


I wrote a red envelope generation algorithm myself. It can be generated correctly, but it cannot be recursively generated?
Because you want to generate random red envelopes, you need to set the amount, minimum value, maximum value, and quantity of the red envelopes to be distributed.
I tried using a for loop, but it got stuck here in do while, so I wrote one using recursion. The correct red envelope can be generated in the memory, but it cannot be recursed. Please help me to find out where the problem lies?
<code><?php //调试函数 function p($arr) { echo "<pre class="brush:php;toolbar:false">" . print_r($arr, true) . ""; } //发红包函数 function hongbao($money, $min, $max, $num, $arr = array(), $first = 'yes') { if ($first == 'yes') { //由于红包是以分为单位所以先转换单位,1元=100分,但只需转换一次 $money = $money * 100; $min = $min * 100; $max = $max * 100; //红包发放最大最小值合法性检测,防止发送死循环 if ($money - $min * $num < 0) { return "你发放红包的金额太小不足以 发给这么多人"; } else { if ($money - $max * $num > 0) { return "你发放红包的金额太大 这些人领不完"; } } } //计算已生成的红包总额 $safe_total = array_sum($arr); //如果红包总额 大于或等于 要发放的总额, 就说明红包已经生成完毕 if ($safe_total >= $money) { //防止出现 最后出现的数据都相同,所以让这里随机排序一下 shuffle($arr); /*这里有异常, 可以打印出来正确的值 却无法return到最外面吧,应该是递归上的问题*/ p($arr); return $arr; } else { //随机生成红包金额 $rand = mt_rand($min, $max); //用当前的钱 减去已发的钱 -减去本次要发的钱 $zx = $money - $safe_total - $rand - ($num - 1) * $min; $zd = $money - $safe_total - $rand - ($num - 1) * $max; if ($zx >= 0 && $zd <= 0) { //说明条件可以执行 $num--; $arr[] = $rand; $xrr = $arr; hongbao($money, $min, $max, $num, $arr, 'no'); } else { //说明发完本次红包就没法继续下次了 所以不做修改。 hongbao($money, $min, $max, $num, $arr, 'no'); } } } //发80块钱的红包, 最小1.3元 最大2, 数量为50个 $myarr = hongbao(80, 1.3, 2, 50); //打印下 myarr 看下赋值是否正确 , 函数里已经打印过一次, 所以要出现打印两次 数组才是正常 p($myarr);
Reply content:
Because you want to generate random red envelopes, you need to set the amount, minimum value, maximum value, and quantity of the red envelopes to be distributed.
I tried using a for loop, but it got stuck here in do while, so I wrote one using recursion. The correct red envelope can be generated in the memory, but it cannot be recursed. Please help me to find out where the problem lies?
<code><?php //调试函数 function p($arr) { echo "<pre class="brush:php;toolbar:false">" . print_r($arr, true) . ""; } //发红包函数 function hongbao($money, $min, $max, $num, $arr = array(), $first = 'yes') { if ($first == 'yes') { //由于红包是以分为单位所以先转换单位,1元=100分,但只需转换一次 $money = $money * 100; $min = $min * 100; $max = $max * 100; //红包发放最大最小值合法性检测,防止发送死循环 if ($money - $min * $num < 0) { return "你发放红包的金额太小不足以 发给这么多人"; } else { if ($money - $max * $num > 0) { return "你发放红包的金额太大 这些人领不完"; } } } //计算已生成的红包总额 $safe_total = array_sum($arr); //如果红包总额 大于或等于 要发放的总额, 就说明红包已经生成完毕 if ($safe_total >= $money) { //防止出现 最后出现的数据都相同,所以让这里随机排序一下 shuffle($arr); /*这里有异常, 可以打印出来正确的值 却无法return到最外面吧,应该是递归上的问题*/ p($arr); return $arr; } else { //随机生成红包金额 $rand = mt_rand($min, $max); //用当前的钱 减去已发的钱 -减去本次要发的钱 $zx = $money - $safe_total - $rand - ($num - 1) * $min; $zd = $money - $safe_total - $rand - ($num - 1) * $max; if ($zx >= 0 && $zd <= 0) { //说明条件可以执行 $num--; $arr[] = $rand; $xrr = $arr; hongbao($money, $min, $max, $num, $arr, 'no'); } else { //说明发完本次红包就没法继续下次了 所以不做修改。 hongbao($money, $min, $max, $num, $arr, 'no'); } } } //发80块钱的红包, 最小1.3元 最大2, 数量为50个 $myarr = hongbao(80, 1.3, 2, 50); //打印下 myarr 看下赋值是否正确 , 函数里已经打印过一次, 所以要出现打印两次 数组才是正常 p($myarr);
I changed it according to what you gave:
<?php //调试函数 function p($arr) { echo "<pre class="brush:php;toolbar:false">" . print_r($arr, true) . ""; } //发红包函数 function luckymoney($money, $min, $max, $num, $arr = array(), $first = 'yes') { if ($first == 'yes') { //由于红包是以分为单位所以先转换单位,1元=100分,但只需转换一次 $money = $money * 100; $min = $min * 100; $max = $max * 100; //红包发放最大最小值合法性检测,防止发送死循环 if ($money - $min * $num < 0) { return "你发放红包的金额太小不足以 发给这么多人"; } else { if ($money - $max * $num > 0) { return "你发放红包的金额太大 这些人领不完"; } } } //计算已生成的红包总额 $safe_total = array_sum($arr); //如果红包总额 大于或等于 要发放的总额, 就说明红包已经生成完毕 if ($num == 1) { // if ($safe_total >= $money) { //防止出现 最后出现的数据都相同,所以让这里随机排序一下 $arr[] = $money - $safe_total; shuffle($arr); /*这里有异常, 可以打印出来正确的值 却无法return到最外面吧,应该是递归上的问题*/ // p($arr); return $arr; } else { //随机生成红包金额 $rand = mt_rand($min, $max); //用当前的钱 减去已发的钱 -减去本次要发的钱 $zx = $money - $safe_total - $rand - ($num - 1) * $min; $zd = $money - $safe_total - $rand - ($num - 1) * $max; if ($zx >= 0 && $zd <= 0) { //说明条件可以执行 $num--; $arr[] = $rand; // $xrr = $arr; return luckymoney($money, $min, $max, $num, $arr, 'no'); } else { //说明发完本次红包就没法继续下次了 所以不做修改。 // luckymoney($money, $min, $max, $num, $arr, 'no'); return luckymoney($money, $min, $max, $num, $arr, 'no'); // p($arr); // $arr[] = $rand; // shuffle($arr); // return $arr; } } } //发80块钱的红包, 最小1.3元 最大2, 数量为50个 //$myarr = luckymoney(5, 1.3, 2, 3); $myarr = luckymoney(80, 1.3, 2, 50); //打印下 myarr 看下赋值是否正确 , 函数里已经打印过一次, 所以要出现打印两次 数组才是正常 p($myarr); $array_sum = array_sum($myarr); p($array_sum);
Note that since you are using recursion, and it is random based on luck, it is easy for stack overflow,
I tested your data luckymoney(80, 1.3, 2, 50) and it succeeded about 2 times out of 5 times. I suggest you change the algorithm...
return hongbao($money, $min, $max, $num, $arr, 'no');
Thank you everyone, I reused the for loop to improve the algorithm.
