prototype1.5 first experience_prototype
I have never had time to look at the prototype. Now it’s good. It has been updated to 1.5 pre1. Haha, I have to learn the powerful functions. This is another shortcut to improve my JS ability.
1. What is Prototype?
Maybe you haven’t used it yet, prototype.js is a JavaScript package written by Sam Stephenson. This wonderfully conceived, well-written, standards-compliant piece of code will shoulder the burden of creating thick-client, highly interactive web applications. Easily add Web 2.0 features.
If you have experienced this package recently, you will most likely find that documentation is not one of its strong points. Like all developers before me, I just dived into the prototype.js source code and experimented with every piece of it. I thought it would be nice to take notes as I studied him and share them with others.
I have also provided an unofficial reference for this package's objects, classes, methods and extensions.
2. Universal methods
This package contains many predefined objects and universal methods. The obvious purpose of writing these methods is to save you a lot of repetitive coding and idioms.
Starting from Prototype 1.5.x version, you can more conveniently operate DOM objects as follows:
var ele = $("myelement");
ele. hide(); //Hide DOM object compared to the previous version var ele = $("myelement");
Element.hide(ele); //Hide DOM object
What are the benefits of such a change Woolen cloth? I think firstly, it is more object-oriented, and secondly, it will facilitate future code prompts in the IDE.
2.1. Use the $() method
The $() method is a convenient shorthand for the document.getElementById() method that is used too frequently in DOM, just like this DOM method, This method returns the element with the id passed in as the parameter.
This is better than the method in DOM. You can pass multiple ids as parameters and $() returns an Array object with all required elements. The following example will describe this to you.
<script> <br> function test1() <br> { <br> var d = $('myp'); <br> alert(d.innerHTML); <br> } <br><br> function test2() <br> { <br> var ps = $('myp','myOtherp'); <br> for(i=0; i<ps.length; i ) <br> { <br> alert(ps[i].innerHTML); <br> } <br> } <br></script>
< BODY>
;
;
Another benefit of this method is that you can pass in the id string or the element object itself, which makes it very useful when creating methods that can pass in any formal parameters. .
2.2. Using the $F() method
The $F() method is another very popular shorthand. It can return the value of any input form control, such as a text box or drop-down box. This method can pass in the id of the element or the element itself.
<script> <br> function test3() <br> { <br> alert( $F('userName') ); <br> } <br></script>
2.3. Use Try.these() method
Try.these() method makes it possible to implement when you want to call different methods until one of them succeeds Normally, this requirement becomes very easy. It takes a series of methods as parameters and executes these methods one by one in order until one of them is successfully executed, and returns the return value of the successfully executed method.
In the example below, xmlNode.text works fine in some browsers, but xmlNode.textContent works fine in others. Using the Try.these() method we can get the return value of the method that works normally.
<script> <br>function getXmlNodeValue(xmlNode){ <br> return Try.these( <br> function() {return xmlNode.text;}, <br> function() {return xmlNode .textContent;) <br> ); <br>} <br></script> Generic methods are great, but let's face it, they're not the most advanced kind of thing. Are they? You've probably written these methods yourself or even have similar functionality in your scripts. But these methods are just the tip of the iceberg.
The Ajax object is a predefined object created by this package in order to encapsulate and simplify the tricky code involved in writing AJAX functionality. This object contains a series of classes that encapsulate AJAX logic. Let’s take a look at some of them.
3.1. Using the Ajax.Request class
If you don't use any helper packages, you're probably writing a whole lot of code to create the XMLHttpRequest object and track it asynchronously process, then parse out the response and process it. You'll be lucky when you don't need to support more than one type of browser.
To support AJAX function. This package defines the Ajax.Request class.
Suppose you have an application that can communicate with the server through the url http://www.php.cn/. It returns an XML response like below.
year-month>
🎜> id>
It is very simple to use the Ajax.Request object to communicate with the server and get this XML. The example below demonstrates how it is done.
