


Detailed explanation of usage examples of Zend Framework action assistant Redirector, zendredirector_PHP tutorial
Zend Framework action assistant Redirector usage example detailed explanation, zendredirector
This article describes the Zend Framework action assistant Redirector usage example. Share it with everyone for your reference, the details are as follows:
Redirector provides another implementation method to help the program redirect to internal or external pages;
The Redirector Helper lets you use a redirector object to help redirect to a new URL. It has several advantages over the _redirect() method. For example, you can pre-configure the behavior of the entire site in the redirector object, or use the gotoSimple($action, $controller, $module, $params) interface similar to Zend_Controller_Action::_forward().
Redirectors have a number of methods that influence redirection behavior:
setCode() sets the HTTP response code used during redirection.
setExit() forces the exit() method after redirection. Set by default.
setGotoSimple() sets the default URL and redirects to this URL when no parameters are provided to the gotoSimple() method. You can use an API similar to Zend_Controller_Action::_forward(): setGotoSimple($action, $controller = null, $module = null, array $params = array());
setGotoRoute() sets the URL based on a registered router. By passing in a key/value array and a router name, it will organize the URLs based on the router's type and definition.
setGotoUrl() sets the default URL. When no parameters are passed to gotoUrl(), the URL will be used. Accepts a single URL string.
setPrependBase() adds the base URL of the request object in front of the URL specified by setGotoUrl(), gotoUrl() or gotoUrlAndExit().
setUseAbsoluteUri() forces the redirector to use an absolute URI when redirecting. When this option is set, $_SERVER['HTTP_HOST'], $_SERVER['SERVER_PORT'], and $_SERVER['HTTPS'] and the URL specified by the redirect method will be used to form a complete URI. This option is currently off by default and may be on by default in a future release.
Additionally, there are tons of methods in the redirector to perform the actual redirection .
gotoSimple() uses setGotoSimple() (an API similar to _forward()) to build the URL and perform redirection.
gotoRoute() uses setGotoRoute() (route assembly route-assembly) to build the URL and perform redirection.
gotoUrl() uses setGotoUrl() URL string) to construct the URL and perform redirection.
Finally, you can determine the current redirect URL at any time using getRedirectUrl().
Basic use cases
Example #5 Setting options
This example changes several options, including setting the HTTP status code used when redirecting to 303, not exiting by default when redirecting, and defining a default URL for redirection.
class SomeController extends Zend_Controller_Action { /** * Redirector - defined for code completion * * @var Zend_Controller_Action_Helper_Redirector */ protected $_redirector = null; public function init() { $this->_redirector = $this->_helper->getHelper('Redirector'); // Set the default options for the redirector // Since the object is registered in the helper broker, these // become relevant for all actions from this point forward $this->_redirector->setCode(303) ->setExit(false) ->setGotoSimple("this-action", "some-controller"); } public function myAction() { /* do some stuff */ // Redirect to a previously registered URL, and force an exit // to occur when done: $this->_redirector->redirectAndExit(); return; // never reached } }
Example #6 Use default settings
This example assumes the default settings, which means any redirect will result in an immediate exit.
// ALTERNATIVE EXAMPLE class AlternativeController extends Zend_Controller_Action { /** * Redirector - defined for code completion * * @var Zend_Controller_Action_Helper_Redirector */ protected $_redirector = null; public function init() { $this->_redirector = $this->_helper->getHelper('Redirector'); } public function myAction() { /* do some stuff */ $this->_redirector ->gotoUrl('/my-controller/my-action/param1/test/param2/test2'); return; // never reached since default is to goto and exit } }
Example #7 _forward()API using goto()
gotoSimple()'s API simulates Zend_Controller_Action::_forward(). The main difference is that it constructs the URL from the parameters passed in, using the default format of the default router: module/:controller/:action/*. Then redirect instead of continuing the action chain loop.
