


PHP gd proportional scaling compression image function, _PHP tutorial
php gd equal scaling compression image function,
The example in this article shares the php gd equal scaling compression image function for your reference, the specific content is as follows
<?php /** * desription 判断是否gif动画 * @param sting $image_file图片路径 * @return boolean t 是 f 否 */ function check_gifcartoon($image_file){ $fp = fopen($image_file,'rb'); $image_head = fread($fp,1024); fclose($fp); return preg_match("/".chr(0x21).chr(0xff).chr(0x0b).'NETSCAPE2.0'."/",$image_head)?false:true; } /** * desription 压缩图片 * @param sting $imgsrc 图片路径 * @param string $imgdst 压缩后保存路径 */ function compressed_image($imgsrc,$imgdst){ list($width,$height,$type)=getimagesize($imgsrc); $new_width = ($width>600?600:$width)*0.9; $new_height =($height>600?600:$height)*0.9; switch($type){ case 1: $giftype=check_gifcartoon($imgsrc); if($giftype){ header('Content-Type:image/gif'); $image_wp=imagecreatetruecolor($new_width, $new_height); $image = imagecreatefromgif($imgsrc); imagecopyresampled($image_wp, $image, 0, 0, 0, 0, $new_width, $new_height, $width, $height); //75代表的是质量、压缩图片容量大小 imagejpeg($image_wp, $imgdst,75); imagedestroy($image_wp); } break; case 2: header('Content-Type:image/jpeg'); $image_wp=imagecreatetruecolor($new_width, $new_height); $image = imagecreatefromjpeg($imgsrc); imagecopyresampled($image_wp, $image, 0, 0, 0, 0, $new_width, $new_height, $width, $height); //75代表的是质量、压缩图片容量大小 imagejpeg($image_wp, $imgdst,75); imagedestroy($image_wp); break; case 3: header('Content-Type:image/png'); $image_wp=imagecreatetruecolor($new_width, $new_height); $image = imagecreatefrompng($imgsrc); imagecopyresampled($image_wp, $image, 0, 0, 0, 0, $new_width, $new_height, $width, $height); //75代表的是质量、压缩图片容量大小 imagejpeg($image_wp, $imgdst,75); imagedestroy($image_wp); break; } }
php and gd function reference table
gd_info Get information about the currently installed GD library
getimagesize Get image size
getimagesizefromstring Get the size of an image from a string
image_type_to_extension Get the file suffix of the image type
image_type_to_mime_type gets the MIME type of the image type returned by getimagesize, exif_read_data, exif_thumbnail, exif_imagetype
image2wbmp Outputs images in WBMP format to a browser or file
imageaffine Return an image containing the affine tramsformed src image, using an optional clipping area
imageaffinematrixconcat Concat two matrices (as in doing many ops in one go)
imageaffinematrixget Return an image containing the affine tramsformed src image, using an optional clipping area
imagealphblending sets the color blending mode of the image
imageantialias Whether to use the antialias function
imagearc Draw elliptical arc
imagechar draws a character horizontally
imagecharup draws a character vertically
imagecolorallocate assigns a color to an image
imagecolorallocatealpha assigns color alpha to an image
imagecolorat Gets the color index value of a certain pixel
imagecolorclosest Gets the index value of the color closest to the specified color
imagecolorclosestalpha Gets the color closest to the specified color plus transparency
imagecolorclosesthwb Gets the black and white index of the chromaticity closest to the given color
imagecolordeallocate cancels the allocation of image color
imagecolorexact Gets the index value of the specified color
imagecolorexactalpha Gets the index value of the specified color plus transparency
imagecolormatch enables a better match between the palette version of an image and the true color version
imagecolorresolve Gets the index value of the specified color or the closest alternative value possible
imagecolorresolvealpha Gets the index value of the specified color alpha or the closest alternative value possible
imagecolorset sets the color for the specified palette index
imagecolorsforindex Get the color of a certain index
imagecolorstotal Gets the number of colors in the palette of an image
imagecolortransparent Defines a color as a transparent color
imageconvolution uses coefficients div and offset to apply for a 3x3 convolution matrix
imagecopy Copy part of an image
imagecopymerge Copy and merge part of an image
imagecopymergegray Copy and merge part of an image in grayscale
imagecopyresampled resamples and copies part of the image and resizes
imagecopyresized Copy part of the image and resize it
imagecreate Create a new palette-based image
imagecreatefromgd2 Create a new image from a GD2 file or URL
imagecreatefromgd2part Creates a new image from a part of the given GD2 file or URL
imagecreatefromgd Create a new image from a GD file or URL
imagecreatefromgif Creates a new image from a file or URL.
imagecreatefromjpeg Creates a new image from a file or URL.
imagecreatefrompng Creates a new image from a file or URL.
imagecreatefromstring creates a new image from the image stream in the string
imagecreatefromwbmp Creates a new image from a file or URL.
imagecreatefromwebp Creates a new image from a file or URL.
imagecreatefromxbm Creates a new image from a file or URL.
imagecreatefromxpm Creates a new image from a file or URL.
imagecreatetruecolor Create a new true color image
imagecrop Crop an image using the given coordinates and size, x, y, width and height
imagecropauto Crop an image automatically using one of the available modes
imagedashedline draw a dotted line
imagedestroy Destroy an image
imageellipse Draw an ellipse
imagefill area filling
imagefilledarc draws an elliptical arc and fills it
imagefilledellipse Draw an ellipse and fill it
imagefilledpolygon Draw a polygon and fill it
imagefilledrectangle Draw a rectangle and fill it
imagefilltoborder fills the area to the border of the specified color
imagefilter applies a filter to an image
imageflip Flips an image using a given mode
imagefontheight Get the font height
imagefontwidth Gets the font width
imageftbbox gives a text box using FreeType 2 font
imagefttext Write text to an image using FreeType 2 fonts
imagegammacorrect applies gamma correction to GD images
imagegd2 Output GD2 images to a browser or file
imagegd Output GD images to a browser or file
imagegif Outputs images to a browser or file.
