Detailed explanation of variable usage in CSS_html/css_WEB-ITnose
Variables in CSS give us many advantages: convenience, code reuse, more reliable code base and improved error prevention capabilities.
Example
:root { --base-font-size: 16px; --link-color: #6495ed; }p { font-size: var( --base-font-size ); }a { font-size: var( --base-font-size ); color: var( --link-color ); }
Basics
There are three main components you should know when working with CSS variables:
Custom properties
You already know some standard CSS properties, such as color, margin, width and font -size.
The following example uses the CSS color property:
body { color: #000000; /* The "color" CSS property */ }
Custom properties simply means that we (the one who created the CSS file) define the name of the property.
In order to customize an attribute name, we need to use -- as a prefix.
If we want to create a custom property named text-color with a value of black, we can do this:
:root { --text-color: #000000; /* A custom property named "text-color" */ }
var() function
In order to apply the customization Attributes need to use the var() function, otherwise the browser will not know what they represent.
If you want to use --text-color in the styles in p, h1 and h2, you can use the var() function like this:
:root { --text-color: #000000; }p { color: var( --text-color ); font-size: 16px; }h1 { color: var( --text-color ); font-size: 42px; }h2 { color: var( --text-color ); font-size: 36px; }
It is equivalent to:
p { color: #000000; font-size: 16px; }h1 { color: #000000; font-size: 42px; }h2 { color: #000000; font-size: 36px; }
:root pseudo-class
We need a place to put custom attributes. Although custom properties can be specified in any style rule, it is not a good idea to define properties everywhere, which is a challenge for CSS maintainability and readability.
The :root pseudo-class represents the root element of an HTML document. This is a good place to put custom attributes because we can override custom attribute values through other more specific selectors.
Benefits of CSS variables
Maintainability
In a web development project, we often reuse a specific CSS property value:
h1 { margin-bottom: 20px; font-size: 42px; line-height: 120%; color: gray; }p { margin-bottom: 20px; font-size: 18px; line-height: 120%; color: gray; }img { margin-bottom: 20px; border: 1px solid gray; }.callout { margin-bottom: 20px; border: 3px solid gray; border-radius: 5px; }
The problem will be exposed when certain attribute values need to be changed.
If we change the property values manually, especially when the CSS file is large, it will not only take a lot of time, but also may cause some errors. Likewise, if we perform a batched find and replace, we may inadvertently affect other style rules.
We can use CSS variables to rewrite:
:root { --base-bottom-margin: 20px; --base-line-height: 120%; --text-color: gray; }h1 { margin-bottom: var( --base-bottom-margin ); font-size: 42px; line-height: var( --base-line-height ); color: var( --text-color ); }p { margin-bottom: var( --base-bottom-margin ); font-size: 18px; line-height: var( --base-line-height ); color: var( --text-color ); }img { margin-bottom: var( --base-bottom-margin ); border: 1px solid gray; }.callout { margin-bottom: var( --base-bottom-margin ); border: 1px solid gray; border-radius: 5px; color: var( --text-color ); }
Suppose your current customer says that the text color is too bright, making it difficult to read, and wants to change the text color. At this time, we only need to update one line CSS rules:
--text-color: black;
Improving the readability of CSS
Custom attributes will make the style sheet more readable and make CSS property declarations more semantic.
Compare this declaration
background-color: yellow;font-size: 18px;
with the following declaration
background-color: var( --highlight-color );font-size: var( --base-font-size );
Attribute values like yellow and 18px don’t give us any useful contextual information, but When we read property names like --base-font-size and --highlight-color, we immediately know what the property value is doing, even in other people's code.
Things to note
Case sensitive
Custom attributes are case sensitive (different from normal CSS rules)
:root { --main-bg-color: green; --MAIN-BG-COLOR: RED; }.box { background-color: var( --main-bg-color ); /* green background */ }.circle { BACKGROUND-COLOR: VAR(--MAIN-BG-COLOR ); /* red background */ border-radius: 9999em; }.box,.circle { height: 100px; width: 100px; margin-top: 25px; box-sizing: padding-box; padding-top: 40px; text-align: center; }
Parsing of custom attribute values
When a custom attribute is repeatedly declared, its assignment follows the usual CSS cascading and inheritance rules. For example, the following example:
HTML
<body> <div class="container"> <a href="">Link</a> </div></body>
CSS
:root { --highlight-color: yellow; }body { --highlight-color: green; }.container { --highlight-color: red; }a { color: var( --highlight-color ); }
When the .container rule is removed, the color value of the link will be green.
