Detailed explanation of lambda expression usage in python
The examples in this article describe the usage of lambda expressions in python. Share it with everyone for your reference, the details are as follows:
Here I will introduce to you the lambda function.
The lambda function is a minimal function that quickly defines a single line. It is borrowed from Lisp and can be used wherever a function is needed. The following example compares the traditional function definition def and lambda definition methods:
>>> def f ( x ,y): ... return x * y ... >>> f ( 2,3 ) 6 >>> g = lambda x ,y: x * y >>> g ( 2,3 ) 6
As you can see, the results obtained by the two functions are the same. For functions that implement simple functions, it is more streamlined and flexible to use lambda functions to define them. You can also directly assign the function to a variable and use the variable name to represent the function. name.
In fact, lambda functions do not need to be assigned to a variable in many cases.
There are some things to note when using lambda functions: The lambda function can receive any number of parameters (including optional parameters) and return the value of a single expression. The lambda function cannot contain commands and cannot contain more than one expression .
The following is a brief demonstration of how to use the lambda function to implement custom sorting.
class People : age = 0 gender = 'male' def __init__ ( self , age , gender ): self . age = age self . gender = gender def toString ( self ): return 'Age:' + str ( self . age ) + ' /t Gender:' + self . gender List = [ People ( 21 , 'male' ), People ( 20 , 'famale' ), People ( 34 , 'male' ), People ( 19 , 'famale' )] print 'Befor sort:' for p in List : print p . toString () List . sort ( lambda p1 , p2 : cmp ( p1 . age , p2 . age )) print ' /n After ascending sort:' for p in List : print p . toString () List . sort ( lambda p1 , p2 : - cmp ( p1 . age , p2 . age )) print ' /n After descending sort:' for p in List : print p . toString ()
The above code defines a People class, and uses the lambda function to sort the list containing People class objects in ascending and descending order according to People's age. The running results are as follows:
Befor sort:
Age:21 Gender:male
Age:20 Gender:famale
Age:34 Gender:male
Age:19 Gender:famale
After ascending sort:
Age:19 Gender:famale
Age:20 Gender:famale
Age:21 Gender:male
Age:34 Gender:male
After descending sort:
Age:34 Gender:male
Age:21 Gender:male
Age:20 Gender:famale
Age:19 Gender:famale
Lambda statements are used to create new function objects and return them at runtime.
Example: Using lambda form
#!/usr/bin/python # Filename: lambda.py def make_repeater(n): return lambda s: s*n twice = make_repeater(2) print twice('word') print twice(5)
Output:
$ python lambda.py wordword 10
How it works
Here, we use the make_repeater function to create a new function object at runtime and return it.
The lambda statement is used to create function objects. Essentially, a lambda takes a parameter, followed by a single expression as the function body, and the value of the expression is returned by the newly created function. Note that even print statements cannot be used in lambda form, only expressions can be used.
The difference between def and lambda
The main difference between them is that python def is a statement and python lambda is an expression. Understanding this is important for you to understand them. Take a look at their applications below.
First of all, statements can be nested in python. For example, if you need to define a method based on a certain condition, you can only use def.
You will get an error if you use lambda.
a = 2 if a > 1 : def info (): print 'haha' else : def test (): print 'test'
Sometimes when you need to operate in python expressions, you need to use expression nesting. In this case, python def cannot get the results you want, so you can only use python lambda
Here’s an example:
g = lambda x : x+2 info = [g(x) for x in range(10)] print info
Through the above examples, I hope you can have a good understanding of the similarities and differences between python def and lambda. If you are interested in python functions, you can take a look: python function return value, python function parameters
Python lambda uses lambda in python to create anonymous functions, and methods created with def have names. In addition to the superficial method names being different, what else is different about python lambda from def?
① python lambda will create a function object, but will not assign the function object to an identifier, while def will assign the function object to a variable.
② python lambda is just an expression, while def is a statement.
The following is the format of python lambda, it looks so streamlined.
lambda x: print x
If you use python lambda in python list analysis, I feel that it is not very meaningful, because python lambda will create a function object, but then discard it immediately because you do not use its return value, that is, function object. It is precisely because lambda is just an expression, it can be directly used as a member of a python list or a python dictionary, such as:
info = [lamba a: a**3, lambda b: b**3]
There is no way to directly replace it with a def statement in this place. Because def is a statement, not an expression, which cannot be nested inside. A lambda expression can only have one expression after ":". That is to say, in def, things that can be returned with return can also be placed after lambda, and things that cannot be returned with return cannot be defined after python lambda. Therefore, statements like if or for or print cannot be used in lambdas. Lambdas are generally only used to define simple functions.
Here are some examples of python lambda
① Single parameter:
g = lambda x:x*2 print g(3)
The result is 6
② Multiple parameters:
m = lambda x,y,z: (x-y)*z print m(3,1,2)
The result is 4
When you have nothing to do when writing programs, you can become proficient by using python lambda. .
