


Comprehensive and detailed jQuery common development skills manual_jquery
This article has compiled a very detailed article on common jQuery development techniques for your reference. The specific content is as follows
1. References about page elements
Referencing elements through jquery's $() includes methods such as id, class, element name, hierarchical relationship of elements, dom or xpath conditions, etc., and the returned object is a jquery object (collection object), which cannot be directly called dom-defined method.
2. Conversion of jQuery objects and dom objects
Only jquery objects can use the methods defined by jquery. Note that there is a difference between dom objects and jquery objects. When calling methods, you should pay attention to whether you are operating on dom objects or jquery objects.
Ordinary DOM objects can generally be converted into jQuery objects through $().
For example: $(document.getElementById("msg")) is a jquery object, and you can use jquery methods.
Since the jquery object itself is a collection. Therefore, if the jquery object is to be converted into a dom object, one of the items must be retrieved, which can generally be retrieved through an index.
For example: $("#msg")[0], $("div").eq(1)[0], $("div").get()[1], $("td")[5 ] These are dom objects, and you can use methods in dom, but you can no longer use Jquery methods.
The following ways of writing are all correct:
The code is as follows:
$("#msg").html(); $("#msg")[0].innerHTML; $("#msg").eq(0)[0].innerHTML; $("#msg").get(0).innerHTML;
3. How to get an item of jQuery collection
For the obtained element set, you can use the eq or get(n) method or index number to obtain an item (specified by index). Please note that eq returns a jquery object, and get(n) and The index returns the dom element object. For jquery objects, you can only use jquery methods, and for dom objects, you can only use dom methods. For example, you want to get the content of the third
$(“div”).eq(2).html(); //调用jquery对象的方法 $(“div”).get(2).innerHTML; //调用dom的方法属性
4. The same function implements set and get
This is true for many methods in Jquery, mainly including the following:
The code is as follows:
$(“#msg”).html(); //返回id为msg的元素节点的html内容。 $(“#msg”).html(“<b>new content</b>”); //将“<b>new content</b>” 作为html串写入id为msg的元素节点内容中,页面显示粗体的new content $(“#msg”).text(); //返回id为msg的元素节点的文本内容。 $(“#msg”).text(“<b>new content</b>”); //将“<b>new content</b>” 作为普通文本串写入id为msg的元素节点内容中,页面显示<b>new content</b> $(“#msg”).height(); //返回id为msg的元素的高度 $(“#msg”).height(“300″); //将id为msg的元素的高度设为300 $(“#msg”).width(); //返回id为msg的元素的宽度 $(“#msg”).width(“300″); //将id为msg的元素的宽度设为300 $(“input”).val(“); //返回表单输入框的value值 $(“input”).val(“test”); //将表单输入框的value值设为test $(“#msg”).click(); //触发id为msg的元素的单击事件 $(“#msg”).click(fn); //为id为msg的元素单击事件添加函数
Similarly, blur, focus, select, and submit events can have two calling methods
5. Collection processing function
For the collection content returned by jquery, we do not need to loop through it ourselves and process each object separately. jquery has provided us with a very convenient method to process the collection.
Includes two forms:
The code is as follows:
$(“p”).each(function(i){this.style.color=['#f00','#0f0','#00f'][ i ]}) //为索引分别为0,1,2的p元素分别设定不同的字体颜色。 $(“tr”).each(function(i){this.style.backgroundColor=['#ccc','#fff'][i%2]}) //实现表格的隔行换色效果 www.222gs.com $(“p”).click(function(){alert($(this).html())}) //为每个p元素增加了click事件,单击某个p元素则弹出其内容
6. Expand the functions we need
The code is as follows:
$.extend({ min: function(a, b){return a < b?a:b; }, max: function(a, b){return a > b?a:b; } }); //为jquery扩展了min,max两个方法
Use extended methods (called via "$.methodname"):
alert(“a=10,b=20,max=”+$.max(10,20)+”,min=”+$.min(10,20));
7. Support method concatenation
The so-called continuous writing means that you can continuously call various methods on a jquery object.
For example:
The code is as follows:
$(“p”).click(function(){alert($(this).html())}) .mouseover(function(){alert(‘mouse over event')}) .each(function(i){this.style.color=['#f00','#0f0','#00f'][ i ]});
8. Style of operating elements
Mainly include the following methods:
The code is as follows:
$(“#msg”).css(“background”); //返回元素的背景颜色 $(“#msg”).css(“background”,”#ccc”) //设定元素背景为灰色 $(“#msg”).height(300); $(“#msg”).width(“200″); //设定宽高 $(“#msg”).css({ color: “red”, background: “blue” });//以名值对的形式设定样式 $(“#msg”).addClass(“select”); //为元素增加名称为select的class $(“#msg”).removeClass(“select”); //删除元素名称为select的class $(“#msg”).toggleClass(“select”); //如果存在(不存在)就删除(添加)名称为select的class
9. Complete event processing function
Jquery has provided us with various event handling methods. We do not need to write events directly on html elements, but can directly add events to objects obtained through jquery.