class ForwardController extends Zend_Controller_Action { /** * Redirector - defined for code completion * * @var Zend_Controller_Action_Helper_Redirector */ protected $_redirector = null; public function init() { $this->_redirector = $this->_helper->getHelper('Redirector'); } public function myAction() { /* do some stuff */ // Redirect to 'my-action' of 'my-controller' in the current // module, using the params param1 => test and param2 => test2 $this->_redirector->gotoSimple('my-action', 'my-controller', null, array('param1' => 'test', 'param2' => 'test2' ) ); } }
Example #8 Using route assembly via gotoRoute()
The following example uses the router's assemble() method to create a URL based on an associative array of passed in parameters. It is assumed that the following route has been registered:
$route = new Zend_Controller_Router_Route( 'blog/:year/:month/:day/:id', array('controller' => 'archive', 'module' => 'blog', 'action' => 'view') ); $router->addRoute('blogArchive', $route);
Given an array, the year is 2006, the month is 4, the date is 24, and the id is 42, based on which the URL/blog/2006/4/24/42 can be assembled.
class BlogAdminController extends Zend_Controller_Action { /** * Redirector - defined for code completion * * @var Zend_Controller_Action_Helper_Redirector */ protected $_redirector = null; public function init() { $this->_redirector = $this->_helper->getHelper('Redirector'); } public function returnAction() { /* do some stuff */ // Redirect to blog archive. Builds the following URL: // /blog/2006/4/24/42 $this->_redirector->gotoRoute( array('year' => 2006, 'month' => 4, 'day' => 24, 'id' => 42), 'blogArchive' ); } }
Source code of Zend_Controller_Action_Helper_Redirector.
It is not difficult to see the implementation method and common usage methods through the source code.
<?php /** * @see Zend_Controller_Action_Helper_Abstract */ require_once 'Zend/Controller/Action/Helper/Abstract.php'; /** * @category Zend * @package Zend_Controller * @subpackage Zend_Controller_Action_Helper * @copyright Copyright (c) 2005-2011 Zend Technologies USA Inc. (http://www.zend.com) * @license http://framework.zend.com/license/new-bsd New BSD License */ class Zend_Controller_Action_Helper_Redirector extends Zend_Controller_Action_Helper_Abstract { /** * HTTP status code for redirects * @var int */ protected $_code = 302; /** * Whether or not calls to _redirect() should exit script execution * @var boolean */ protected $_exit = true; /** * Whether or not _redirect() should attempt to prepend the base URL to the * passed URL (if it's a relative URL) * @var boolean */ protected $_prependBase = true; /** * Url to which to redirect * @var string */ protected $_redirectUrl = null; /** * Whether or not to use an absolute URI when redirecting * @var boolean */ protected $_useAbsoluteUri = false; /** * Whether or not to close the session before exiting * @var boolean */ protected $_closeSessionOnExit = true; /** * Retrieve HTTP status code to emit on {@link _redirect()} call * * @return int */ public function getCode() { return $this->_code; } /** * Validate HTTP status redirect code * * @param int $code * @throws Zend_Controller_Action_Exception on invalid HTTP status code * @return true */ protected function _checkCode($code) { $code = (int)$code; if ((300 > $code) || (307 < $code) || (304 == $code) || (306 == $code)) { require_once 'Zend/Controller/Action/Exception.php'; throw new Zend_Controller_Action_Exception('Invalid redirect HTTP status code (' . $code . ')'); } return true; } /** * Retrieve HTTP status code for {@link _redirect()} behaviour * * @param int $code * @return Zend_Controller_Action_Helper_Redirector Provides a fluent interface */ public function setCode($code) { $this->_checkCode($code); $this->_code = $code; return $this; } /** * Retrieve flag for whether or not {@link _redirect()} will exit when finished. * * @return boolean */ public function getExit() { return $this->_exit; } /** * Retrieve exit flag for {@link _redirect()} behaviour * * @param boolean $flag * @return Zend_Controller_Action_Helper_Redirector Provides a fluent interface */ public function setExit($flag) { $this->_exit = ($flag) ? true : false; return $this; } /** * Retrieve flag for whether or not {@link _redirect()} will prepend the * base URL on relative URLs * * @return boolean */ public function getPrependBase() { return $this->_prependBase; } /** * Retrieve 'prepend base' flag for {@link _redirect()} behaviour * * @param boolean $flag * @return Zend_Controller_Action_Helper_Redirector Provides a fluent interface */ public function setPrependBase($flag) { $this->_prependBase = ($flag) ? true : false; return $this; } /** * Retrieve flag for whether or not {@link redirectAndExit()} shall close the session before * exiting. * * @return boolean */ public function getCloseSessionOnExit() { return $this->_closeSessionOnExit; } /** * Set flag for whether or not {@link redirectAndExit()} shall close the session before exiting. * * @param boolean $flag * @return Zend_Controller_Action_Helper_Redirector Provides a fluent interface */ public function setCloseSessionOnExit($flag) { $this->_closeSessionOnExit = ($flag) ? true : false; return $this; } /** * Return use absolute URI flag * * @return boolean */ public function getUseAbsoluteUri() { return $this->_useAbsoluteUri; } /** * Set use absolute URI flag * * @param boolean $flag * @return Zend_Controller_Action_Helper_Redirector Provides a fluent interface */ public function setUseAbsoluteUri($flag = true) { $this->_useAbsoluteUri = ($flag) ? true : false; return $this; } /** * Set redirect in response object * * @return void */ protected function _redirect($url) { if ($this->getUseAbsoluteUri() && !preg_match('#^(https?|ftp)://#', $url)) { $host = (isset($_SERVER['HTTP_HOST'])?$_SERVER['HTTP_HOST']:''); $proto = (isset($_SERVER['HTTPS'])&&$_SERVER['HTTPS']!=="off") ? 'https' : 'http'; $port = (isset($_SERVER['SERVER_PORT'])?$_SERVER['SERVER_PORT']:80); $uri = $proto . '://' . $host; if ((('http' == $proto) && (80 != $port)) || (('https' == $proto) && (443 != $port))) { // do not append if HTTP_HOST already contains port if (strrchr($host, ':') === false) { $uri .= ':' . $port; } } $url = $uri . '/' . ltrim($url, '/'); } $this->_redirectUrl = $url; $this->getResponse()->setRedirect($url, $this->getCode()); } /** * Retrieve currently set URL for redirect * * @return string */ public function getRedirectUrl() { return $this->_redirectUrl; } /** * Determine if the baseUrl should be prepended, and prepend if necessary * * @param string $url * @return string */ protected function _prependBase($url) { if ($this->getPrependBase()) { $request = $this->getRequest(); if ($request instanceof Zend_Controller_Request_Http) { $base = rtrim($request->getBaseUrl(), '/'); if (!empty($base) && ('/' != $base)) { $url = $base . '/' . ltrim($url, '/'); } else { $url = '/' . ltrim($url, '/'); } } } return $url; } /** * Set a redirect URL of the form /module/controller/action/params * * @param string $action * @param string $controller * @param string $module * @param array $params * @return void */ public function setGotoSimple($action, $controller = null, $module = null, array $params = array()) { $dispatcher = $this->getFrontController()->getDispatcher(); $request = $this->getRequest(); $curModule = $request->getModuleName(); $useDefaultController = false; if (null === $controller && null !== $module) { $useDefaultController = true; } if (null === $module) { $module = $curModule; } if ($module == $dispatcher->getDefaultModule()) { $module = ''; } if (null === $controller && !$useDefaultController) { $controller = $request->getControllerName(); if (empty($controller)) { $controller = $dispatcher->getDefaultControllerName(); } } $params[$request->getModuleKey()] = $module; $params[$request->getControllerKey()] = $controller; $params[$request->getActionKey()] = $action; $router = $this->getFrontController()->getRouter(); $url = $router->assemble($params, 'default', true); $this->_redirect($url); } /** * Build a URL based on a route * * @param array $urlOptions * @param string $name Route name * @param boolean $reset * @param boolean $encode * @return void */ public function setGotoRoute(array $urlOptions = array(), $name = null, $reset = false, $encode = true) { $router = $this->getFrontController()->getRouter(); $url = $router->assemble($urlOptions, $name, $reset, $encode); $this->_redirect($url); } /** * Set a redirect URL string * * By default, emits a 302 HTTP status header, prepends base URL as defined * in request object if url is relative, and halts script execution by * calling exit(). * * $options is an optional associative array that can be used to control * redirect behaviour. The available option keys are: * - exit: boolean flag indicating whether or not to halt script execution when done * - prependBase: boolean flag indicating whether or not to prepend the base URL when a relative URL is provided * - code: integer HTTP status code to use with redirect. Should be between 300 and 307. * * _redirect() sets the Location header in the response object. If you set * the exit flag to false, you can override this header later in code * execution. * * If the exit flag is true (true by default), _redirect() will write and * close the current session, if any. * * @param string $url * @param array $options * @return void */ public function setGotoUrl($url, array $options = array()) { // prevent header injections $url = str_replace(array("\n", "\r"), '', $url); if (null !== $options) { if (isset($options['exit'])) { $this->setExit(($options['exit']) ? true : false); } if (isset($options['prependBase'])) { $this->setPrependBase(($options['prependBase']) ? true : false); } if (isset($options['code'])) { $this->setCode($options['code']); } } // If relative URL, decide if we should prepend base URL if (!preg_match('|^[a-z]+://|', $url)) { $url = $this->_prependBase($url); } $this->_redirect($url); } /** * Perform a redirect to an action/controller/module with params * * @param string $action * @param string $controller * @param string $module * @param array $params * @return void */ public function gotoSimple($action, $controller = null, $module = null, array $params = array()) { $this->setGotoSimple($action, $controller, $module, $params); if ($this->getExit()) { $this->redirectAndExit(); } } /** * Perform a redirect to an action/controller/module with params, forcing an immdiate exit * * @param mixed $action * @param mixed $controller * @param mixed $module * @param array $params * @return void */ public function gotoSimpleAndExit($action, $controller = null, $module = null, array $params = array()) { $this->setGotoSimple($action, $controller, $module, $params); $this->redirectAndExit(); } /** * Redirect to a route-based URL * * Uses route's assemble method tobuild the URL; route is specified by $name; * default route is used if none provided. * * @param array $urlOptions Array of key/value pairs used to assemble URL * @param string $name * @param boolean $reset * @param boolean $encode * @return void */ public function gotoRoute(array $urlOptions = array(), $name = null, $reset = false, $encode = true) { $this->setGotoRoute($urlOptions, $name, $reset, $encode); if ($this->getExit()) { $this->redirectAndExit(); } } /** * Redirect to a route-based URL, and immediately exit * * Uses route's assemble method tobuild the URL; route is specified by $name; * default route is used if none provided. * * @param array $urlOptions Array of key/value pairs used to assemble URL * @param string $name * @param boolean $reset * @return void */ public function gotoRouteAndExit(array $urlOptions = array(), $name = null, $reset = false) { $this->setGotoRoute($urlOptions, $name, $reset); $this->redirectAndExit(); } /** * Perform a redirect to a url * * @param string $url * @param array $options * @return void */ public function gotoUrl($url, array $options = array()) { $this->setGotoUrl($url, $options); if ($this->getExit()) { $this->redirectAndExit(); } } /** * Set a URL string for a redirect, perform redirect, and immediately exit * * @param string $url * @param array $options * @return void */ public function gotoUrlAndExit($url, array $options = array()) { $this->setGotoUrl($url, $options); $this->redirectAndExit(); } /** * exit(): Perform exit for redirector * * @return void */ public function redirectAndExit() { if ($this->getCloseSessionOnExit()) { // Close session, if started if (class_exists('Zend_Session', false) && Zend_Session::isStarted()) { Zend_Session::writeClose(); } elseif (isset($_SESSION)) { session_write_close(); } } $this->getResponse()->sendHeaders(); exit(); } /** * direct(): Perform helper when called as * $this->_helper->redirector($action, $controller, $module, $params) * * @param string $action * @param string $controller * @param string $module * @param array $params * @return void */ public function direct($action, $controller = null, $module = null, array $params = array()) { $this->gotoSimple($action, $controller, $module, $params); } /** * Overloading * * Overloading for old 'goto', 'setGoto', and 'gotoAndExit' methods * * @param string $method * @param array $args * @return mixed * @throws Zend_Controller_Action_Exception for invalid methods */ public function __call($method, $args) { $method = strtolower($method); if ('goto' == $method) { return call_user_func_array(array($this, 'gotoSimple'), $args); } if ('setgoto' == $method) { return call_user_func_array(array($this, 'setGotoSimple'), $args); } if ('gotoandexit' == $method) { return call_user_func_array(array($this, 'gotoSimpleAndExit'), $args); } require_once 'Zend/Controller/Action/Exception.php'; throw new Zend_Controller_Action_Exception(sprintf('Invalid method "%s" called on redirector', $method)); } }
Readers who are interested in more zend-related content can check out the special topics of this site: "Zend FrameWork Framework Introductory Tutorial", "php Excellent Development Framework Summary", "Yii Framework Introduction and Summary of Common Techniques", "ThinkPHP Introductory Tutorial" , "php object-oriented programming introductory tutorial", "php mysql database operation introductory tutorial" and "php common database operation skills summary"
I hope this article will be helpful to everyone in PHP programming.
Articles you may be interested in:
- Analysis of Controller Usage of MVC Framework in Zend Framework Tutorial
- Zend Framework Tutorial Road explained in detail by function Zend_Controller_Router
- Zend Framework tutorial: Detailed explanation of Zend_Controller_Plugin plug-in usage
- Zend Framework tutorial: Detailed explanation of the encapsulation of the response object Zend_Controller_Response instance
- Zend Framework tutorial: Detailed explanation of the encapsulation of the request object Zend_Controller_Request instance
- Zend Framework tutorial Detailed explanation of the action's base class Zend_Controller_Action
- Zend Framework tutorial's detailed explanation of the usage of the distributor Zend_Controller_Dispatcher
- Zend Framework tutorial's detailed explanation of the usage of the front-end controller Zend_Controller_Front
- Zend Framework action assistant Url usage Detailed explanation
- Zend Framework action assistant Json usage example analysis
- Zend Framework action assistant FlashMessenger usage detailed explanation
- Zend Framework tutorial Resource Autoloading usage example

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
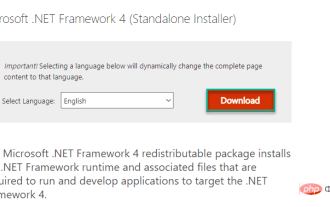
.NET Framework 4 is required by developers and end users to run the latest versions of applications on Windows. However, while downloading and installing .NET Framework 4, many users complained that the installer stopped midway, displaying the following error message - " .NET Framework 4 has not been installed because Download failed with error code 0x800c0006 ". If you are also experiencing it while installing .NETFramework4 on your device then you are at the right place
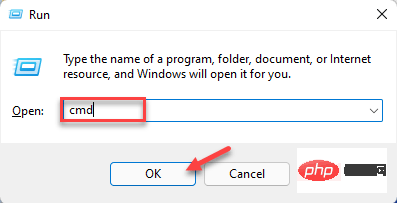
Whenever your Windows 11 or Windows 10 PC has an upgrade or update issue, you will usually see an error code indicating the actual reason behind the failure. However, sometimes confusion can arise when an upgrade or update fails without an error code being displayed. With handy error codes, you know exactly where the problem is so you can try to fix it. But since no error code appears, it becomes challenging to identify the issue and resolve it. This will take up a lot of your time to simply find out the reason behind the error. In this case, you can try using a dedicated tool called SetupDiag provided by Microsoft that helps you easily identify the real reason behind the error.
