PHP Lesson 6 Usage of Arrays_PHP Tutorial
PHP Lesson 6 Usage of Arrays
Learning Summary:
* Understand the use of basic array functions
*Understand array traversal
*Understand the basic relationship and use of superglobal arrays
Array
1. Array definition and traversal2. Array function
Array definition:
$arr=array(1,2,3);//Index array, all subscripts are numbers
$arr=array("name"=>"user1","age"=>"30");//Associative array, the subscript contains letters
//There are only two kinds of subscripts, either letters or numbers without double quotes
<?php $arr=array("name"=>1,3,"age"=>4,5,100=>6,7,400=>8,9); echo "<pre class="code">"; print_r ($arr); echo "
Array subscript:
If it is a letter
$arr=array("name"=>1,3,"age"=>4,5,100=>6,7,400=>8,9);
//Subscript printing: "name" 0
[name] => 1
[0] => 3
[age] => 4
[1] => 5
[100] => 6
[101] => 7
[400] => 8
[401] => 9
Array value:
1. Output the entire array
print_r($arr)
2. Output a certain value in the array
$arr=array("name"=>1,3,"age"=>4,5,"100"=>6,7,"400"=>8,9); echo $arr['age']; echo "<br>"; echo $arr[100];
3. Array assignment:
1.$arr['age']=30;
Array assignment can also define arrays:
$arr[]=1;
$arr[]=2;
4. Array traversal:
1.for loop
<?php $arr[]=1; $arr[]=2; $arr[]=3; $arr[]=4; $arr[]=5; $arr[]=6; for($i=0;$i<5;$i++){ echo "<h1 id="第-i-个人的名字是-arr-i">第".($i+1)."个人的名字是{$arr[$i]}</h1>"; } ?>
Loop plus judgment:
<?php $arr[]=1; $arr[]=2; $arr[]=3; $arr[]=4; $arr[]=5; $arr[]=6; for($i=0;$i<5;$i++){ if($i%2==0){ echo "<h1 id="第-i-个人的名字是-arr-i">第".($i+1)."个人的名字是{$arr[$i]}</h1>"; }else{ echo "<h1 id="第-i-个人的名字是-arr-i">第".($i+1)."个人的名字是{$arr[$i]}</h1>"; } } ?>
2.foreach loop
foreach performs array traversal:
<?php //键值对 name="user1" 就是数组下标和值,key和value $arr['name']="junzai"; $arr['age']=20; $arr['sex']="man"; $arr[]="abc"; echo "<pre class="code">"; print_r ($arr); echo "
{$key}:{$val}
"; }else{ echo "{$key}:{$val}
"; } } ?>3.while....list ..each loop traversal
while(list($key,$val)=each($arr)){
echo $key.$val;
}
//It is recommended to use foreach to traverse the array
Multidimensional array:
1. One-dimensional array $arr=array(1,2,3);
$arr[0];
2. Two-dimensional array $arr=array(1,2,array(4,5));
$arr[2][0];
2. Two-dimensional array $arr=array(1,2,array(3,array(4,5)));
$arr[2][1][0];
Two-dimensional array traversal:
<?php header("content-type:text/html;charset=utf-8"); $arr=array("a","b",array("c","d"),array("e")); echo "<pre class="code">"; print_r($arr); echo "
"; foreach($arr as $val){ if(is_array($val)){ foreach($val as $val2){ echo $val2."
"; } } else{ echo $val."
"; } } ?>
Three-dimensional array value:
<?php header("content-type:text/html;charset=utf-8"); $arr=array("a","b",array("c","d"),array("e",array("f","z"))); echo "<pre class="code">"; print_r($arr); echo "
"; foreach($arr as $val){ if(is_array($val)){ foreach($val as $val2){ if(is_array($val2)){ foreach($val2 as $val3){ echo $val3."
"; } }else { echo $val2."
"; } } } else{ echo $val."
