


A brief discussion on regular expressions, regular expressions_PHP tutorial
A brief talk about regular expressions, regular expressions
1. What is a regular expression?
Simply put: Regular expression (Regular Expression) is a language for processing string matching;
Regular expression describes a string matching pattern, which can be used to check whether a string contains a certain substring, and perform "removal" or "replacement" operations on the matched substring.
2. Application of regular expressions
Regular expressions are very practical in the actual development process and can quickly solve some complex string processing problems. Below I will make some simple classifications of the applications of regular expressions:
The first type: data verification
For example, if you want to verify whether a string is the correct EMail, Telphone, IP, etc., then it is very convenient to use regular expressions.
Second type: content search
For example, if you want to grab a picture from a web page, then you must find the tag. At this time, you can use regular expressions to accurately match it.
The third type: content replacement
For example, if you want to hide the middle four digits of your mobile phone number and change it to this pattern, 123****4567, then it will be very convenient to use regular expressions.
3. What are the contents of regular expressions
I will briefly introduce regular expressions below:
1. Several important concepts of regular expressions
- Subexpression: In a regular expression, if the content enclosed by "()" is used, it is called a "subexpression"
- Capture: The result matched by the subexpression will be placed in the buffer by the system. This process is called "capture"
- Back reference: We use "n", where n is a number, indicating the content between a certain buffer before the reference, we call it "back reference"
2. Quantity qualifier
- X+ means: 1 or more
- X* represents: 0 or more
- X? Represents: 0 or 1
- X{n} means: n
- X{n,} means: at least n
- X{n,m} means: n to m, greedy principle, will match as many as possible; if you add one at the end? , then it is the non-greedy principle
Note: X represents the character to be found
3. Character qualifier
- d means: match a numeric character, [0-9]
- D means: match a non-numeric character, [^0-9]
- w means: match word characters including underscores, [0-9a-zA-Z_]
- W means: matches any non-word character, [^0-9a-zA-Z_]
- s means: matches any whitespace character, space, carriage return, tab
- S means: match any non-whitespace character
- . Represents: Match any single character
In addition, there are the following:
Range characters: [a-z], [A-Z], [0-9], [0-9a-z], [0-9a-zA-Z]
Any character: [abcd], [1234]
Except characters: [^a-z], [^0-9], [^abcd]
4. Locator
- ^ represents: starting mark
- $ represents: ending mark
- b means: word boundary
- B represents: non-word boundary
5. Escape character
- Used to match certain special characters
6. Select matching character
- | Can match multiple rules
7. Special usage
- (?=): Forward lookup: match the string ending with the specified content
- (?!): Negative lookup: matches a string that does not end with the specified content
- (?:): Do not put the content of the selected match into the buffer
4. How to use regular expressions in Javascript
There are two ways to use regular expressions in Javascript:
First method: use RegExp class
The methods provided are:
- test(str): Whether there is a string matching the pattern in string matching, return true/false
- exec(str): Returns the string matched by the matching pattern. If yes, returns the corresponding string. If not, returns null;
// If there are subexpressions in the regular expression, when using the exec method
//What is returned is: result[0] = matching result, result[1] = matching result of sub-expression 1...
The second method is: use String class
The methods provided are:
- search: Returns the position where the string matching the pattern appears, if not, returns -1
- match: Returns the string matched by the matching pattern, if any, returns an array, if not, returns null
- replace: Replace the string matched by the matching pattern
- split: Separate the string with matching pattern as delimiter and return array
5. How to use regular expressions in PHP
There are two functions using regular expressions under PHP:
The first one is: Perl regular expression function
The methods provided are:
- preg_grep -- Returns the array elements matching the pattern
- preg_match_all -- perform global regular expression matching
- preg_match -- Regular expression matching
- preg_quote -- escape regular expression characters
- preg_replace_callback -- Use callback function to perform regular expression search and replacement
- preg_replace -- Perform regular expression search and replacement
- preg_split -- Split string using regular expression
The second one is: POSIX regular expression function
The methods provided are:
- ereg_replace -- Replace regular expression
- ereg -- Regular expression matching
- eregi_replace -- case-insensitive replacement regular expression
- eregi -- case-insensitive regular expression matching
- split -- Use regular expressions to split strings into arrays
- spliti -- Use regular expressions to split strings into arrays without case sensitivity
- sql_regcase -- Generate a regular expression for size-insensitive matching
6. Summary
Regular expression is a tool for us to implement a certain function. This tool:
1. Powerful functions
Different combinations of various qualifiers in regular expressions will achieve different functions. Sometimes to implement a complex function requires writing a long regular expression. How to achieve accurate matching will test the ability of a programmer. .
2. Simple and convenient
Usually when we search for string content, we can only search for a specific string, but regular expressions can help us perform fuzzy searches, which is faster and more convenient and only requires a regular expression string.
3. Basically all languages are supported
Currently mainstream languages such as JAVA, PHP, Javascript, C#, C++, etc. all support regular expressions.
4. Learning is easy, application is profound
Learning regular expressions is quick and easy, but how to write efficient and accurate regular expressions in actual development still requires a long period of trial and accumulation.
Regular expressions are often used in js to determine mobile phone numbers, email addresses, etc., to achieve powerful functions through simple methods
Symbol explanation
Character description
\ Mark the next character as A special character, or a literal character, or a backreference, or an octal escape character. For example, 'n' matches the character "n". '\n' matches a newline character. The sequence '\\' matches "\" and "\(" matches "(".
^ matches the beginning of the input string. If the Multiline property of the RegExp object is set, ^ also matches '\n' or ' The position after \r'.
$ matches the end of the input string. If the Multiline property of the RegExp object is set, $ also matches the position before '\n' or '\r'. subexpression zero or more times. For example, zo* matches "z" and "zoo". * is equivalent to {0,}. For example, 'zo+' matches "zo" and "zoo", but not "z". + is equivalent to {1,}? Matches the preceding subexpression zero or once. For example, "do(es". )?" can match "do" in "do" or "does". ? is equivalent to {0,1}.
{n} n is a non-negative integer. Matches a certain n times. For example, ' o{2}' cannot match 'o' in "Bob", but can match two o's in "food".
{n,} n is a non-negative integer, for example, '. o{2,}' cannot match 'o' in "Bob", but it can match all o's in "foooood". 'o{1,}' is equivalent to 'o{0,}' then. Equivalent to 'o*'.
{n,m} m and n are non-negative integers, where n ? When this The matching pattern is non-greedy when the character immediately follows any of the other qualifiers (*, +, ?, {n}, {n,}, {n,m}). The non-greedy pattern searches for as few matches as possible. string, while the default greedy mode matches as many of the searched strings as possible. For example, for the string "oooo", 'o+?' will match a single "o", while 'o+' will match all 'o's. '.
. Matches any single character except "\n". To match any character including '\n', use a pattern like '[.\n]'. y matches x or y. For example, 'z... The rest of the text >>
What is a regular expression? Give an example

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
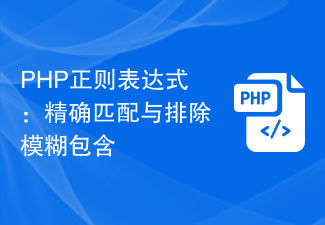
PHP Regular Expressions: Exact Matching and Exclusion Fuzzy inclusion regular expressions are a powerful text matching tool that can help programmers perform efficient search, replacement and filtering when processing text. In PHP, regular expressions are also widely used in string processing and data matching. This article will focus on how to perform exact matching and exclude fuzzy inclusion operations in PHP, and will illustrate it with specific code examples. Exact match Exact match means matching only strings that meet the exact condition, not any variations or extra words.
