return imgzoom(this);">
As mentioned above, the browser does not guarantee that the event processing function will be executed. Therefore, a more stable way is needed to track the image loading process and execute the set callback function.
Implementation
image.complete attribute indicates the image loading status. If its value is true, it means the loading is successful. If the image does not exist or the loading times out, the value is false. By using the setInterval() function to regularly check this status, you can track the image loading status. The code snippet is as follows:
ImageLoader = Class.create();
ImageLoader.prototype = {
initialize : function(options) {
this.options = Object .extend({
timeout: 60, //60s
onInit: Prototype.emptyFunction,
onLoad: Prototype.emptyFunction,
onError: Prototype.emptyFunction
}, options || {} );
this.images = [];
this.pe = new PeriodicalExecuter(this._load.bind(this), 0.02);
},
........
}
Use Prototype's PeriodicalExecuter class to create a timer, check the loading status of the image every 20 milliseconds, and execute the callback function defined in the options parameter based on the status.
Use
var loader = new ImageLoader({
timeout: 30,
onInit: function(img) {
img.style. width = '100px';
},
onLoad: function(img) {
img.style.width = '';
if (img.width > 500)
img.style .width = '500px';
},
onError: function(img) {
img.src = 'error.jpg'; //hint image
}
});loader .loadImage(document.getElementsByTagName('img'));
The above code defines that the image is initially displayed at 100px. After successful loading, if the actual width of the image exceeds 500px, it will be forced to be defined as 500px, otherwise the original size will be displayed. . If the image does not exist or the loading times out (30 seconds is the timeout), an error image is displayed.
Similarly, you can use the callback function of ImageLoader to customize the effect according to your needs. For example, loading is displayed by default, and then the original image is displayed after loading is completed; the image is first displayed in grayscale, and then the brightness is restored after loading is completed, etc.For example:
//need scriptaculous effects.js
var loader = new ImageLoader({
onInit: function(img) {
Element.setOpacity(img, 0.5); //Default level 5 transparency
},
onLoad: function(img) {
Effect.Appear(img); //Restore the original image display
}
});
The attached example contains complete code and a test using pconline pictures as an example. Note that the latest Prototype 1.5.0_rc1 is used in the example.
nbsp;HTML PUBLIC "-//W3C//DTD HTML 4.0 //EN">



Loading failure test

<script></script> <script></script><script> <BR>ImageLoader = Class.create(); <BR>ImageLoader.prototype = { <br><br> initialize : function(options) { <BR> this.options = Object.extend({ <BR> timeout: 60, //60s <BR> onInit: Prototype.emptyFunction, <BR> onLoad: Prototype.emptyFunction, <BR> onError: Prototype.emptyFunction <BR> }, options || {}); <BR> this.images = []; <BR> this.pe = new PeriodicalExecuter(this._load.bind(this), 0.02); <BR> }, <br><br> loadImage : function() { <BR> var self = this; <BR> $A(arguments).each(function(img) { <BR> if (typeof(img) == 'object') <BR> $A(img).each(self._addImage.bind(self)); <BR> else <BR> self._addImage(img); <BR> }); <BR> }, <br><br> _addImage : function(img) { <BR> img = $(img); <BR> img.onerror = this._onerror.bind(this, img); <BR> this.options.onInit.call(this, img); <BR> if (this.options.timeout > 0) { <BR> setTimeout(this._ontimeout.bind(this, img), this.options.timeout*1000); <BR> } <BR> this.images.push(img); <BR> if (!this.pe.timer) <BR> this.pe.registerCallback(); <BR> }, <br><br> <BR> _load: function() { <BR> this.images = this.images.select(this._onload.bind(this)); <BR> if (this.images.length == 0) { <BR> this.pe.stop(); <BR> } <BR> }, <br><br> _checkComplete : function(img) { <BR> if (img._error) { <BR> return true; <BR> } else { <BR> return img.complete; <BR> } <BR> }, <br><br> _onload : function(img) { <BR> if (this._checkComplete(img)) { <BR> this.options.onLoad.call(this, img); <BR> img.onerror = null; <BR> if (img._error) <BR> try {delete img._error}catch(e){} <BR> return false; <BR> } <BR> return true; <BR> }, <br><br> _onerror : function(img) { <BR> img._error = true; <BR> img.onerror = null; <BR> this.options.onError.call(this, img); <BR> }, <br><br> _ontimeout : function(img) { <BR> if (!this._checkComplete(img)) { <BR> this._onerror(img); <BR> } <BR> } <br><br>} <br><br>var loader = new ImageLoader({ <BR> timeout: 30, <BR> onInit: function(img) { <BR> img.style.width = '100px'; <BR> }, <BR> onLoad: function(img) { <BR> img.style.width = ''; <BR> if (img.width > 500) { <BR> img.style.width = '500px'; <BR> } <BR> }, <BR> onError: function(img) { <BR> img.src = 'http://img.pconline.com.cn/nopic.gif'; <BR> } <BR>}); <br><br>loader.loadImage(document.getElementsByTagName('img')); <br><br>/* <BR>var loader = new ImageLoader({ <BR> timeout: 30, <BR> onInit: function(img) { <BR> Element.setOpacity(img, 0.5); <BR> }, <BR> onLoad: function(img) { <BR> Effect.Appear(img); <BR> }, <BR> onError: function(img) { <BR> img.src = 'http://img.pconline.com.cn/nopic.gif'; <BR> } <BR>}); <BR>*/ <br><br>/* <BR>$A(document.getElementsByTagName('img')).each( <BR>function(img) { <BR> img.onload = function() { <BR> img.style.width = '300px'; <BR> } <BR>} <BR>); <BR>*/ <br><br></script>

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
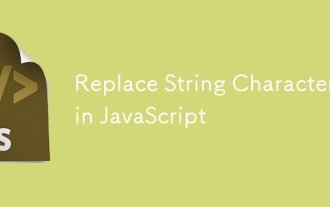
Detailed explanation of JavaScript string replacement method and FAQ This article will explore two ways to replace string characters in JavaScript: internal JavaScript code and internal HTML for web pages. Replace string inside JavaScript code The most direct way is to use the replace() method: str = str.replace("find","replace"); This method replaces only the first match. To replace all matches, use a regular expression and add the global flag g: str = str.replace(/fi
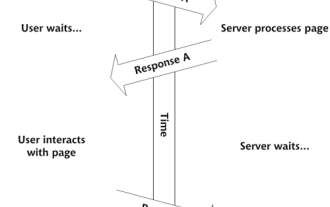
So here you are, ready to learn all about this thing called AJAX. But, what exactly is it? The term AJAX refers to a loose grouping of technologies that are used to create dynamic, interactive web content. The term AJAX, originally coined by Jesse J
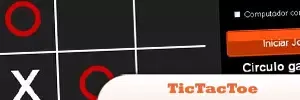
10 fun jQuery game plugins to make your website more attractive and enhance user stickiness! While Flash is still the best software for developing casual web games, jQuery can also create surprising effects, and while not comparable to pure action Flash games, in some cases you can also have unexpected fun in your browser. jQuery tic toe game The "Hello world" of game programming now has a jQuery version. Source code jQuery Crazy Word Composition Game This is a fill-in-the-blank game, and it can produce some weird results due to not knowing the context of the word. Source code jQuery mine sweeping game
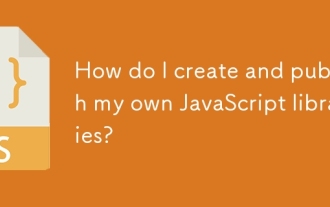
Article discusses creating, publishing, and maintaining JavaScript libraries, focusing on planning, development, testing, documentation, and promotion strategies.
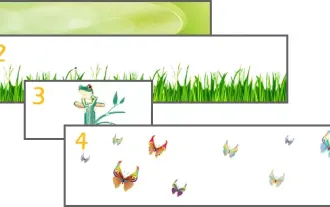
This tutorial demonstrates how to create a captivating parallax background effect using jQuery. We'll build a header banner with layered images that create a stunning visual depth. The updated plugin works with jQuery 1.6.4 and later. Download the
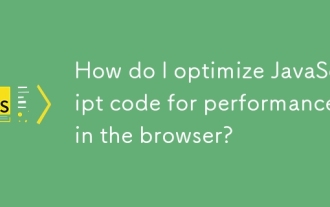
The article discusses strategies for optimizing JavaScript performance in browsers, focusing on reducing execution time and minimizing impact on page load speed.
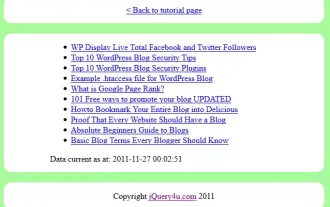
This article demonstrates how to automatically refresh a div's content every 5 seconds using jQuery and AJAX. The example fetches and displays the latest blog posts from an RSS feed, along with the last refresh timestamp. A loading image is optiona
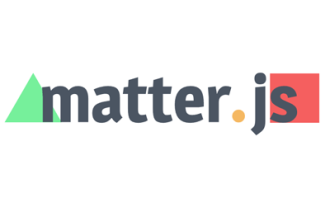
Matter.js is a 2D rigid body physics engine written in JavaScript. This library can help you easily simulate 2D physics in your browser. It provides many features, such as the ability to create rigid bodies and assign physical properties such as mass, area, or density. You can also simulate different types of collisions and forces, such as gravity friction. Matter.js supports all mainstream browsers. Additionally, it is suitable for mobile devices as it detects touches and is responsive. All of these features make it worth your time to learn how to use the engine, as this makes it easy to create a physics-based 2D game or simulation. In this tutorial, I will cover the basics of this library, including its installation and usage, and provide a
