PHP implements hash table_PHP tutorial
PHP implements hash table
//A simple hash table implementation. . . .
<?php class hashTable { private $collection; private $size = 100; //初始化哈希表的大小 public function __construct($size='') { $bucketsSize = is_int($size)?$size:$this->size; $this->collection = new SplFixedArray($bucketsSize); } //生成散列值,作为存储数据的位置 private function _hashAlgorithm($key) { $length = strlen($key); $hashValue = 0; for($i=0; $i<$length; $i++) { $hashValue += ord($key[$i]); } return ($hashValue%($this->size)); } //在相应的位置存储对应的值 public function set($key, $val) { $index = $this->_hashAlgorithm($key); $this->collection[$index] = $val; } //根据键生成散列值,进而找到对应的值 public function get($key) { $index = $this->_hashAlgorithm($key); return $this->collection[$index]; } //删除某个值,成功返回1,失败返回0 public function del($key) { $index = $this->_hashAlgorithm($key); if(isset($this->collection[$index])) { unset($this->collection[$index]); return 1; } else { return 0; } } //判断某个值是否存在,存在返回1, 不存在返回0 public function exist($key) { $index = $this->_hashAlgorithm($key); if($this->collection[$index]){ return 1; } else { return 0; } } //返回key的个数 public function size() { $size = 0; $length = count($this->collection); for($i=0; $i<$length; $i++) { if($this->collection[$i]) { $size++; } } return $size; } //返回value的序列 public function val() { $size = 0; $length = count($this->collection); for($i=0; $i<$length; $i++) { if($this->collection[$i]) { echo $this->collection[$i]."<br />"; } } } //排序输出 public function sort($type=1) { $length = count($this->collection); $temp = array(); for($i=0; $i<$length; $i++) { if($this->collection[$i]) { $temp[] = $this->collection[$i]; } } switch ($type) { case 1: //正常比较 sort($temp, SORT_REGULAR); break; case 2: //按照数字比较 sort($temp, SORT_NUMERIC); break; //按照字符串进行比较 case 3: sort($temp, SORT_STRING); break; //根据本地字符编码环境进行比较 case 4: sort($temp, SORT_LOCALE_STRING); break; } echo "<pre class="code">"; print_r($temp); } //逆序输出 public function rev($type=1) { $length = count($this->collection); $temp = array(); for($i=0; $i<$length; $i++) { if($this->collection[$i]) { $temp[] = $this->collection[$i]; } } switch ($type) { case 1: //正常比较 rsort($temp, SORT_REGULAR); break; case 2: //按照数字比较 rsort($temp, SORT_NUMERIC); break; //按照字符串进行比较 case 3: rsort($temp, SORT_STRING); break; //根据本地字符编码环境进行比较 case 4: rsort($temp, SORT_LOCALE_STRING); break; } echo "<pre class="code">"; print_r($temp); } } //简单的测试 $list = new hashTable(200); $list->set("zero", "zero compare"); $list->set("one", "first test"); $list->set("two", "second test"); $list->set("three", "three test"); $list->set("four", "fouth test"); echo $list->val(); echo "after sorted : <br />"; $list->rev(3);

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


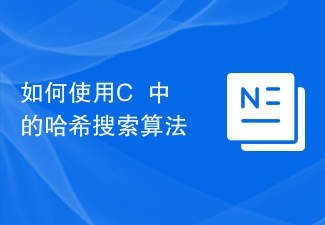
How to use the hash search algorithm in C++ The hash search algorithm is an efficient search and storage technology. It converts keywords into a fixed-length index through a hash function, and then uses this index in the data structure Search. In C++, we can implement hash search algorithms by using hash containers and hash functions from the standard library. This article will introduce how to use the hash search algorithm in C++ and provide specific code examples. Introducing header files and namespaces First, before using the hash search algorithm in C++
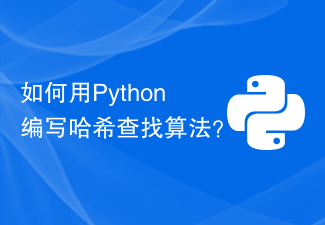
How to write a hash lookup algorithm in Python? Hash search algorithm, also known as hash search algorithm, is a data search method based on hash table. Compared with traditional search algorithms such as linear search and binary search, the hash search algorithm has higher search efficiency. In Python, we can use a dictionary to implement a hash table and then implement a hash lookup. The basic idea of the hash search algorithm is to convert the keyword to be searched into an index value through a hash function, and then search it in the hash table based on the index value.
