


Application skills of objects and arrays in javascript_Basic knowledge
I have been using JavaScript for more than three years, but I still know little about some details, such as objects and arrays, and I have been using some of the most basic operations. This is a bad habit I have in studying - I am lazy. I only understand a lot of things. I have no patience when I see slightly more complicated logic. Just like when you learn ASP, you don't know the relationship between ASP and scripts, and when you learn html, you don't know what DOM is... As a result, you learn slowly and not solidly, and you stop learning when you encounter slightly complicated problems (I'm going too far).
I continued to optimize the script today and encountered problems when trying to merge some arrays, so I read the manual carefully and did some tests.
Javascript 1.2 and later allows the use of [] to create arrays:
var firstArray = [];
var secondArray = ["red", "green", "blue" ];
var thirdArray = [,,,,];
You can add elements after the array is created:
secondArray[4] = 28;
Like this The result is 1 empty element between the 4th element and the 2nd element.
The result is similar to this: ["red", "green", "blue",,28]
It should be noted that the array must be initialized. I tested the definition of the array as an array element:
var arrArray = [[]];
Originally I envisioned implementing a two-dimensional array, but the result is that using arrArray[i][0] is invalid when i>0!
After looking carefully, I realized that arrArray[0] is actually defined as an empty array, while other elements are undefined, which is equivalent to
var arrArray=[];
arrArray[0]=[] ;
So arrArray[1] is not defined, so if you operate it as an array, you will get an error.
Delete an array element: delete
var myColors = ["red", "green", "blue"];
delete myColors[1];
alert("The value of myColors[1] is: " myColors[1]);
The result of delete is myColors[1]=undefined, but myColors.length remains unchanged, and myColors becomes like this : ["red",, "blue"]
Replace or absolutely delete array elements with slice():
var myArray = [1, 2, 3, 4, 5 ];
myArray.slice(2); // returns [3, 4, 5]
myArray.slice(1, 3); // returns [2, 3]
myArray.slice(- 3); // returns [3, 4, 5]
myArray.slice(-3, -1); // returns [3, 4]
myArray.slice(-4, 3); // returns [2, 3]
myArray.slice(3, 1); // returns []
var myArray = [1, 2, 3, 4, 5];
myArray.splice (3,2,''a'',''b'');
// returns 4,5 [1,2,3,''a'',''b'']
myArray .splice(1,1,"in","the","middle");
// returns 2 [1,"in","the","middle",3,''a'', ''b'']
After reading the documentation, I found out that arrays are passed by reference!
var x = [10, 9, 8];
var y = x;
x[0] = 2;
alert("The value of y's first element is: " y[0]);
What do you guess the result is?
Look at this again:
// Declare a reference type (array)
var refType = ["first ", " second", " third"];
// Declare a primitive type (number)
var primType = 10;
// Declare a function taking two arguments, which it will modify
function modifyValues(ref, prim)
{
ref[0] = "changed"; // modify the first argument, an array
prim = prim - 8; // modify the second, a number
}
// Invoke the function
modifyValues(refType, primType);
// Print the value of the reference type
document.writeln("The value of refType is: ", refType ">");
//Print the value of the primitive type
document.writeln("The value of primType is: ", primType);
A problem was found during testing:
var arr=[];
arr['a']=1;
arr['b']=2;
alert(arr.length);
The number that pops up is 0!
After reading the documentation, I learned that such arrays are called Associative Arrays. arr['a'] is equivalent to arr.a, arr.length is equivalent to arr['length'], and arr.length When we initialize arr (var arr=[];), it is automatically assigned a value of 0.
Some people call this associative array a JavaScript hash table. Strictly speaking, associative arrays and ordinary arrays themselves are objects (nonsense, everything in JavaScript is an object - -), and their meaning and usage are the same.Look at the following example:
var arr=[];
arr=[1,2,3];
arr.test='test';
alert(arr);
alert(arr[1]);
alert(arr['test']);
var arr={}; arr=[2,3,4]; alert(arr[0 ]);
var arr={}; and var arr=[] can both be written as var arr=function(){};
As can be seen from the above code, the subscript array and the associative array are independent. Subscripts only act on subscript arrays and cannot access associative arrays. Correspondingly, associative arrays do not affect the length attribute. The label of the associative array is an attribute, but the subscript of the subscript array is not an attribute. arr[0]!=arr.0, and an error will occur when accessing arr.0.
At some point, it will be more efficient to use arr['a'] instead of arr.a as a method call, because the 'a' in arr['a'] can be passed in as a variable, such as method Assign to variables:
var d=document,l=links;
At this time, an error will occur if you use direct d.l, but if you use d[l], it can be executed correctly. Alert You'll know :)
alert(d.l);//Script error
alert(d[l]);//Display object
Since the array is an object with lenght attribute, So are all objects with a length attribute an array? Such as String. After testing, I found that Firefox can process String as an array, but IE cannot:
var myString = "Hello world";
alert(myString.length);
alert (myString[0]);
The object of the array should be relatively unique, and the user object cannot be completely simulated:
function myarray(size){
this.length =size;
var x=0;
}
var arr=new myarray(5);
arr[9]=1;
alert(arr);
alert( arr[9]);
alert(arr.length);
I thought that the length attribute of Array is an ordinary object attribute. However, after the above example, I found that the length of arr is no longer the array length. The structure of arr is not an ordinary array structure.
There are several ways to define a function/class:
function fName(arguments){
//function body
};
var fName = function(arguments){
//function body
}
var fName = new Function("arguments","/*function body*/");
The third method is more fun, look at the following example:
var arr=new Function("var total=0; for (var i=0; i
Use This method can parse the JSON returned by ajax, so that the inefficient eval is not needed (no test whether it is faster than eval):
Assume {b:{c:2}} is the returned json string:
var arr=new Function("this.a={b:{c:2}};");
var aa =new arr();
alert(aa.a.b.c);

