


jQuery determines that all images have been loaded_html/css_WEB-ITnose
For image processing, such as slide show, zoom, etc., all operations rely on completing all images.
Today, let’s look at how to determine when all images have been loaded. Before loading is complete, you can use the loading gif image to indicate that it is loading.
1. Ordinary methods
Monitor the load method of img and compare it every time an image is loaded. The key code is as follows:
var num = $img.length; $imgs.load(function() { num--; if (num > 0) { return; } console.log('load compeleted'); }
2. Use the Deferred object in jQuery
The Deferred object is a new feature introduced from jQuery version 1.5.0. For detailed introduction, please see the official documentation.
Simply put, the Deferred object is jQuery's callback function solution. It solves the problem of how to handle time-consuming operations, provides better control over those operations, and a unified programming interface.
Ruan Yifeng has an article that introduces Deferred objects. It is written in more detail and is more useful for getting started.
Detailed explanation of jQuery’s deferred object
Here, we used:
Key code:
var defereds = [];$imgs.each(function() { var dfd = $.Deferred(); $(this).load(dfd.resolve); defereds.push(dfd); }); $.when.apply(null, defereds).done(function() { console.log('load compeleted'); });
Basic idea:
resolve(), when() after each image is loaded done() is executed when all Deferreds are completed.
Note: Because the parameters supported by $.when are $.when(dfd1, dfd2, dfd3, ...), we use apply here to accept array parameters.

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


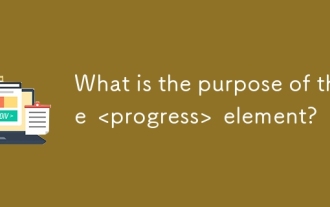
The article discusses the HTML <progress> element, its purpose, styling, and differences from the <meter> element. The main focus is on using <progress> for task completion and <meter> for stati
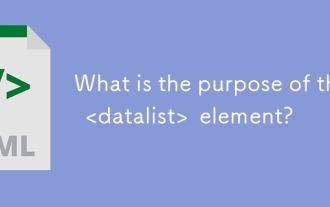
The article discusses the HTML <datalist> element, which enhances forms by providing autocomplete suggestions, improving user experience and reducing errors.Character count: 159

HTML is suitable for beginners because it is simple and easy to learn and can quickly see results. 1) The learning curve of HTML is smooth and easy to get started. 2) Just master the basic tags to start creating web pages. 3) High flexibility and can be used in combination with CSS and JavaScript. 4) Rich learning resources and modern tools support the learning process.
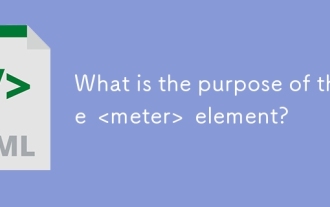
The article discusses the HTML <meter> element, used for displaying scalar or fractional values within a range, and its common applications in web development. It differentiates <meter> from <progress> and ex
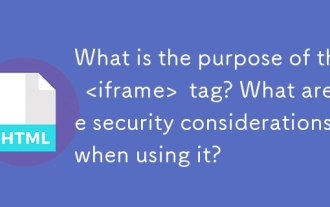
The article discusses the <iframe> tag's purpose in embedding external content into webpages, its common uses, security risks, and alternatives like object tags and APIs.
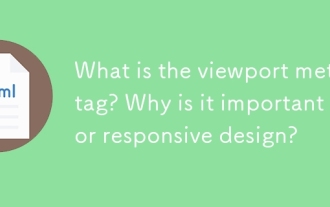
The article discusses the viewport meta tag, essential for responsive web design on mobile devices. It explains how proper use ensures optimal content scaling and user interaction, while misuse can lead to design and accessibility issues.

HTML defines the web structure, CSS is responsible for style and layout, and JavaScript gives dynamic interaction. The three perform their duties in web development and jointly build a colorful website.

AnexampleofastartingtaginHTMLis,whichbeginsaparagraph.StartingtagsareessentialinHTMLastheyinitiateelements,definetheirtypes,andarecrucialforstructuringwebpagesandconstructingtheDOM.
