


About how to use curl to read HTTP chunked data in PHP, curlchunked_PHP tutorial
About how PHP uses curl to read HTTP chunked data, curlchunked
For the HTTP chunked data returned by the web server, we may want to get a callback when each chunk returns, instead of Callback after all responses are returned. For example, when the server is icomet.
The code for using curl in PHP is as follows:
1 2 3 4 5 6 7 8 9 10 11 |
|
However, there is a problem here. For a chunk, the callback function may be called multiple times, each time about 16k of data. This is obviously not what we want. Because a chunk of icomet ends with "n" , so the callback function can do some buffering.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 |
|
Let me introduce chunked php to use fsockopen to read segmented data (transfer-encoding: chunked)
I encountered a magical problem when using fsockopen to read data. The specific situation is as follows:
Reading address: http://blog.maxthon.cn/?feed=rss2
Read code:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 |
|
Return http content:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 |
|
Please pay attention to the four characters marked in red above. They will appear every other piece of data, but data retrieved using other methods such as curl, file_get_contents, etc. do not have these things. If I switch to other websites to crawl, this will happen to only a few websites. After multiple searches without a solution, I accidentally saw such a statement in the return header above: Transfer-Encoding: chunked, and the common Content The -lenght field is gone. The general meaning of this statement is that the transfer encoding is segmented.
Search this keyword on Google and find the explanation of this statement on Wikipedia (since there is no Chinese version, I can only translate it myself):
1 |
|
Chunked transfer encoding is a mechanism that allows HTTP messages to be transmitted in several parts. Applies to both HTTP requests (from client to server) and HTTP responses (from server to client)
For example, let us consider the way in which an HTTP server may transmit data to a client application (usually a web browser). Normally, data delivered in HTTP responses is sent in one piece, whose length is indicated by the Content -Length header field. The length of the data is important, because the client needs to know where the response ends and any following response starts. With chunked encoding, however, the data is broken up into a series of blocks of data and transmitted in one or more "chunks" so that a server may start sending data before it knows the final size of the content that it's sending. Often, the size of these blocks is the same, but this is not always the case.
For example, let's consider the ways in which an HTTP server can transmit data to a client application (usually a web browser). Normally, HTTP response data is sent to the client in one block, and the length of the data is represented by the Content-Length header field. The length of the data is important because the client needs to know where the response ends and when subsequent responses start. With Chunked encoding, the data is divided into a series of data chunks and one or more forwarded "chunks" anyway, so the server can start sending data before it knows the length of the content. Usually, the sizes of these data blocks are the same, but this is not absolute.
After understanding the general meaning, let’s look at an example:
Chunked encoding is formed by concatenating several Chunks and ends with a chunk indicating a length of 0. Each Chunk is divided into two parts: the header and the text. The header content specifies the total number of characters (hexadecimal numbers) and the quantity unit (generally not written) of the next paragraph of text. The text part is the actual content of the specified length. The two parts Separate them with carriage return and line feed (CRLF). The content in the last Chunk of length 0 is called footer, which is some additional Header information (usually can be ignored directly). The specific Chunk encoding format is as follows:
Encoded response content:
HTTP/1.1 200 OK
Content-Type: text/plain
Transfer-Encoding: chunked
25
This is the first piece of data
1A
Then this is the second piece of data
0
Decoded data:
This is the first piece of content, and then this is the second piece of data
The situation is clear, so how do we decode this encoded data?
In the comments under the fsockopen function in the official PHP manual, many people have already proposed solutions
Method 1.
1 2 3 4 5 6 7 8 9 10 11 12 |
|
Method 2.
1 2 3 4 5 6 7 8 9 10 11 12 13 |
|
Note: The parameters of these two functions are the returned http raw data (including headers)
Articles you may be interested in:
- Introduction to PHP’s cURL library function to capture web pages, POST data and others
- Use three methods of Curl, socket and file_get_contents in PHP POST submit data
- Code of PHP using CURL to POST data to API interface
- Analysis of PHP using curl to submit json format data
- php example of using curl to send json format data
- Example of php curl simulating post submission data
- Example of php using curl and regular expressions to grab web page data
- PHP function sharing using curl method to obtain data, simulate login, and POST data

