


Learn PHP design patterns PHP implements the adapter pattern_php skills
1. Intention
Convert the interface of a class into another interface desired by the client. The Adapter mode allows classes that were originally unable to work together due to incompatible interfaces to work together
2. Adapter pattern structure diagram
3. Main characters in adapter mode
Target role: Defines the interface used by the client related to a specific domain. This is what we expect
Source (Adaptee) role: interface that needs to be adapted
Adapter role: Adapt the Adaptee interface and the Target interface; the adapter is the core of this model. The adapter converts the source interface into the target interface. This role is a concrete class
4. Applicable Scenarios of Adapter Mode
1. You want to use an existing class, but its interface does not meet your needs
2. You want to create a reusable class that can work with other unrelated or unforeseen classes
3. You want to use an existing subclass, but it's not possible to subclass each one to match their interface. Object adapter can adapt to its parent class interface (object adapter only)
5. Class Adapter Pattern and Object Adapter
Class adapter: Adapter and Adaptee are inheritance relationships
1. Use a specific Adapter class to match the Target. The result is that when we want to match a class and all its subclasses, the class Adapter will not do the job
2. Allow Adapter to redefine some behaviors of Adaptee, because Adapter is a subset of Adaptee
3. Only introduce an object, no additional pointers are needed to indirectly obtain the adaptee
Object adapter: Adapter and Adaptee are in a delegation relationship
1. Allow one Adapter to work with multiple Adaptee at the same time. Adapter can also add functions to all Adaptees at once
2. It is difficult to redefine the behavior of Adaptee
Adapter pattern and other patterns
Bridge mode (bridge mode): The bridge mode is similar to the object adapter, but the starting point of the bridge mode is different: the purpose of the bridge mode is to separate the interface part and the implementation part, so that they can be changed relatively easily and relatively independently. The object adapter pattern means changing the interface of an existing object
Decorator mode (decorator mode): Decoration mode enhances the functionality of other objects without changing its interface. Decoration mode therefore provides better transparency to the application than adapters.
6. Class Adapter Pattern PHP Example
Class adapters use inheritance
<?php /** * 目标角色 */ interface Target { /** * 源类也有的方法1 */ public function sampleMethod1(); /** * 源类没有的方法2 */ public function sampleMethod2(); } /** * 源角色 */ class Adaptee { /** * 源类含有的方法 */ public function sampleMethod1() { echo 'Adaptee sampleMethod1 <br />'; } } /** * 类适配器角色 */ class Adapter extends Adaptee implements Target { /** * 源类中没有sampleMethod2方法,在此补充 */ public function sampleMethod2() { echo 'Adapter sampleMethod2 <br />'; } } class Client { /** * Main program. */ public static function main() { $adapter = new Adapter(); $adapter->sampleMethod1(); $adapter->sampleMethod2(); } } Client::main(); ?>
7. Object Adapter Pattern PHP Example
The object adapter uses delegation
<?php /** * 目标角色 */ interface Target { /** * 源类也有的方法1 */ public function sampleMethod1(); /** * 源类没有的方法2 */ public function sampleMethod2(); } /** * 源角色 */ class Adaptee { /** * 源类含有的方法 */ public function sampleMethod1() { echo 'Adaptee sampleMethod1 <br />'; } } /** * 类适配器角色 */ class Adapter implements Target { private $_adaptee; public function __construct(Adaptee $adaptee) { $this->_adaptee = $adaptee; } /** * 委派调用Adaptee的sampleMethod1方法 */ public function sampleMethod1() { $this->_adaptee->sampleMethod1(); } /** * 源类中没有sampleMethod2方法,在此补充 */ public function sampleMethod2() { echo 'Adapter sampleMethod2 <br />'; } } class Client { /** * Main program. */ public static function main() { $adaptee = new Adaptee(); $adapter = new Adapter($adaptee); $adapter->sampleMethod1(); $adapter->sampleMethod2(); } } Client::main(); ?>
The above is the code to implement the adapter mode using PHP. There are also some conceptual distinctions about the adapter mode. I hope it will be helpful to everyone's learning.