"; } } ?>
//It is recommended to use one-dimensional array and two-dimensional array
A data table is actually a two-dimensional array, and each row of records in it is a one-dimensional array
Query database:
<?php header("content-type:text/html;charset=utf-8"); mysql_connect("localhost","root","1234"); mysql_select_db("test"); mysql_query("set names utf8"); $sql = "select * from user"; $result = mysql_query($sql); $row1 = mysql_fetch_assoc($result); echo "<pre class="code">"; print_r($row1); echo "
Super global array:
Superglobal array
$_SERVER
$_GET
$_POST
$_REQUEST
$_FILES
$_COOKIES
$_SESSION
$GLOBALS
$_SERVER View server information
<?php header("content-type:text/html;charset=utf-8"); echo "<pre class="code">"; print_r($_SERVER); echo "
Apache/2.2.8 (Win32) PHP/5.2.6 Server at localhost Port 80
[SERVER_SOFTWARE] => Apache/2.2.8 (Win32) PHP/5.2.6
[SERVER_NAME] => localhost//Server domain name
[SERVER_ADDR] => 127.0.0.1//Server ip
[SERVER_PORT] => 80//Port number
[REMOTE_ADDR] => 127.0.0.1 //Client access ip
[DOCUMENT_ROOT] => E:/AppServ/www
[SERVER_ADMIN] => goxuexi@126.com
[SCRIPT_FILENAME] => E:/AppServ/www/index.php //The absolute path of the script file name
[REMOTE_PORT] => 49881
[GATEWAY_INTERFACE] => CGI/1.1
[SERVER_PROTOCOL] => HTTP/1.1
[REQUEST_METHOD] => GET
[QUERY_STRING] => //Request string
[REQUEST_URI] => ///Request url address
[SCRIPT_NAME] => /index.php//Script name (relative to website root directory)
[PHP_SELF] => /index.php
[REQUEST_TIME] => 1407568551//Access time
[argv] => Array
(
)
[argc] => 0
)
$_GET Get the data submitted with get
http://localhost/index.php?id=10&name=user1
Communication between two pages:
1. Form value
The first: get method
The second way: post method
2.a tag passing value
You can only use the get method
The a tag recommends using the get method to submit data
It is recommended to use post method to submit data
magic_quotes_gpc = on; means that when the get request is enabled, the ' in the get data will be preceded by
get instance:
index.php
<html> <head> <title> 接收信息 </title> </head> <body> junjun2<br> junzai3<br> junjun4<br> junjun5<br> </body> </html>
rev.php
<html> <head> <title> 接收信息 </title> </head> <body> <h1>欢迎: <?php echo $_GET['name'];?> </h1> <hr> <h1 id="姓名-php-echo-GET-name">姓名:<?php echo $_GET['name']?></h1> <h1 id="年龄-php-echo-GET-age">年龄:<?php echo $_GET['age']?></h1> </body> </html>
post实例
$_POST:获取表单post过来的数据
index.php
<html> <head> <title> 接收信息 </title> </head> <body> <h1 id="提交用户信息">提交用户信息</h1> </body> </html>
rev.php
<html> <head> <header content-type="text/html";charset="gbk"> <title> 接收信息 </title> </head> <body> <h1>欢迎: <?php echo $_POST['name'];?> </h1> <hr> <h1 id="姓名-php-echo-POST-name">姓名:<?php echo $_POST['name']?></h1> <h1 id="年龄-php-echo-POST-age">年龄:<?php echo $_POST['age']?></h1> </body> </html>
$_REQUEST
获取a或者表单get或post过来的数据.
$_COOKIES
同一个页面在多个页面获取
$_SESSION
同一个变量在多个页面获取到
$_FILES
获取表单中的文件,并生成一个数组.
$GLOBALS
$GLOBALS[_SERVER]
$GLOBALS[_GET]
$GLOBALS[_POST]
$GLOBALS[_FILES]
$GLOBALS[_REQUEST]
$GLOBALS[_COOKIES]
$GLOBALS[username]//里面包含页面内的全局变量,并且通过$GLOBALS[username]="user2"改变$username的值.