![SCNotification has stopped working [5 steps to fix it]](https://img.php.cn/upload/article/000/887/227/168433050522031.png?x-oss-process=image/resize,m_fill,h_207,w_330)
As a Windows user, you are likely to encounter SCNotification has stopped working error every time you start your computer. SCNotification.exe is a Microsoft system notification file that crashes every time you start your PC due to permission errors and network failures. This error is also known by its problematic event name. So you might not see this as SCNotification having stopped working, but as bug clr20r3. In this article, we will explore all the steps you need to take to fix SCNotification has stopped working so that it doesn’t bother you again. What is SCNotification.e
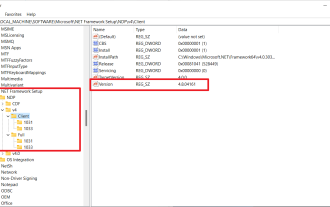
Microsoft Windows users who have installed Microsoft.NET version 4.5.2, 4.6, or 4.6.1 must install a newer version of the Microsoft Framework if they want Microsoft to support the framework through future product updates. According to Microsoft, all three frameworks will cease support on April 26, 2022. After the support date ends, the product will not receive "security fixes or technical support." Most home devices are kept up to date through Windows updates. These devices already have newer versions of frameworks installed, such as .NET Framework 4.8. Devices that are not updating automatically may
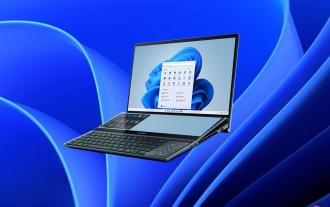
It's been a week since we talked about the new safe mode bug affecting users who installed KB5012643 for Windows 11. This pesky issue didn't appear on the list of known issues Microsoft posted on launch day, thus catching everyone by surprise. Well, just when you thought things couldn't get any worse, Microsoft drops another bomb for users who have installed this cumulative update. Windows 11 Build 22000.652 causes more problems So the tech company is warning Windows 11 users that they may experience problems launching and using some .NET Framework 3.5 applications. Sound familiar? But please don't be surprised
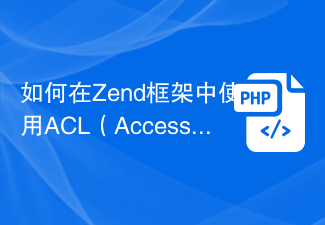
How to use ACL (AccessControlList) for permission control in Zend Framework Introduction: In a web application, permission control is a crucial function. It ensures that users can only access the pages and features they are authorized to access and prevents unauthorized access. The Zend framework provides a convenient way to implement permission control, using the ACL (AccessControlList) component. This article will introduce how to use ACL in Zend Framework
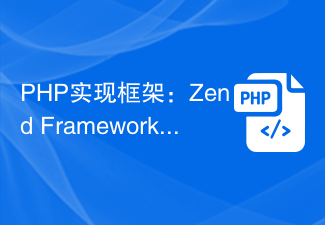
PHP implementation framework: ZendFramework introductory tutorial ZendFramework is an open source website framework developed by PHP and is currently maintained by ZendTechnologies. ZendFramework adopts the MVC design pattern and provides a series of reusable code libraries to serve the implementation of Web2.0 applications and Web Serve. ZendFramework is very popular and respected by PHP developers and has a wide range of
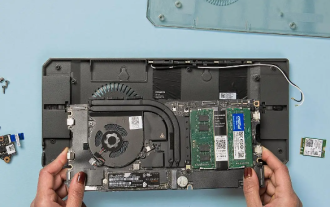
According to news on December 9, Cooler Master recently demonstrated a mini chassis kit in cooperation with notebook modular solution provider Framework at a demonstration event at the Taipei Compute Show. The unique thing about this kit is that it can be compatible with and Install the motherboard from the framework notebook. Currently, this product has begun to be sold on the market, priced at 39 US dollars, which is equivalent to approximately 279 yuan at the current exchange rate. The model number of this chassis kit is named "frameWORKMAINBOARDCASE". In terms of design, it embodies the ultimate compactness and practicality, measuring only 297x133x15 mm. Its original design is to be able to seamlessly connect to framework notebooks