Fallback value
When using the var() function, you can assign a fallback attribute value as an extra parameter separated from the first parameter by,. Look at the following example:
HTML
<div class="box">A Box</div>
CSS
div { --container-bg-color: black; }.box { width: 100px; height: 100px; padding-top: 40px; box-sizing: padding-box; background-color: var( --container-bf-color, red ); color: white; text-align: center; }
Because a fallback value parameter is passed to var(), the background color of the div is rendered. into red.
Invalid value
Beware of assigning the wrong type of value to a CSS property.
In the following example, since the custom attribute --small-button is assigned a length unit, it is invalid when used in the .small-button style (Translator's Note: Because the color attribute type value is wrong)
:root { --small-button: 200px; }.small-button { color: var(--small-button); }
A good way to avoid this is to come up with descriptive custom property names. For example, name the above custom attribute --small-button-width
Browser support for CSS variables
CSS variables are very convenient to use, but the browser does not support it. Great:
Or click this link: var supported
This article is translated based on @Jacob Gube's "Introduction to CSS Variables". The entire translation contains my own understanding and thoughts. If translated If you don’t get it right or something is wrong, please ask your friends in the industry for advice. If you want to reprint this translation, please indicate the English source: http://sixrevisions.com/css/variables/.

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


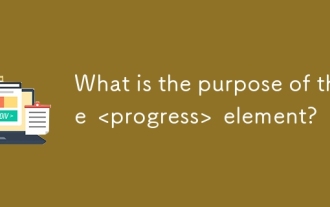
The article discusses the HTML <progress> element, its purpose, styling, and differences from the <meter> element. The main focus is on using <progress> for task completion and <meter> for stati
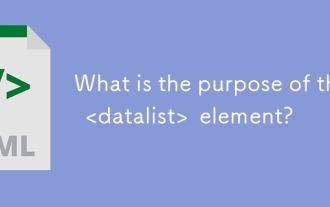
The article discusses the HTML <datalist> element, which enhances forms by providing autocomplete suggestions, improving user experience and reducing errors.Character count: 159
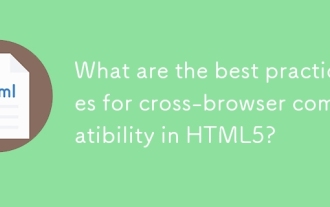
Article discusses best practices for ensuring HTML5 cross-browser compatibility, focusing on feature detection, progressive enhancement, and testing methods.
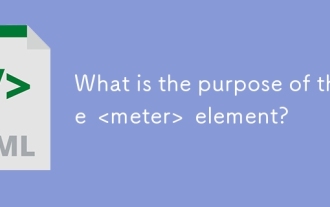
The article discusses the HTML <meter> element, used for displaying scalar or fractional values within a range, and its common applications in web development. It differentiates <meter> from <progress> and ex
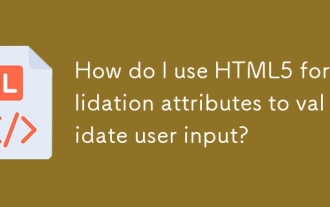
The article discusses using HTML5 form validation attributes like required, pattern, min, max, and length limits to validate user input directly in the browser.
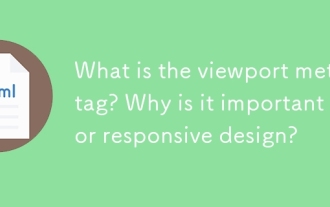
The article discusses the viewport meta tag, essential for responsive web design on mobile devices. It explains how proper use ensures optimal content scaling and user interaction, while misuse can lead to design and accessibility issues.
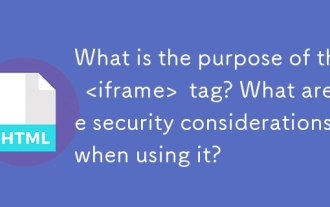
The article discusses the <iframe> tag's purpose in embedding external content into webpages, its common uses, security risks, and alternatives like object tags and APIs.
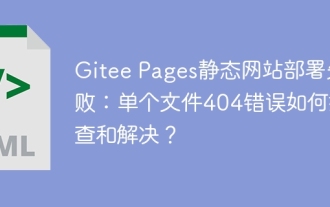
GiteePages static website deployment failed: 404 error troubleshooting and resolution when using Gitee...
