9 classic PHP code snippets to share, _PHP tutorial
9 classic PHP code snippets to share,
1. Check whether the email has been read
When you send an email, you may want to know whether the email has been read by the other party. Here’s a very interesting snippet of code that displays the actual date and time the record was read by the other party’s IP address.
error_reporting(0);
Header("Content-Type: image/jpeg");
//Get IP
if (!empty($_SERVER['HTTP_CLIENT_IP']))
{
$ip=$_SERVER['HTTP_CLIENT_IP'];
}
elseif (!empty($_SERVER['HTTP_X_FORWARDED_FOR']))
{
$ip=$_SERVER['HTTP_X_FORWARDED_FOR'];
}
else
{
$ip=$_SERVER['REMOTE_ADDR'];
}
//Time
$actual_time = time();
$actual_day = date('Y.m.d', $actual_time);
$actual_day_chart = date('d/m/y', $actual_time);
$actual_hour = date('H:i:s', $actual_time);
//GET Browser
$browser = $_SERVER['HTTP_USER_AGENT'];
//LOG
$myFile = "log.txt";
$fh = fopen($myFile, 'a+');
$stringData = $actual_day . ' ' . $actual_hour . ' ' . $ip . ' ' . $browser . ' ' . "rn";
fwrite($fh, $stringData);
fclose($fh);
//Generate Image (Es. dimesion is 1x1)
$newimage = ImageCreate(1,1);
$grigio = ImageColorAllocate($newimage,255,255,255);
ImageJPEG($newimage);
ImageDestroy($newimage);
?>
2. Extract keywords from web pages
A great code snippet to easily extract keywords from web pages.
$meta = get_meta_tags('http://www.emoticode.net/');
$keywords = $meta['keywords'];
// Split keywords
$keywords = explode(',', $keywords );
// Trim them
$keywords = array_map( 'trim', $keywords );
// Remove empty values
$keywords = array_filter( $keywords );
print_r( $keywords );
3. Find all links on the page
Using DOM, you can easily grab links from any page. The code example is as follows:
$html = file_get_contents('http://www.example.com');
$dom = new DOMDocument();
@$dom->loadHTML($html);
// grab all the on the page
$xpath = new DOMXPath($dom);
$hrefs = $xpath->evaluate("/html/body//a");
for ($i = 0; $i < $hrefs->length; $i++) {
$href = $hrefs->item($i);
$url = $href->getAttribute('href');
echo $url.'
';
}
4. Automatically convert URL and jump to hyperlink
In WordPress, if you want to automatically convert the URL and jump to the hyperlinked page, you can use the built-in function make_clickable() to perform this operation. If you want to operate the program outside of WordPress, then you can refer to the wp-includes/formatting.php source code.
function _make_url_clickable_cb($matches) {
$ret = '';
$url = $matches[2];
if ( empty($url) )
return $matches[0];
// removed trailing [.,;:] from URL
if ( in_array(substr($url, -1), array('.', ',', ';', ':')) === true ) {
$ret = substr($url, -1);
$url = substr($url, 0, strlen($url)-1);
}
return $matches[1] . "$url" . $ret;
}
function _make_web_ftp_clickable_cb($matches) {
$ret = '';
$dest = $matches[2];
$dest = 'http://' . $dest;
if ( empty($dest) )
return $matches[0];
// removed trailing [,;:] from URL
if ( in_array(substr($dest, -1), array('.', ',', ';', ':')) === true ) {
$ret = substr($dest, -1);
$dest = substr($dest, 0, strlen($dest)-1);
}
return $matches[1] . "$dest" . $ret;
}
function _make_email_clickable_cb($matches) {
$email = $matches[2] . '@' . $matches[3];
return $matches[1] . "$email";
}
function make_clickable($ret) {
$ret = ' ' . $ret;
// in testing, using arrays here was found to be faster
$ret = preg_replace_callback('#([s>])([w]+?://[w\x80-\xff#$%&~/.-;:=,?@[]+]*)#is', '_make_url_clickable_cb', $ret);
$ret = preg_replace_callback('#([s>])((www|ftp).[w\x80-\xff#$%&~/.-;:=,?@[]+]*)#is', '_make_web_ftp_clickable_cb', $ret);
$ret = preg_replace_callback('#([s>])([.0-9a-z_+-]+)@(([0-9a-z-]+.)+[0-9a-z]{2,})#i', '_make_email_clickable_cb', $ret);
// this one is not in an array because we need it to run last, for cleanup of accidental links within links
$ret = preg_replace("#(]+?>|>))]+?>([^>]+?)#i", "$1$3", $ret);
$ret = trim($ret);
return $ret;
}
五、创建数据URL
数据URL可以直接嵌入到HTML/CSS/JS中,以节省大量的 HTTP请求。 下面的这段代码可利用$file轻松创建数据URL。
function data_uri($file, $mime) {
$contents=file_get_contents($file);
$base64=base64_encode($contents);
echo "data:$mime;base64,$base64";
}
六、从服务器上下载&保存一个远程图片
当你在搭建网站时,从远程服务器下载某张图片并且将其保存在自己的服务器上,这一操作会经常用到。代码如下:
$image = file_get_contents('http://www.url.com/image.jpg');
file_put_contents('/images/image.jpg', $image); //Where to save the image
七、移除Remove Microsoft Word HTML Tag
当你使用Microsoft Word会创建许多Tag,比如font,span,style,class等。这些标签对于Word本身而言是非常有用的,但是当你从Word粘贴至网页时,你会发现很多无用的Tag。因此,下面的这段代码可帮助你删除所有无用的Word HTML Tag。
function cleanHTML($html) {
///
/// Removes all FONT and SPAN tags, and all Class and Style attributes.
