


7 little-known but super practical PHP functions, little-known php_PHP tutorial
7 little-known but super practical PHP functions, little-known php
PHP has many built-in functions, most of which are widely used by programmers. But there are also some functions hidden in the corners. This article will introduce to you 7 functions that are little known but very useful. Programmers who have never used it may wish to come and take a look.
1.highlight_string()
When you need to display PHP code in a website, the highlight_string() function becomes very useful. This function outputs or returns a syntax-highlighted version of the given PHP code, using the colors defined in the PHP syntax highlighter.
Example:
1 2 3 |
<?php
highlight_string( '<?php phpinfo(); ?>' );
?>
|
2.str_word_count()
This function must pass a parameter and return the number of words according to the parameter type. As shown below:
1 2 3 4 |
<?php
$str = "How many words do I have?" ;
echo str_word_count ( $str ); //Outputs 6
?>
|
3.levenshtein()
This function mainly returns the Levenshtein distance between two strings. Levenshtein distance, also known as edit distance, refers to the minimum number of edit operations required between two strings to convert one into the other. Permitted editing operations include replacing one character with another, inserting a character, and deleting a character. This function is useful for finding typos submitted by users.
Example:
1 2 3 4 5 |
<?php
$str1 = "carrot" ;
$str2 = "carrrott" ;
echo levenshtein( $str1 , $str2 ); //Outputs 2
?>
|
4.get_defined_vars()
This function returns a multidimensional array containing a list of all defined variables, including environment variables, server variables and user-defined variables.
Example:
1 |
print_r(get_defined_vars());
|
5.escapeshellcmd()
This function is used to avoid special symbols in strings and prevent users from playing tricks to crack the server system. You can use this function with the exec() or system() functions, which can reduce the malicious destructive behavior of online users.
Example:
1 2 3 4 5 |
<?php
$command = './configure ' . $_POST [ 'configure_options' ];
$escaped_command = escapeshellcmd ( $command );
system( $escaped_command );
?>
|
6.checkdate()
This function can be used to check whether the date is valid, for example, the year ranges from 0 to 32767, the month ranges from 1 to December, and the day changes with the month and leap year.
Example:
1 2 3 4 5 6 7 |
<?php
var_dump( checkdate (12, 31, 2000));
var_dump( checkdate (2, 29, 2001));
//Output
//bool(true)
//bool(false)
?>
|
7.php_strip_whitespace()
This function can return the source code file with PHP comments and whitespace characters removed, which is useful for comparing the actual number of codes and the number of comments.
Example:
1 2 3 4 5 6 7 8 9 |
<?php
// PHP comment here
/*
* Another PHP comment
*/
echo php_strip_whitespace( __FILE__ );
// Newlines are considered whitespace, and are removed too:
do_nothing();
?>
|
Output result:
1 2 |
<?php
echo php_strip_whitespace( __FILE__ ); do_nothing(); ?>
|
Article from: Cats Who Code
You can pass a parameter to point it to this page~such as myinfo.php?act=xxx
Then this page will start to judge the value of act. If it is xxx, execute the function you want to execute
The more you practice, the happier you are. At the beginning of each issue, there is a very funny sketch, played by Wang Han, Mark and Chen Yingjun. Each one is borrowed from one
I once borrowed a skit from the exam and it was well received

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
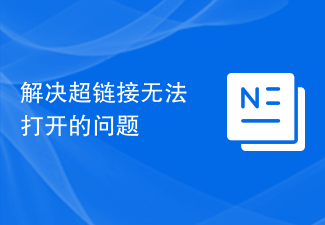
How to solve the problem that hyperlinks cannot be opened. With the rapid development of the Internet, hyperlinks have become an indispensable part of our daily lives. We often see and use hyperlinks on various platforms such as web pages, emails, and social media. However, sometimes we may encounter problems with hyperlinks not opening, which makes us confused and frustrated. In this article, we will discuss the reasons why hyperlinks cannot be opened and their solutions, hoping to help you solve this problem. First, let us understand the possible reasons why hyperlinks cannot be opened. The following are some common
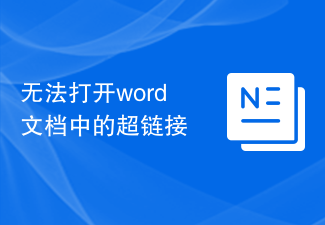
