


SOAP call implementation process under PHP5, php5soap call process_PHP tutorial
SOAP call implementation process under PHP5, php5soap call process
This article takes the iPhone 6 mobile phone reservation interface development of a company as an example to introduce the implementation process of SOAP call under PHP5.
1. Basic concepts
SOAP (Simple Object Access Protocol) Simple Object Access Protocol is a simple protocol for exchanging information in a decentralized or distributed environment. It is an XML-based protocol. It includes four parts: SOAP envelop (envelop), encapsulation Defines a framework that describes what is in the message, who sent it, who should accept and process it and how to process them; SOAP encoding rules (encoding rules), which are used to represent instances of the data types that the application needs to use; SOAP RPC representation represents the protocol for remote procedure calls and responses; SOAP binding uses the underlying protocol to exchange information.
WSDL (Web Service Description Language) is the standard XML format for describing XML Web services. WSDL was proposed by developers such as Ariba, Intel, IBM and Microsoft. It defines the relevant operations and messages sent and received by a given Web service in an abstract way that is independent of specific languages. By its definition, you cannot yet think of WSDL as an object interface definition language. For example, application architectures such as CORBA or COM will use object interface definition languages. WSDL remains protocol neutral, but it does have built-in support for binding to SOAP, thus establishing an inseparable link with SOAP. So, when I discuss WSDL in this article, I will assume that you use SOAP as your communication protocol.
Although SOAP and WSDL are two major standards for web services, they are not necessarily connected and can be used independently. The relationship between them is similar to the relationship between HTTP and Html. The former is a protocol, and the latter is a description of a Web Server.
2. Configuration under PHP5
In the php configuration file php.ini, find
extension=php_soap.dll
Then remove the “;” sign in front, and then restart the web service
3. Query web service methods, parameters, and data types
The order entry interface of a provincial telecommunications company is http://***.******.com/services/AcceptedBusiness?wsdl
We use SoapClient’s __geunctions() and __getTypes() Method View the methods, parameters and data types of the interface
Only the interfaces listed in __getFunctions can be called by soap.
Create the code soap.php in the root directory
<?<span>php </span><span>header</span>("content-type:text/html;charset=utf-8"<span>); </span><span>try</span><span> { </span><span>$client</span> = <span>new</span> SoapClient("http://***.******.com/services/AcceptedBusiness?wsdl"<span>); </span><span>print_r</span>(<span>$client</span>-><span>__getFunctions()); </span><span>print_r</span>(<span>$client</span>-><span>__getTypes()); } </span><span>catch</span> (SOAPFault <span>$e</span><span>) { </span><span>print</span> <span>$e</span><span>; } </span>?>
After running: http://localhost/soap.php in the browser, the return result is as follows
<span>Array</span><span> ( [</span>0] => ArrayOf_xsd_anyType introduceAcceptedBusiness(<span>string</span> <span>$c3</span>, <span>string</span> <span>$c4</span>, <span>string</span> <span>$linkman</span>, <span>string</span> <span>$linknum</span>, <span>string</span> <span>$num</span>, <span>string</span> <span>$idcard</span>, <span>string</span> <span>$remark</span>, <span>string</span> <span>$address</span><span>) [</span>1] => ArrayOf_xsd_anyType