


ViewPager implements startup guidance page (personally think it is very detailed)_html/css_WEB-ITnose
The effect is as shown below:
The startup page is a picture delay effect, which is not shown here. The layout file is given.
WelcomeActivity analysis: Check whether it is the first time to run the program on the startup page. If so, first jump to the Activity of the boot interface??AndyViewPagerActivity; if not, jump directly to MainActivity.
The judgment is very simple, use SharedPreferences.
WelcomeActivity.java specific implementation:
package com.example.qidong; import android.os.Bundle; import android.os.Handler; import android.app.Activity; import android.content.Context; import android.content.Intent; import android.content.SharedPreferences; import android.content.SharedPreferences.Editor; public class WelcomeActivity extends Activity { private final int SPLASH_DISPLAY_LENGHT = 3000; // 延迟3秒 private SharedPreferences preferences; private Editor editor; @Override protected void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.welcome); preferences = getSharedPreferences("phone", Context.MODE_PRIVATE); new Handler().postDelayed(new Runnable() { @Override public void run() { if (preferences.getBoolean("firststart", true)) { editor = preferences.edit(); // 将登录标志位设置为false,下次登录时不再显示引导页面 editor.putBoolean("firststart", false); editor.commit(); Intent intent = new Intent(); intent.setClass(WelcomeActivity.this, AndyViewPagerActivity.class); WelcomeActivity.this.startActivity(intent); WelcomeActivity.this.finish(); } else { Intent intent = new Intent(); intent.setClass(WelcomeActivity.this, Manactivity2.class); WelcomeActivity.this.startActivity(intent); WelcomeActivity.this.finish(); } } }, SPLASH_DISPLAY_LENGHT); } }
The first is to guide the page layout: the parent layout is RelaytiveLayout; the child layout is ViewPager, and ViewPager fills the entire screen; the navigation points are 4 ImageViews and have a certain height relative to the bottom of the parent layout; then there is a Button above the navigation point
, and set its attribute android:visibility="gone" (when the control visibility attribute When it is INVISIBLE, the interface retains the space occupied by the view control; when the control property is GONE, the interface does not retain the space occupied by the view control).
Main.xml specific implementation:
<?xml version="1.0" encoding="utf-8"?><RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android" android:layout_width="fill_parent" android:layout_height="fill_parent" android:orientation="vertical" > <android.support.v4.view.ViewPager android:id="@+id/viewpager" android:layout_width="fill_parent" android:layout_height="fill_parent" /> <LinearLayout android:id="@+id/ll" android:orientation="horizontal" android:layout_width="wrap_content" android:layout_height="wrap_content" android:layout_marginBottom="24.0dip" android:layout_alignParentBottom="true" android:layout_centerHorizontal="true"> <ImageView android:layout_width="wrap_content" android:layout_height="wrap_content" android:layout_gravity="center_vertical" android:clickable="true" android:padding="15.0dip" android:src="@drawable/dot" /> <ImageView android:layout_width="wrap_content" android:layout_height="wrap_content" android:layout_gravity="center_vertical" android:clickable="true" android:padding="15.0dip" android:src="@drawable/dot" /> <ImageView android:layout_width="wrap_content" android:layout_height="wrap_content" android:layout_gravity="center_vertical" android:clickable="true" android:padding="15.0dip" android:src="@drawable/dot" /> <ImageView android:layout_width="wrap_content" android:layout_height="wrap_content" android:layout_gravity="center_vertical" android:clickable="true" android:padding="15.0dip" android:src="@drawable/dot" /> </LinearLayout> <Button android:id="@+id/button" android:background="@android:color/dark_gray" android:layout_width="wrap_content" android:layout_height="wrap_content" android:text="立即体验" android:layout_above="@id/ll" android:layout_centerHorizontal="true" android:visibility="gone" /> </RelativeLayout>
Next, first define a class ViewPagerAdapter to inherit PagerAdapter. PagerAdapter is an adapter that manages each View of ViewPager.
ViewPagerAdapter.java specific implementation:
package com.example.qidong; import java.util.List; import android.os.Parcelable; import android.support.v4.view.PagerAdapter; import android.support.v4.view.ViewPager; import android.view.View; public class ViewPagerAdapter extends PagerAdapter{ //界面列表 private List<View> views; public ViewPagerAdapter (List<View> views){ this.views = views; } //删除界面 @Override public void destroyItem(View arg0, int arg1, Object arg2) { ((ViewPager) arg0).removeView(views.get(arg1)); } @Override public void finishUpdate(View arg0) { // TODO Auto-generated method stub } //获得当前界面数量 @Override public int getCount() { if (views != null) { return views.size(); } return 0; } //初始化arg1位置的界面 @Override public Object instantiateItem(View arg0, int arg1) { ((ViewPager) arg0).addView(views.get(arg1), 0); return views.get(arg1); } //判断是否由对象生成界面 @Override public boolean isViewFromObject(View arg0, Object arg1) { return (arg0 == arg1); } @Override public void restoreState(Parcelable arg0, ClassLoader arg1) { // TODO Auto-generated method stub } @Override public Parcelable saveState() { // TODO Auto-generated method stub return null; } @Override public void startUpdate(View arg0) { // TODO Auto-generated method stub } }
The next step is AndyViewPagerActivity.java. AndyViewPagerActivity inherits Activity and implements the OnPageChangeListener interface. When the page changes, the system calls the onPageSelected() method of the OnPageChangeListener interface
and overrides this method. In this method, the selected state of the small dot at the bottom is changed, which includes changing the small circle before the interface slides. The state of the point is unselected, and the state of the small dot in the current interface is changed to selected. So set a
global variable currentIndex to record the position of the interface before sliding (it is an int value).
AndyViewPagerActivity.java specific implementation:
package com.example.qidong; import java.util.ArrayList; import java.util.List; import android.app.Activity; import android.content.Intent; import android.os.Bundle; import android.support.v4.view.ViewPager; import android.support.v4.view.ViewPager.OnPageChangeListener; import android.view.View; import android.view.View.OnClickListener; import android.widget.Button; import android.widget.ImageView; import android.widget.LinearLayout; public class AndyViewPagerActivity extends Activity implements OnPageChangeListener { private ViewPager vp; private ViewPagerAdapter vpAdapter; private List<View> views; private Button button; // 引导图片资源 private static final int[] pics = { R.drawable.guide1, R.drawable.guide2, R.drawable.guide3, R.drawable.guide4 }; // 底部小店图片 private ImageView[] dots; // 记录当前选中位置 private int currentIndex; /** Called when the activity is first created. */ @Override public void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.main); initView(); views = new ArrayList<View>(); // 为引导图片提供布局参数 LinearLayout.LayoutParams mParams = new LinearLayout.LayoutParams( LinearLayout.LayoutParams.WRAP_CONTENT, LinearLayout.LayoutParams.WRAP_CONTENT); // 初始化引导图片列表 for (int i = 0; i < pics.length; i++) { ImageView iv = new ImageView(this); iv.setLayoutParams(mParams); iv.setImageResource(pics[i]); views.add(iv); } // 初始化Adapter vpAdapter = new ViewPagerAdapter(views); vp.setAdapter(vpAdapter); // 绑定回调 vp.setOnPageChangeListener(this); button.setOnClickListener(new OnClickListener() { @Override public void onClick(View arg0) { Intent intent = new Intent(); intent.setClass(AndyViewPagerActivity.this, Manactivity2.class); AndyViewPagerActivity.this.startActivity(intent); finish(); } }); } private void initView() { button = (Button) findViewById(R.id.button); vp = (ViewPager) findViewById(R.id.viewpager); // 初始化底部小圆点 initDots(); } private void initDots() { LinearLayout ll = (LinearLayout) findViewById(R.id.ll); dots = new ImageView[pics.length]; // 循环取得小点图片 for (int i = 0; i < pics.length; i++) { //View android.view.ViewGroup.getChildAt(int index): //Returns the view at the specified position in the group. dots[i] = (ImageView) ll.getChildAt(i); dots[i].setEnabled(false);// 都设为灰色 dots[i].setTag(i);// 设置位置tag,方便取出与当前位置对应 //View中的setTag(Onbect)表示给View添加一个格外的数据,以后可以用getTag()将这个数据取出来。 } currentIndex = 0; dots[currentIndex].setEnabled(true);// 设置为红色,即选中状态 } /** * 改变当前引导小点颜色? */ private void setCurDot(int positon) { if (positon < 0 || positon > pics.length - 1 || currentIndex == positon) { return; } System.out.println("positon="+positon); dots[positon].setEnabled(true); //此时的currentIndex指的是上一个圆点 System.out.println("currentIndex="+currentIndex); dots[currentIndex].setEnabled(false); //现在的currentIndex指的是当前的小圆点 currentIndex = positon; } // 当滑动状态改变时调用 @Override public void onPageScrollStateChanged(int arg0) { // TODO Auto-generated method stub } // 当前页面被滑动时调用 @Override public void onPageScrolled(int arg0, float arg1, int arg2) { // TODO Auto-generated method stub } // 当新的页面被选中时调用 @Override public void onPageSelected(int arg0) { // 设置底部小点选中状态 setCurDot(arg0); if (arg0 == 3) { button.setVisibility(View.VISIBLE); } else { button.setVisibility(View.GONE); } } }