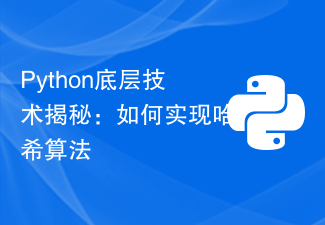
Revealing the underlying technology of Python: How to implement the hash algorithm, specific code examples are required Summary: The hash algorithm is one of the commonly used technologies in the computer field and is used to quickly determine the unique identification of data. As a high-level language, Python provides many built-in hash functions, such as the hash() function and the implementation of various hash algorithms. This article will reveal the principles of hashing algorithms and the details of Python's underlying implementation, and provide specific code examples. Introduction to Hash Algorithm Hash algorithm, also known as hash algorithm, is a method of converting data of any length into
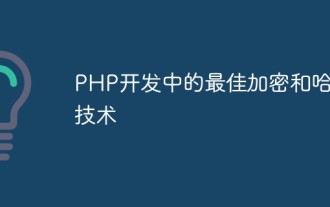
In today's digital age, with the development of the Internet and the increasing importance of information, data confidentiality and security have become increasingly important. To ensure that data is not stolen or tampered with during transmission, PHP developers often use encryption and hashing techniques to protect sensitive data. This article will introduce the most commonly used encryption and hashing technologies in PHP development, as well as their advantages and disadvantages. 1. Encryption technology Encryption is a technology that protects data security. It uses algorithms to convert data into meaningless forms. Only the person holding the key can restore it to readable
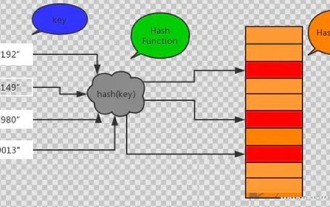
When understanding Bitcoin investment and blockchain technology, hash algorithms can be said to appear frequently. It is said in the currency circle that hip-hop has hip-hop and algorithms have hashes. As for the word "algorithm", it is currently used vaguely by domestic users. Sometimes it refers to the consensus mechanism, and sometimes it refers to the specific Hash algorithm. As a blockchain algorithm, the Hash algorithm has always been obscure to the general public. So, what is Hash algorithm? Hash algorithm? Next, the editor of the currency circle will give you a simple explanation of what a hash algorithm is? I hope that investors can understand the hash algorithm after reading this article. What is a hash algorithm? Hash is transliterated from "Hash", also known as "hash". Essentially a computer program that accepts any
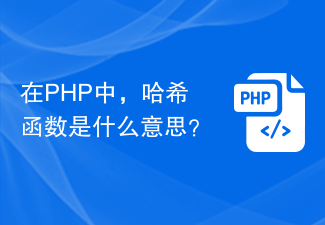
A hash function is any function that can be used to map data of any size to data of a fixed size. The value returned by a hash function is called a hash value, hash code, digest, or simply hash. Syntax stringhash(string$algo,string$data[,bool$raw_output=FALSE]) Parameter algo The name of the selected hash algorithm (such as "md5", "sha256", "haval160,4", etc.) data to be hashed information. When raw_output is set to TRUE, raw binary data is output. FALSE outputs lowercase hexadecimal. Example<?php  
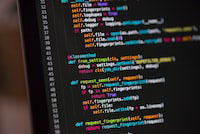
Introduction In today's fast-paced and connected digital world, ensuring high availability of applications is critical. Load balancing technology enables applications to distribute incoming traffic across multiple servers, improving performance and reliability. PHP provides support for a range of load balancing technologies, each with its own unique advantages and limitations. Round Robin Round Robin is a simple and effective load balancing technique that distributes requests to a server pool in order. This approach is easy to implement and ensures that requests are evenly distributed among servers. $servers=array("server1","server2","server3");$index=0;while(true)
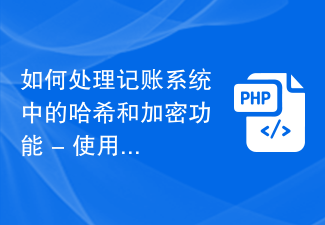
How to handle hashing and encryption functions in accounting systems - Development methods for hashing and encryption using PHP Introduction: With the advent of the digital age, the security of various information systems has become more and more important. When designing and developing accounting systems, protecting user privacy data is crucial. Among them, the use of hashing and encryption functions can effectively protect users' sensitive information. This article will introduce how to use PHP to implement hashing and encryption functions in accounting systems, and provide specific code examples. 1. Implementation of hash function Hash is a one-way encryption algorithm