In recent years, with the continuous development of network technology, our lives are inseparable from various digital tools and the Internet. When processing documents, especially in writing, we often use word documents. However, sometimes we may encounter a difficult problem, that is, the hyperlink in the word document cannot be opened. This issue will be discussed below. First of all, we need to make it clear that hyperlinks refer to links added in word documents to other documents, web pages, directories, bookmarks, etc. When we click on these links, I
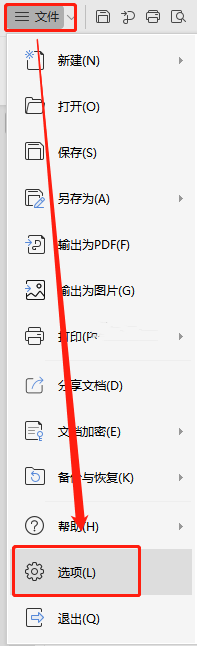
When many of our friends use WPS to edit content, they sometimes add hyperlinks to specific text. Recently, some friends have reported that WPS hyperlinks cannot be opened and cannot be clicked to access after using many methods. About this How to solve the problem? In this software tutorial, the editor will share the specific solutions, hoping to help the majority of users. Solution to WPS hyperlink not opening: Method 1: Move the mouse over the hyperlink to see if it becomes clickable. 1. Open the software, click "File" in the upper left corner of the page, and select "Options" in the menu below. 2. After entering the new interface, click "Edit&rdq" on the left
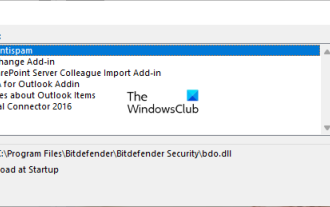
If you encounter freezing issues when inserting hyperlinks into Outlook, it may be due to unstable network connections, old Outlook versions, interference from antivirus software, or add-in conflicts. These factors may cause Outlook to fail to handle hyperlink operations properly. Fix Outlook freezes when inserting hyperlinks Use the following fixes to fix Outlook freezes when inserting hyperlinks: Check installed add-ins Update Outlook Temporarily disable your antivirus software and then try creating a new user profile Fix Office apps Program Uninstall and reinstall Office Let’s get started. 1] Check the installed add-ins. It may be that an add-in installed in Outlook is causing the problem.
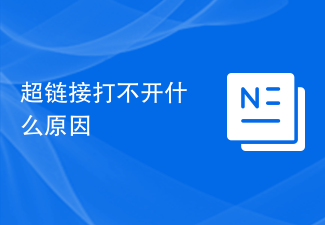
As one of the common elements in web pages, hyperlinks are often used to point to other web pages, files or specific locations. However, sometimes we encounter a situation where a hyperlink cannot be opened, which can be caused by a number of reasons. First, the failure to open a hyperlink may be caused by an incorrect link address. When creating a hyperlink, we need to make sure that the link's address is correct. If the address contains special characters or spaces, the link may not open. In addition, if the link address changes or the target file has been moved or deleted, the link will not be opened.
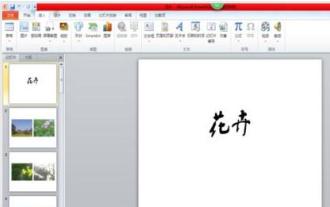
1. Open a PPT, or make a PPT that requires hyperlinks. 2. Select the content that needs to be inserted into a hyperlink. For example, we select the word "flower" and insert a hyperlink into it. 3. Click Insert in the menu bar, find the Hyperlink in the Link column, and click the hyperlink. 4. A window will appear, with the link on the left to select the location in this document, and then each slide in the PPT will appear. 5. You can select the slide you want to link, click once, and the preview mode of this slide will appear on the right. 6. With the hyperlink, the font color will change. We only need to click on the hyperlinked word when playing the slide, and the slide will automatically play to the slide we linked to.
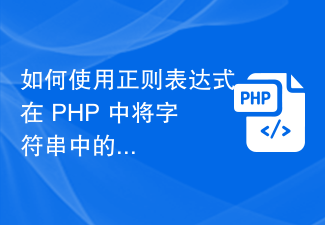
With the popularity of the Internet, people are increasingly inseparable from the Internet in their daily lives, and they increasingly need the support of web pages. In web pages, a very common operation is to convert the URL address into a hyperlink. In PHP, we can use regular expressions to achieve this operation. Next, let us take a look at the specific implementation method. 1. Use regular expressions to match URLs. Before using regular expressions to replace URLs with hyperlinks, we need to use regular expressions to match URLs. The specific matching rules are as follows: $patter
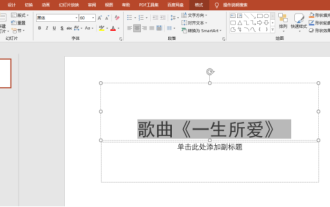
PPT hyperlinks can quickly go to a specified website or open a specified file, or jump directly to a certain page, improving efficiency and making playback more flexible. So, how do we set up hyperlinks? Here I will share with you how to set up hyperlinks in ppt. Next, learning is about to begin. Are you ready, students? The steps are as follows: 1. First, we need to open the Microsoft PowerPoint slide on the computer; then, we enter the text content we want to set a hyperlink in the slide. (As shown in the picture) 2. Next, we use the mouse to select the text content we want to set a hyperlink; then, we click the [right button] of the mouse and select the [Hyperlink] option in the [drop-down menu].
