


Effective method to delete cookies in php, delete cookies in php_PHP tutorial
php effective way to delete cookies, php delete cookies
Effective way to delete cookies in php
Instructions on deleting cookies begin-----
bool setcookie ( string name [, string value [, int expire [, string path [, string domain [, bool secure]]]]] )
To delete a cookie, you need to ensure that its expiration date is in the past to trigger the browser's deletion mechanism.
The following example illustrates how to delete the cookie just set:
//Set the expiration time to one hour ago
setcookie("TestCookie",
"", time() - 3600);
setcookie("TestCookie", "", time() - 3600, "/~rasmus/",
".utoronto.ca", 1);
?>
-----End of instructions on deleting cookies-----
The way to delete a cookie is to set the validity period of the cookie to before the current time, which is what almost all PHP programmers do.
Later, a friend who was new to PHP told me that he wanted to set the value of a cookie to empty in the program, but the cookie was deleted directly. My first reaction at the time was that I didn’t believe it, so I tested it
:
setcookie("testcookie",
'');
print_r($_COOKIE);
The result is that the entire $_COOKIE array is empty, not just $_COOKIE['testcookie']. So I used winsock to capture the packet and observed the returned http header. I found that the http header turned out to be "Set-Cookie:
testcookie=deleted; expires=Mon, 18-Jun-2007 02:42:33
GMT", which means "setcookie("testcookie",
'');" indeed deletes the cookie testcookie directly, and there is no explanation at all in the PHP manual about this situation.
Finally read the php source code and finally found the truth (this is the benefit of open source, if there is any unclear inside story, just check the source code directly).
The following code can be found near line 99 of ext/standard/head.c in the linux source package of php5.20:
if (value &&
value_len == 0) {
/*
* MSIE doesn't delete a cookie when you set
it to a null value
* so in order to force cookies to be deleted, even on
MSIE, we
* pick an expiry date 1 year and 1 second in the past
*/
time_t t = time(NULL) - 31536001;
dt = php_format_date("D,
d-M-Y H:i:s T", sizeof("D, d-M-Y H:i:s T")-1, t, 0 TSRMLS_CC);
sprintf(cookie, "Set-Cookie: %s=deleted; expires=%s", name, dt);
efree(dt);
} else {
sprintf(cookie, "Set-Cookie: %s=%s", name, value?
encoded_value : "");
if (expires > 0) {
strcat(cookie, ";
expires=");
dt = php_format_date("D, d-M-Y H:i:s T", sizeof("D, d-M-Y
H:i:s T")-1, expires, 0 TSRMLS_CC);
strcat(cookie, dt);
efree(dt);
}
}
The source code clearly shows “if (value && value_len ==
0)", when "value_len" is 0, "sprintf(cookie, "Set-Cookie: %s=deleted; expires=%s", name,
dt);" will send the http header to delete the cookie to the browser.
Finally we can draw the conclusion: use "setcookie($cookiename, '');" or "setcookie($cookiename, NULL);" will delete cookies, which of course is not in these manuals.
Source: http://www.111cn.net/phper/21/f0eace11b1229a0f2c7c54e3c1ea4654.htm

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
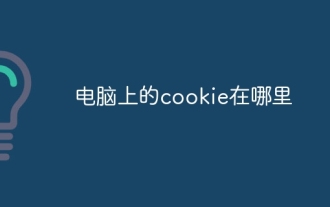
Cookies on your computer are stored in specific locations on your browser, depending on the browser and operating system used: 1. Google Chrome, stored in C:\Users\YourUsername\AppData\Local\Google\Chrome\User Data\Default \Cookies etc.