introduceAcceptedBusinessByAiZhuangWei(<span>string</span> <span>$subname</span>, <span>string</span> <span>$linkphone</span>, <span>string</span> <span>$idcard</span>, <span>string</span> <span>$address</span>, <span>string</span> <span>$businesstype</span>, <span>string</span> <span>$marketcode</span>, <span>string</span> <span>$surveycode</span>, <span>string</span> <span>$commanager</span>, <span>string</span> <span>$commanagerphone</span>, <span>string</span> <span>$bendiwang</span>, <span>string</span> <span>$fenju</span>, <span>string</span> <span>$zhiju</span>, <span>string</span> <span>$remark</span><span>) [</span>2] => <span>string</span> <strong>introduceAcceptedBusinessByStandardInterface</strong>(<span>string</span> <span>$xmlStr</span><span>) [</span>3] => <span>string</span> introduceAcceptedBusinessByCallOut(<span>string</span> <span>$xmlStr</span><span>) [</span>4] => <span>string</span> introduceAcceptedBusinessByYddj(<span>string</span> <span>$xmlParam</span><span>) [</span>5] => ArrayOf_xsd_anyType queryAcceptedBusinessByAiZhuangWei(<span>string</span> <span>$surveycode</span>, <span>string</span> <span>$starttime</span>, <span>string</span> <span>$endtime</span><span>) [</span>6] => <span>string</span> queryCallOutOrderByConfig(<span>string</span> <span>$xmlParam</span><span>) ) </span><span>Array</span><span> ( [</span>0] =><span> anyType ArrayOf_xsd_anyType[] )</span>
There is a method introduceAcceptedBusinessByStandardInterface(string $xmlStr), which will be the interface to be used mentioned in the development document, and the parameter is an xml string
In addition, some interfaces mention SoapHeader authentication, which requires adding the __setSoapHeaders method. For details, see http://php.net/manual/zh/soapclient.setsoapheaders.php
4. Submit order
This step is to splice the xml string according to the development document, and then pass it in as a parameter of introduceAcceptedBusinessByStandardInterface
Create acceptedbusiness.php with the following content
<?<span>php </span><span>header</span>("content-type:text/html;charset=utf-8"<span>); </span><span>try</span><span> { </span><span>$client</span> = <span>new</span> SoapClient('http://***.*******.com/services/AcceptedBusiness?wsdl'<span>); </span><span>$xml</span> = "<span> <?xml version='1.0' encoding='UTF-8' ?> <PACKAGE> <C3>**电信</C3> <C4></C4> <LINKMAN>张三</LINKMAN> <LINKNUM>13412341234</LINKNUM> <LINKADDRESS>广东深圳</LINKADDRESS> <REMARK>iPhone 6</REMARK> <CHANNEL></CHANNEL> <GRIDCODE>1111111111111111111111111111111</GRIDCODE> <AGENTCODE>2111</AGENTCODE> <KEY>1111111111111111111111111111111111111111111111111111111111111111111111111111111111111111111111111111111111111111111111111111111111111111111111111111111111111111111111111111</KEY> </PACKAGE> </span>"<span>; </span><span>$return</span> = <span>$client</span>->introduceAcceptedBusinessByStandardInterface(<span>$xml</span><span>); </span><span>print_r</span>(<span>$return</span><span>); } </span><span>catch</span> (SOAPFault <span>$e</span><span>) { </span><span>print_r</span>('Exception:'.<span>$e</span><span>); } </span>?>
After executing in the browser, return
<span><?</span><span>xml version="1.0" encoding="UTF-8"</span><span>?></span> <span><</span><span>PACKAGE</span><span>></span> <span><</span><span>STATUS</span><span>></span>0<span></</span><span>STATUS</span><span>></span> <span><</span><span>REASON</span><span>></span>入单成功!<span></</span><span>REASON</span><span>></span> <span><</span><span>ORDERSEQ</span><span>></span>2014100905523549742<span></</span><span>ORDERSEQ</span><span>></span> <span></</span><span>PACKAGE</span><span>></span>
One of the advantages of soap over nusoap is that it is developed in c and compiled into php internal function library, while NuSOAP is completely written in PHP language and consists of a series of PHP classes. The second advantage is that nusoap existed a long time ago and has stopped updating since 2005-07-27. Soap was added in the php5 version. With php6's support for webservice, I believe that the status of soap as a function library is certain. will keep rising.
The Soap function library of php5 is very convenient to use, and wsdl can be generated using the zend Development Environment development tool.
Note a few issues:
1. In order to improve efficiency, PHP provides a caching function for wsdl files. During development, you can use ini_set("soap.wsdl_cache_enabled", 0); to invalidate it, because the development process It is often necessary to modify wsdl files;
2. SOAP (Simple Object Access Protocol) Simple Object Access Protocol. In php5, it can not only provide the object setClass for remote access calls, but also provide the method addFunctions. So the 'O' in SOAP has been expanded.
3. The server may not be able to get the data POST from the client. This may be a bug in php5 soap functions; the solution is in the server example program below:
if ( isset($HTTP_RAW_POST_DATA)) {
$request = $HTTP_RAW_POST_DATA;
} else {
$request = file_get_contents('php://input');
}
The following is an example program source code.
soap client example:
ini_set("soap.wsdl_cache_enabled", 0);
try{
$soap = new SoapClient('authenticate/idolol.wsdl');
$soap->get_avatar(230);
$functions = $soap->__getFunctions();
print_r($functions);
$types = $soap->__getTypes();
print_r($types);
}catch(SoapFault $fault){
trigger_error("SOAP Fault: (faultcode: {$fault-> ;faultcode}, faultstring: {$fault->faultstring})", E_USER_ERROR);
}
?>
soap server example:
require './soap_functions.php';
ini_set("soap.wsdl_cache_enabled", 0);
$server = new SoapServer('authenticate /idolol.wsdl',array('encoding'=>'UTF-8'));
$server->addFunction(array("user_login","se...the rest of the text>>
There are many types of webservices, and only SOAP-type webservices are called using SoapClient.
In fact, SoapClient is an encapsulation of soap requests. You can also use the underlying interface to access, but this requires you to understand the SOAP protocol and is very troublesome.
There is a library called nusoap, which can easily write SOAP services and SOAP requests. You can learn about it.
Hope to adopt it, thank you for your support!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
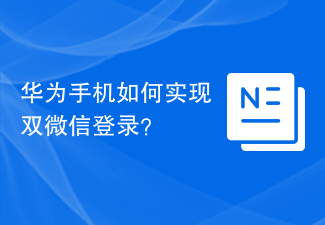
How to implement dual WeChat login on Huawei mobile phones? With the rise of social media, WeChat has become one of the indispensable communication tools in people's daily lives. However, many people may encounter a problem: logging into multiple WeChat accounts at the same time on the same mobile phone. For Huawei mobile phone users, it is not difficult to achieve dual WeChat login. This article will introduce how to achieve dual WeChat login on Huawei mobile phones. First of all, the EMUI system that comes with Huawei mobile phones provides a very convenient function - dual application opening. Through the application dual opening function, users can simultaneously
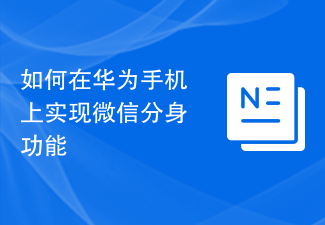
How to implement the WeChat clone function on Huawei mobile phones With the popularity of social software and people's increasing emphasis on privacy and security, the WeChat clone function has gradually become the focus of people's attention. The WeChat clone function can help users log in to multiple WeChat accounts on the same mobile phone at the same time, making it easier to manage and use. It is not difficult to implement the WeChat clone function on Huawei mobile phones. You only need to follow the following steps. Step 1: Make sure that the mobile phone system version and WeChat version meet the requirements. First, make sure that your Huawei mobile phone system version has been updated to the latest version, as well as the WeChat App.
