Detailed explanation of JavaScript triggers_javascript skills
The front-end of a website consists of three layers. The structural layer built by XHTML, which includes structured and semantic tags, as well as the content of the website. You can add a presentation layer (CSS) and a behavior layer (JavaScript) on top of this layer, which make the website look more beautiful and more user-friendly. Strict separation should be maintained between these three layers. For example, it should be possible to rewrite the entire presentation layer without touching the structural and behavioral layers.
In addition to this strict separation, both the presentation layer and the behavioral layer need instructions from the structural layer. They have to know where to apply styles and when to initialize behavior - in other words: they need triggers.
Everyone knows about CSS triggers. The class and id attributes give you complete control over the performance of your website. However, you can work without these triggers by using inline style attributes (Translator's Note: Referring to the style="..." attribute written in the XHTML tag), but this usage is It should be strongly opposed. When you want to redefine the performance of your website, you will be forced to change the XHTML structure layer as well. Their presence destroys the separation between performance and structure.
JavaScript Triggers
Behavior layers should work the same way. By moving away from using inline event handlers (such as onmouseover="switchImages('fearful',6,false)"), we can separate behavior from structure. Like CSS, we should use triggers to tell the script where to deploy the behavior.
The simplest JavaScript trigger is the id attribute.
Advanced Triggers
Unfortunately there are cases where you cannot use ids as triggers:
An id can only appear once in a document, sometimes you may want the same behavior Added to several (or a group of) elements.
Sometimes the script needs more information than just saying "deploy here" (Translator's Note: such as passing some parameters).
We use form scripts to illustrate the above two questions. It can be useful to add form validation triggers to XHTML, such as specifying "this input field (Translator's Note: text input box, password input box, etc.) is required."In order to implement such a trigger, we are likely to get the following script:
function validateForm(){ var x = document.forms[0].elements; for (var i=0;i
if (x[i].className == 'required' && !x[i].value) //Prompt the user to enter this field
However, strictly speaking , the class attribute is used to define CSS triggers. It is not impossible to define CSS and JavaScript triggers together, but doing so is likely to make the code confusing:
if ( x[i].className.indexOf('required') != -1 && !x[i].value)
In my opinion, the class attribute should only be used in CSS. Class is the main part of the XHTML presentation layer. Triggers will complicate the problem if they are allowed to carry information from the behavior layer. Using class attributes to trigger both layers at the same time conflicts with the separation of behavior and performance, but it is up to you to decide how to do it. Depends.
Messaging Triggers
Additionally, triggers can be more complex than just a command that states "deploy (behavior) here". Sometimes you might want to. Adding a variable to the trigger makes the behavior layer more versatile and can respond to the individual needs of each XHTML element instead of stupidly executing a standard script.
Take a form as an example. , this form includes some text input boxes with an upper limit on string length. The original maxlength attribute no longer works on textarea elements, so we have to write a script to do this. In addition, not all text in this form. The input boxes all have the same maximum string length, so it is necessary to store their respective maximum lengths somewhere.
We hope to have something like this:
. var x = document.getElementsByTagName('textarea');for (var i=0;i
This script requires two key information:
Is there an upper limit on the length of this text input box? This is a very general trigger that tells the script that certain actions should be added here.
What is the upper limit? This is a value that allows the script to correctly check user input.
Here, the class-based approach is no longer appropriate. While it's not technically impossible to do, the code required would become too complex. For example, let's add a trigger to a text input box that has a class called "large" to tell the script that it is required and that the maximum length is 300:
Not only does this mix the presentation layer and the behavior layer, but the script used to read this value will also become weird:
var max = this.className .substring( this.className.indexOf('maxlength') 10);if (this.value.length > max) // Remind the user that something went wrong.
It’s easy to notice that this script only works if we put maxlength=x at the end. If we want this script to handle maxlength=x that is not the last one (this is often the case, for example, if we want to add a value-passing trigger), it will become more complicated.
Problems facing
This is the problem we are facing now. How can we add the perfect JavaScript trigger that allows us to easily pass both a general statement ("Deploy action here") and an element-specific value to the script?
Technically, it is possible to add this information to the class attribute, but the question is is the class designed to do this?
Custom Properties
I turned to another solution. Let's look at the textarea length limit example mentioned earlier. We need two pieces of information:
Is there an upper limit on the length of this text input box?
What is the upper limit?
To express this information in a natural and semantic way requires adding a custom attribute to the textarea:
The maxlength attribute notifies the script to check the user input and pass the attribute's The value passes the maximum length to the script.In the same way, we can change the "required" trigger to a custom attribute. For example: required="true", no matter what value is assigned to it, because it only serves as a notification and does not need to be accompanied by any additional information.
Technically speaking, there is nothing wrong with doing this. W3C DOM's getAttribute() method can read the value of any attribute from any tag. Only Opera versions prior to 7.54 do not allow reading an incorrect attribute (such as src) from a tag (such as
). Fortunately, later versions provide full support for getAttribute().
Here is my solution:
function validateForm(){ var x = document.forms[0].elements; for (var i=0;i max) / / notify user of error}
In my opinion, this solution is easy to implement and consistent with the form of a JavaScript trigger: a "variable name/value" pair provides the name of the trigger and what the script needs value, which also allows you to define behavior for each element individually. Finally, this way of adding triggers to XHTML is fairly simple for beginners.
Custom DTD
But there is another problem here. The page produced with this solution cannot pass the verification. The validator considers required and maxlength to be illegal. Of course, the validator is completely correct to do this. The former attribute does not exist in XHTML, and the latter attribute only belongs to the element.
