


Comparative analysis of database connection methods pdo and mysqli in php, pdomysqli_PHP tutorial
Comparative analysis of pdo and mysqli database connection methods in php, pdomysqli
1) Overall comparison
|
MySQLi | ||||||||||||||||||||||||||||
Database support | 12 different databases supported | Support MySQL | |||||||||||||||||||||||||||
API | OOP | OOP + Process | |||||||||||||||||||||||||||
Connection | Easy | Easy | |||||||||||||||||||||||||||
Named parameters | Support | Not supported | |||||||||||||||||||||||||||
Object mapping support | Support | Support | |||||||||||||||||||||||||||
Prepared statements (Client) |
Support | Not supported | |||||||||||||||||||||||||||
Performance | Fast | Fast | |||||||||||||||||||||||||||
Support stored procedures | Support | Support |
2 Connection methods
Let’s first look at how the two connect to the database:
// PDO
$pdo = new PDO("mysql:host=localhost;dbname=database", 'username', 'password');
// mysqli, process-oriented approach
$mysqli = mysqli_connect('localhost','username','password','database');
// mysqli, object-oriented
$mysqli = new mysqli('localhost','username','password','database');
3 database support
PDO supports multiple databases, but MYSQLI only supports MYSQL
4 named parameter name parameter
PDO method:
$params = array(':username' => 'test', ':email' => $mail, ':last_login' => time() - 3600);
$pdo->prepare('
SELECT * FROM users
WHERE username = :username
AND email = :email
AND last_login > :last_login');
But MYSQLI is a bit more troublesome. It does not support this, so it can only:
$query = $mysqli->prepare('
SELECT * FROM users
WHERE username = ?
AND email = ?
AND last_login > ?');
$query->bind_param('sss', 'test', $mail, time() - 3600);
$query->execute();
In this case, it would be troublesome and inconvenient to order the question marks one by one.
5 ORM mapping support
For example, there is a class user, as follows:
class User
{
Public $id;
Public $first_name;
Public $last_name;
Public function info()
{
return '#' . $this->id . ': ' . $this->first_name . ' ' . $this->last_name;
}
}
$query = "SELECT id, first_name, last_name FROM users";
// PDO
$result = $pdo->query($query);
$result->setFetchMode(PDO::FETCH_CLASS, 'User');
while ($user = $result->fetch())
{
echo $user->info() . "n";
}
MYSQLI uses a process-oriented approach:
if ($result = mysqli_query($mysqli, $query)) {
while ($user = mysqli_fetch_object($result, 'User')) {
echo $user->info()."n";
}
}
MYSQLI adopts a process-oriented approach:
// MySQLi, object oriented way
if ($result = $mysqli->query($query)) {
while ($user = $result->fetch_object('User')) {
echo $user->info()."n";
}
}
6 Preventing SQL injection:
PDO Manual Settings
$username = PDO::quote($_GET['username']);
$pdo->query("SELECT * FROM users WHERE username = $username");
Use mysqli
$username = mysqli_real_escape_string($_GET['username']);
$mysqli->query("SELECT * FROM users WHERE username = '$username'");
7 preparestament
PDO method:
$pdo->prepare('SELECT * FROM users WHERE username = :username');
$pdo->execute(array(':username' => $_GET['username']));
MYSQLI:
$query = $mysqli->prepare('SELECT * FROM users WHERE username = ?');
$query->bind_param('s', $_GET['username']);
$query->execute();
Have you gained a new understanding of PHP’s two linking methods, PDO and mysqli, through this article? I hope this article can be helpful to you.

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
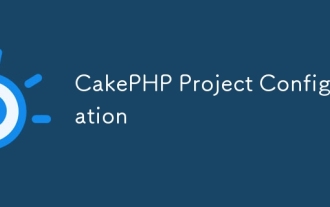
In this chapter, we will understand the Environment Variables, General Configuration, Database Configuration and Email Configuration in CakePHP.
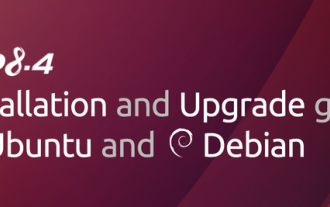
PHP 8.4 brings several new features, security improvements, and performance improvements with healthy amounts of feature deprecations and removals. This guide explains how to install PHP 8.4 or upgrade to PHP 8.4 on Ubuntu, Debian, or their derivati
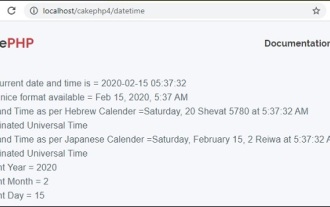
To work with date and time in cakephp4, we are going to make use of the available FrozenTime class.
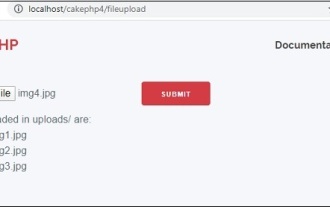
To work on file upload we are going to use the form helper. Here, is an example for file upload.
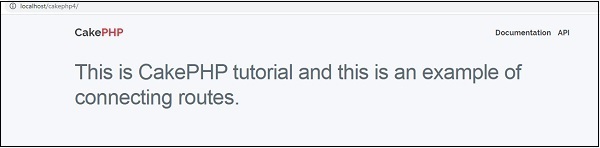
In this chapter, we are going to learn the following topics related to routing ?
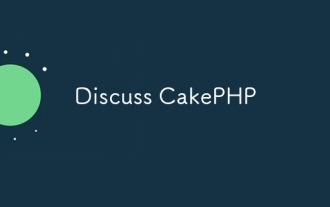
CakePHP is an open-source framework for PHP. It is intended to make developing, deploying and maintaining applications much easier. CakePHP is based on a MVC-like architecture that is both powerful and easy to grasp. Models, Views, and Controllers gu
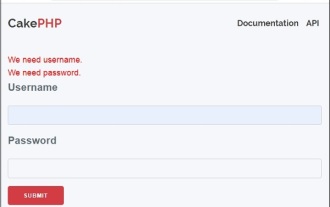
Validator can be created by adding the following two lines in the controller.
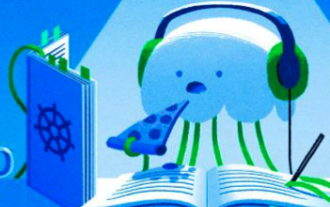
Visual Studio Code, also known as VS Code, is a free source code editor — or integrated development environment (IDE) — available for all major operating systems. With a large collection of extensions for many programming languages, VS Code can be c
