


Examples to explain polymorphism in PHP object-oriented programming_php skills
What is polymorphism?
Polymorphism, the definition from dictionary.com is "occurring in different forms, stages or types in independent organizations or in the same organization without fundamental difference." From this definition, we can think ,Polymorphism is a programming way of describing the same ,object through multiple states or phases. In fact, its real meaning is: in actual development, we only need to focus on the programming of an interface or base class, and do not have to worry about the specific class (class) to which an object belongs.
If you are familiar with design patterns, even if you have just a preliminary understanding, then you will understand this concept. In fact, PHP5 polymorphism may be the greatest tool in pattern-based design programming. It allows us to organize similar objects in a logical way so that we don't have to worry about the specific type of the object when coding; moreover, we only need to program a desired interface or base class. The more abstract an application is, the more flexible it becomes -- and polymorphism is one of the best ways to abstract behavior.
For example, let's consider a class called Person. We can subclass Person with classes called David, Charles and Alejandro. Person has an abstract method AcceptFeedback(), and all subclasses must implement this method. This means that any code that uses a subclass of the base Person class can call the AcceptFeedback() method. You don't have to check whether the object is a David or an Alejandro, just knowing that it is a Person is enough. As a result, your code only needs to focus on the "lowest common denominator" - the Person class.
The Person class in this example could also be created as an interface. Of course, there are some differences compared with the above, mainly: an interface does not give any behavior, but only determines a set of rules. A Person interface requires "You must support the AddFeedback() method", while a Person class can provide some default code for the AddFeedback() method - your understanding of this can be "If you do not choose to support AddFeedback(), then You should provide a default implementation. "How to choose an interface or a base class is beyond the topic of this article; however, in general, you need to implement a default method through the base class. You can also use an interface if you can simply outline a desired set of functionality that your class implements.
Popular understanding
The most direct definition of polymorphism is to allow objects of different classes with inheritance relationships to call member functions with the same name and produce different reaction results
Polymorphic code
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 |
|

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


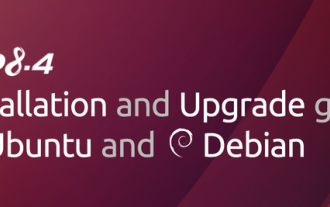
PHP 8.4 brings several new features, security improvements, and performance improvements with healthy amounts of feature deprecations and removals. This guide explains how to install PHP 8.4 or upgrade to PHP 8.4 on Ubuntu, Debian, or their derivati
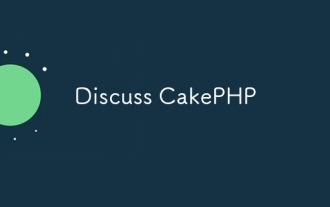
CakePHP is an open-source framework for PHP. It is intended to make developing, deploying and maintaining applications much easier. CakePHP is based on a MVC-like architecture that is both powerful and easy to grasp. Models, Views, and Controllers gu
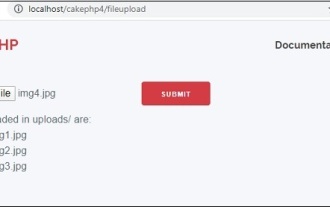
To work on file upload we are going to use the form helper. Here, is an example for file upload.
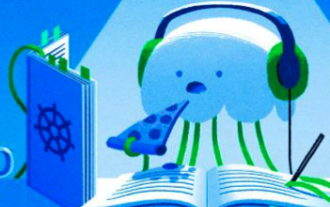
Visual Studio Code, also known as VS Code, is a free source code editor — or integrated development environment (IDE) — available for all major operating systems. With a large collection of extensions for many programming languages, VS Code can be c
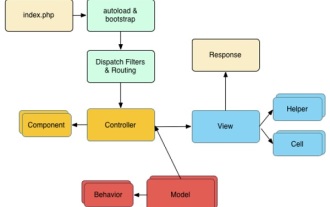
CakePHP is an open source MVC framework. It makes developing, deploying and maintaining applications much easier. CakePHP has a number of libraries to reduce the overload of most common tasks.
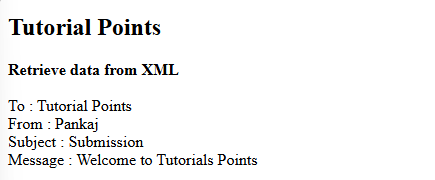
This tutorial demonstrates how to efficiently process XML documents using PHP. XML (eXtensible Markup Language) is a versatile text-based markup language designed for both human readability and machine parsing. It's commonly used for data storage an
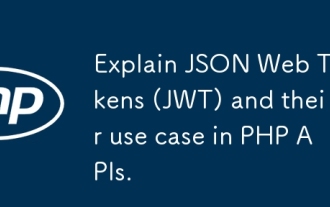
JWT is an open standard based on JSON, used to securely transmit information between parties, mainly for identity authentication and information exchange. 1. JWT consists of three parts: Header, Payload and Signature. 2. The working principle of JWT includes three steps: generating JWT, verifying JWT and parsing Payload. 3. When using JWT for authentication in PHP, JWT can be generated and verified, and user role and permission information can be included in advanced usage. 4. Common errors include signature verification failure, token expiration, and payload oversized. Debugging skills include using debugging tools and logging. 5. Performance optimization and best practices include using appropriate signature algorithms, setting validity periods reasonably,
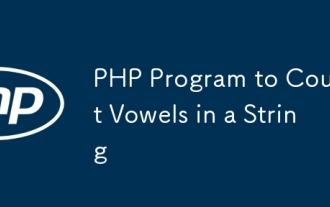
A string is a sequence of characters, including letters, numbers, and symbols. This tutorial will learn how to calculate the number of vowels in a given string in PHP using different methods. The vowels in English are a, e, i, o, u, and they can be uppercase or lowercase. What is a vowel? Vowels are alphabetic characters that represent a specific pronunciation. There are five vowels in English, including uppercase and lowercase: a, e, i, o, u Example 1 Input: String = "Tutorialspoint" Output: 6 explain The vowels in the string "Tutorialspoint" are u, o, i, a, o, i. There are 6 yuan in total
