Error handling and exception handling mechanism in PHP
Error handling is an important part when writing PHP programs. If your program lacks error detection code, it looks unprofessional and opens the door to security risks
Error reporting level (just understand it)
These error reporting levels are different types of errors that error handlers are designed to handle:
Value | Constant | Description | ||||||||||||||||||||||||
---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|
2 | E_WARNING | Nonfatal run-time error. Do not pause script execution. | ||||||||||||||||||||||||
8 | E_NOTICE |
|
||||||||||||||||||||||||
256 | E_USER_ERROR | Fatal user-generated error. This is similar to E_ERROR set by the programmer using the PHP function trigger_error(). | ||||||||||||||||||||||||
512 | E_USER_WARNING | Non-fatal user-generated warning. This is similar to the E_WARNING set by the programmer using the PHP function trigger_error(). | ||||||||||||||||||||||||
1024 | E_USER_NOTICE | User-generated notifications. This is similar to E_NOTICE set by the programmer using the PHP function trigger_error(). | ||||||||||||||||||||||||
4096 | E_RECOVERABLE_ERROR | Catchable fatal error. Like E_ERROR, but can be caught by a user-defined handler. (see set_error_handler()) | ||||||||||||||||||||||||
8191 | E_ALL | All errors and warnings except level E_STRICT. (In PHP 6.0, E_STRICT is part of E_ALL) |
Requirement: For example, if you want to receive an age, if the number is greater than 120, it is considered an errorTraditional method:
if($age>120){
echo 'Wrong age';exit();
}
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 |
|
- Try - 使用异常的函数应该位于 "try" 代码块内。如果没有触发异常,则代码将照常继续执行。但是如果异常被触发,会抛出一个异常。
- Throw - 这里规定如何触发异常。每一个 "throw" 必须对应至少一个 "catch"
- Catch - "catch" 代码块会捕获异常,并创建一个包含异常信息的对象
让我们触发一个异常:
1 |
|
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 |
|
1 |
|
1 |
|
上面代码将获得类似这样一个错误:
1 |
|
例子解释:
上面的代码抛出了一个异常,并捕获了它:
- 创建 checkNum() 函数。它检测数字是否大于 1。如果是,则抛出一个异常。
- 在 "try" 代码块中调用 checkNum() 函数。
- checkNum() 函数中的异常被抛出
- "catch" 代码块接收到该异常,并创建一个包含异常信息的对象 ($e)。
- 通过从这个 exception 对象调用 $e->getMessage(),输出来自该异常的错误消息
不过,为了遵循“每个 throw 必须对应一个 catch”的原则,可以设置一个顶层的异常处理器来处理漏掉的错误。
set_exception_handler()函数可设置处理所有未捕获异常的用户定义函数
//设置一个顶级异常处理器
function myexception($e){
echo 'this is top exception';
} //修改默认的异常处理器
set_exception_handler("myexception");
try{
$i=5;
if($i
throw new exception('$i must greater than 10');
}
}catch(Exception $e){
//处理异常
echo $e->getMessage().'
';
//不处理异常,继续抛出
throw new exception('errorinfo'); //也可以用throw $e 保留原错误信息;
}
创建一个自定义的异常类
class customException extends Exception{
public function errorMessage(){
//error message $errorMsg = 'Error on line '.$this->getLine().' in '.$this->getFile().': '.$this->getMessage().' is not a valid E-Mail address'; return $errorMsg;
}
}
//Use
try{
Throw new customException('error message');
}catch(customException $e){
echo $e->errorMsg();
}
You can use multiple catches to return error messages under different circumstances
try{
$i=5;
if($i>0){
Throw new customException('error message');//Use a custom exception class to handle
} if($i
Throw new exception('error2');//Use system default exception handling
}
}catch(customException $e){
echo $e->getMessage();
}catch(Exception $e1){
echo $e1->getMessage();
}
Abnormal rules
- Code that requires exception handling should be placed within a try code block to catch potential exceptions.
- Each try or throw block must have at least one corresponding catch block.
- Use multiple catch code blocks to catch different kinds of exceptions.
- Exceptions can be re-thrown in the catch block within the try code.
In short: if an exception is thrown, you must catch it.