/// Designed to get rid of non-standard Microsoft Word HTML tags.
///
// start by completely removing all unwanted tags
$html = ereg_replace("<(/)?(font|span|del|ins)[^>]*>","",$html);
// then run another pass over the html (twice), removing unwanted attributes
$html = ereg_replace("<([^>]*)(class|lang|style|size|face)=("[^"]*"|'[^']*'|[^>] +)([^>]*)>","<1>",$html);
$html = ereg_replace("<([^>]*)(class|lang|style|size|face)=("[^"]*"|'[^']*'|[^>] +)([^>]*)>","<1>",$html);
return $html
}
[code]
8. Detect browser language
If your website has multiple languages, you can use this code as the default language to detect the browser language. This code will return the initial language used by the browser client.
[code]
function get_client_language($availableLanguages, $default='en'){
If (isset($_SERVER['HTTP_ACCEPT_LANGUAGE'])) {
$langs=explode(',',$_SERVER['HTTP_ACCEPT_LANGUAGE']);
foreach ($langs as $value){
$choice=substr($value,0,2);
If(in_array($choice, $availableLanguages)){
return $choice;
}
}
}
Return $default;
}
9. Display the number of Facebook fans
If you have an internally linked Facebook page on your website or blog, you may want to know how many fans you have. This code will help you check the number of Facebook fans. Remember, don’t forget to add this code on the second line of your page ID.
$page_id = "YOUR PAGE-ID";
$xml = @simplexml_load_file("http://api.facebook.com/restserver.php?method=facebook.fql.query&query=SELECT%20fan_count%20FROM%20page%20WHERE%20page_id=".$page_id."") or die ("a lot");
$fans = $xml->page->fan_count;
echo $fans;
?>
The above 9 super practical and classic PHP codes are very easy to use. Friends can use them as a reference and use them in their own projects with slight modifications.