Such as:
The code is as follows:
$(“#msg”).click(function(){alert(“good”)}) //为元素添加了单击事件 $(“p”).click(function(i){this.style.color=['#f00','#0f0','#00f'][ i ]}) //为三个不同的p元素单击事件分别设定不同的处理
Several custom events in jQuery:
(1)hover(fn1,fn2): A method that simulates hover events (the mouse moves over an object and out of the object). When the mouse moves over a matching element, the first specified function will be triggered. When the mouse moves out of this element, the specified second function will be triggered.
The code is as follows:
//当鼠标放在表格的某行上时将class置为over,离开时置为out。 $(“tr”).hover(function(){ $(this).addClass(“over”); }, function(){ $(this).addClass(“out”); });
(2) ready(fn): Bind a function to be executed when the DOM is loaded and ready for query and manipulation.
The code is as follows:
$(document).ready(function(){alert(“Load Success”)}) //页面加载完毕提示“Load Success”,相当于onload事件,与$(fn)等价
(3) toggle(evenFn,oddFn): Switch the function to be called every time you click. If a matching element is clicked, the first function specified is triggered, and when the same element is clicked again, the second function specified is triggered. Each subsequent click repeats the call to these two functions in turn.
The code is as follows:
//每次点击时轮换添加和删除名为selected的class。 $(“p”).toggle(function(){ $(this).addClass(“selected”); },function(){ $(this).removeClass(“selected”); });
(4) trigger(eventtype): Trigger a certain type of event on each matching element.
For example:
$("p").trigger("click"); //Trigger the click event of all p elements
(5) bind(eventtype,fn), unbind(eventtype): binding and unbinding of events
Removes (adds) the bound event from each matching element.
For example:
The code is as follows:
$(“p”).bind(“click”, function(){alert($(this).text());}); //为每个p元素添加单击事件 $(“p”).unbind(); //删除所有p元素上的所有事件 $(“p”).unbind(“click”) //删除所有p元素上的单击事件
10、几个实用特效功能
其中toggle()和slidetoggle()方法提供了状态切换功能。
如toggle()方法包括了hide()和show()方法。
slideToggle()方法包括了slideDown()和slideUp方法。
11、几个有用的jQuery方法
$.browser.浏览器类型:检测浏览器类型。有效参数:safari, opera, msie, mozilla。如检测是否ie:$.browser.isie,是ie浏览器则返回true。
$.each(obj, fn):通用的迭代函数。可用于近似地迭代对象和数组(代替循环)。
如
代码如下:
$.each( [0,1,2], function(i, n){ alert( “Item #” + i + “: ” + n ); });
等价于:
代码如下:
var tempArr=[0,1,2]; for(var i=0;i<tempArr.length;i++){ alert(“Item #”+i+”: “+tempArr[ i ]); }
也可以处理json数据,如
$.each( { name: “John”, lang: “JS” }, function(i, n){ alert( “Name: ” + i + “, Value: ” + n ); });
结果为:
Name:name, Value:John
Name:lang, Value:JS
$.extend(target,prop1,propN):用一个或多个其他对象来扩展一个对象,返回这个被扩展的对象。这是jquery实现的继承方式。
如:
$.extend(settings, options); //合并settings和options,并将合并结果返回settings中,相当于options继承setting并将继承结果保存在setting中。 var settings = $.extend({}, defaults, options); //合并defaults和options,并将合并结果返回到setting中而不覆盖default内容。
可以有多个参数(合并多项并返回)
$.map(array, fn):数组映射。把一个数组中的项目(处理转换后)保存到到另一个新数组中,并返回生成的新数组。
如:
var tempArr=$.map( [0,1,2], function(i){ return i + 4; }); tempArr内容为:[4,5,6] var tempArr=$.map( [0,1,2], function(i){ return i > 0 ? i + 1 : null; }); tempArr内容为:[2,3] $.merge(arr1,arr2):合并两个数组并删除其中重复的项目。
如:$.merge( [0,1,2], [2,3,4] ) //返回[0,1,2,3,4]
$.trim(str):删除字符串两端的空白字符。
如:$.trim(” hello, how are you? “); //返回”hello,how are you? ”
12、解决自定义方法或其他类库与jQuery的冲突
很多时候我们自己定义了$(id)方法来获取一个元素,或者其他的一些js类库如prototype也都定义了$方法,如果同时把这些内容放在一起就会引起变量方法定义冲突,Jquery对此专门提供了方法用于解决此问题。
使 用jquery中的jQuery.noConflict();方法即可把变量$的控制权让渡给第一个实现它的那个库或之前自定义的$方法。之后应用 Jquery的时候只要将所有的$换成jQuery即可,如原来引用对象方法$(“#msg”)改为jQuery(“#msg”)。
如:
代码如下:
jQuery.noConflict(); // 开始使用jQuery jQuery(“div p”).hide(); // 使用其他库的 $() $(“content”).style.display = ‘none';
jquery开发过程中经验丰富可以提高开发技巧,所以大家一定要注意平时经验的积累,希望本文所述对大家学习有所帮助。

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


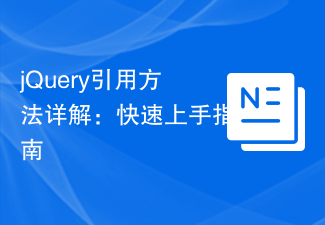
Detailed explanation of jQuery reference method: Quick start guide jQuery is a popular JavaScript library that is widely used in website development. It simplifies JavaScript programming and provides developers with rich functions and features. This article will introduce jQuery's reference method in detail and provide specific code examples to help readers get started quickly. Introducing jQuery First, we need to introduce the jQuery library into the HTML file. It can be introduced through a CDN link or downloaded
