


SMS verification code sending class with IMEI restriction implemented in PHP_PHP tutorial
The IMEI restricted SMS verification code sending class implemented by php
?
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 98 99 100 101 102 103 104 105 106 107 108 109 110 111 112 113 114 115 116 117 118 119
|
class Api_Sms{ const EXPIRE_SEC = 1800; // Expiration interval const RESEND_SEC = 60; // Resend interval const ONE_DAY_FREQ = 5; // Number of times a day to send text messages to the same mobile phone number const ONE_DAY_IMEI_COUNT = 3; // Number of IMEIs sent to the same mobile phone number every day
public $error = array();
/** * Send verification code to the specified mobile phone number * @param $mobile * @param $imei * @return bool */ public function sendVerifyCode($mobile, $imei) { if(!$this->isMobile($mobile)) { $this->error = array('code' => -1, 'msg' => 'This mobile phone number is very strange, please enter it correctly and try again'); return false; }
$redis = Api_Common::redis(); $vcKey = 'VC_'.$mobile; $limitKey = 'VC_LIMIT_'.$mobile;
//Verification code resend limit $data = json_decode($redis->get($vcKey), true); if($data && time() < $data['resend_expire']) { $this->error = array('code' => -1, 'msg' => 'The message has been sent within 1 minute, please wait patiently'); return false; }
//Mobile phone number and IMEI restrictions $sendCnt = $redis->zScore($limitKey, $imei); if($sendCnt && $sendCnt >= self::ONE_DAY_FREQ) { $this->error = array('code' => -1, 'msg' => 'Didn't receive the text message? Please wait or check whether the text message has been blocked'); return false; } $imeiCnt = $redis->zCard($limitKey); if($imeiCnt >= self::ONE_DAY_IMEI_COUNT && !$sendCnt) { $this->error = array('code' => -1, 'msg' => 'Verification code sending device limit exceeded'); return false; }
//Get verification code if(!$data) { $vc = strval(rand(100000, 999999)); $data = array('vc' => $vc, 'resend_expire' => 0); $redis->set($vcKey, json_encode($data)); $redis->expire($vcKey, self::EXPIRE_SEC); // Set the verification code expiration time } $vc = $data['vc'];
$content = 'Security verification code:'.$vc; $result = $this->send($mobile, $content); if($result) { //Reset resend time limit $data['resend_expire'] = time() self::RESEND_SEC; $ttl = $redis->ttl($vcKey); $redis->set($vcKey, json_encode($data)); $redis->expire($vcKey, $ttl);
//Set mobile phone number and IMEI restrictions $redis->zIncrBy($limitKey, 1, $imei); $redis->expireAt($limitKey, strtotime(date('Y-m-d',strtotime(' 1 day')))); } return $result; }
/** * Send SMS to designated mobile phone number * @param $mobile * @param $content * @return bool */ public function send($mobile, $content){ //TODO call specific service provider API return true; }
/** * Determine whether it is a legal mobile phone number * @param $mobile * @return bool */ private function isMobile($mobile) { if(preg_match('/^1d{10}$/', $mobile)) return true; return false; }
/** * Verify SMS verification code * @param $mobile * @param $vc * @return bool */ public function checkVerifyCode($mobile, $vc) { $vcKey = 'VC_'.$mobile; $vcData = json_decode(Api_Common::redis()->get($vcKey), true); if($vcData && $vcData['vc'] === $vc) { return true; } return false; }
/** * Clear verification code * @param $mobile */ public function cleanVerifyCode($mobile) { $redis = Api_Common::redis(); $vcKey = 'VC_'.$mobile; $limitKey = 'VC_LIMIT_'.$mobile; $redis->del($vcKey); $redis->del($limitKey); } } |
Pay additional SMS verification code implemented by other netizens
?
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 |
/*-------------------------------- Function: China SMS Network PHP HTTP interface to send SMS Modification date: 2009-04-08 Instructions: http://http.c123.com/tx/?uid=user account&pwd=MD5-digit 32-digit password&mobile=number&content=content Status: 100 sent successfully 101 Verification failed 102 Insufficient SMS 103 Operation failed 104 illegal characters 105 Too much content 106 Too Many Numbers 107 frequency is too fast 108 number content is empty 109 Account Freeze 110 Frequent sending of single messages is prohibited 111 system tentatively sends 112 number is incorrect 120 System Upgrade --------------------------------*/ $uid = '9999'; //User account $pwd = '9999'; //Password $mobile = '13912341234,13312341234,13512341234,02122334444'; //Number $content = 'China SMS Network PHP HTTP interface'; //Content //Send immediately $res = sendSMS($uid,$pwd,$mobile,$content); echo $res;
//Send regularly /* $time = '2010-05-27 12:11'; $res = sendSMS($uid,$pwd,$mobile,$content,$time); echo $res; */ function sendSMS($uid,$pwd,$mobile,$content,$time='',$mid='') { $http = 'http://http.c123.com/tx/'; $data = array ( 'uid'=>$uid, //User account 'pwd'=>strtolower(md5($pwd)), //MD5 digit 32 password 'mobile'=>$mobile, //Number 'content'=>$content, //Content 'time'=>$time, //Send regularly 'mid'=>$mid //Sub extension number ); $re= postSMS($http,$data); //Submit via POST if( trim($re) == '100' ) { return "Sent successfully!"; } else { return "Sending failed! Status:".$re; } }
function postSMS($url,$data='') { $row = parse_url($url); $host = $row['host']; $port = $row['port'] ? $row['port']:80; $file = $row['path']; while (list($k,$v) = each($data)) { $post .= rawurlencode($k)."=".rawurlencode($v)."&"; //转URL标准码 } $post = substr( $post , 0 , -1 ); $len = strlen($post); $fp = @fsockopen( $host ,$port, $errno, $errstr, 10); if (!$fp) { return "$errstr ($errno)n"; } else { $receive = ''; $out = "POST $file HTTP/1.1rn"; $out .= "Host: $hostrn"; $out .= "Content-type: application/x-www-form-urlencodedrn"; $out .= "Connection: Closern"; $out .= "Content-Length: $lenrnrn"; $out .= $post; fwrite($fp, $out); while (!feof($fp)) { $receive .= fgets($fp, 128); } fclose($fp); $receive = explode("rnrn",$receive); unset($receive[0]); return implode("",$receive); } } ?> |
以上所述就是本文的全部内容了,希望大家能够喜欢。

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
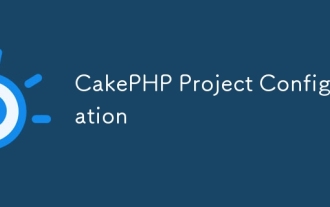
In this chapter, we will understand the Environment Variables, General Configuration, Database Configuration and Email Configuration in CakePHP.
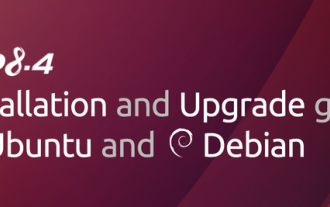
PHP 8.4 brings several new features, security improvements, and performance improvements with healthy amounts of feature deprecations and removals. This guide explains how to install PHP 8.4 or upgrade to PHP 8.4 on Ubuntu, Debian, or their derivati
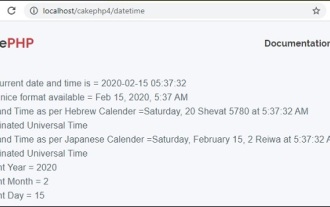
To work with date and time in cakephp4, we are going to make use of the available FrozenTime class.
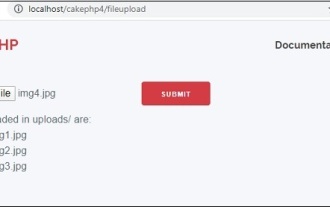
To work on file upload we are going to use the form helper. Here, is an example for file upload.
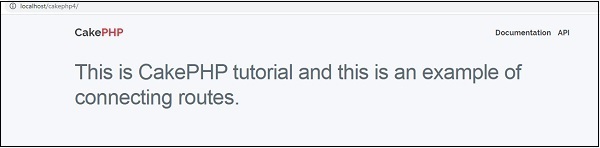
In this chapter, we are going to learn the following topics related to routing ?
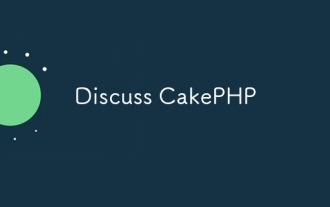
CakePHP is an open-source framework for PHP. It is intended to make developing, deploying and maintaining applications much easier. CakePHP is based on a MVC-like architecture that is both powerful and easy to grasp. Models, Views, and Controllers gu
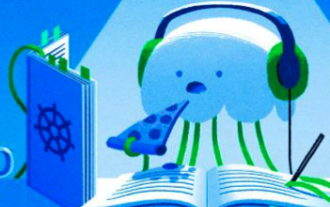
Visual Studio Code, also known as VS Code, is a free source code editor — or integrated development environment (IDE) — available for all major operating systems. With a large collection of extensions for many programming languages, VS Code can be c
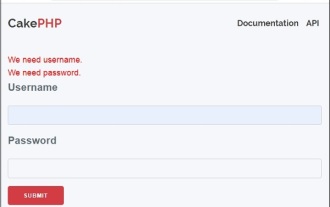
Validator can be created by adding the following two lines in the controller.