Readers who are interested in more Python-related content can check out the special topics on this site: "Summary of Python Image Operation Skills", "Python Data Structure and Algorithm Tutorial", "Python Socket Programming Skills Summary", "Python Function Using Skills Summary" ", "Python String Operation Skills Summary", "Python Introduction and Advanced Classic Tutorial" and "Python File and Directory Operation Skills Summary"
I hope this article will be helpful to everyone in Python programming.

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics



HadiDB: A lightweight, high-level scalable Python database HadiDB (hadidb) is a lightweight database written in Python, with a high level of scalability. Install HadiDB using pip installation: pipinstallhadidb User Management Create user: createuser() method to create a new user. The authentication() method authenticates the user's identity. fromhadidb.operationimportuseruser_obj=user("admin","admin")user_obj.
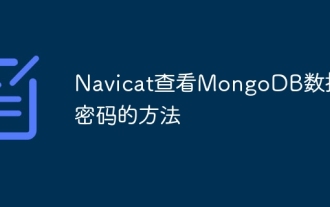
It is impossible to view MongoDB password directly through Navicat because it is stored as hash values. How to retrieve lost passwords: 1. Reset passwords; 2. Check configuration files (may contain hash values); 3. Check codes (may hardcode passwords).
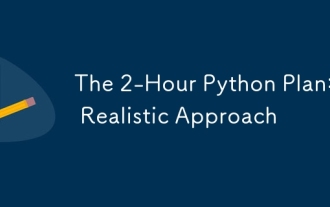
You can learn basic programming concepts and skills of Python within 2 hours. 1. Learn variables and data types, 2. Master control flow (conditional statements and loops), 3. Understand the definition and use of functions, 4. Quickly get started with Python programming through simple examples and code snippets.
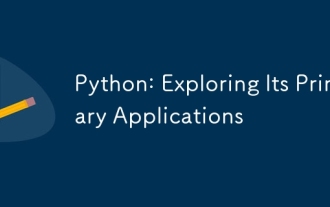
Python is widely used in the fields of web development, data science, machine learning, automation and scripting. 1) In web development, Django and Flask frameworks simplify the development process. 2) In the fields of data science and machine learning, NumPy, Pandas, Scikit-learn and TensorFlow libraries provide strong support. 3) In terms of automation and scripting, Python is suitable for tasks such as automated testing and system management.
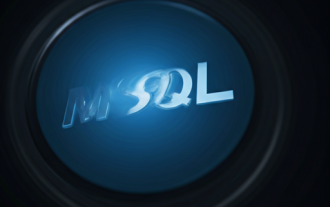
MySQL database performance optimization guide In resource-intensive applications, MySQL database plays a crucial role and is responsible for managing massive transactions. However, as the scale of application expands, database performance bottlenecks often become a constraint. This article will explore a series of effective MySQL performance optimization strategies to ensure that your application remains efficient and responsive under high loads. We will combine actual cases to explain in-depth key technologies such as indexing, query optimization, database design and caching. 1. Database architecture design and optimized database architecture is the cornerstone of MySQL performance optimization. Here are some core principles: Selecting the right data type and selecting the smallest data type that meets the needs can not only save storage space, but also improve data processing speed.
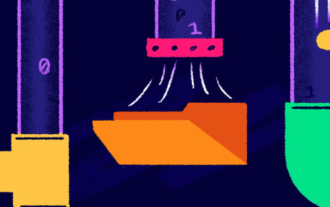
As a data professional, you need to process large amounts of data from various sources. This can pose challenges to data management and analysis. Fortunately, two AWS services can help: AWS Glue and Amazon Athena.
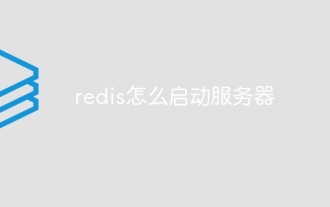
The steps to start a Redis server include: Install Redis according to the operating system. Start the Redis service via redis-server (Linux/macOS) or redis-server.exe (Windows). Use the redis-cli ping (Linux/macOS) or redis-cli.exe ping (Windows) command to check the service status. Use a Redis client, such as redis-cli, Python, or Node.js, to access the server.
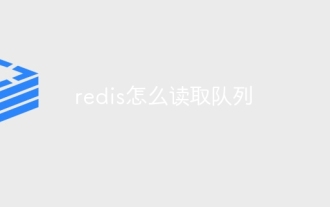
To read a queue from Redis, you need to get the queue name, read the elements using the LPOP command, and process the empty queue. The specific steps are as follows: Get the queue name: name it with the prefix of "queue:" such as "queue:my-queue". Use the LPOP command: Eject the element from the head of the queue and return its value, such as LPOP queue:my-queue. Processing empty queues: If the queue is empty, LPOP returns nil, and you can check whether the queue exists before reading the element.