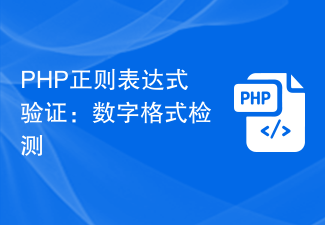
PHP regular expression verification: Number format detection When writing PHP programs, it is often necessary to verify the data entered by the user. One of the common verifications is to check whether the data conforms to the specified number format. In PHP, you can use regular expressions to achieve this kind of validation. This article will introduce how to use PHP regular expressions to verify number formats and provide specific code examples. First, let’s look at common number format validation requirements: Integers: only contain numbers 0-9, can start with a plus or minus sign, and do not contain decimal points. floating point
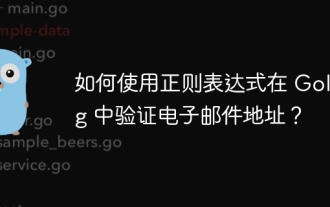
To validate email addresses in Golang using regular expressions, follow these steps: Use regexp.MustCompile to create a regular expression pattern that matches valid email address formats. Use the MatchString function to check whether a string matches a pattern. This pattern covers most valid email address formats, including: Local usernames can contain letters, numbers, and special characters: !.#$%&'*+/=?^_{|}~-`Domain names must contain at least One letter, followed by letters, numbers, or hyphens. The top-level domain (TLD) cannot be longer than 63 characters.
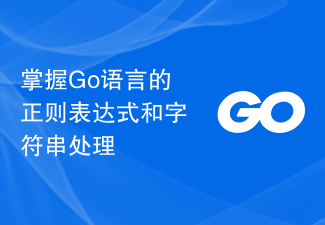
As a modern programming language, Go language provides powerful regular expressions and string processing functions, allowing developers to process string data more efficiently. It is very important for developers to master regular expressions and string processing in Go language. This article will introduce in detail the basic concepts and usage of regular expressions in Go language, and how to use Go language to process strings. 1. Regular expressions Regular expressions are a tool used to describe string patterns. They can easily implement operations such as string matching, search, and replacement.
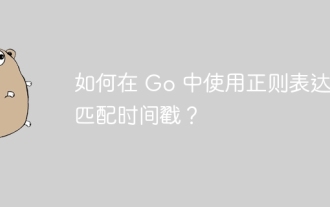
In Go, you can use regular expressions to match timestamps: compile a regular expression string, such as the one used to match ISO8601 timestamps: ^\d{4}-\d{2}-\d{2}T \d{2}:\d{2}:\d{2}(\.\d+)?(Z|[+-][0-9]{2}:[0-9]{2})$ . Use the regexp.MatchString function to check if a string matches a regular expression.
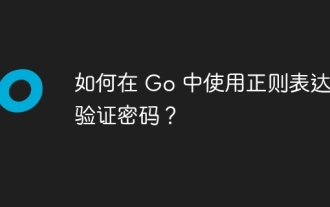
The method of using regular expressions to verify passwords in Go is as follows: Define a regular expression pattern that meets the minimum password requirements: at least 8 characters, including lowercase letters, uppercase letters, numbers, and special characters. Compile regular expression patterns using the MustCompile function from the regexp package. Use the MatchString method to test whether the input string matches a regular expression pattern.
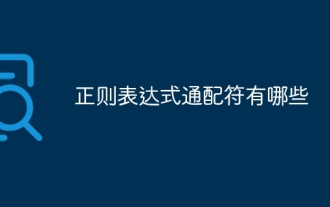
Regular expression wildcards include ".", "*", "+", "?", "^", "$", "[]", "[^]", "[a-z]", "[A-Z] ","[0-9]","\d","\D","\w","\W","\s&quo
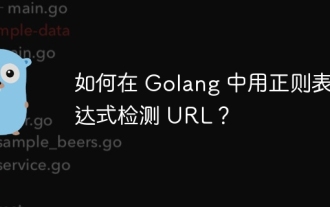
The steps to detect URLs in Golang using regular expressions are as follows: Compile the regular expression pattern using regexp.MustCompile(pattern). Pattern needs to match protocol, hostname, port (optional), path (optional) and query parameters (optional). Use regexp.MatchString(pattern,url) to detect whether the URL matches the pattern.
