


requireJS loads different versions of jquery_html/css_WEB-ITnose in the same HTML/JSP page
requireJS supports JS modularization and can also load different versions of modules on the same HTML/JSP page. This feature is great, let’s see how to load multiple versions of jquery. Assume that in the HTML page, the data-main attribute entry file is main.js, and the directory structure where the file is stored is as follows:
test.html
require.js
main. js
scripts/
demo1.js
demo2.js
libs/
jquery-1.10.2.js
jquery-2.1.1.js
We write the following code in main.js:
//创建1个contextvar reqOne = requirejs.config({ baseUrl: 'scripts/libs/', context:"context1", paths:{ jquery: 'jquery-1.10.2' }}); reqOne(['jquery'], function($) { console.log("v1=" + $().jquery); });//创建1个context var reqTwo = requirejs.config({ baseUrl: 'scripts/libs/', context:"context2", paths:{ jquery: 'jquery-2.1.1' }}); reqTwo(['require','jquery'], function(require,$) { console.log("v1=" + $().jquery); });
Through this multi-context approach, we can Implement multi-version module coexistence. But it also leads to two problems:
1. What happens if the modules loaded in different contexts are the same and have the same version (the same js file)? Will this js file be downloaded multiple times? ?
Conclusion: Through httpwatch observation, if different contexts load the same js file, then this js file will be downloaded multiple times. Obviously this is wasteful.
2. If both contexts require jquery-1.10.1 version, then how to ensure that only js is downloaded once?
Conclusion: We can define a context similar to the parent class, Store public modules, and each context can access the rootContext.
//定义rootContextvar rootContext = require.config({ baseUrl: 'scripts/'});var reqOne = requirejs.config({ baseUrl: 'scripts/libs/', context:"context1", paths:{ jquery: 'jquery-1.10.2' }});reqOne(['jquery'], function($) { console.log("v1=" + $().jquery); // 访问rootContext中的JS模块 rootContext(['demo1'],function(m){ console.log("require1=" + m.name); }); }); var reqTwo = requirejs.config({ baseUrl: 'scripts/libs/', context:"context2", paths:{ jquery: 'jquery-2.1.1' }}); reqTwo(['require','jquery'], function(require,$) { console.log("v1=" + $().jquery); // 访问rootContext中的JS模块 rootContext(['demo1'],function(m){ console.log("require1=" + m.name); }); });

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


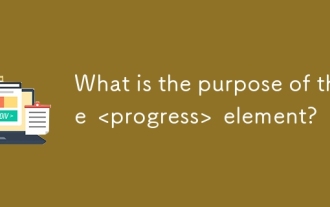
The article discusses the HTML <progress> element, its purpose, styling, and differences from the <meter> element. The main focus is on using <progress> for task completion and <meter> for stati

HTML is suitable for beginners because it is simple and easy to learn and can quickly see results. 1) The learning curve of HTML is smooth and easy to get started. 2) Just master the basic tags to start creating web pages. 3) High flexibility and can be used in combination with CSS and JavaScript. 4) Rich learning resources and modern tools support the learning process.
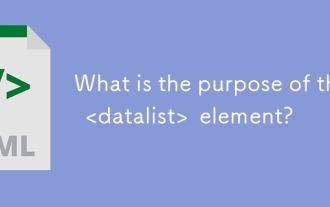
The article discusses the HTML <datalist> element, which enhances forms by providing autocomplete suggestions, improving user experience and reducing errors.Character count: 159
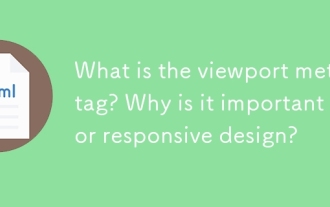
The article discusses the viewport meta tag, essential for responsive web design on mobile devices. It explains how proper use ensures optimal content scaling and user interaction, while misuse can lead to design and accessibility issues.
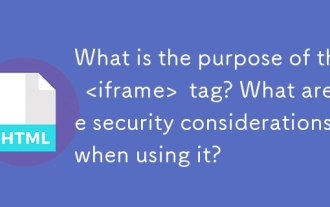
The article discusses the <iframe> tag's purpose in embedding external content into webpages, its common uses, security risks, and alternatives like object tags and APIs.

HTML defines the web structure, CSS is responsible for style and layout, and JavaScript gives dynamic interaction. The three perform their duties in web development and jointly build a colorful website.
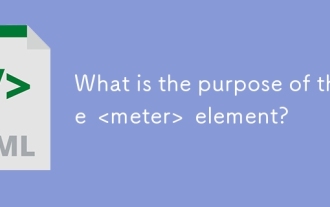
The article discusses the HTML <meter> element, used for displaying scalar or fractional values within a range, and its common applications in web development. It differentiates <meter> from <progress> and ex

WebdevelopmentreliesonHTML,CSS,andJavaScript:1)HTMLstructurescontent,2)CSSstylesit,and3)JavaScriptaddsinteractivity,formingthebasisofmodernwebexperiences.