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


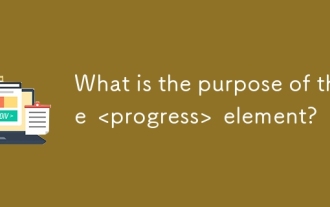
The article discusses the HTML <progress> element, its purpose, styling, and differences from the <meter> element. The main focus is on using <progress> for task completion and <meter> for stati

HTML is suitable for beginners because it is simple and easy to learn and can quickly see results. 1) The learning curve of HTML is smooth and easy to get started. 2) Just master the basic tags to start creating web pages. 3) High flexibility and can be used in combination with CSS and JavaScript. 4) Rich learning resources and modern tools support the learning process.
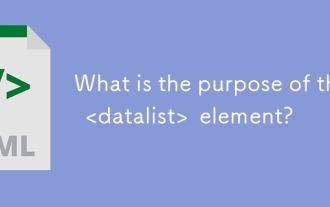
The article discusses the HTML <datalist> element, which enhances forms by providing autocomplete suggestions, improving user experience and reducing errors.Character count: 159
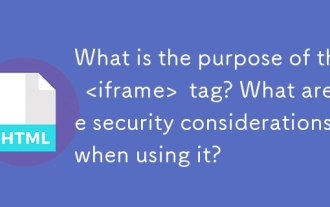
The article discusses the <iframe> tag's purpose in embedding external content into webpages, its common uses, security risks, and alternatives like object tags and APIs.
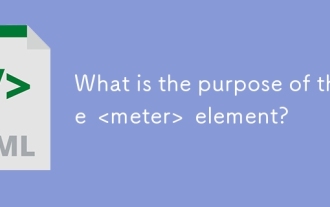
The article discusses the HTML <meter> element, used for displaying scalar or fractional values within a range, and its common applications in web development. It differentiates <meter> from <progress> and ex
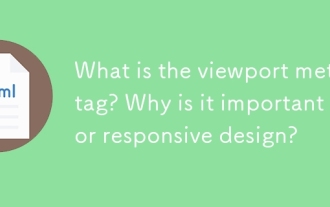
The article discusses the viewport meta tag, essential for responsive web design on mobile devices. It explains how proper use ensures optimal content scaling and user interaction, while misuse can lead to design and accessibility issues.

HTML defines the web structure, CSS is responsible for style and layout, and JavaScript gives dynamic interaction. The three perform their duties in web development and jointly build a colorful website.

WebdevelopmentreliesonHTML,CSS,andJavaScript:1)HTMLstructurescontent,2)CSSstylesit,and3)JavaScriptaddsinteractivity,formingthebasisofmodernwebexperiences.