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
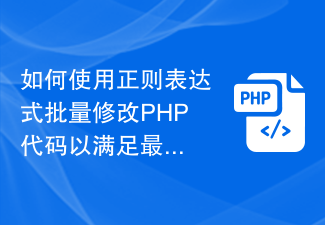
How to use regular expressions to batch modify PHP code to meet the latest code specifications? Introduction: As time goes by and technology develops, code specifications are constantly updated and improved. During the development process, we often need to modify old code to comply with the latest code specifications. However, manual modification can be a tedious and time-consuming task. In this case, regular expressions can be a powerful tool. Using regular expressions, we can modify the code in batches and automatically meet the latest code specifications. 1. Preparation: before using
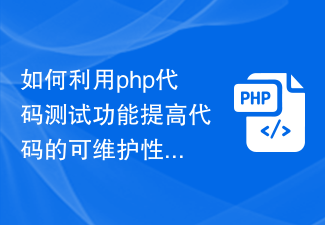
How to use the PHP code testing function to improve the maintainability of the code. In the software development process, the maintainability of the code is a very important aspect. A maintainable code means that it is easy to understand, easy to modify, and easy to maintain. Testing is a very effective means of improving code maintainability. This article will introduce how to use PHP code testing function to achieve this purpose, and provide relevant code examples. Unit testing Unit testing is a testing method commonly used in software development to verify the smallest testable unit in the code. in P
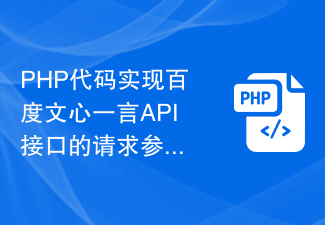
The PHP code implements the request parameter encryption and decryption processing of Baidu Wenxin Yiyan API interface. Hitokoto is a service that provides access to random sentences. Baidu Wenxin Yiyan API is one of the interfaces that developers can call. In order to ensure data security, we can encrypt the request parameters and decrypt the response after receiving the response. The following is an example of PHP code implementing the request parameter encryption and decryption processing of Baidu Wenxinyiyan API interface: <?phpfunction
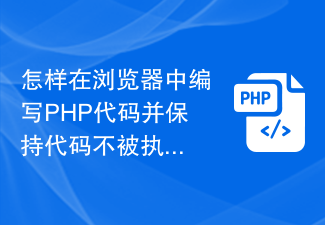
How to write PHP code in the browser and keep the code from being executed? With the popularization of the Internet, more and more people have begun to come into contact with web development, and learning PHP has also attracted more and more attention. PHP is a scripting language that runs on the server side and is often used to write dynamic web pages. However, during the exercise phase, we want to be able to write PHP code in the browser and see the results, but we don't want the code to be executed. So, how to write PHP code in the browser and keep it from being executed? This will be described in detail below. first,
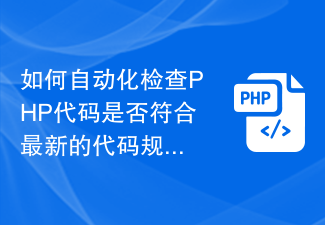
How to use tools to automatically check whether PHP code complies with the latest coding standards? Introduction: In the software development process, we often need to follow certain code specifications to ensure the readability, maintainability and scalability of the code. However, manually checking code specifications is a tedious and error-prone task. In order to improve efficiency and reduce errors, we can use some tools to automatically check code specifications. In this article, I will introduce how to use some popular tools to automatically check whether PHP code complies with the latest coding standards. 1. PH
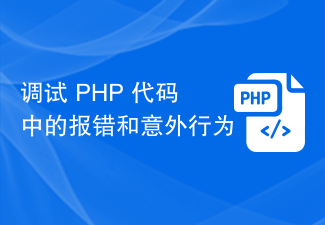
Title: Debugging PHP Code: Parsing Errors and Unexpected Behavior Introduction: Debugging is an important skill when developing PHP applications. When our code reports errors or unexpected behavior, we need to quickly locate the problem and fix it. This article will explore some common PHP errors and unexpected behaviors, and give corresponding code examples and debugging methods. 1. Grammatical errors Grammatical errors are one of the most common errors. In PHP, syntax errors can cause the entire script to fail to execute properly. Here is a sample code: <?php
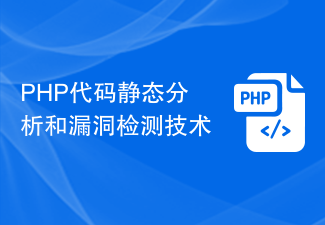
Introduction to PHP code static analysis and vulnerability detection technology: With the development of the Internet, PHP, as a very popular server-side scripting language, is widely used in website development and dynamic web page generation. However, due to the flexible and unstandardized nature of PHP syntax, security vulnerabilities are easily introduced during the development process. In order to solve this problem, PHP code static analysis and vulnerability detection technology came into being. 1. Static analysis technology Static analysis technology refers to analyzing the source code and using static rules to identify potential security issues before the code is run.
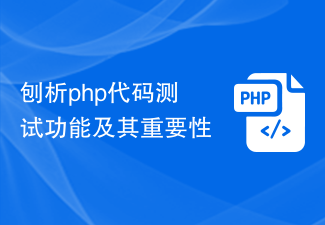
Analyzing the PHP code testing function and its importance Preface: In the software development process, code testing is an indispensable link. By testing the code, potential bugs and errors can be effectively discovered and resolved, and the quality and stability of the code can be improved. In PHP development, testing functions is also important. This article will delve into the function and importance of PHP code testing, and illustrate it with examples. 1. Functional unit testing (UnitTesting) of PHP code testing Unit testing is the most common testing method