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


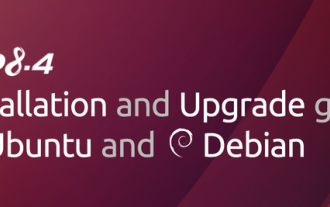
PHP 8.4 brings several new features, security improvements, and performance improvements with healthy amounts of feature deprecations and removals. This guide explains how to install PHP 8.4 or upgrade to PHP 8.4 on Ubuntu, Debian, or their derivati
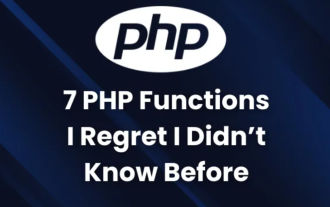
If you are an experienced PHP developer, you might have the feeling that you’ve been there and done that already.You have developed a significant number of applications, debugged millions of lines of code, and tweaked a bunch of scripts to achieve op
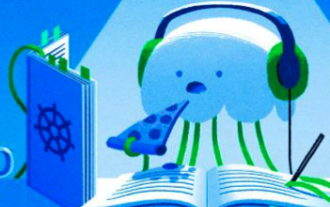
Visual Studio Code, also known as VS Code, is a free source code editor — or integrated development environment (IDE) — available for all major operating systems. With a large collection of extensions for many programming languages, VS Code can be c
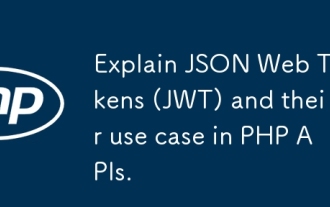
JWT is an open standard based on JSON, used to securely transmit information between parties, mainly for identity authentication and information exchange. 1. JWT consists of three parts: Header, Payload and Signature. 2. The working principle of JWT includes three steps: generating JWT, verifying JWT and parsing Payload. 3. When using JWT for authentication in PHP, JWT can be generated and verified, and user role and permission information can be included in advanced usage. 4. Common errors include signature verification failure, token expiration, and payload oversized. Debugging skills include using debugging tools and logging. 5. Performance optimization and best practices include using appropriate signature algorithms, setting validity periods reasonably,
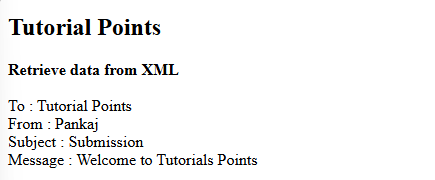
This tutorial demonstrates how to efficiently process XML documents using PHP. XML (eXtensible Markup Language) is a versatile text-based markup language designed for both human readability and machine parsing. It's commonly used for data storage an
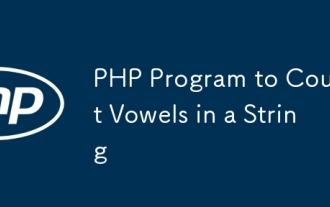
A string is a sequence of characters, including letters, numbers, and symbols. This tutorial will learn how to calculate the number of vowels in a given string in PHP using different methods. The vowels in English are a, e, i, o, u, and they can be uppercase or lowercase. What is a vowel? Vowels are alphabetic characters that represent a specific pronunciation. There are five vowels in English, including uppercase and lowercase: a, e, i, o, u Example 1 Input: String = "Tutorialspoint" Output: 6 explain The vowels in the string "Tutorialspoint" are u, o, i, a, o, i. There are 6 yuan in total
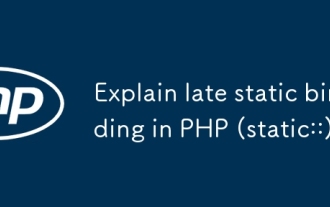
Static binding (static::) implements late static binding (LSB) in PHP, allowing calling classes to be referenced in static contexts rather than defining classes. 1) The parsing process is performed at runtime, 2) Look up the call class in the inheritance relationship, 3) It may bring performance overhead.

What are the magic methods of PHP? PHP's magic methods include: 1.\_\_construct, used to initialize objects; 2.\_\_destruct, used to clean up resources; 3.\_\_call, handle non-existent method calls; 4.\_\_get, implement dynamic attribute access; 5.\_\_set, implement dynamic attribute settings. These methods are automatically called in certain situations, improving code flexibility and efficiency.
