Understanding dynamic loading of Javascript files_javascript skills
Dynamic loading of Javascript files has always been a troublesome thing, such as the common method of uploading over the Internet:
function loadjs(fileurl){ var sct = document.createElement("script"); sct.src = fileurl; document.head.appendChild(sct); }
Then let’s test the results:
<html> <head> <link rel="stylesheet" type="text/css" href="//maxcdn.bootstrapcdn.com/bootstrap/3.3.2/css/bootstrap.min.css" media="screen" /> </head> <body> <script> function loadjs(fileurl){ var sct = document.createElement("script"); sct.src = fileurl; document.head.appendChild(sct); } loadjs("http://code.jquery.com/jquery-1.12.0.js"); loadjs("http://maxcdn.bootstrapcdn.com/bootstrap/3.3.2/js/bootstrap.min.js") loadjs("http://bootboxjs.com/bootbox.js") </script> </body> </html>
After the code is loaded, the following error will appear:
jquery is obviously loaded in the first processing, why is it still reported that jQuery does not exist?
Because loading like this is equivalent to opening three threads, but the jquery file starts the thread first, and the time it takes for jquery to finish executing this thread exceeds the next two times. Therefore, jquery may not be found after it is executed later. this object.
How to deal with this method?
In fact, the loading of files is processed in a state. There is an onload event for file loading, which is an event that can monitor whether the file is loaded.
So we can consider this method to handle the results we want. We handle it in an intuitive way. The improved code is as follows:
<html> <head> <link rel="stylesheet" type="text/css" href="http://maxcdn.bootstrapcdn.com/bootstrap/3.3.2/css/bootstrap.min.css" media="screen" /> </head> <body> <script> function loadjs(fileurl, fn){ var sct = document.createElement("script"); sct.src = fileurl; if(fn){ sct.onload = fn; } document.head.appendChild(sct); } loadjs("http://code.jquery.com/jquery-1.12.0.js",function(){ loadjs("http://maxcdn.bootstrapcdn.com/bootstrap/3.3.2/js/bootstrap.min.js",function(){ loadjs("http://bootboxjs.com/bootbox.js") }) }); </script> </body> </html>
OK, after executing this code, the loaded file will not be loaded until the previous one is loaded, so that the used object will not be found.
Then we will perform the effect of a pop-up box. The Bootbox.js plug-in is used in the code. The loading code is as follows:
loadjs("http://code.jquery.com/jquery-1.12.0.js",function(){ loadjs("http://maxcdn.bootstrapcdn.com/bootstrap/3.3.2/js/bootstrap.min.js",function(){ loadjs("http://bootboxjs.com/bootbox.js",function(){ bootbox.alert("Hello world!", function() { Example.show("Hello world callback"); }); }) }) });
Refresh the page and the pop-up box will be displayed directly:
Dynamically loaded code is often easy to spend a lot of time debugging here. The best way is to write the simplest example and understand the reason. The code here can be encapsulated, and CSS files can also be added to load. .Use as your own plug-in.

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


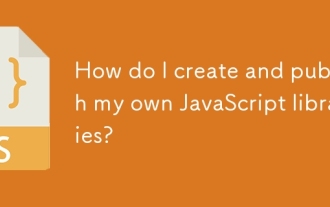
Article discusses creating, publishing, and maintaining JavaScript libraries, focusing on planning, development, testing, documentation, and promotion strategies.
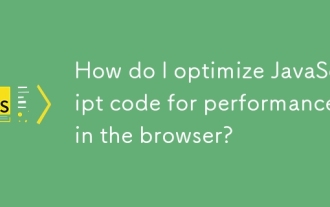
The article discusses strategies for optimizing JavaScript performance in browsers, focusing on reducing execution time and minimizing impact on page load speed.
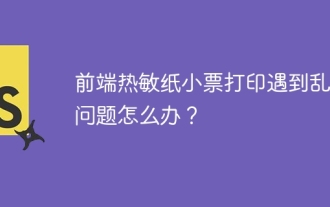
Frequently Asked Questions and Solutions for Front-end Thermal Paper Ticket Printing In Front-end Development, Ticket Printing is a common requirement. However, many developers are implementing...
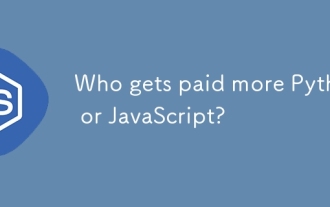
There is no absolute salary for Python and JavaScript developers, depending on skills and industry needs. 1. Python may be paid more in data science and machine learning. 2. JavaScript has great demand in front-end and full-stack development, and its salary is also considerable. 3. Influencing factors include experience, geographical location, company size and specific skills.
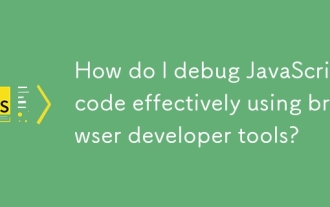
The article discusses effective JavaScript debugging using browser developer tools, focusing on setting breakpoints, using the console, and analyzing performance.
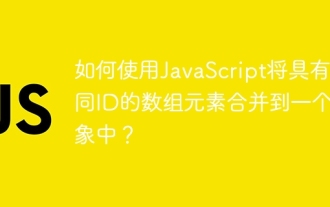
How to merge array elements with the same ID into one object in JavaScript? When processing data, we often encounter the need to have the same ID...
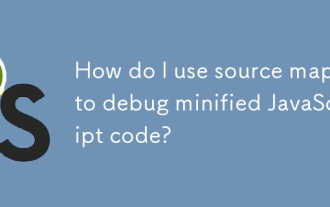
The article explains how to use source maps to debug minified JavaScript by mapping it back to the original code. It discusses enabling source maps, setting breakpoints, and using tools like Chrome DevTools and Webpack.
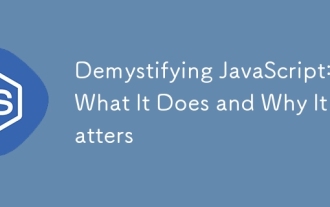
JavaScript is the cornerstone of modern web development, and its main functions include event-driven programming, dynamic content generation and asynchronous programming. 1) Event-driven programming allows web pages to change dynamically according to user operations. 2) Dynamic content generation allows page content to be adjusted according to conditions. 3) Asynchronous programming ensures that the user interface is not blocked. JavaScript is widely used in web interaction, single-page application and server-side development, greatly improving the flexibility of user experience and cross-platform development.
