The second day of learning YUI.Ext_YUI.Ext related
I encountered some grammatical problems with JS, but I didn’t understand them thoroughly, so I had to make up for the basics!
Anonymous function An anonymous function
An anonymous function without a name is called an “anonymous function”, like this x y}
Without a name, of course it cannot be called directly or called; at most it can only be assigned a value or processed with a closure (what a closure is will be discussed below), such as:
var sum =function(x,y){return x y};
alert(sum(1,2));
At this time, it is equivalent to the traditional writing function sum(x,y ){return x y}. This way of writing makes people feel more OOP, because the sum variable contains the function...this function body;
The function can also be called in a closure way:
(functioin(x,y){return x y})(1,2) //Return value 3
The code is very concise. Note the use of parentheses, in the form (exp)(). This usage can be called closure.
The following brackets are parameters. Put these parameters into fn and calculate them immediately to get a value of 3. This is actually an expression operation. Unexpectedly, the fn function body can also be put in to participate in calculations ^_^ (Using function as an expression)! (Basic skill: Expression, which means that after calculation, a value will always be returned, no matter how long the expression is)
fn can also be passed in the form of parameters (passing function as argument to other functions)
var main_fn = function(fn,x,y){return fn(x,y)}
var sum = function (x,y){
return x y;
}
alert(main_fn(sum,1,2)) // result:3
Summarize (by an IBM Engineer's article, refer to IBM website, it is best to remember it carefully )
Functions need not have names all the time.
Functions can be assigned to variables like other values.
A function expression can be written and enclosed in parenetheses for application later.
Functions can be passed as arguments to oher funcitons.
Let’s talk about closures again. The function of closures is to form a domain. Let’s give a very idiotic example 1 (2 3) , the parenthesis part takes precedence, or to put it another way, what is inside the parentheses is classified into a range. I don’t care what you do in this range, it’s all what you do inside, and has nothing to do with the outside world of the parentheses (it seems to be nonsense, - that’s what I think) , that’s how it’s written @#@), and the same is true for program understanding. JS has a function scope, so when there is a problem using this to point to an object, you can consider using a closure. Specific examples are at: http://www.svendtofte.com/code/practical_functional_js/

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


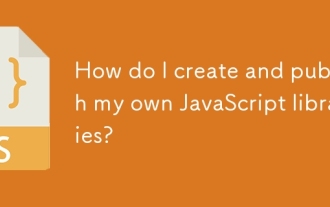
Article discusses creating, publishing, and maintaining JavaScript libraries, focusing on planning, development, testing, documentation, and promotion strategies.
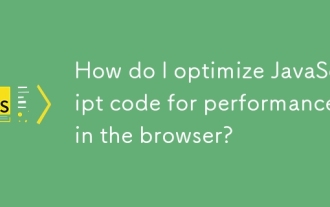
The article discusses strategies for optimizing JavaScript performance in browsers, focusing on reducing execution time and minimizing impact on page load speed.
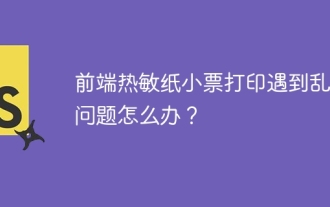
Frequently Asked Questions and Solutions for Front-end Thermal Paper Ticket Printing In Front-end Development, Ticket Printing is a common requirement. However, many developers are implementing...
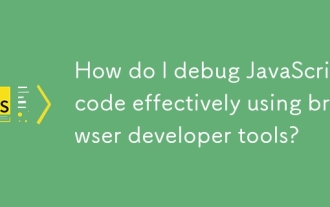
The article discusses effective JavaScript debugging using browser developer tools, focusing on setting breakpoints, using the console, and analyzing performance.
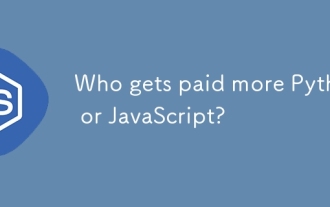
There is no absolute salary for Python and JavaScript developers, depending on skills and industry needs. 1. Python may be paid more in data science and machine learning. 2. JavaScript has great demand in front-end and full-stack development, and its salary is also considerable. 3. Influencing factors include experience, geographical location, company size and specific skills.
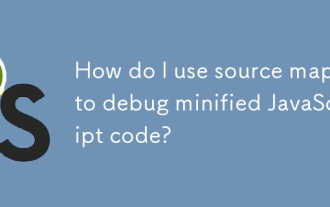
The article explains how to use source maps to debug minified JavaScript by mapping it back to the original code. It discusses enabling source maps, setting breakpoints, and using tools like Chrome DevTools and Webpack.

This tutorial will explain how to create pie, ring, and bubble charts using Chart.js. Previously, we have learned four chart types of Chart.js: line chart and bar chart (tutorial 2), as well as radar chart and polar region chart (tutorial 3). Create pie and ring charts Pie charts and ring charts are ideal for showing the proportions of a whole that is divided into different parts. For example, a pie chart can be used to show the percentage of male lions, female lions and young lions in a safari, or the percentage of votes that different candidates receive in the election. Pie charts are only suitable for comparing single parameters or datasets. It should be noted that the pie chart cannot draw entities with zero value because the angle of the fan in the pie chart depends on the numerical size of the data point. This means any entity with zero proportion
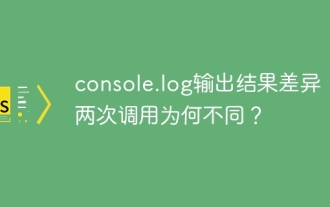
In-depth discussion of the root causes of the difference in console.log output. This article will analyze the differences in the output results of console.log function in a piece of code and explain the reasons behind it. �...