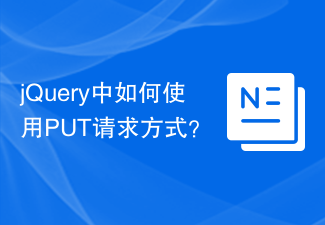
How to use PUT request method in jQuery? In jQuery, the method of sending a PUT request is similar to sending other types of requests, but you need to pay attention to some details and parameter settings. PUT requests are typically used to update resources, such as updating data in a database or updating files on the server. The following is a specific code example using the PUT request method in jQuery. First, make sure you include the jQuery library file, then you can send a PUT request via: $.ajax({u
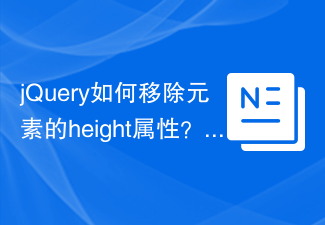
How to remove the height attribute of an element with jQuery? In front-end development, we often encounter the need to manipulate the height attributes of elements. Sometimes, we may need to dynamically change the height of an element, and sometimes we need to remove the height attribute of an element. This article will introduce how to use jQuery to remove the height attribute of an element and provide specific code examples. Before using jQuery to operate the height attribute, we first need to understand the height attribute in CSS. The height attribute is used to set the height of an element
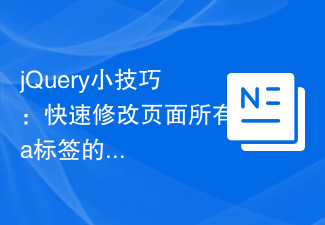
Title: jQuery Tips: Quickly modify the text of all a tags on the page In web development, we often need to modify and operate elements on the page. When using jQuery, sometimes you need to modify the text content of all a tags in the page at once, which can save time and energy. The following will introduce how to use jQuery to quickly modify the text of all a tags on the page, and give specific code examples. First, we need to introduce the jQuery library file and ensure that the following code is introduced into the page: <
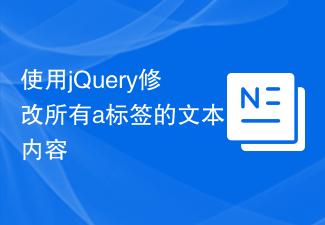
Title: Use jQuery to modify the text content of all a tags. jQuery is a popular JavaScript library that is widely used to handle DOM operations. In web development, we often encounter the need to modify the text content of the link tag (a tag) on the page. This article will explain how to use jQuery to achieve this goal, and provide specific code examples. First, we need to introduce the jQuery library into the page. Add the following code in the HTML file:
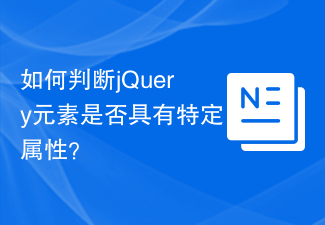
How to tell if a jQuery element has a specific attribute? When using jQuery to operate DOM elements, you often encounter situations where you need to determine whether an element has a specific attribute. In this case, we can easily implement this function with the help of the methods provided by jQuery. The following will introduce two commonly used methods to determine whether a jQuery element has specific attributes, and attach specific code examples. Method 1: Use the attr() method and typeof operator // to determine whether the element has a specific attribute
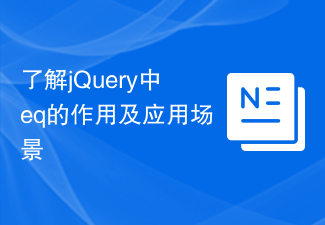
jQuery is a popular JavaScript library that is widely used to handle DOM manipulation and event handling in web pages. In jQuery, the eq() method is used to select elements at a specified index position. The specific usage and application scenarios are as follows. In jQuery, the eq() method selects the element at a specified index position. Index positions start counting from 0, i.e. the index of the first element is 0, the index of the second element is 1, and so on. The syntax of the eq() method is as follows: $("s
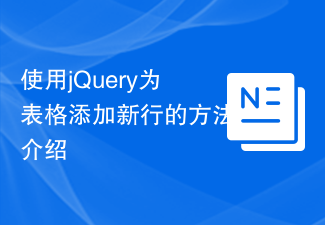
jQuery is a popular JavaScript library widely used in web development. During web development, it is often necessary to dynamically add new rows to tables through JavaScript. This article will introduce how to use jQuery to add new rows to a table, and provide specific code examples. First, we need to introduce the jQuery library into the HTML page. The jQuery library can be introduced in the tag through the following code:
