How to use JSON in php, how to use phpJSON_PHP tutorial
How to use JSON in php, how to use phpJSON
Starting from version 5.2, PHP natively provides json_encode() and json_decode() functions. The former is used for encoding, and the latter is used for decoding.
json_encode()
This function is mainly used to convert arrays and objects into json format.
Copy code The code is as follows:
$arr = array ('a'=>'a','b'=>'b','c'='c','d'=>'d','e'='e' );
echo json_encode($arr);
json only accepts UTF-8 encoded characters, and the parameters of json_encode() must be UTF-8 encoded.
class person { public $name; public $age; public $height; function __construct($name,$age,$height) { $this->name = $name; $this->age = $age; $this->height = $height; } } $obj = new person("zhangsan",20,100); $foo_json = json_encode($obj); echo $foo_json;
When the attributes in the class are private variables, they will not be output.
json_decode()
This function is used to convert json text into the corresponding PHP data structure.
Copy code The code is as follows:
$json = '{"a":"hello","b":"world","c":"zhangsan","d":20,"e":170}';
var_dump(json_decode($json));
Output result:
Convert to array:
Copy code The code is as follows:
$json = '{"a":"hello","b":"world","c":"zhangsan","d":20,"e":170}';
var_dump(json_decode($json,ture));
The above is the entire content of this article, I hope you all like it.
http://www.bkjia.com/PHPjc/992544.html

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


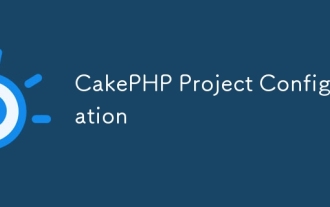
In this chapter, we will understand the Environment Variables, General Configuration, Database Configuration and Email Configuration in CakePHP.
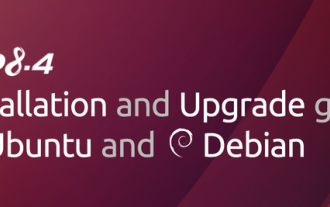
PHP 8.4 brings several new features, security improvements, and performance improvements with healthy amounts of feature deprecations and removals. This guide explains how to install PHP 8.4 or upgrade to PHP 8.4 on Ubuntu, Debian, or their derivati
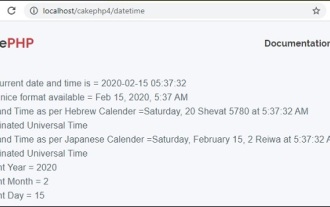
To work with date and time in cakephp4, we are going to make use of the available FrozenTime class.
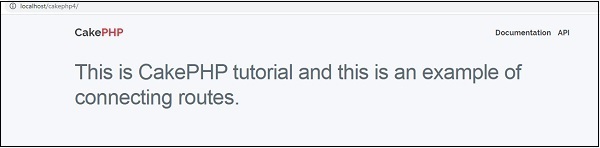
In this chapter, we are going to learn the following topics related to routing ?
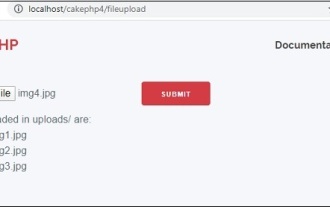
To work on file upload we are going to use the form helper. Here, is an example for file upload.
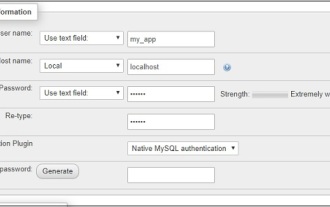
Working with database in CakePHP is very easy. We will understand the CRUD (Create, Read, Update, Delete) operations in this chapter.
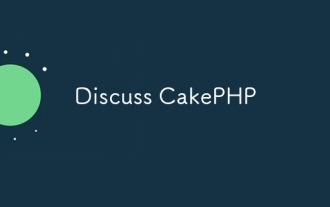
CakePHP is an open-source framework for PHP. It is intended to make developing, deploying and maintaining applications much easier. CakePHP is based on a MVC-like architecture that is both powerful and easy to grasp. Models, Views, and Controllers gu
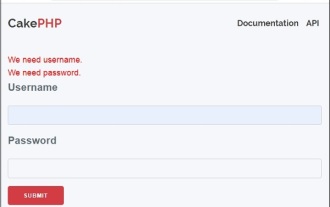
Validator can be created by adding the following two lines in the controller.
