


Android Webview chooses to load local js, css and other resource files when loading external html_html/css_WEB-ITnose
When using WebView to load a web page, there are some fixed resource files such as js's jquery package, css, pictures and other resources that will be relatively large. If they are loaded directly from the network, the page will load slower and will Consumes more traffic. So these files should be placed in assets and packaged with the app.
To solve this problem, you need to use the shouldInterceptRequest(WebView view, String url) function provided by API 11 (HONEYCOMB) to load local resources. In API 21, this method was deprecated and a new shouldInterceptRequest was overloaded. The required parameters replaced url with request.
For example, if there is an image icon.png, which has been placed in assets, and now an external HTML is loaded, the image in assets needs to be directly taken out and loaded without re-obtaining it from the network. Of course, you can change the image link in html to file:///android_asset/icon.png, but then this html cannot be shared in android, ios, and WAP.
Implementation code:
webView.setWebViewClient(new WebViewClient() { @Override public WebResourceResponse shouldInterceptRequest(WebView view, String url) { WebResourceResponse response = null; if (Build.VERSION.SDK_INT >= Build.VERSION_CODES.HONEYCOMB){ response = super.shouldInterceptRequest(view,url); if (url.contains("icon.png")){ try { response = new WebResourceResponse("image/png","UTF-8",getAssets().open("icon.png")); } catch (IOException e) { e.printStackTrace(); } } }// return super.shouldInterceptRequest(view, url); return response; } @TargetApi(Build.VERSION_CODES.LOLLIPOP) @Override public WebResourceResponse shouldInterceptRequest(WebView view, WebResourceRequest request) { WebResourceResponse response = null; response = super.shouldInterceptRequest(view, request); if (url.contains("icon.png")){ try { response = new WebResourceResponse("image/png","UTF-8",getAssets().open("icon.png")); } catch (IOException e) { e.printStackTrace(); } } return response; }}

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


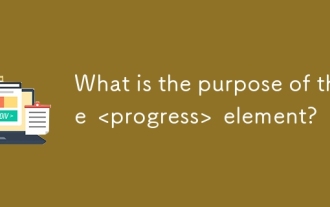
The article discusses the HTML <progress> element, its purpose, styling, and differences from the <meter> element. The main focus is on using <progress> for task completion and <meter> for stati
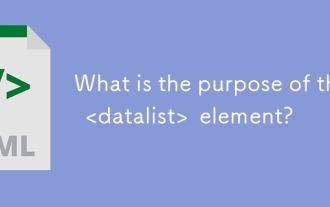
The article discusses the HTML <datalist> element, which enhances forms by providing autocomplete suggestions, improving user experience and reducing errors.Character count: 159
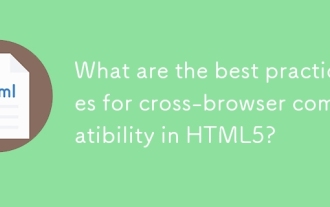
Article discusses best practices for ensuring HTML5 cross-browser compatibility, focusing on feature detection, progressive enhancement, and testing methods.
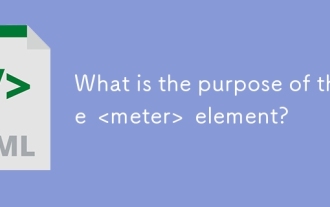
The article discusses the HTML <meter> element, used for displaying scalar or fractional values within a range, and its common applications in web development. It differentiates <meter> from <progress> and ex
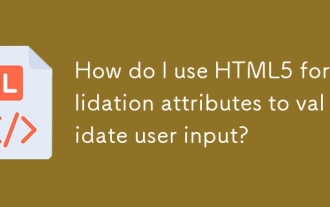
The article discusses using HTML5 form validation attributes like required, pattern, min, max, and length limits to validate user input directly in the browser.
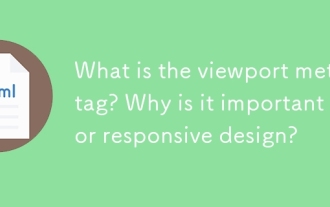
The article discusses the viewport meta tag, essential for responsive web design on mobile devices. It explains how proper use ensures optimal content scaling and user interaction, while misuse can lead to design and accessibility issues.
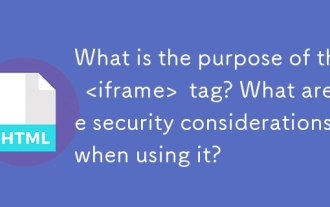
The article discusses the <iframe> tag's purpose in embedding external content into webpages, its common uses, security risks, and alternatives like object tags and APIs.
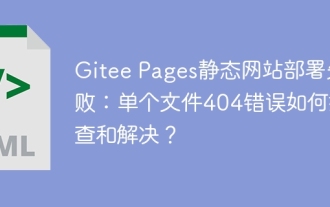
GiteePages static website deployment failed: 404 error troubleshooting and resolution when using Gitee...