Calculated based on sending 200 yuan, minimum 1.3, maximum 3, 200 red envelopes are sent
Using the for loop algorithm, the function is executed 100 times, and the average execution time is 0.004 seconds each time
Using the recursive algorithm, the function is executed 100 times, and the average execution time is 0.211 seconds
The efficiency difference is 52.75 times. Above code. Thank you @aristotll for your suggestion
The following is the for loop code. If you have a better idea, please guide me, thank you
<code><?php //调试函数 function p($arr) { echo "<pre class="brush:php;toolbar:false">" . print_r($arr, true) . ""; } //发红包函数 function hongbao($money, $min, $max, $num) { $arr = array(); //由于红包是以分为单位所以先转换单位,1元=100分,但只需转换一次 $money = $money * 100; $min = $min * 100; $max = $max * 100; //红包发放最大最小值合法性检测,防止发送死循环 if ($money - $min * $num < 0) { return "你发放红包的金额太小不足以 发给这么多人"; } else { if ($money - $max * $num > 0) { return "你发放红包的金额太大 这些人领不完"; } } $tempnum = $num; for ($i = 0; $i < $tempnum; $i++) { $flag = 'no'; do { //随机生成一个红包 $rand = mt_rand($min, $max); $anum = count($arr); $zx = $money - array_sum($arr) - $rand - ($num - 1) * $min; $zd = $money - array_sum($arr) - $rand - ($num - 1) * $max; $all = array_sum($arr); if ($zx >= 0 && $zd <= 0) { $arr[] = $rand; $flag = 'yes'; $num--; } } while ($flag == 'no'); } shuffle($arr); return $arr; } $myarr = hongbao(200, 1, 3, 100); p($myarr); echo array_sum($myarr)/100;

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
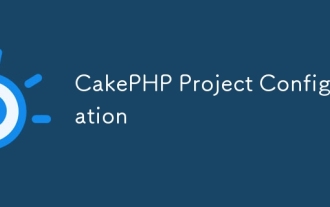
In this chapter, we will understand the Environment Variables, General Configuration, Database Configuration and Email Configuration in CakePHP.
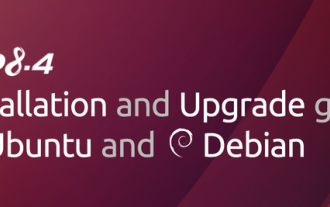
PHP 8.4 brings several new features, security improvements, and performance improvements with healthy amounts of feature deprecations and removals. This guide explains how to install PHP 8.4 or upgrade to PHP 8.4 on Ubuntu, Debian, or their derivati
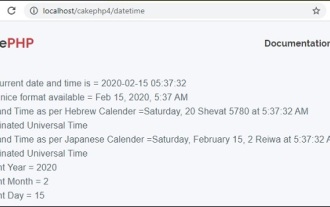
To work with date and time in cakephp4, we are going to make use of the available FrozenTime class.
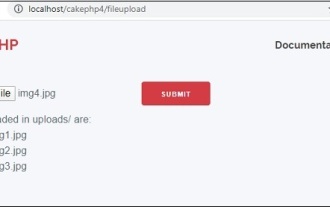
To work on file upload we are going to use the form helper. Here, is an example for file upload.
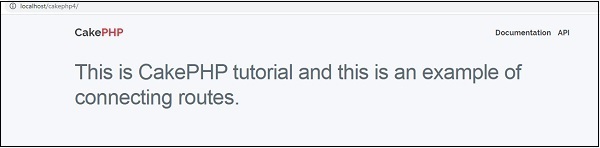
In this chapter, we are going to learn the following topics related to routing ?
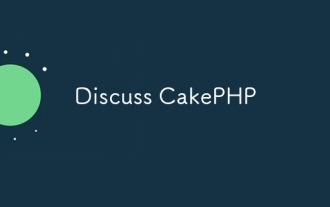
CakePHP is an open-source framework for PHP. It is intended to make developing, deploying and maintaining applications much easier. CakePHP is based on a MVC-like architecture that is both powerful and easy to grasp. Models, Views, and Controllers gu
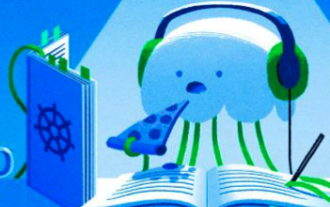
Visual Studio Code, also known as VS Code, is a free source code editor — or integrated development environment (IDE) — available for all major operating systems. With a large collection of extensions for many programming languages, VS Code can be c
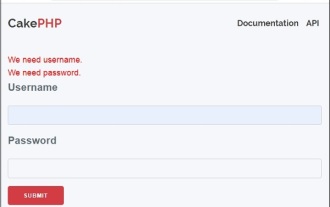
Validator can be created by adding the following two lines in the controller.
