


Examples of using PHP magic methods, php magic examples_PHP tutorial
Usage examples of PHP magic methods, php magic examples
① __get/__set: Take over the properties of the object
When accessing a non-existent object property:
index.php
Copy code The code is as follows:
define('BASEDIR',__DIR__); //Define root directory constants
include BASEDIR.'/Common/Loader.php';
spl_autoload_register('\Common\Loader::autoload');
$obj = new CommonObject();
//When accessing a non-existent object property in php
echo $obj->title;
will throw an error: Notice: Undefined property: CommonObject::$title in D:practisephpdesignpsr0index.php on line 9
After adding __set and __get methods in Common/Object.php
Object.php
Copy code The code is as follows:
namespace Common;
class Object{
Function __set($key,$value){
}
Function __get($key){
}
}
Execute index.php again and no more errors will be reported.
Modify Common/Object.php again
Copy code The code is as follows:
namespace Common;
class Object{
Protected $array = array();
Function __set($key,$value){
var_dump(__METHOD__);
$this->array[$key] = $value;
}
Function __get($key){
var_dump(__METHOD__);
return $this->array[$key];
}
}
index.php
Copy code The code is as follows:
define('BASEDIR',__DIR__); //Define root directory constants
include BASEDIR.'/Common/Loader.php';
spl_autoload_register('\Common\Loader::autoload');
$obj = new CommonObject();
$obj->title = 'hello';
echo $obj->title;
Execute index.php, page output:
Copy code The code is as follows:
string 'CommonObject::__set' (length=20)
string 'CommonObject::__get' (length=20)
hello
② __call/__callStatic: Control the invocation of PHP object methods (__callStatic is used to control static methods of classes)
When executing a non-existent php method
index.php:
Copy code The code is as follows:
define('BASEDIR',__DIR__); //Define root directory constants
include BASEDIR.'/Common/Loader.php';
spl_autoload_register('\Common\Loader::autoload');
$obj = new CommonObject();
//When executing a non-existent php method
$obj->test('hello',123);
Executing index.php will report a fatal error: Fatal error: Call to undefined method CommonObject::test() in D:practisephpdesignpsr0index.php on line 9
If you define a __call method in Common/Object, it will automatically call back when the method does not exist:
Copy code The code is as follows:
namespace Common;
class Object{
Function __call($func, $param){ //$func method name $param parameter
var_dump($func, $param);
return "magic functionn"; //Return a string as the return value
}
}
index.php
Copy code The code is as follows:
define('BASEDIR',__DIR__); //Define root directory constants
include BASEDIR.'/Common/Loader.php';
spl_autoload_register('\Common\Loader::autoload');
$obj = new CommonObject();
//When executing a non-existent php method
echo $obj->test('hello',123);
Page output:
Copy code The code is as follows:
string 'test' (length=4)
array
0 => string 'hello' (length=5)
1 => int 123
magic function
When calling a non-existent static method
Common/Object.php
Copy code The code is as follows:
namespace Common;
class Object{
static function __callStatic($name, $arguments) {
var_dump($name, $arguments);
return "magic functionn"; //Return a string as the return value
}
}