<script> <br> function searchSales() <br> { <br> var empID = $F('lstEmployees'); <br> var y = $F('lstYears'); <br> var url = 'http://yoursever/app/get_sales'; <br> var pars = 'empID=' empID '&year=' y; <br> var myAjax = new Ajax.Request( <br> url, <br> ponse(originalRequest) <br> { <br> // put returned XML in the textarea <br> $('result').value = originalRequest.responseText; <br> } <br></script>
Did you see the second object passed into the Ajax.Request constructor? Parameters {method: 'get', parameters: pars, onComplete: showResponse} represent the actual writing of an anonymous object. He means that the object you passed in has an attribute named method with a value of 'get', another attribute named parameters that contains the query string of the HTTP request, and an onComplete attribute/method that contains the function showResponse.
There are some other properties that can be defined and set in this object, such as asynchronous, which can be true or false to determine whether the AJAX call to the server is asynchronous (the default value is true).
This parameter defines the options for the AJAX call. In our example, when requesting that url via the HTTP GET command as the first parameter, passing in the query string contained in the variable pars, the Ajax.Request object will call the showResponse method when it has finished receiving the response.
As you may know, XMLHttpRequest will report progress during the HTTP request. This progress is described as four different stages: Loading, Loaded, Interactive, or Complete. You can make the Ajax.Request object call custom methods at any stage, Complete is the most commonly used one. To call a custom method, simply provide a custom method object in the onXXXXX attribute/method in the option parameter of the request. Like onComplete in our example. The method you pass in will be called with one parameter, which is the XMLHttpRequest object itself. You will use this object to get the returned data and perhaps check the status attribute containing the HTTP result code in this call.
There are two other useful options for processing the results. We can pass a method in the onSuccess option and call it when AJAX is executed without error. On the contrary, we can also pass a method in the onFailure option and call it when an error occurs on the server side. Just like the methods passed in the onXXXXX option, these two also pass in an XMLHttpRequest object with an AJAX request when called.
Our example doesn’t process the XML response in any interesting way, we just put the XML into a text field. A typical application for this response might be to find the desired information in it and then update some elements in the page, or maybe even do some XSLT transformations to produce some HTML in the page.
3.2. Use the Ajax.Updater class
If the information returned by the other end of your server is already HTML, then using the Ajax.Updater class in this package will allow you to life becomes easier. With it you just need to provide which element needs to be filled with the HTML returned by the AJAX request. The example is clearer than what I wrote.
<script> <br> function getHTML() <br> { <br> var url = 'http://yourserver/app/getSomeHTML'; <br> var pars = 'someParameter=ABC' ; <br><br> var myAjax = new Ajax.Updater('placeholder', url, {method: 'get', parameters: pars}); <br><br> } <br></script>
You can see that this code is more concise than the previous example and does not include the onComplete method, but an element id is passed in the constructor. Let's modify the code slightly to describe how it is possible to handle server segmentation faults on the client side.
We will add more options to specify a way to handle errors. This is done using the onFailure option.
We also specify a placeholder that will only be filled after a successful request. To accomplish this we changed the first parameter from a simple element id to an object with two properties, success (used when everything is OK) and failure (used when something goes wrong) In the following example, the failure attribute is not used, but only the reportError method is used at onFailure.
<script> <br> function getHTML() <br> { <br> var url = 'http://yourserver/app/getSomeHTML'; <br> var pars = 'someParameter=ABC'; <br> var myAjax = new Ajax.Updater( <br> {success: 'placeholder'}, <br> url, <br> {method : 'get', parameters: pars, onFailure: reportError}); <br><br> } <br><br> function reportError(request) <br> { <br> alert('Sorry. There was an error.'); <br> } <br></script>
< input type=button value=GetHtml onclick="getHTML()">
If your server The logic is to return JavaScript code instead of simple HTML markup, and the Ajax.Updater object can execute that JavaScript code. To make this object treat responses as JavaScript, you simply add the evalScripts: true attribute to the last parameter of the object's constructor.

