


Complete example of SHA-1 encryption algorithm implemented in JavaScript_javascript skills
May 16, 2016 pm 03:16 PMThe example in this article describes the SHA-1 encryption algorithm implemented in JavaScript. Share it with everyone for your reference, the details are as follows:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 98 99 100 101 102 103 104 105 106 107 108 109 110 111 112 113 114 115 116 117 118 119 120 121 122 123 124 125 126 127 128 129 130 131 132 133 134 135 136 137 138 |
|
Readers who are interested in more JavaScript-related content can check out the special topics on this site: "Summary of JavaScript data structure and algorithm techniques" and "Summary of JavaScript mathematical operation usage"
I hope this article will be helpful to everyone in JavaScript programming.

Hot Article

Hot tools Tags

Hot Article

Hot Article Tags

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
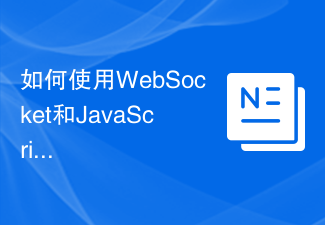
How to implement an online speech recognition system using WebSocket and JavaScript
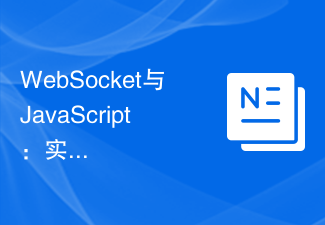
WebSocket and JavaScript: key technologies for implementing real-time monitoring systems
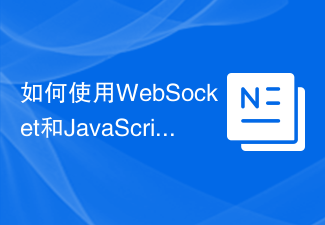
How to implement an online reservation system using WebSocket and JavaScript
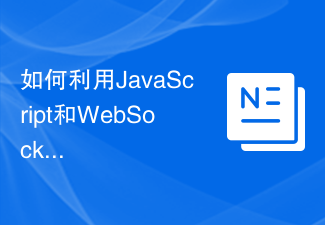
How to use JavaScript and WebSocket to implement a real-time online ordering system
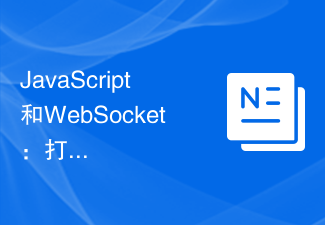
JavaScript and WebSocket: Building an efficient real-time weather forecasting system
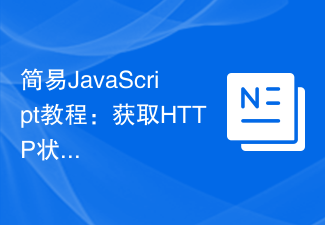
Simple JavaScript Tutorial: How to Get HTTP Status Code
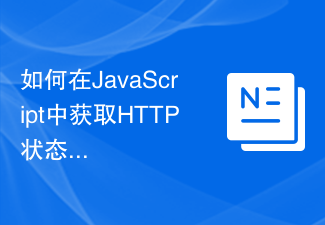
How to get HTTP status code in JavaScript the easy way

How to use insertBefore in javascript