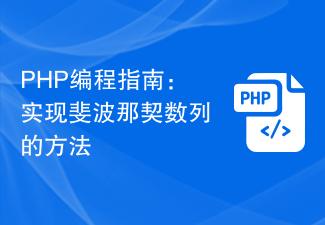
The programming language PHP is a powerful tool for web development, capable of supporting a variety of different programming logics and algorithms. Among them, implementing the Fibonacci sequence is a common and classic programming problem. In this article, we will introduce how to use the PHP programming language to implement the Fibonacci sequence, and attach specific code examples. The Fibonacci sequence is a mathematical sequence defined as follows: the first and second elements of the sequence are 1, and starting from the third element, the value of each element is equal to the sum of the previous two elements. The first few elements of the sequence
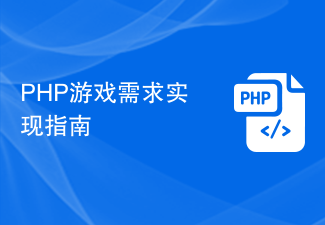
PHP Game Requirements Implementation Guide With the popularity and development of the Internet, the web game market is becoming more and more popular. Many developers hope to use the PHP language to develop their own web games, and implementing game requirements is a key step. This article will introduce how to use PHP language to implement common game requirements and provide specific code examples. 1. Create game characters In web games, game characters are a very important element. We need to define the attributes of the game character, such as name, level, experience value, etc., and provide methods to operate these
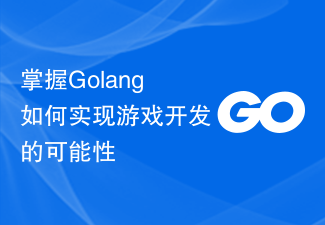
In today's software development field, Golang (Go language), as an efficient, concise and highly concurrency programming language, is increasingly favored by developers. Its rich standard library and efficient concurrency features make it a high-profile choice in the field of game development. This article will explore how to use Golang for game development and demonstrate its powerful possibilities through specific code examples. 1. Golang’s advantages in game development. As a statically typed language, Golang is used in building large-scale game systems.
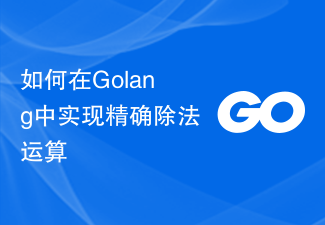
Implementing exact division operations in Golang is a common need, especially in scenarios involving financial calculations or other scenarios that require high-precision calculations. Golang's built-in division operator "/" is calculated for floating point numbers, and sometimes there is a problem of precision loss. In order to solve this problem, we can use third-party libraries or custom functions to implement exact division operations. A common approach is to use the Rat type from the math/big package, which provides a representation of fractions and can be used to implement exact division operations.
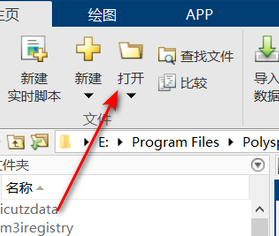
Many friends still don’t know how to call m files in matlab, so the editor below explains how to call m files in matlab. If you are in need, please take a look. I believe it will be helpful to everyone. 1. First open the matlab software and click "Open" in the main interface, as shown in the figure below. 2. Then select an m file that needs to be opened and select Open, as shown in the figure below. 3. Then look at the file name and number of variables of the m file in the editor, as shown in the figure below. 4. You can enter the m file name followed by the variable value in brackets on the command line to call it, as shown in the figure below. 5. Finally, the m file can be successfully called, as shown in the figure below. The above is the complete description of how to call m files in matlab brought to you by the editor.
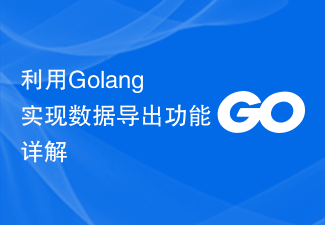
Title: Detailed explanation of data export function using Golang. With the improvement of informatization, many enterprises and organizations need to export data stored in databases into different formats for data analysis, report generation and other purposes. This article will introduce how to use the Golang programming language to implement the data export function, including detailed steps to connect to the database, query data, and export data to files, and provide specific code examples. To connect to the database first, we need to use the database driver provided in Golang, such as da
