PHP array function array_map() notes_PHP tutorial
PHP array function array_map() notes
Definition and usage
The array_map() function returns the array after the user-defined function is applied. The callback function should accept the same number of arguments as the number of arrays passed to the array_map() function.
Grammar
array_map(function,array1,array2,array3...)
参数 | 描述 |
---|---|
function | 必需。用户自定义函数的名称,或者是 null。 |
array1 | 必需。规定数组。 |
array2 | 可选。规定数组。 |
array3 | 可选。规定数组。 |
Example 1
<?php function myfunction($v) { if ($v === "Dog") { return "Fido"; } return $v; } $a = array("Horse", "Dog", "Cat"); print_r(array_map("myfunction", $a)); ?>
Output:
Array ( [0] => Horse [1] => Fido [2] => Cat )
Example 2
Use multiple parameters:
<?php function myfunction($v1, $v2) { if ($v1 === $v2) { return "same"; } return "different"; } $a1 = array("Horse", "Dog", "Cat"); $a2 = array("Cow", "Dog", "Rat"); print_r(array_map("myfunction", $a1, $a2)); ?>
Output:
Array ( [0] => different [1] => same [2] => different )
Example 3
Please see what happens when the custom function name is set to null:
<?php $a1 = array("Dog", "Cat"); $a2 = array("Puppy", "Kitten"); print_r(array_map(null, $a1, $a2)); ?>
Output:
Array (
[0] => Array ( [0] => Dog [1] => Puppy )
[1] => Array ( [0] => Cat [1] => Kitten )
)
Articles you may be interested in
- PHP array function array_walk() notes
- PHP generates continuous numeric (letter) array function range() analysis, PHP Lottery program function
- php pushes elements to the head of the array (usage of array_unshift)
- Using php functions in smarty templates and how to use multiple functions for one variable in smarty templates
- How PHP uses the filter function to verify email, url and IP address
- The difference between PHP merging array + and array_merge
- Introduction to the union, intersection and difference functions of arrays in PHP
- php finds whether a certain value exists in the array (in_array(), array_search(), array_key_exists())

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


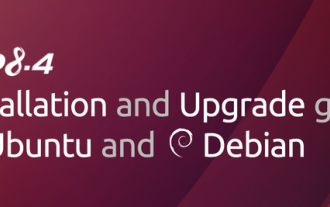
PHP 8.4 brings several new features, security improvements, and performance improvements with healthy amounts of feature deprecations and removals. This guide explains how to install PHP 8.4 or upgrade to PHP 8.4 on Ubuntu, Debian, or their derivati
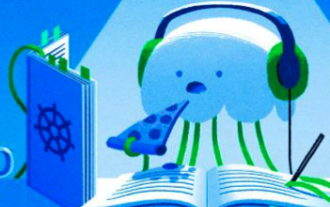
Visual Studio Code, also known as VS Code, is a free source code editor — or integrated development environment (IDE) — available for all major operating systems. With a large collection of extensions for many programming languages, VS Code can be c
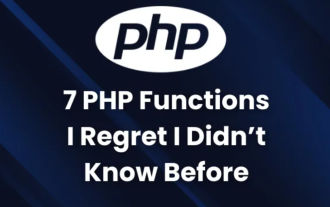
If you are an experienced PHP developer, you might have the feeling that you’ve been there and done that already.You have developed a significant number of applications, debugged millions of lines of code, and tweaked a bunch of scripts to achieve op
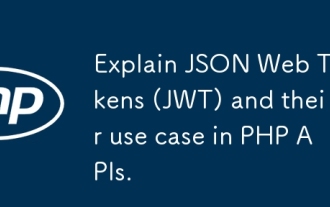
JWT is an open standard based on JSON, used to securely transmit information between parties, mainly for identity authentication and information exchange. 1. JWT consists of three parts: Header, Payload and Signature. 2. The working principle of JWT includes three steps: generating JWT, verifying JWT and parsing Payload. 3. When using JWT for authentication in PHP, JWT can be generated and verified, and user role and permission information can be included in advanced usage. 4. Common errors include signature verification failure, token expiration, and payload oversized. Debugging skills include using debugging tools and logging. 5. Performance optimization and best practices include using appropriate signature algorithms, setting validity periods reasonably,
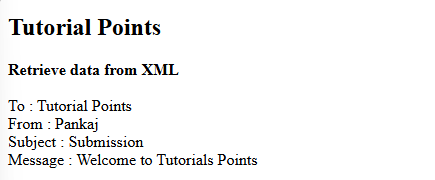
This tutorial demonstrates how to efficiently process XML documents using PHP. XML (eXtensible Markup Language) is a versatile text-based markup language designed for both human readability and machine parsing. It's commonly used for data storage an
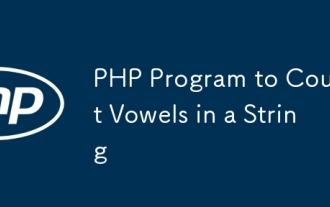
A string is a sequence of characters, including letters, numbers, and symbols. This tutorial will learn how to calculate the number of vowels in a given string in PHP using different methods. The vowels in English are a, e, i, o, u, and they can be uppercase or lowercase. What is a vowel? Vowels are alphabetic characters that represent a specific pronunciation. There are five vowels in English, including uppercase and lowercase: a, e, i, o, u Example 1 Input: String = "Tutorialspoint" Output: 6 explain The vowels in the string "Tutorialspoint" are u, o, i, a, o, i. There are 6 yuan in total
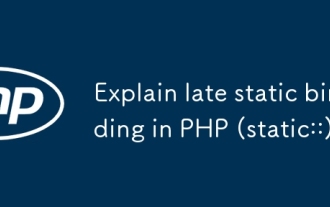
Static binding (static::) implements late static binding (LSB) in PHP, allowing calling classes to be referenced in static contexts rather than defining classes. 1) The parsing process is performed at runtime, 2) Look up the call class in the inheritance relationship, 3) It may bring performance overhead.

What are the magic methods of PHP? PHP's magic methods include: 1.\_\_construct, used to initialize objects; 2.\_\_destruct, used to clean up resources; 3.\_\_call, handle non-existent method calls; 4.\_\_get, implement dynamic attribute access; 5.\_\_set, implement dynamic attribute settings. These methods are automatically called in certain situations, improving code flexibility and efficiency.