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


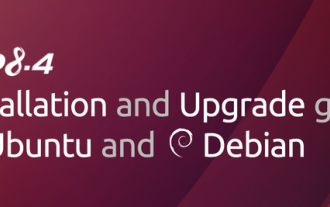
PHP 8.4 brings several new features, security improvements, and performance improvements with healthy amounts of feature deprecations and removals. This guide explains how to install PHP 8.4 or upgrade to PHP 8.4 on Ubuntu, Debian, or their derivati
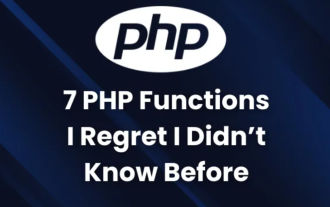
If you are an experienced PHP developer, you might have the feeling that you’ve been there and done that already.You have developed a significant number of applications, debugged millions of lines of code, and tweaked a bunch of scripts to achieve op
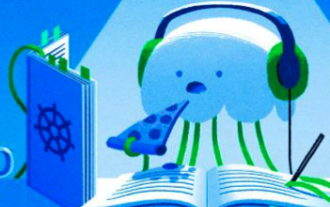
Visual Studio Code, also known as VS Code, is a free source code editor — or integrated development environment (IDE) — available for all major operating systems. With a large collection of extensions for many programming languages, VS Code can be c
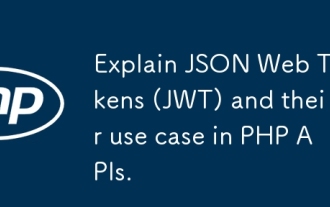
JWT is an open standard based on JSON, used to securely transmit information between parties, mainly for identity authentication and information exchange. 1. JWT consists of three parts: Header, Payload and Signature. 2. The working principle of JWT includes three steps: generating JWT, verifying JWT and parsing Payload. 3. When using JWT for authentication in PHP, JWT can be generated and verified, and user role and permission information can be included in advanced usage. 4. Common errors include signature verification failure, token expiration, and payload oversized. Debugging skills include using debugging tools and logging. 5. Performance optimization and best practices include using appropriate signature algorithms, setting validity periods reasonably,
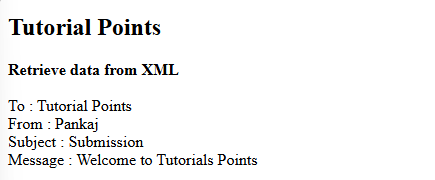
This tutorial demonstrates how to efficiently process XML documents using PHP. XML (eXtensible Markup Language) is a versatile text-based markup language designed for both human readability and machine parsing. It's commonly used for data storage an
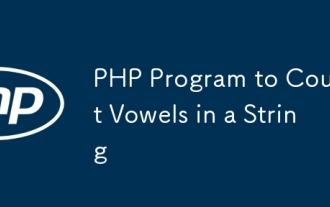
A string is a sequence of characters, including letters, numbers, and symbols. This tutorial will learn how to calculate the number of vowels in a given string in PHP using different methods. The vowels in English are a, e, i, o, u, and they can be uppercase or lowercase. What is a vowel? Vowels are alphabetic characters that represent a specific pronunciation. There are five vowels in English, including uppercase and lowercase: a, e, i, o, u Example 1 Input: String = "Tutorialspoint" Output: 6 explain The vowels in the string "Tutorialspoint" are u, o, i, a, o, i. There are 6 yuan in total
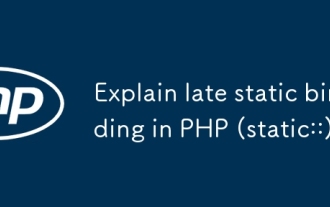
Static binding (static::) implements late static binding (LSB) in PHP, allowing calling classes to be referenced in static contexts rather than defining classes. 1) The parsing process is performed at runtime, 2) Look up the call class in the inheritance relationship, 3) It may bring performance overhead.

What are the magic methods of PHP? PHP's magic methods include: 1.\_\_construct, used to initialize objects; 2.\_\_destruct, used to clean up resources; 3.\_\_call, handle non-existent method calls; 4.\_\_get, implement dynamic attribute access; 5.\_\_set, implement dynamic attribute settings. These methods are automatically called in certain situations, improving code flexibility and efficiency.