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
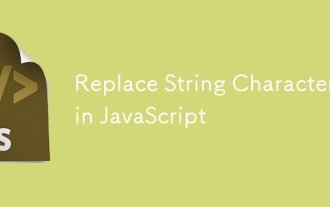
Detailed explanation of JavaScript string replacement method and FAQ This article will explore two ways to replace string characters in JavaScript: internal JavaScript code and internal HTML for web pages. Replace string inside JavaScript code The most direct way is to use the replace() method: str = str.replace("find","replace"); This method replaces only the first match. To replace all matches, use a regular expression and add the global flag g: str = str.replace(/fi
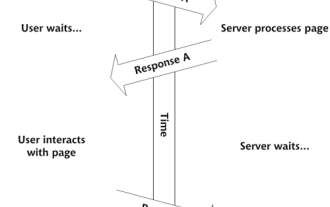
So here you are, ready to learn all about this thing called AJAX. But, what exactly is it? The term AJAX refers to a loose grouping of technologies that are used to create dynamic, interactive web content. The term AJAX, originally coined by Jesse J
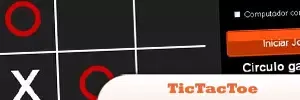
10 fun jQuery game plugins to make your website more attractive and enhance user stickiness! While Flash is still the best software for developing casual web games, jQuery can also create surprising effects, and while not comparable to pure action Flash games, in some cases you can also have unexpected fun in your browser. jQuery tic toe game The "Hello world" of game programming now has a jQuery version. Source code jQuery Crazy Word Composition Game This is a fill-in-the-blank game, and it can produce some weird results due to not knowing the context of the word. Source code jQuery mine sweeping game
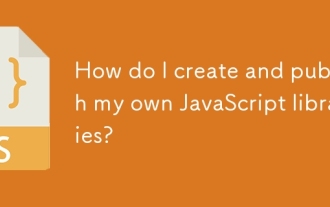
Article discusses creating, publishing, and maintaining JavaScript libraries, focusing on planning, development, testing, documentation, and promotion strategies.
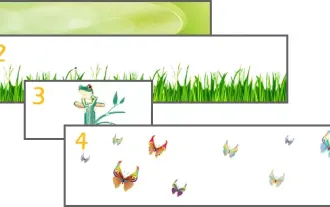
This tutorial demonstrates how to create a captivating parallax background effect using jQuery. We'll build a header banner with layered images that create a stunning visual depth. The updated plugin works with jQuery 1.6.4 and later. Download the
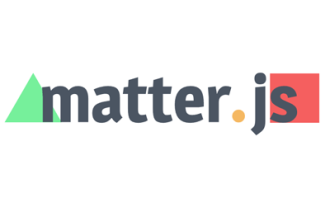
Matter.js is a 2D rigid body physics engine written in JavaScript. This library can help you easily simulate 2D physics in your browser. It provides many features, such as the ability to create rigid bodies and assign physical properties such as mass, area, or density. You can also simulate different types of collisions and forces, such as gravity friction. Matter.js supports all mainstream browsers. Additionally, it is suitable for mobile devices as it detects touches and is responsive. All of these features make it worth your time to learn how to use the engine, as this makes it easy to create a physics-based 2D game or simulation. In this tutorial, I will cover the basics of this library, including its installation and usage, and provide a
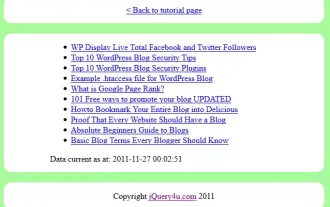
This article demonstrates how to automatically refresh a div's content every 5 seconds using jQuery and AJAX. The example fetches and displays the latest blog posts from an RSS feed, along with the last refresh timestamp. A loading image is optiona
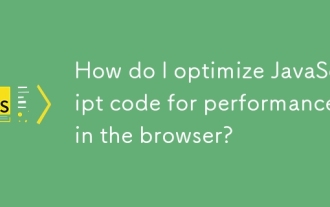
The article discusses strategies for optimizing JavaScript performance in browsers, focusing on reducing execution time and minimizing impact on page load speed.