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


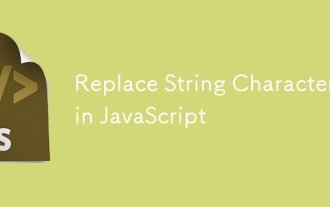
Detailed explanation of JavaScript string replacement method and FAQ This article will explore two ways to replace string characters in JavaScript: internal JavaScript code and internal HTML for web pages. Replace string inside JavaScript code The most direct way is to use the replace() method: str = str.replace("find","replace"); This method replaces only the first match. To replace all matches, use a regular expression and add the global flag g: str = str.replace(/fi
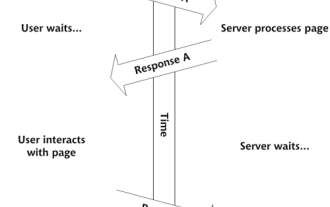
So here you are, ready to learn all about this thing called AJAX. But, what exactly is it? The term AJAX refers to a loose grouping of technologies that are used to create dynamic, interactive web content. The term AJAX, originally coined by Jesse J
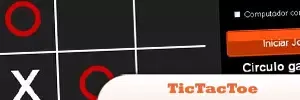
10 fun jQuery game plugins to make your website more attractive and enhance user stickiness! While Flash is still the best software for developing casual web games, jQuery can also create surprising effects, and while not comparable to pure action Flash games, in some cases you can also have unexpected fun in your browser. jQuery tic toe game The "Hello world" of game programming now has a jQuery version. Source code jQuery Crazy Word Composition Game This is a fill-in-the-blank game, and it can produce some weird results due to not knowing the context of the word. Source code jQuery mine sweeping game
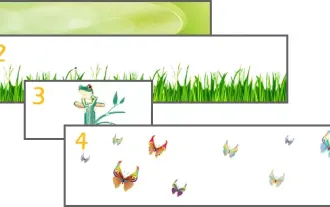
This tutorial demonstrates how to create a captivating parallax background effect using jQuery. We'll build a header banner with layered images that create a stunning visual depth. The updated plugin works with jQuery 1.6.4 and later. Download the
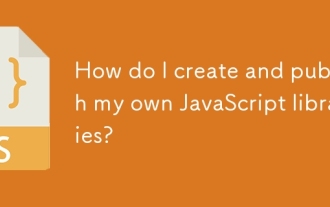
Article discusses creating, publishing, and maintaining JavaScript libraries, focusing on planning, development, testing, documentation, and promotion strategies.
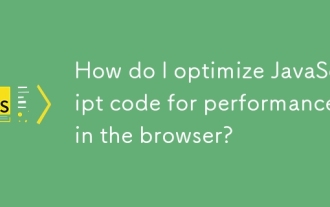
The article discusses strategies for optimizing JavaScript performance in browsers, focusing on reducing execution time and minimizing impact on page load speed.
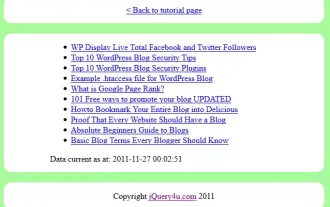
This article demonstrates how to automatically refresh a div's content every 5 seconds using jQuery and AJAX. The example fetches and displays the latest blog posts from an RSS feed, along with the last refresh timestamp. A loading image is optiona
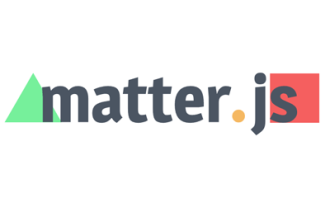
Matter.js is a 2D rigid body physics engine written in JavaScript. This library can help you easily simulate 2D physics in your browser. It provides many features, such as the ability to create rigid bodies and assign physical properties such as mass, area, or density. You can also simulate different types of collisions and forces, such as gravity friction. Matter.js supports all mainstream browsers. Additionally, it is suitable for mobile devices as it detects touches and is responsive. All of these features make it worth your time to learn how to use the engine, as this makes it easy to create a physics-based 2D game or simulation. In this tutorial, I will cover the basics of this library, including its installation and usage, and provide a