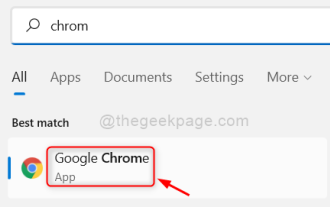
Many Windows users have recently encountered an unusual error called Roblox403 Forbidden Error while trying to access website URLs in Google Chrome browser. Even after restarting the Chrome app multiple times, they were unable to do anything. There could be several potential causes for this error, some of which we've outlined and listed below. Browsing history and other cache of Chrome and corrupted data Unstable internet connection Incorrect website URLs Extensions installed from third-party sources After considering all the above aspects, we have come up with some fixes that can help users resolve this issue. If you encounter the same problem, check out the solutions in this article. Fix 1
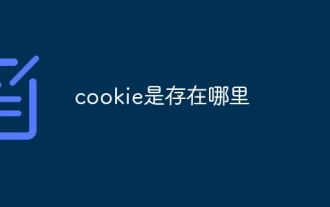
Cookies are usually stored in the cookie folder of the browser. Cookie files in the browser are usually stored in binary or SQLite format. If you open the cookie file directly, you may see some garbled or unreadable content, so it is best to use Use the cookie management interface provided by your browser to view and manage cookies.
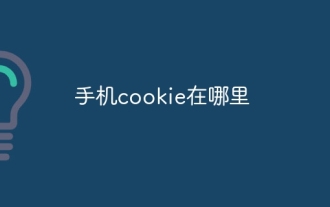
Cookies on the mobile phone are stored in the browser application of the mobile device: 1. On iOS devices, Cookies are stored in Settings -> Safari -> Advanced -> Website Data of the Safari browser; 2. On Android devices, Cookies Stored in Settings -> Site settings -> Cookies of Chrome browser, etc.
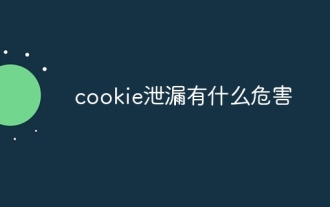
The dangers of cookie leakage include theft of personal identity information, tracking of personal online behavior, and account theft. Detailed introduction: 1. Personal identity information is stolen, such as name, email address, phone number, etc. This information may be used by criminals to carry out identity theft, fraud and other illegal activities; 2. Personal online behavior is tracked and analyzed through cookies With the data in the account, criminals can learn about the user's browsing history, shopping preferences, hobbies, etc.; 3. The account is stolen, bypassing login verification, directly accessing the user's account, etc.
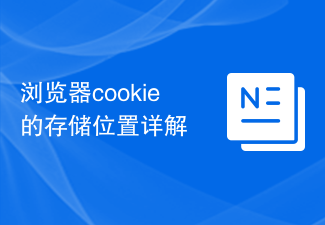
With the popularity of the Internet, we use browsers to surf the Internet have become a way of life. In the daily use of browsers, we often encounter situations where we need to enter account passwords, such as online shopping, social networking, emails, etc. This information needs to be recorded by the browser so that it does not need to be entered again the next time you visit. This is when cookies come in handy. What are cookies? Cookie refers to a small data file sent by the server to the user's browser and stored locally. It contains user behavior of some websites.
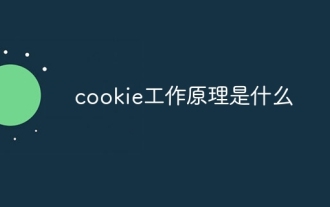
The working principle of cookies involves the server sending cookies, the browser storing cookies, and the browser processing and storing cookies. Detailed introduction: 1. The server sends a cookie, and the server sends an HTTP response header containing the cookie to the browser. This cookie contains some information, such as the user's identity authentication, preferences, or shopping cart contents. After the browser receives this cookie, it will be stored on the user's computer; 2. The browser stores cookies, etc.
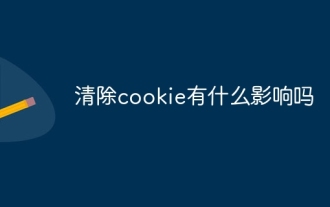
The effects of clearing cookies include resetting personalization settings and preferences, affecting ad experience, and destroying login status and password remembering functions. Detailed introduction: 1. Reset personalized settings and preferences. If cookies are cleared, the shopping cart will be reset to empty and products need to be re-added. Clearing cookies will also cause the login status on social media platforms to be lost, requiring re-adding. Enter your username and password; 2. It affects the advertising experience. If cookies are cleared, the website will not be able to understand our interests and preferences, and will display irrelevant ads, etc.