The solution is to make them legal: define a custom Document Type Definition (DTD), and make a small extension of XHTML to include the attributes we defined. This custom DTD defines the correct location where the new attributes should appear, and then the validator will validate the document according to our custom XHTML flavor. If the DTD says these properties are correct, then they are correct.
If you don’t know how to create a custom DTD, please read this article "Creating a Custom DTD" published by J.David Eisenberg (Translator's Note: Decide whether to translate based on your opinions^_^) , in this article he will tell you all you need to know.
In my opinion, using custom properties to trigger the behavior layer - coupled with a custom DTD that makes these properties legal - can help us separate the behavior layer from the structure layer while maintaining clean code and Efficient script. Furthermore, once these properties and scripts are defined, even the most novice can easily add triggers to XHTML documents.
In my opinion, this solution is easy to implement and consistent with the form of a JavaScript trigger: a "variable name/value" pair provides the name of the trigger and what the script needs value, which also allows you to define behavior for each element individually. Finally, this way of adding triggers to XHTML is fairly simple for beginners.
Custom DTD
But there is another problem here. The page produced with this solution cannot pass the verification. The validator considers required and maxlength to be illegal. Of course, the validator is completely correct to do this. The former attribute does not exist in XHTML, and the latter attribute only belongs to the element.
The solution is to make them legal: define a custom Document Type Definition (DTD), and make a small extension of XHTML to include the attributes we defined. This custom DTD defines the correct location where the new attributes should appear, and then the validator will validate the document according to our custom XHTML flavor. If the DTD says these properties are correct, then they are correct.
If you don’t know how to create a custom DTD, please read this article "Creating a Custom DTD" published by J.David Eisenberg (Translator's Note: Decide whether to translate based on your opinions^_^) , in this article he will tell you all you need to know.
In my opinion, using custom properties to trigger the behavior layer - coupled with a custom DTD that makes these properties legal - can help us separate the behavior layer from the structure layer while maintaining clean code and Efficient script. Furthermore, once these properties and scripts are defined, even the most novice can easily add triggers to XHTML documents.

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
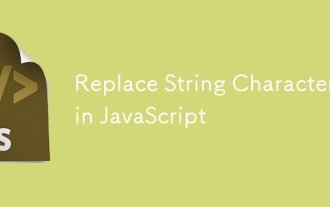
Detailed explanation of JavaScript string replacement method and FAQ This article will explore two ways to replace string characters in JavaScript: internal JavaScript code and internal HTML for web pages. Replace string inside JavaScript code The most direct way is to use the replace() method: str = str.replace("find","replace"); This method replaces only the first match. To replace all matches, use a regular expression and add the global flag g: str = str.replace(/fi
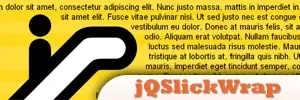
Leverage jQuery for Effortless Web Page Layouts: 8 Essential Plugins jQuery simplifies web page layout significantly. This article highlights eight powerful jQuery plugins that streamline the process, particularly useful for manual website creation
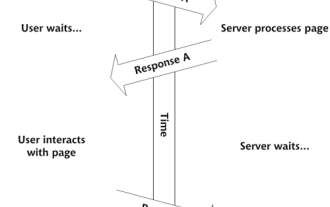
So here you are, ready to learn all about this thing called AJAX. But, what exactly is it? The term AJAX refers to a loose grouping of technologies that are used to create dynamic, interactive web content. The term AJAX, originally coined by Jesse J
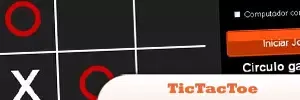
10 fun jQuery game plugins to make your website more attractive and enhance user stickiness! While Flash is still the best software for developing casual web games, jQuery can also create surprising effects, and while not comparable to pure action Flash games, in some cases you can also have unexpected fun in your browser. jQuery tic toe game The "Hello world" of game programming now has a jQuery version. Source code jQuery Crazy Word Composition Game This is a fill-in-the-blank game, and it can produce some weird results due to not knowing the context of the word. Source code jQuery mine sweeping game
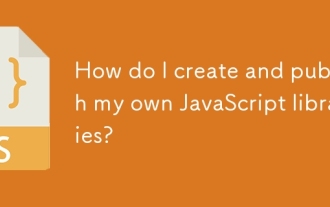
Article discusses creating, publishing, and maintaining JavaScript libraries, focusing on planning, development, testing, documentation, and promotion strategies.
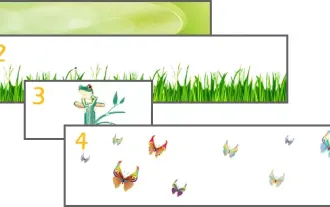
This tutorial demonstrates how to create a captivating parallax background effect using jQuery. We'll build a header banner with layered images that create a stunning visual depth. The updated plugin works with jQuery 1.6.4 and later. Download the
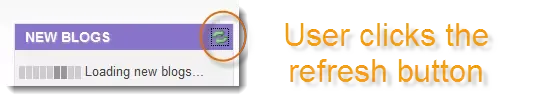
This tutorial demonstrates creating dynamic page boxes loaded via AJAX, enabling instant refresh without full page reloads. It leverages jQuery and JavaScript. Think of it as a custom Facebook-style content box loader. Key Concepts: AJAX and jQuery
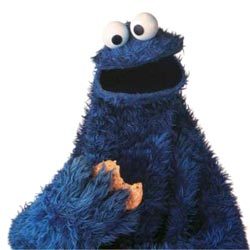
This JavaScript library leverages the window.name property to manage session data without relying on cookies. It offers a robust solution for storing and retrieving session variables across browsers. The library provides three core methods: Session
