Image verification code file sharing written in PHP,
Share picture verification code class files written in PHP,
Suitable for customized verification code class!
<?php /* * To change this license header, choose License Headers in Project Properties. * To change this template file, choose Tools | Templates * and open the template in the editor. */ Class Image{ private $img; public $width = 85; public $height = 25; public $code; public $code_len = 4; public $code_str = "329832983DSDSKDSLKQWEWQ2lkfDSFSDjfdsfdsjwlkfj93290KFDSKJFDSOIDSLK"; public $bg_color = '#DCDCDC'; public $font_size = 16; public $font = 'font.ttf'; public $font_color = '#000000'; //创建验证码饿字符创 public function create_code(){ $code = ''; for( $i=0;$i<$this->code_len;$i++ ){ $code .= $this->code_str[mt_rand(0, strlen($this->code_str)-1)]; } return $this->code = $code; } //输出图像 public function getImage(){ $w = $this->width; $h = $this->height; $bg_color = $this->bg_color; $img = imagecreatetruecolor($w, $h); $bg_color = imagecolorallocate($img, hexdec(substr($bg_color, 1,2)), hexdec(substr($bg_color, 3,2)), hexdec(substr($bg_color, 5,2))); imagefill($img, 0, 0, $bg_color); $this->img = $img; $this->create_font(); $this->create_pix(); $this->show_code(); } //写入验证码 public function create_font(){ $this->create_code(); $color = $this->font_color; $font_color = imagecolorallocate($this->img, hexdec(substr($color,1,2)), hexdec(substr($color, 3,2)), hexdec(substr($color,5,2))); $x = $this->width/$this->code_len; for( $i=0;$i<$this->code_len;$i++ ){ $txt_color = imagecolorallocate($this->img, mt_rand(0,100), mt_rand(0, 150), mt_rand(0, 200)); imagettftext($this->img, $this->font_size, mt_rand(-30, 30), $x*$i+mt_rand(3, 6), mt_rand($this->height/1.2, $this->height), $txt_color, $this->font , $this->code[$i]); //imagestring($this->img, $this->font_size, $x*$i+mt_rand(3, 6),mt_rand(0, $this->height/4) , $this->code[$i], $font_color); } $this->font_color = $font_color; } //画干扰线 public function create_pix(){ $pix_color= $this->font_color; for($i=0;$i<100;$i++){ imagesetpixel($this->img, mt_rand(0, $this->width),mt_rand(0, $this->height), $pix_color); } for($j=0;$j<4;$j++){ imagesetthickness($this->img, mt_rand(1, 2)); imageline($this->img, mt_rand(0, $this->width), mt_rand(0, $this->height), mt_rand(0, $this->width), mt_rand(0, $this->height), $pix_color); } } //得到验证码 public function getCode(){ return strtoupper($this->code); } //输出验证码 private function show_code(){ header("Content-type:image/png"); imagepng($this->img); imagedestroy($this->img); } }
Let’s look at another example code:
Generate an image type verification code. The verification code contains numbers and capital letters. The md5 encrypted verification code is stored in the session
<?php /** * 图片验证码类 * 生成图片类型验证码,验证码包含数字和大写字母,session中存放md5加密后的验证码 * * 使用方法: * $captcha = new Catpcha(); * $captcha->buildAndExportImage(); * * 作 者: luojing * 创建时间: 2013-3-27 上午11:42:12 */ class Captcha { private $width;//宽度 private $height; //高度 private $codeNum;//验证码字符数量 private $image;//验证码图像资源 private $sessionKey;//session中保存的名字 private $captcha;//验证码字符串 const charWidth = 10;//单个字符宽度,根据输出字符大小而变 /** * 创建验证码类,初始化相关参数 * @param $width 图片宽度 * @param $height 图片高度 * @param $codeNum 验证码字符数量 * @param $sessionKey session中保存的名字 */ function __construct($width = 50, $height = 20, $codeNum = 4, $sessionKey = 'captcha') { $this->width = $width; $this->height = $height; $this->codeNum = $codeNum; $this->sessionKey = $sessionKey; //保证最小高度和宽度 if($height < 20) { $this->height = 20; } if($width < ($codeNum * self::charWidth + 10)) {//左右各保留5像素空隙 $this->width = $codeNum * self::charWidth + 10; } } /** * 