


A very good continuous scrolling class, compatible with multiple browsers_javascript skills
How to call:
First you have to link the script to your page, or you can directly call the link below. Of course, it's best to download to your own machine.
sampleDiv.move();
This will create an uninterrupted DIV object based on a certain DIV object with id="divId" in the document Cycle through the scrolling area. The width of this area is 200px, the height is 100px, the background color is yellow (don't worry about your English being bad, you can also use a format like "#ff00ff"), and the direction is scrolling up. In fact, you can also choose to scroll left, just change "up" to "left". But scrolling to the right and down is not supported, so there is no need to try "right" and "down" - it is actually very easy to implement these two directions, but I personally think it is not practical, so I didn't do it - 1px per scroll The delay time is 10ms, which means that the ideal scrolling is 100px per second. Pause every 20px of scrolling, and each pause is 2 seconds. And supports mouse hover.
The order of the parameters mentioned above must be strictly adhered to, that is to say, they must be arranged in the following order. If you want to be lazy, you can use commas to skip it:
2. The width of the scrolling area, the default value is 200px, and all default values can be modified in scrollingAD;
3. The width of the scrolling area Height, the default value is 50px;
4. Background color, the default value is "transparent", which is transparent;
5. Direction, the optional value "up/left";
6. Every scroll The delay time of 1px, the default value is 20, the unit is ms - the larger the value, the slower the scrolling;
7. The stagnation time after each scrolling limit distance, the unit is also ms, the default value is 2000, which is 2 seconds - —If you don’t want to stagnate, just set it to 0;
8. The scrolling distance between each two stagnations, the default value is one screen. That is to say, if the direction you set is "up", the default value is equal to the height of the scroll area, otherwise the direction is "left", the default value is the width of the scroll area;
9. Whether to hover, the default is true, it doesn’t matter if you don’t fill it in;
The second method is like this:
sampleDiv.move();
All the values used in this way are default values, but you should If you feel that you have to change a certain parameter, you can do this:
var sampleDiv = new scrollingAD("divId");
sampleDiv.width = 500;
sampleDiv.height = 100;
sampleDiv.bgColor = "red";
sampleDiv.direction = "left";
sampleDiv.delay = 10;
sampleDiv.pauseTime = 1000;
sampleDiv.size = 50;
sampleDiv.isHover = false;
sampleDiv.move();
Of course you don’t need to write everything out like above, you just need to modify the necessary parameters. If you use the first method to create a scroll area, but later modify it in this way, the latter method shall prevail.
It should be noted that you should use this order to create this scroll area:
If you need to introduce external Js, you need to refresh it to execute
]
Tip: You can modify it first Run part of the code again
Update:
You can now use percentages to define the size parameter, like this:
var sampleDiv = new scrollingAD("sampleDiv");
sampleDiv.size = "50%" ;// Note, be sure to add quotation marks, otherwise an error will occur.
sampleDiv.move(); The effect obtained is that a cycle only scrolls twice. Similarly, if you scroll through a cycle at one time, just set size to "100%". However, it is not recommended to set the percentage arbitrarily. Please try to set it to a value consistent with the number of rows, otherwise unexpected blanks may appear. Of course, numbers are still supported :)

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


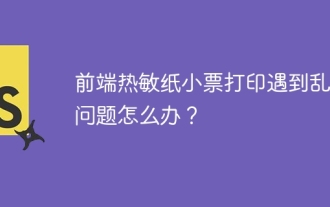
Frequently Asked Questions and Solutions for Front-end Thermal Paper Ticket Printing In Front-end Development, Ticket Printing is a common requirement. However, many developers are implementing...
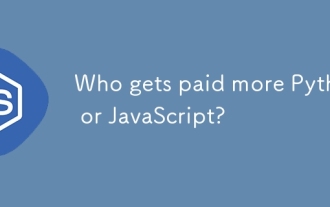
There is no absolute salary for Python and JavaScript developers, depending on skills and industry needs. 1. Python may be paid more in data science and machine learning. 2. JavaScript has great demand in front-end and full-stack development, and its salary is also considerable. 3. Influencing factors include experience, geographical location, company size and specific skills.
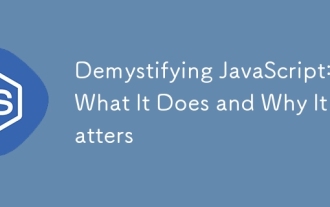
JavaScript is the cornerstone of modern web development, and its main functions include event-driven programming, dynamic content generation and asynchronous programming. 1) Event-driven programming allows web pages to change dynamically according to user operations. 2) Dynamic content generation allows page content to be adjusted according to conditions. 3) Asynchronous programming ensures that the user interface is not blocked. JavaScript is widely used in web interaction, single-page application and server-side development, greatly improving the flexibility of user experience and cross-platform development.
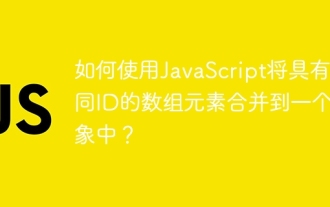
How to merge array elements with the same ID into one object in JavaScript? When processing data, we often encounter the need to have the same ID...
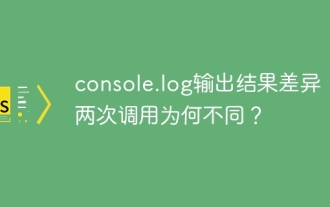
In-depth discussion of the root causes of the difference in console.log output. This article will analyze the differences in the output results of console.log function in a piece of code and explain the reasons behind it. �...
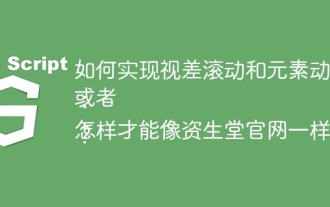
Discussion on the realization of parallax scrolling and element animation effects in this article will explore how to achieve similar to Shiseido official website (https://www.shiseido.co.jp/sb/wonderland/)...
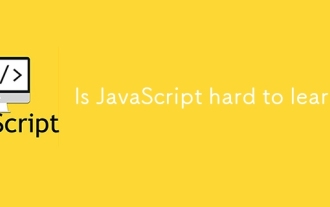
Learning JavaScript is not difficult, but it is challenging. 1) Understand basic concepts such as variables, data types, functions, etc. 2) Master asynchronous programming and implement it through event loops. 3) Use DOM operations and Promise to handle asynchronous requests. 4) Avoid common mistakes and use debugging techniques. 5) Optimize performance and follow best practices.
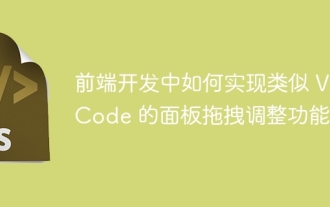
Explore the implementation of panel drag and drop adjustment function similar to VSCode in the front-end. In front-end development, how to implement VSCode similar to VSCode...