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


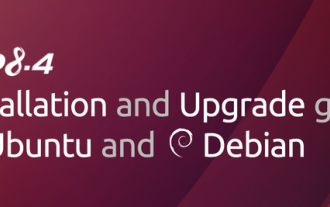
PHP 8.4 brings several new features, security improvements, and performance improvements with healthy amounts of feature deprecations and removals. This guide explains how to install PHP 8.4 or upgrade to PHP 8.4 on Ubuntu, Debian, or their derivati
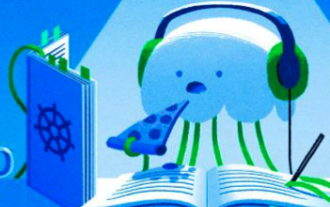
Visual Studio Code, also known as VS Code, is a free source code editor — or integrated development environment (IDE) — available for all major operating systems. With a large collection of extensions for many programming languages, VS Code can be c
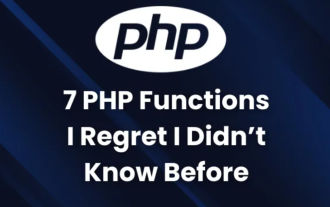
If you are an experienced PHP developer, you might have the feeling that you’ve been there and done that already.You have developed a significant number of applications, debugged millions of lines of code, and tweaked a bunch of scripts to achieve op
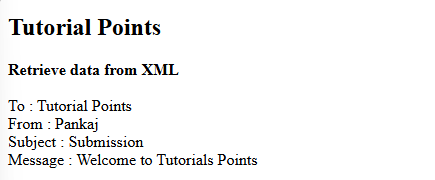
This tutorial demonstrates how to efficiently process XML documents using PHP. XML (eXtensible Markup Language) is a versatile text-based markup language designed for both human readability and machine parsing. It's commonly used for data storage an
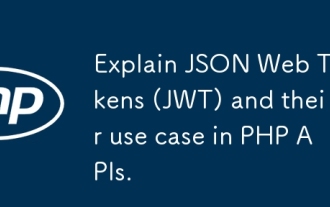
JWT is an open standard based on JSON, used to securely transmit information between parties, mainly for identity authentication and information exchange. 1. JWT consists of three parts: Header, Payload and Signature. 2. The working principle of JWT includes three steps: generating JWT, verifying JWT and parsing Payload. 3. When using JWT for authentication in PHP, JWT can be generated and verified, and user role and permission information can be included in advanced usage. 4. Common errors include signature verification failure, token expiration, and payload oversized. Debugging skills include using debugging tools and logging. 5. Performance optimization and best practices include using appropriate signature algorithms, setting validity periods reasonably,
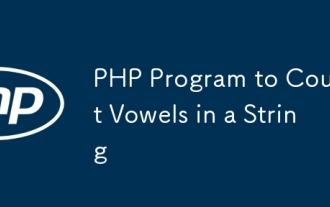
A string is a sequence of characters, including letters, numbers, and symbols. This tutorial will learn how to calculate the number of vowels in a given string in PHP using different methods. The vowels in English are a, e, i, o, u, and they can be uppercase or lowercase. What is a vowel? Vowels are alphabetic characters that represent a specific pronunciation. There are five vowels in English, including uppercase and lowercase: a, e, i, o, u Example 1 Input: String = "Tutorialspoint" Output: 6 explain The vowels in the string "Tutorialspoint" are u, o, i, a, o, i. There are 6 yuan in total
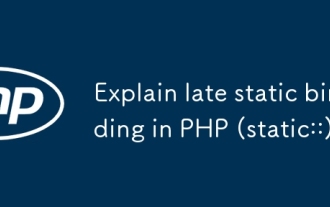
Static binding (static::) implements late static binding (LSB) in PHP, allowing calling classes to be referenced in static contexts rather than defining classes. 1) The parsing process is performed at runtime, 2) Look up the call class in the inheritance relationship, 3) It may bring performance overhead.
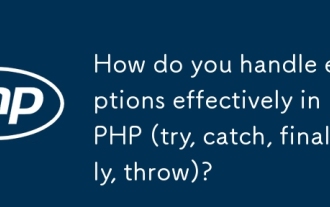
In PHP, exception handling is achieved through the try, catch, finally, and throw keywords. 1) The try block surrounds the code that may throw exceptions; 2) The catch block handles exceptions; 3) Finally block ensures that the code is always executed; 4) throw is used to manually throw exceptions. These mechanisms help improve the robustness and maintainability of your code.