构造并输出验证码图片 */ public function buildAndExportImage() { $this->createImage(); $this->setDisturb(); $this->setCaptcha(); $this->exportImage(); } /** * 构造图像,设置底色 */ private function createImage() { //创建图像 $this->image = imagecreatetruecolor($this->width, $this->height); //创建背景色 $bg = imagecolorallocate($this->image, mt_rand(220, 255), mt_rand(220, 255), mt_rand(220, 255)); //填充背景色 imagefilledrectangle($this->image, 0, 0, $this->width - 1, $this->height - 1, $bg); } /** * 设置干扰元素 */ private function setDisturb() { //设置干扰点 for($i = 0; $i < 150; $i++) { $color = imagecolorallocate($this->image, mt_rand(150, 200), mt_rand(150, 200), mt_rand(150, 200)); imagesetpixel($this->image, mt_rand(5, $this->width - 10), mt_rand(5, $this->height - 3), $color); } //设置干扰线 for($i = 0; $i < 10; $i++) { $color = imagecolorallocate($this->image, mt_rand(150, 220), mt_rand(150, 220), mt_rand(150, 220)); imagearc($this->image, mt_rand(-10, $this->width), mt_rand(-10, $this->height), mt_rand(30, 300), mt_rand(20, 200), 55, 44, $color); } //创建边框色 $border = imagecolorallocate($this->image, mt_rand(0, 50), mt_rand(0, 50), mt_rand(0, 50)); //画边框 imagerectangle($this->image, 0, 0, $this->width - 1, $this->height - 1, $border); } /** * 产生并绘制验证码 */ private function setCaptcha() { $str = '23456789ABCDEFGHJKLMNPQRSTUVWXYZ'; //生成验证码字符 for($i = 0; $i < $this->codeNum; $i++) { $this->captcha .= $str{mt_rand(0, strlen($str) - 1)}; } //绘制验证码 for($i = 0; $i < strlen($this->captcha); $i++) { $color = imagecolorallocate($this->image, mt_rand(0, 200), mt_rand(0, 200), mt_rand(0, 200)); $x = floor(($this->width - 10)/$this->codeNum); $x = $x*$i + floor(($x-self::charWidth)/2) + 5; $y = mt_rand(2, $this->height - 20); imagechar($this->image, 5, $x, $y, $this->captcha{$i}, $color); } } /* * 输出图像,验证码保存到session中 */ private function exportImage() { if(imagetypes() & IMG_GIF){ header('Content-type:image/gif'); imagegif($this->image); } else if(imagetypes() & IMG_PNG){ header('Content-type:image/png'); imagepng($this->iamge); } else if(imagetypes() & IMG_JPEG) { header('Content-type:image/jpeg'); imagepng($this->iamge); } else { imagedestroy($this->image); die("Don't support image type!"); } //将验证码信息保存到session中,md5加密 if(!isset($_SESSION)){ session_start(); } $_SESSION[$this->sessionKey] = md5($this->captcha); imagedestroy($this->image); } function __destruct() { unset($this->width, $this->height, $this->codeNum,$this->captcha); } }
Example 3:
<?php class ValidationCode { private $width; private $height; private $codeNum; private $image; //图像资源 private $disturbColorNum; private $checkCode; function __construct($width=80, $height=20, $codeNum=4){ $this->width=$width; $this->height=$height; $this->codeNum=$codeNum; $this->checkCode=$this->createCheckCode(); $number=floor($width*$height/15); if($number > 240-$codeNum){ $this->disturbColorNum= 240-$codeNum; }else{ $this->disturbColorNum=$number; } } //通过访问该方法向浏览器中输出图像 function showImage($fontFace=""){ //第一步:创建图像背景 $this->createImage(); //第二步:设置干扰元素 $this->setDisturbColor(); //第三步:向图像中随机画出文本 $this->outputText($fontFace); //第四步:输出图像 $this->outputImage(); } //通过调用该方法获取随机创建的验证码字符串 function getCheckCode(){ return $this->checkCode; } private function createImage(){ //创建图像资源 $this->image=imagecreatetruecolor($this->width, $this->height); //随机背景色 $backColor=imagecolorallocate($this->image, rand(225, 255), rand(225,255), rand(225, 255)); //为背景添充颜色 imagefill($this->image, 0, 0, $backColor); //设置边框颜色 $border=imagecolorallocate($this->image, 0, 0, 0); //画出矩形边框 imagerectangle($this->image, 0, 0, $this->width-1, $this->height-1, $border); } private function setDisturbColor(){ for($i=0; $i<$this->disturbColorNum; $i++){ $color=imagecolorallocate($this->image, rand(0, 255), rand(0, 255), rand(0, 255)); imagesetpixel($this->image, rand(1, $this->width-2), rand(1, $this->height-2), $color); } for($i=0; $i<10; $i++){ $color=imagecolorallocate($this->image, rand(200, 255), rand(200, 255), rand(200, 255)); imagearc($this->image, rand(-10, $this->width), rand(-10, $this->height), rand(30, 300), rand(20, 200), 55, 44, $color); } } private function createCheckCode(){ //这里主要产生随机码,从2开始是为了区分1和l $code="23456789abcdefghijkmnpqrstuvwxyzABCDEFGHIJKMNPQRSTUVWXYZ"; $string=''; for($i=0; $i < $this->codeNum; $i++){ $char=$code{rand(0, strlen($code)-1)}; $string.=$char; } return $string; } private function outputText($fontFace=""){ for($i=0; $i<$this->codeNum; $i++){ $fontcolor=imagecolorallocate($this->image, rand(0, 128), rand(0, 128), rand(0, 128)); if($fontFace==""){ $fontsize=rand(3, 5); $x=floor($this->width/$this->codeNum)*$i+3; $y=rand(0, $this->height-15); imagechar($this->image,$fontsize, $x, $y, $this->checkCode{$i},$fontcolor); }else{ $fontsize=rand(12, 16); $x=floor(($this->width-8)/$this->codeNum)*$i+8; $y=rand($fontSize+5, $this->height); imagettftext($this->image,$fontsize,rand(-30, 30),$x,$y ,$fontcolor, $fontFace, $this->checkCode{$i}); } } } private function outputImage() { if(imagetypes() & IMG_GIF){ header("Content-Type:image/gif"); imagepng($this->image); }else if(imagetypes() & IMG_JPG){ header("Content-Type:image/jpeg"); imagepng($this->image); }else if(imagetypes() & IMG_PNG){ header("Content-Type:image/png"); imagepng($this->image); }else if(imagetypes() & IMG_WBMP){ header("Content-Type:image/vnd.wap.wbmp"); imagepng($this->image); }else{ die("PHP不支持图像创建"); } } function __destruct(){ imagedestroy($this->image); } }
Use as follows:
Test, call the verification code class
code.php
<?php session_start(); include "validationcode.class.php"; $code=new ValidationCode(80, 20, 4); $code->showImage(); //输出到页面中供 注册或登录使用 $_SESSION["code"]=$code->getCheckCode(); //将验证码保存到服务器中

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
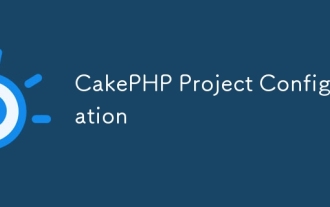
In this chapter, we will understand the Environment Variables, General Configuration, Database Configuration and Email Configuration in CakePHP.
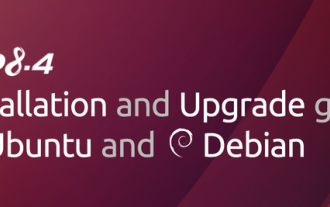
PHP 8.4 brings several new features, security improvements, and performance improvements with healthy amounts of feature deprecations and removals. This guide explains how to install PHP 8.4 or upgrade to PHP 8.4 on Ubuntu, Debian, or their derivati
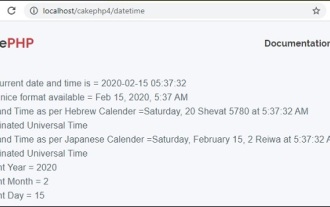
To work with date and time in cakephp4, we are going to make use of the available FrozenTime class.
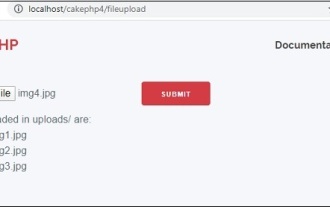
To work on file upload we are going to use the form helper. Here, is an example for file upload.
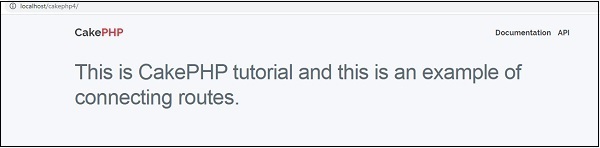
In this chapter, we are going to learn the following topics related to routing ?
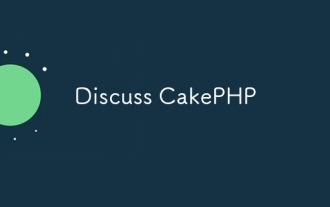
CakePHP is an open-source framework for PHP. It is intended to make developing, deploying and maintaining applications much easier. CakePHP is based on a MVC-like architecture that is both powerful and easy to grasp. Models, Views, and Controllers gu
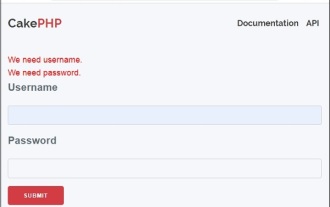
Validator can be created by adding the following two lines in the controller.
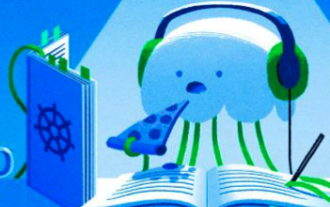
Visual Studio Code, also known as VS Code, is a free source code editor — or integrated development environment (IDE) — available for all major operating systems. With a large collection of extensions for many programming languages, VS Code can be c