imagegrabscreen Captures the whole screen
imagegrabwindow Captures a window
imageinterlace Activate or disable interlacing
imageistruecolor Checks whether the image is a true color image
imagejpeg Outputs images to a browser or file.
imagelayereffect sets the alpha blending flag to use the bundled libgd layering effect
imageline Draw a line segment
imageloadfont Load a new font
imagepalettecopy Copies a palette from one image to another
imagepalettetotruecolor Converts a palette based image to true color
imagepng Output an image to a browser or file in PNG format
imagepolygon Draw a polygon
imagepsbbox gives a text box using PostScript Type1 font
imagepsencodefont changes the character encoding vector in the font
imagepsextendfont Expand or streamline fonts
imagepsfreefont Frees the memory occupied by a PostScript Type 1 font
imagepsloadfont loads a PostScript Type 1 font from a file
imagepsslantfont tilt a certain font
imagepstext uses PostScript Type1 font to draw text strings on images
imagerectangle Draw a rectangle
imagerotate rotates the image by a given angle
imagesavealpha Set flag to save full alpha channel information when saving PNG images (as opposed to a single transparent color)
imagescale Scale an image using the given new width and height
imagesetbrush sets the brush image used for drawing lines
imagesetinterpolation Set the interpolation method
imagesetpixel draws a single pixel
imagesetstyle Set the style of line drawing
imagesethickness sets the width of the line
imagesettile sets the texture used for filling
imagestring draws a line of string horizontally
imagestringup draws a line of string vertically
imagesx gets image width
imagesy Get image height
imagetruecolortopalette Converts a true color image to a palette image
imagettfbbox Get the range of text using TrueType font
imagettftext Write text to image using TrueType font
imagetypes returns the image types supported by the current PHP version
imagewbmp Outputs images in WBMP format to a browser or file
imagewebp Output an WebP image to browser or file
imagexbm Outputs XBM images to a browser or file
iptcembed embeds binary IPTC data into a JPEG image
iptcparse parses binary IPTC chunks into individual tokens
jpeg2wbmp Convert JPEG image files to WBMP image files
png2wbmp Convert PNG image files to WBMP image files
The above is the entire content of this article. I hope it will be helpful to everyone in learning PHP programming.

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
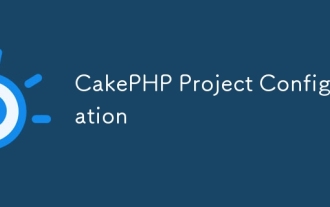
In this chapter, we will understand the Environment Variables, General Configuration, Database Configuration and Email Configuration in CakePHP.
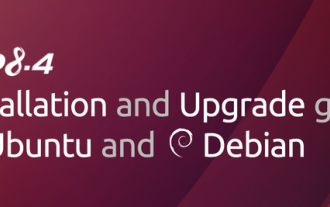
PHP 8.4 brings several new features, security improvements, and performance improvements with healthy amounts of feature deprecations and removals. This guide explains how to install PHP 8.4 or upgrade to PHP 8.4 on Ubuntu, Debian, or their derivati
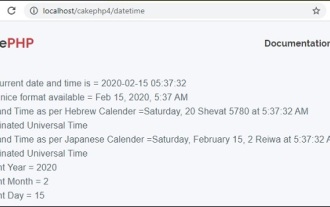
To work with date and time in cakephp4, we are going to make use of the available FrozenTime class.
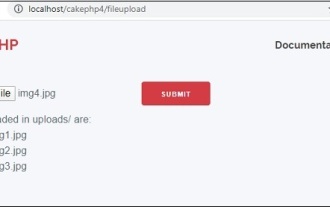
To work on file upload we are going to use the form helper. Here, is an example for file upload.
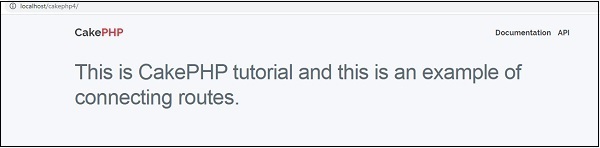
In this chapter, we are going to learn the following topics related to routing ?
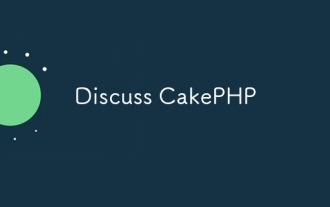
CakePHP is an open-source framework for PHP. It is intended to make developing, deploying and maintaining applications much easier. CakePHP is based on a MVC-like architecture that is both powerful and easy to grasp. Models, Views, and Controllers gu
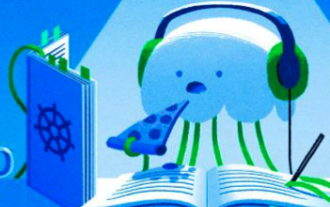
Visual Studio Code, also known as VS Code, is a free source code editor — or integrated development environment (IDE) — available for all major operating systems. With a large collection of extensions for many programming languages, VS Code can be c
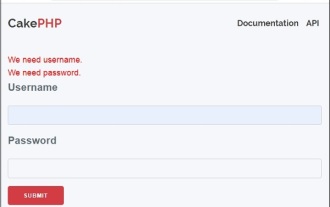
Validator can be created by adding the following two lines in the controller.