Note: The __callStatic method must also be declared as a static method
index.php
Copy code The code is as follows:
define('BASEDIR',__DIR__); //Define root directory constants
include BASEDIR.'/Common/Loader.php';
spl_autoload_register('\Common\Loader::autoload');
//Execute a non-existent static method
echo CommonObject::test("hello",1234);
Execute index.php, page output:
Copy code The code is as follows:
string 'test' (length=4)
array
0 => string 'hello' (length=5)
1 => int 1234
magic function
③ __toString: Convert a PHP object into a string
index.php
Copy code The code is as follows:
define('BASEDIR',__DIR__); //Define root directory constants
include BASEDIR.'/Common/Loader.php';
spl_autoload_register('\Common\Loader::autoload');
$obj = new CommonObject();
echo $obj;
An error will be reported at this time: Catchable fatal error: Object of class CommonObject could not be converted to string in D:practisephpdesignpsr0index.php on line 8
Add __toString method in Object.php
Copy code The code is as follows:
namespace Common;
class Object{
Function __toString() {
return __CLASS__;
}
}
④ __invoke: When a PHP object is executed as a function, this magic method will be called back
index.php
Copy code The code is as follows:
define('BASEDIR',__DIR__); //Define root directory constants
include BASEDIR.'/Common/Loader.php';
spl_autoload_register('\Common\Loader::autoload');
$obj = new CommonObject();
echo $obj("test");
Object.php
Copy code The code is as follows:
namespace Common;
class Object{
Function __invoke($param) {
var_dump($param);
return 'invoke';
}
}
Page output:
Copy code The code is as follows:
string 'test' (length=4)
invoke

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
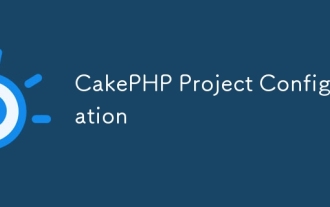
In this chapter, we will understand the Environment Variables, General Configuration, Database Configuration and Email Configuration in CakePHP.
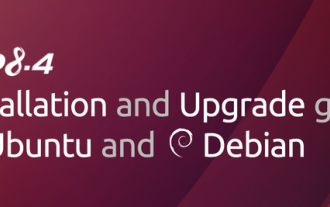
PHP 8.4 brings several new features, security improvements, and performance improvements with healthy amounts of feature deprecations and removals. This guide explains how to install PHP 8.4 or upgrade to PHP 8.4 on Ubuntu, Debian, or their derivati
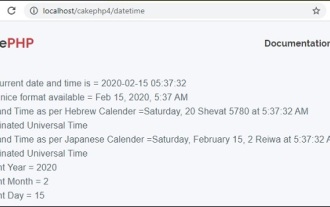
To work with date and time in cakephp4, we are going to make use of the available FrozenTime class.
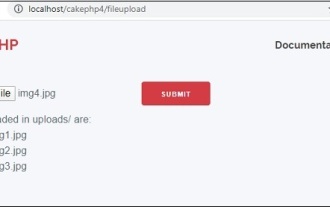
To work on file upload we are going to use the form helper. Here, is an example for file upload.
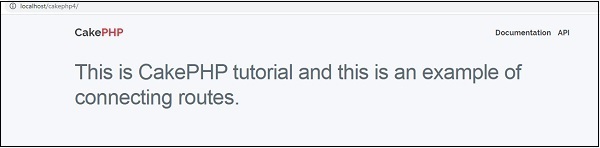
In this chapter, we are going to learn the following topics related to routing ?
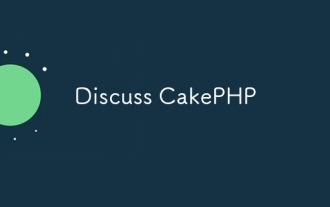
CakePHP is an open-source framework for PHP. It is intended to make developing, deploying and maintaining applications much easier. CakePHP is based on a MVC-like architecture that is both powerful and easy to grasp. Models, Views, and Controllers gu
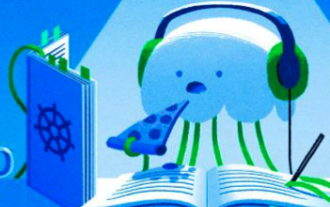
Visual Studio Code, also known as VS Code, is a free source code editor — or integrated development environment (IDE) — available for all major operating systems. With a large collection of extensions for many programming languages, VS Code can be c
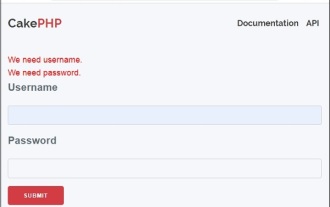
Validator can be created by adding the following two lines in the controller.