实例:使用$GLOBALS改变全局变量的值.
<?php $username111="user1"; function show(){ $GLOBALS[username111]="USER2"; } show(); echo $username111; echo "<pre class="code">"; print_r($GLOBALS); echo "
转载请注明出处: http://blog.csdn.net/junzaivip

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
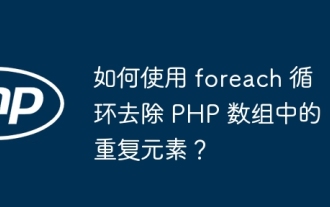
The method of using a foreach loop to remove duplicate elements from a PHP array is as follows: traverse the array, and if the element already exists and the current position is not the first occurrence, delete it. For example, if there are duplicate records in the database query results, you can use this method to remove them and obtain results without duplicate records.
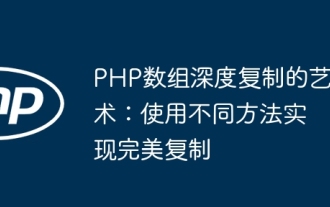
Methods for deep copying arrays in PHP include: JSON encoding and decoding using json_decode and json_encode. Use array_map and clone to make deep copies of keys and values. Use serialize and unserialize for serialization and deserialization.

The performance comparison of PHP array key value flipping methods shows that the array_flip() function performs better than the for loop in large arrays (more than 1 million elements) and takes less time. The for loop method of manually flipping key values takes a relatively long time.
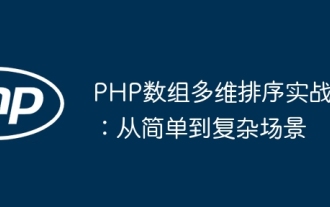
Multidimensional array sorting can be divided into single column sorting and nested sorting. Single column sorting can use the array_multisort() function to sort by columns; nested sorting requires a recursive function to traverse the array and sort it. Practical cases include sorting by product name and compound sorting by sales volume and price.
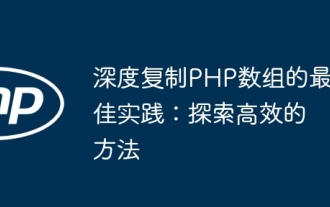
The best practice for performing an array deep copy in PHP is to use json_decode(json_encode($arr)) to convert the array to a JSON string and then convert it back to an array. Use unserialize(serialize($arr)) to serialize the array to a string and then deserialize it to a new array. Use the RecursiveIteratorIterator to recursively traverse multidimensional arrays.
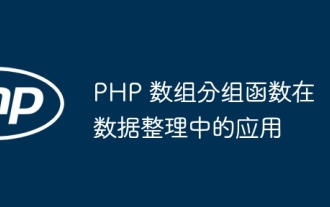
PHP's array_group_by function can group elements in an array based on keys or closure functions, returning an associative array where the key is the group name and the value is an array of elements belonging to the group.
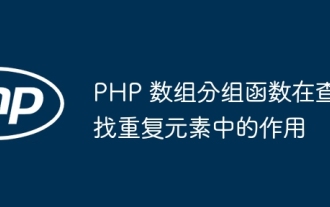
PHP's array_group() function can be used to group an array by a specified key to find duplicate elements. This function works through the following steps: Use key_callback to specify the grouping key. Optionally use value_callback to determine grouping values. Count grouped elements and identify duplicates. Therefore, the array_group() function is very useful for finding and processing duplicate elements.
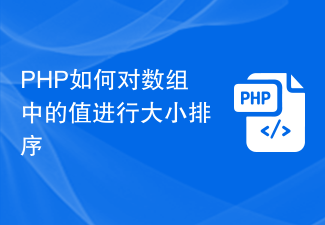
PHP is a commonly used server-side scripting language widely used in website development and data processing fields. In PHP, it is a very common requirement to sort the values in an array by size. By using the built-in sort function, you can easily sort arrays. The following will introduce how to use PHP to sort the values in an array by size, with specific code examples: 1. Sort the values in the array in ascending order:
