Ajax in brief_html/css_WEB-ITnose
You must be familiar with Ajax, but do you really want to talk about what it is? It is estimated that not many people have given a complete definition.
The specific definition of Ajax from W3C is:
AJAX = Asynchronous JavaScript and XML (asynchronous JavaScript and XML).
In other words, AJAX is the art of exchanging data with the server and updating parts of the web page without reloading the entire page.
AJAX is a technology for creating fast, dynamic web pages.
AJAX allows web pages to be updated asynchronously by exchanging a small amount of data with the server in the background. This means that parts of a web page can be updated without reloading the entire page.
Traditional web pages (not using AJAX) must reload the entire web page if the content needs to be updated.
Of course we must have a basic understanding of the following knowledge before learning Ajax:
XMLHttpRequest is the basis of AJAX.
All modern browsers support the XMLHttpRequest object (IE5 and IE6 use ActiveXObject). All modern browsers (IE7, Firefox, Chrome, Safari and Opera) have built-in XMLHttpRequest objects.
XMLHttpRequest is used to exchange data with the server in the background. This means that parts of a web page can be updated without reloading the entire page.
The specific code to create XMLHttpRequest is as follows:
//为了应对所有的现代浏览器,包括 IE5 和 IE6,请检查浏览器是否支持 XMLHttpRequest 对象。如果支持,则创建 XMLHttpRequest 对象。如果不支持,则创建 ActiveXObject var xmlhttp;if (window.XMLHttpRequest) {// code for IE7+, Firefox, Chrome, Opera, Safari xmlhttp = new XMLHttpRequest();}else {// code for IE6, IE5 老版本的 Internet Explorer (IE5 和 IE6)使用 ActiveX 对象 xmlhttp = new ActiveXObject("Microsoft.XMLHTTP");}
Before introducing the XMLHttpRequest object for exchanging data with the server, let’s briefly understand it Let’s take a look at the pros and cons of the get and post methods to facilitate choosing a more suitable request method during subsequent development.
Compared to POST, GET is simpler and faster, and works in most cases.
However, use POST requests in the following situations:
XMLHttpRequest exchanges data with the server mainly through the open and send methods, where if If the get method is used in the open method, then the send method does not need to pass any parameters. If the post method is used, then what is passed in the send method is a string similar to querystring.
The following is a brief introduction to several main methods:
Method | Description | ||||||||
open(method,url,async) |
| ||||||||
send(string) | <🎜>Sends the request to the server. <🎜> <🎜>string: only used for POST requests <🎜> | ||||||||
setRequestHeader(header,value) | <🎜>Add HTTP to the request head. <🎜> <🎜>header: specifies the name of the header <🎜> <🎜>value: specifies the value of the header <🎜> |
事实上,open方法中的async参数如果设计为false的话,那么发送的请求则跟传统的方式没有区别,也就是说必须等待服务器端数据回来之后才能接着进行下步操作。javaScript 会等到服务器响应就绪才继续执行。如果服务器繁忙或缓慢,应用程序会挂起或停止。所以我们不推荐使用 async=false。
当您使用 async=false 时,请不要编写 onreadystatechange 函数 - 把代码放到 send() 语句后面即可:
xmlhttp.open("GET", "test1.txt", false);xmlhttp.send();document.getElementById("myDiv").innerHTML = xmlhttp.responseText;
当使用 async=true 时,请规定在响应处于 onreadystatechange 事件中的就绪状态时执行的函数:
xmlhttp.onreadystatechange = function () { if (xmlhttp.readyState == 4 && xmlhttp.status == 200) { document.getElementById("myDiv").innerHTML = xmlhttp.responseText; }}xmlhttp.open("GET", "test1.txt", true);xmlhttp.send();
大家注意当使用async=true 时,它的返回值当中有两个重要的属性,那便是responseText 和responseXML 。其中responseText 获得字符串形式的响应数据而responseXML 获得 XML 形式的响应数据。如果来自服务器的响应并非 XML,请使用 responseText 属性。如果来自服务器的响应是 XML,而且需要作为 XML 对象进行解析,请使用 responseXML 属性。
在 onreadystatechange 事件中,我们规定当服务器响应已做好被处理的准备时所执行的任务。
当 readyState 等于 4 且状态为 200 时,表示响应已就绪
当请求被发送到服务器时,我们需要执行一些基于响应的任务。
每当 readyState 改变时,就会触发 onreadystatechange 事件。
readyState 属性存有 XMLHttpRequest 的状态信息。
下面是 XMLHttpRequest 对象的三个重要的属性:
onreadystatechange | 存储函数(或函数名),每当 readyState 属性改变时,就会调用该函数。 |
readyState | 存有 XMLHttpRequest 的状态。从 0 到 4 发生变化。 |
status | 200: "OK" 404: 未找到页面 |
下面以一个简单的demo温习一下上述介绍的基础知识
<!DOCTYPE html PUBLIC "-//W3C//DTD XHTML 1.0 Transitional//EN" "http://www.w3.org/TR/xhtml1/DTD/xhtml1-transitional.dtd"><html xmlns="http://www.w3.org/1999/xhtml"><head> <title></title> <script type="text/javascript"> var xmlhttp; function loadXMLDoc(url) { xmlhttp = null; if (window.XMLHttpRequest) {// code for Firefox, Opera, IE7, etc. xmlhttp = new XMLHttpRequest(); } else if (window.ActiveXObject) {// code for IE6, IE5 xmlhttp = new ActiveXObject("Microsoft.XMLHTTP"); } if (xmlhttp != null) { xmlhttp.onreadystatechange = state_Change; xmlhttp.open("GET", url, true); xmlhttp.send(null); } else { alert("Your browser does not support XMLHTTP."); } } function state_Change() { if (xmlhttp.readyState == 4) {// 4 = "loaded" if (xmlhttp.status == 200) {// 200 = "OK" document.getElementById('T1').innerHTML = xmlhttp.responseText; } else { alert("Problem retrieving data:" + xmlhttp.statusText); } } } </script></head><body onload="loadXMLDoc('example/ajax/test_xmlhttp.txt')"> <div id="T1" style="border: 1px solid black; height: 40px; width: 300px; padding: 5px"> </div> <br /> <button onclick="loadXMLDoc('example/ajax/test_xmlhttp2.txt')"> Click</button></body></html>

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


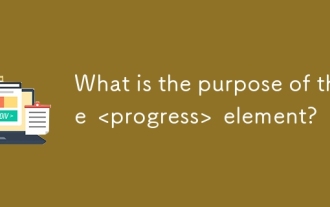
The article discusses the HTML <progress> element, its purpose, styling, and differences from the <meter> element. The main focus is on using <progress> for task completion and <meter> for stati
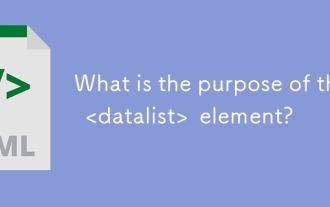
The article discusses the HTML <datalist> element, which enhances forms by providing autocomplete suggestions, improving user experience and reducing errors.Character count: 159
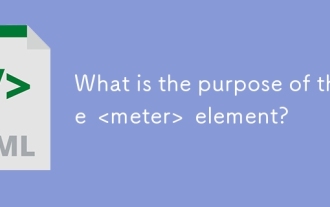
The article discusses the HTML <meter> element, used for displaying scalar or fractional values within a range, and its common applications in web development. It differentiates <meter> from <progress> and ex
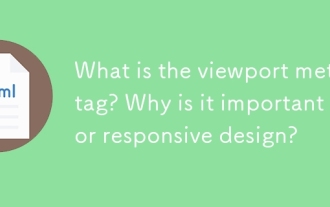
The article discusses the viewport meta tag, essential for responsive web design on mobile devices. It explains how proper use ensures optimal content scaling and user interaction, while misuse can lead to design and accessibility issues.
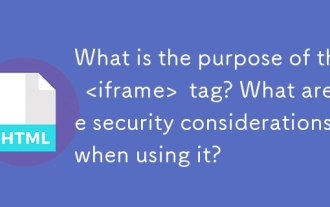
The article discusses the <iframe> tag's purpose in embedding external content into webpages, its common uses, security risks, and alternatives like object tags and APIs.

HTML is suitable for beginners because it is simple and easy to learn and can quickly see results. 1) The learning curve of HTML is smooth and easy to get started. 2) Just master the basic tags to start creating web pages. 3) High flexibility and can be used in combination with CSS and JavaScript. 4) Rich learning resources and modern tools support the learning process.

HTML defines the web structure, CSS is responsible for style and layout, and JavaScript gives dynamic interaction. The three perform their duties in web development and jointly build a colorful website.

AnexampleofastartingtaginHTMLis,whichbeginsaparagraph.StartingtagsareessentialinHTMLastheyinitiateelements,definetheirtypes,andarecrucialforstructuringwebpagesandconstructingtheDOM.
